In C# and all the other programming languages, there are special symbols and combination of symbols which which we use for creating conditional statements with one or more conditions or for reversing the output of a conditional calculation.
These symbols and combination of symbols are what we call Logical Operators in programming.
In the articles before, we have already talked about Arithmetic Operators, Assignment Operators and Comparison Operators.
In this article, we are going to cover Logical Operators.
So, let’s dive directly into it.
This Post is Part of : How To Make A Game Series where i’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
If you have not read the article on Intro To Game Development in which i introduce the Art of Game Development, Roles in Game Development and your Recipe for Success as a Game Developer to you, you can read that article next, as i can’t emphasize how necessary it is and how helpful it will be for you to have the right mindset required to learn how to make a game and become an exceptional Game Developer.
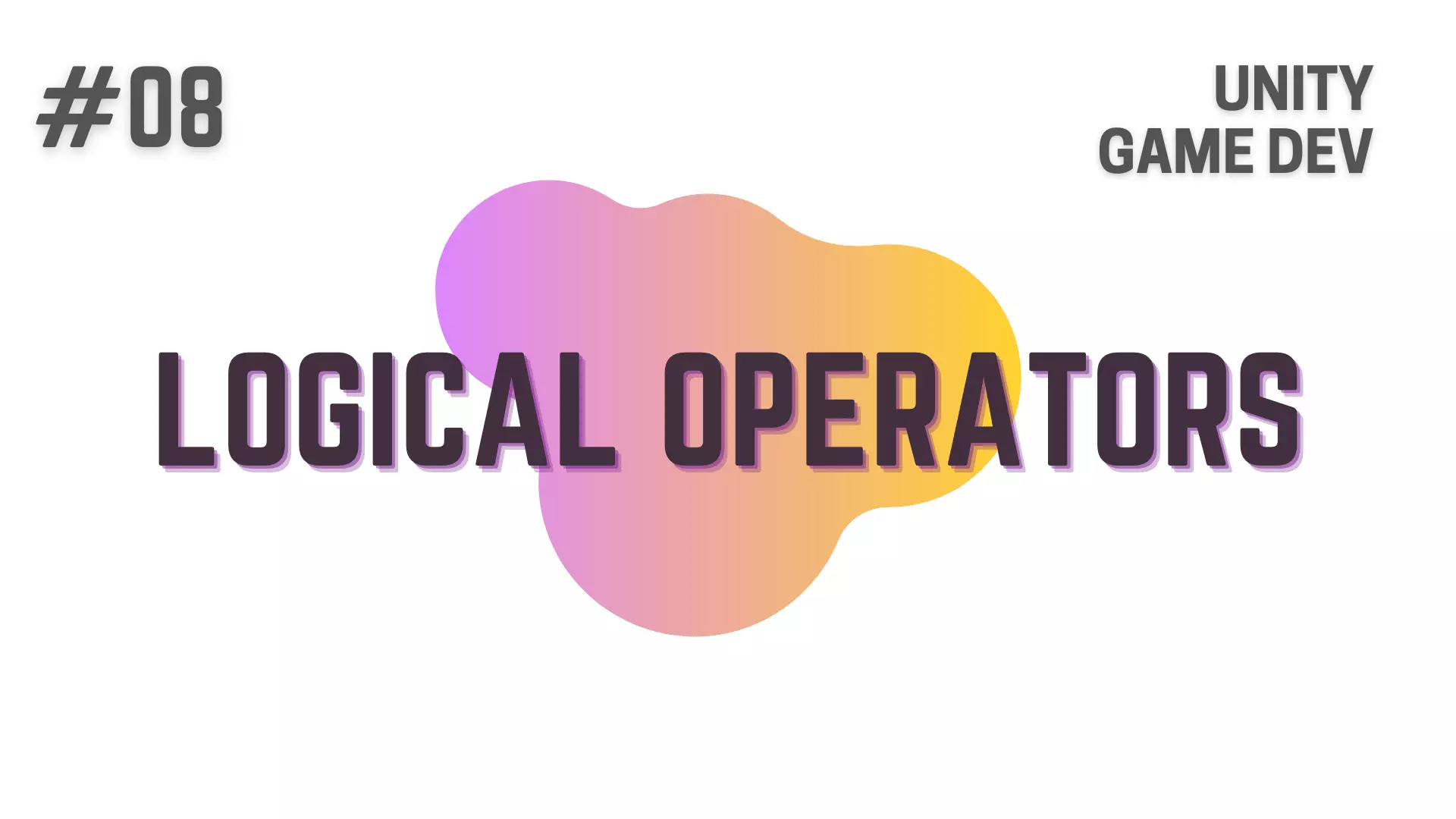
Articles Related To Operators in C#
Logical Operators
Moving Forward, now let’s talk about Logical Operators
that we will be using to compare data inside a variable with other variables or with a hard-coded value.
There are 3 Logical Operators that will be of immense use to you as a Game Developer :
- Logical AND Operator ( && )
- Logical OR Operator ( || )
- Logical NOT Operator ( ! )
Logical AND Operator | Double Ampersand Operator ( && )
// Everything Below is inside a Class.
private int _playerHP = 300;
private int _enemyHP = 500;
void Start()
{
if(_playerHP == 300 && _enemyHP < 450)
{
// These Logs will not be printed.
Debug.log("Player HP : " + _playerHP);
Debug.log("Enemy HP : " + _enemyHP);
}
}
The first operator is the Logical AND Operator ( && ) which you can use by typing the ampersand symbol twice.
When we use the Logical AND Operator ( && ) then all the conditions that we join using it, will have to be true
for the code inside the conditional block to be executed
if even a single one of the conditions was not met i.e the condition returned false,
then the code inside the conditional block will not be executed.
Logical NOT Operator | Double Pipeline Operator ( || )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(_playerHP == 300 || _enemyHP < 450)
{
// These Logs will be printed.
Debug.log("Player HP : " + _playerHP);
Debug.log("Enemy HP : " + _enemyHP);
}
}
Next is the Logical OR which can used by typing the Pipeline symbol twice
When we use the Logical OR Operator ( || ), any one of the conditions that we join using it, will have to be true
for the code inside the conditional block to be executed
if none of the conditions that you joined with the Logical OR operator were met
then the code inside the conditional block will not be executed.
Logical NOT Operator ( ! )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(!(_playerHP == 300 && _enemyHP < 450))
{
// These Logs will be printed.
Debug.log("Player HP : " + _playerHP);
Debug.log("Enemy HP : " + _enemyHP);
}
}
and the last one is the Logical Not Operator ( ! )
which you can use by prefixing an exclamation mark to the equation.
Logical NOT operator ( ! ) is used to reverse the output of any condition.
So, if the output of a condition or a compound condition, returns true
and you have prefixed the condition with the Logical NOT operator
then the program will reverse the output of the equation
and instead of true it will return false.
RPG Analogy
Going to the RPG Analogy,
we are going to use the same example that we used in the article about Comparison Operators
albeit we are just going to change some variable names and values.
So, think about a town in your game,
with shops that the player can go in an out of during a certain period of time,
lets say from 10 am to 7 pm.
Now to do this, we will have to define a boolean to set the state of the door like
private bool _isDoorOpen;
Next, in the script which manages game world time and day night cycle,
when the time hits 10 am we will set the _isDoorOpen boolean to true.
and at 7 pm we will set the _isDoorOpen boolean to false.
After that, in the script that allows the player to move in and out of the shops,
you can use this boolean and create a condition
which will only allow the player to enter the shop if _isDoorOpen is true
and will not allow the player to enter the shop if _isDoorOpen is false.
Now, just like in the article about Comparison Operators
we are not going to make a day and night cycle script
what we are going to instead is we are going to put all the code in a single C# script
and hard code the current game time in it, so that we can test out our logic.
Code Example
Note: In the code below I’m using the 24 Hour Time cycle.
// Everything Below is inside a Class.
private bool _isDoorOpen;
private byte _currentGameHour = 7;
private byte _shopOpeningHour = 10;
private byte _shopClosingHour = 19;
// This method is called on every single frame.
void Update()
{
// Section 1.
if(_currentGameHour >= _shopOpeningHour && _currentGameHour < _shopClosingHour)
{
_isDoorOpen = true;
}
else
{
_isDoorOpen = false;
}
// Section 2
if(_isDoorOpen == true)
{
Debug.Log("The Player Is Allowed To Enter The Shop");
}
else if(_isDoorOpen != true)
{
Debug.log("The Player Is Not Allowed To Enter The Shop");
}
else
{
Debug.log("Something Went Wrong.");
}
}
In the Code Example above,
First we have declared the variables and hard-coded the values
After that in Section 1, we are trying to determine whether to set the _isDoorOpen variable to true or false
based on the Compound Condition that we have created
using the Logical AND Operator ( && ) and two Comparison Operators.
Next, in Section 2, we are using the value inside _isDoorOpen variable, that have set in Section 1
to determine whether we will allow the Player to enter the Shop
or will we stop the Player from entering the Shop.
On top of that, if for some reason _isDoorOpen variable is undefined
that is, we have not set it’s value
then we will print “Something Went Wrong.” in the Unity Console.
So, if we execute this piece of code in the Unity Console,
As we have set _currentGameHour to 7 i.e. 7 AM
and also set the Shop to be open between 10 AM & 7 PM
The player will not be allow to enter the shop.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords