Abstract Class is one of the fundamental concept of OOPS (Object Oriented Programming System)
and we use it to create Completely or Partially implemented Classes in C#, which give Game Developer such as us more control over how our Classes behaves.
In this Article, we will be going over Abstract Classes and Abstract Methods to talk about
- What Abstract Classes and Abstract Methods are
- Why you should be using Abstract Classes and Abstract Methods
- How to define Abstract Class and Abstract Methods when using C# and Unity
- Errors that you might face when defining Abstract Class and Abstract Methods
- and some other important points regarding Abstract Class and Abstract Methods in C#
So, without further ado, let’s get started!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Defining a Method is one of the most fundamental parts of programming and one that all the beginners need to have a strong grasp of.
Now because the Concept of Methods is so fundamental a lot of beginners just overview it without going deep enough
and skip the parts where they need to understand all the different sorts of things that they can do with Methods.
So, to help those who really want to have a strong base when programming their games, I’ve made this 4 Part Article Series on Methods
in which I’ve gone over everything about Methods that as a Game Developer you should know
to take full advantage of this wonderful feature that a lot of people overlook.
2 – Multiple, Required & Optional Parameters + Named Arguments
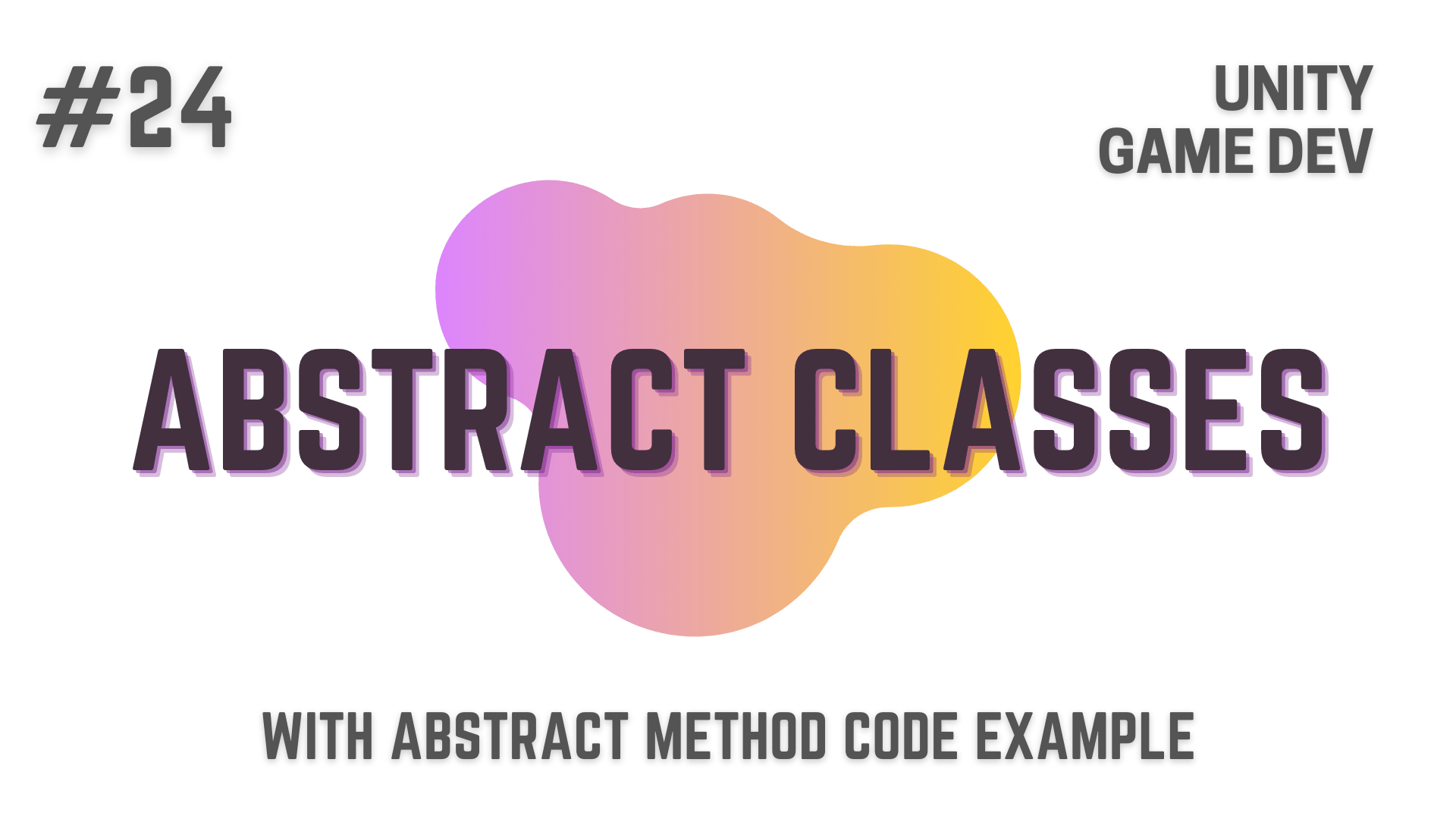
What Is An Abstract Class & Abstract Method in C# | Unity Game Development Tutorial
When you define a Class in C#, you can define variables and declare methods which have an implementation attached to it,
Now there are times when as a game developer you know that you will need to declare a particular method
but you don’t yet know how the implementation of this declared method will actually work.
For example, suppose you have guns in your game and you know that all your guns need to shoot bullets
So, knowing that you define a method named Shoot
but you get to thinking again and realize that you will have a ton of guns in your game, each having it’s own way of shooting bullets
and you don’t really know how each of these implementations will work.
Now you can use virtual and override keywords to solve this problem, as i have shown in the article about polymorphism
but if you do that you will still need to give an implementation for the Shoot method in the base class
which we don’t want to give because it is completely unnecessary
So to solve this issue and make your code Simpler, Cleaner and more Debuggable
we can use Abstract Classes to decouple the implementation of the method from the method declaration.
Defining Abstract Class and Abstract Method in C# | Code Example | Unity Game Development Tutorial
To give more context, let’s go to the code
Now, as we have multiple types of guns we will of course be creating a hierarchy,
where we will have a base class weapon_gun
and then different classes deriving from this base class for each sort of gun i.e. gun_bazooka & gun_uzi.
After that we will define the common variables and methods in the base class weapon_gun.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_gun : MonoBehaviour
{
protected int _ammo;
protected float _range;
// Start is called before the first frame update
void Start()
{
}
public void Shoot()
{
}
}
Now if we were just defining our base class like we normally do,
then we will have a code block attached to the Shoot method in the base class as shown in the code block above.
But if you think about it, we don’t really need an implementation for the Shoot method in the weapon_gun
as there is never going to be a gun in our game that is directly made using the weapon_gun base class
The base class is just going to act as a blueprint for the derived classes
and we will be using those derived classes to create all the different kinds of guns in the game, each with their own unique implementations.
Now, if we try removing the code block from the Shoot method directly
then we will get an compile time error as you can see in the image below
because normally you need to provide a code block when you declare a method, even if it’s just empty.
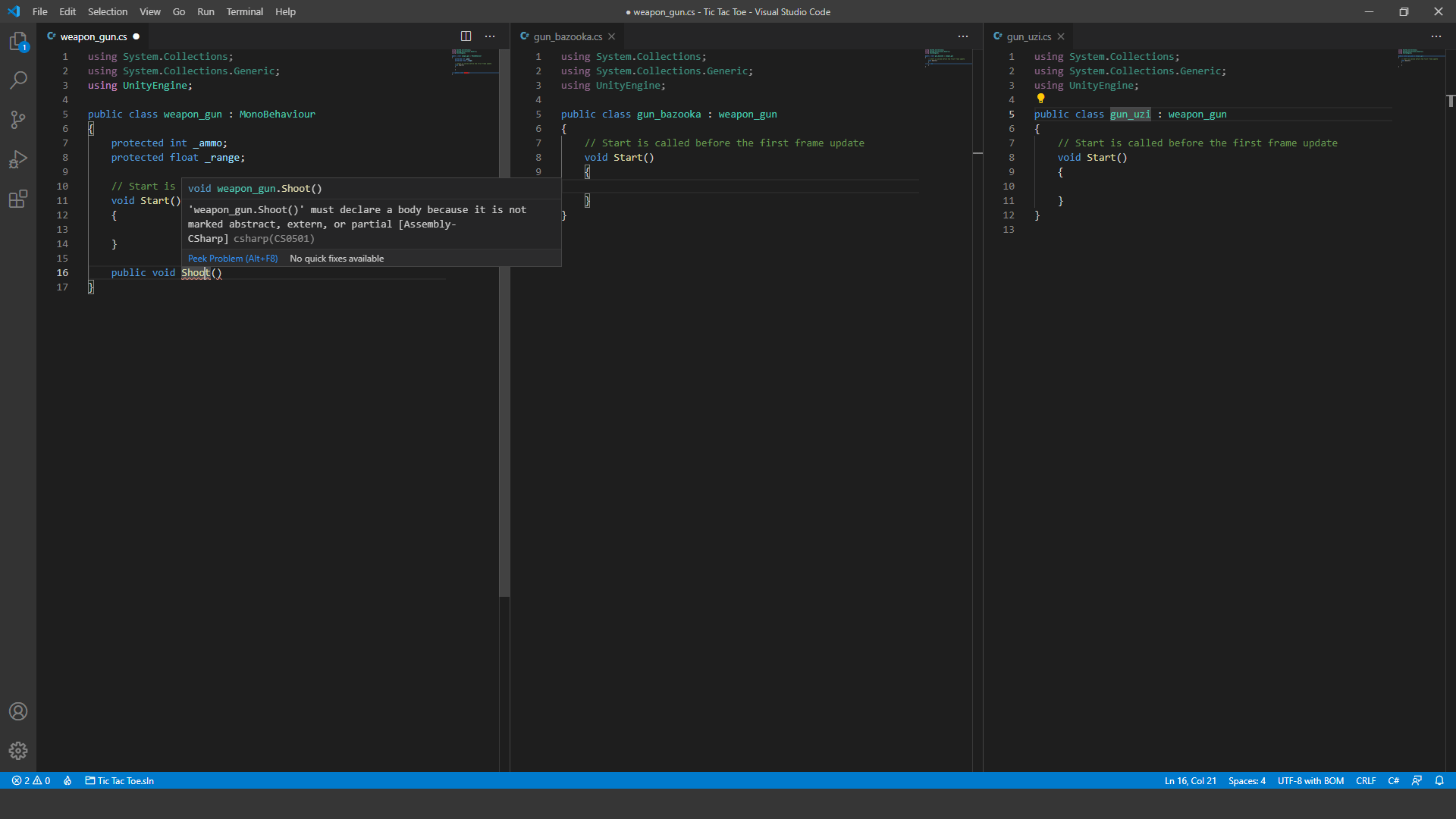
So to get past this issue, we will need to convert our weapon_gun class into an Abstract Class by using the abstract keyword when declaring it
as shown in the code block below.
and after that when declaring the Shoot method, we will use the abstract keyword to convert it into an Abstract Method
so that we don;t need to add the code block to it.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public abstract class weapon_gun : MonoBehaviour
{
protected int _ammo;
protected float _range;
// Start is called before the first frame update
void Start()
{
}
public abstract void Shoot();
}
By doing this, we will solve the compile time error that we are getting in the weapon_gun class
but we will start getting compile time errors in the gun_bazooka and gun_uzi Classes which are deriving from weapon_gun Class
If we hover over these error, then as you can see in the image below it says that
the class is not implementing the Shoot method that it has inherited from weapon_gun class
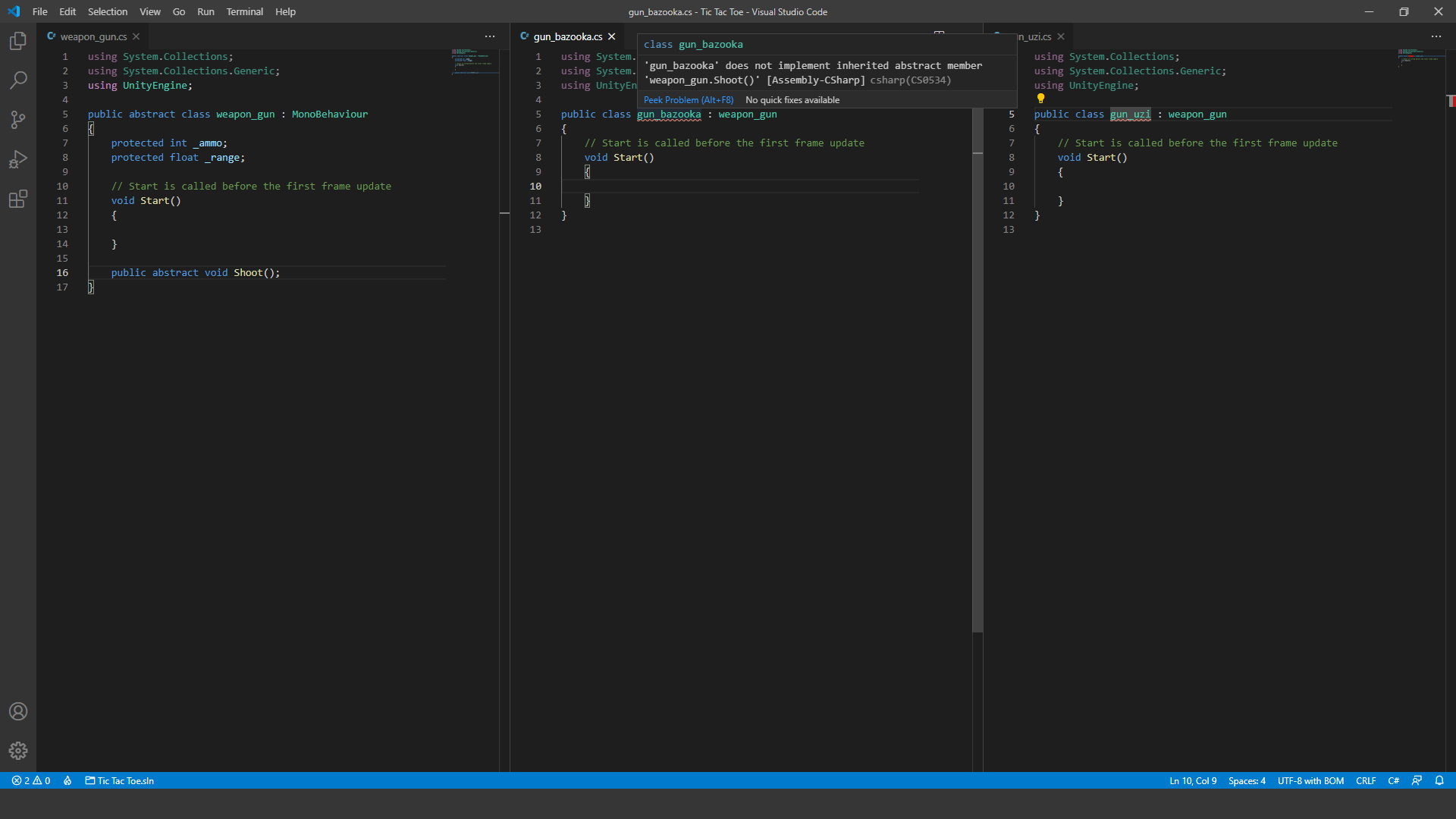
so we will need to implement the Shoot Method in both these Classes
and to do so we will need to use the override keyword.
For those who don’t know, the override keyword can be used to modify the implementation i.e. code block of an Inherited Method
as we have seen in the article about polymorphism, where we used it with Virtual Methods
So, let’s implement the shoot method in gun_bazooka and gun_uzi classes
To do this, you can either type out the method as shown below
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class gun_bazooka : weapon_gun
{
// Start is called before the first frame update
void Start()
{
}
public override void Shoot()
{
// Type Implimentation Here!
}
}
or if you are using a code editor with Intellisense,
then you can click on the error and a light bulb will pop up
then you can click on the light bulb and then click on Implement abstract class
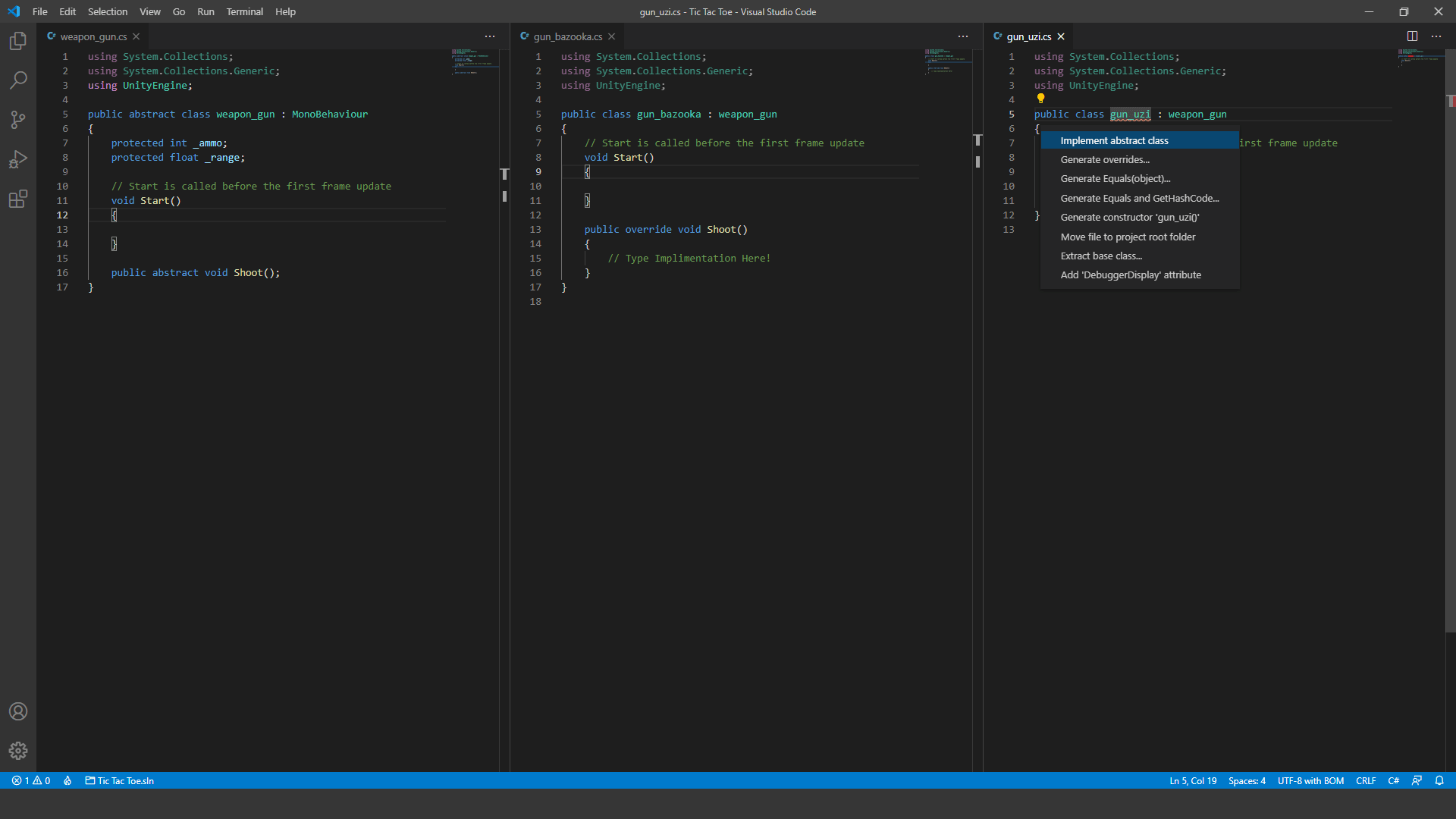
By doing so, VS Code will implement the code for you and you won’t need to type it out.
The auto implemented code will look something like shown below
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class gun_uzi : weapon_gun
{
public override void Shoot()
{
throw new System.NotImplementedException();
}
// Start is called before the first frame update
void Start()
{
}
}
With that done, you have successfully implemented an Abstract Class with an Abstract Method in C#.
Some Important Points about Abstract Class & Abstract Method in C# | Unity Game Development Tutorial
First, As Multiple Inheritance is not Supported in C#, you can only inherit from a single Class, Abstract or otherwise.
if that is not enough that you might consider using Interfaces as you can extend from multiple interfaces.
Second, You can’t create an instance i.e. an Object of an Abstract Class like you do for a normal Class
An Abstract Class is just to be used as a Base Class which contains common variables, abstract methods, concrete methods, etc that you want the Derived Classes to inherit.
Third, there is no need for an Abstract Class to contain Abstract Methods,
it is just fine if all it contains is Concrete Methods i.e. Method declarations with code block
Fourth, If you want to make even a single Abstract Method, then you need to convert your Class to an Abstract Class.
Fifth, You can choose to not provide implementation for an inherited Abstract Class in the Derived Class
by using the abstract keyword in the Derived Class again,
but there should come a point further down in your hierarchy where you provide the implementation.
Sixth, We should use Abstract Classes when things are connected in an hierarchy to each other via Inheritance
and that hierarchy is the limit for the declared Abstract Methods
as by design the concept of Abstract Classes is to declare or implement functionality that is common across a set of related classes
If this is not the case for what you are trying to do, then an Interface might be a better option.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords