In the previous article, we talked about For loops and came to a clear understanding on how and when you should be using For loop.
Now, Foreach Loop, just like the For Loop is used to repeatedly execute a block of code.
In this article, we will be going through everything about Foreach Loops
to understand the differences between For Loops & Foreach Loops
and use Real World RPG Scenario as base to write a small piece of code for an AOE Attack.
So without wasting any time, let’s get started!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Game Development can a long and arduous process in which you have to keep on modifying and improving you game continuously
but if you made a mistake when picking the core game idea,
you can be in for a hellish torment which might lead you towards dropping your game completely
So, to stop that from happening to you, I wrote this article on 3 Points To Consider Before Committing To A Game Idea
which contains some points and questions you should ask yourself before you make your final decision of choosing the game idea or dropping it.
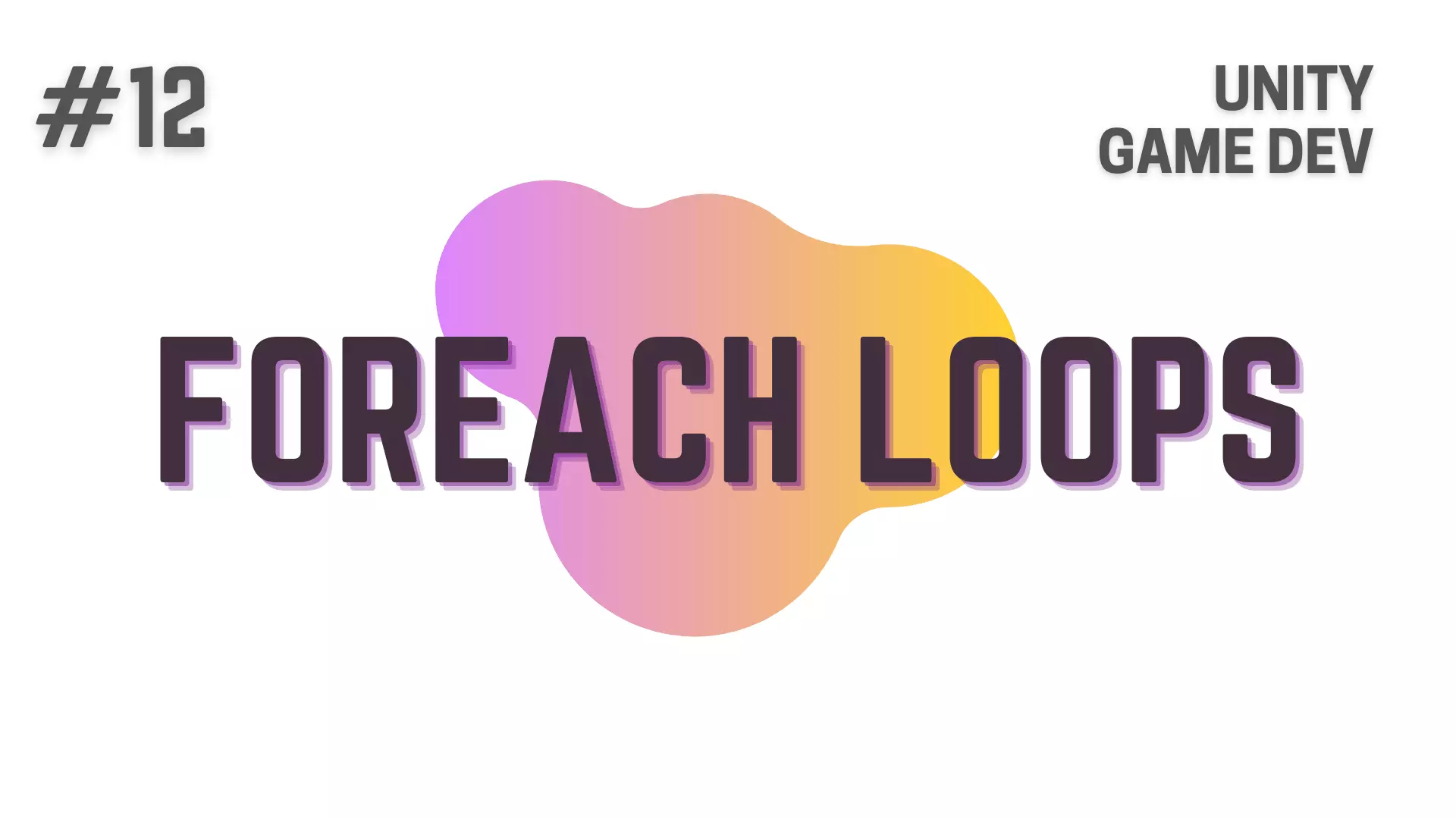
List Of Other Articles on Loops In C#
Difference between For Loop & Foreach Loop
Now since For Loops and Foreach Loops are so similar
of course there has to be some differences between them.
The First difference is that, Foreach Loop can be less intimidating to beginners
as when they first look at it the loop syntax for foreach loop almost looks like an English sentence.
on the other hand, For Loop can be intimidating to beginners
because it looks like you are going to start doing some math problem.
This of course makes most people feel at home when using Foreach Loop
and within seconds they fall in love with it’s simplicity
and throw the For Loop in the back closet and forget about it.
There is a Right Tool For Every Job
Now some of you might have heard the saying that there is a right tool for every job
And this is quite true for this case but there is a caveat
and that is, it depends on the person doing the job what the right tool is
every coder will have his/ her idiosyncrasy
and even though it does matter which loops you use where
it’s a matter of optimization
and as a complete beginner, at least until you have strong enough base
you don’t need to think about which loop to use
and instead, just use whichever one you feel more comfortable with.
if you want to know my idiosyncrasy
When working with things that have indexes, like Arrays
i like using For Loop as for me it’s just easier.
While when working with Lists, Dictionaries or Collections, i use Foreach Loop
as it does a much better job for that workload for me.
So when and how to use either of these loops
is really dependent on the coder and what they are comfortable using
Sadly, For Loop just looks more intimidating to newcomers
and a lot of beginners might find it a bit cumbersome to use as opposed to the Foreach Loop.
So, they just never try using it.
The Next difference is that,
For loop needs us to tell while setting it up that where it starts and where it ends
On the other hand, Foreach Loop just like it’s name suggests
loops through Each and Every Element of any Array, List, Dictionary or Collection that you are iterating over.
Another difference is that
in For Loop you can initialize multiple variables when you setup the loop
while this is not possible in Foreach Loop.
Syntax To Write / Code A Foreach Loop in C# Unity
// Everything below is just a placeholder code to understand syntax.
// For working example go further down in the article.
foreach(DataType VariableName in Array / List/ Dictionary / Collection)
{
// Code Block.
}
With that done, let’s move on to the Syntax of a Foreach Loop and break it down into sections.
first of all we have the Foreach Keyword and the two Opening and Closing parenthesis
which tells the compiler that we are writing a Foreach Loop.
After that, we have the Data Type and Name of the Local Variable that we are declaring
which will be local to the Foreach Loop and will not be accessible outside this Foreach Loop.
This local variable is what we would be using to store the current member that we are accessing
from the Array, List, Dictionary or Collection that we are iterating over.
So, if we are iterating over a string array
then the data type that we should use for the local variable is string.
now instead of strongly typing the datatype like this
you can also use the var Keyword and let the compiler decide on the suitable data type for your local variable
based on the data that you are placing inside it.
If you use the var keyword,
you should make sure to name the variable correctly with a descriptive name
so that you can infer what data is inside the variable is
As, if it is not named correctly it will be really bothersome to decipher what this variable refers to.
Moving forward, now comes the The third section
which is the array, list, dictionary or collection that you are going through
And the final section is everything inside the curly braces that is you code block.
And That’s the Syntax of a Foreach Loop.
Follow Naming Convention When Coding Foreach Loops Or Anything Else For Your Game
Now Moving Forward, Another important thing to remember is that,
In C#, var is strongly typed i.e. Once it is declared and initialized, it’s data type cannot be changed.
There is a huge debate on whether you should use or not use the var keyword and to that i would say
do what you are comfortable with if you are working on the project alone
but of course don’t forget to follow a rough naming convention and coding style
so that it is easy for you to go through your own code.
While if you are working in a team, make a guideline on how things will be named
and whether all of you will be clearly mentioning the data types or not.
Make this guideline rigid and follow it
because now there are too many cooks working on this project
and if you don’t do it, there will definitely be trouble down the road.
These sort of guidelines and conventions are important and will help maintain consistency
so that anyone on your team with programming knowledge can read through the code and understand it.
or if a new person joins the team
all you need to do is give him/her this piece of guidelines and conventions
and they can be up and running quickly.
RPG Analogy For Foreach Loop
Now let’s move on to my favorite part of the article it’s RPG analogy time.
For today’s analogy, let’s go with something that’s a bit smaller than the one in the article of For Loops.
So, Think about a battle scene in your game
where the player and his allies are fighting with enemies
while neutrals are waiting on the sidelines.
Now, the player wants to use an Area of Effect or AOE attack
but let’s say that the AOE Attack is kinda sentient and will only attack the Enemies
So what we have to do as the God of Creation
is to write the code that will find all the enemies within the range of our AOE attack and inflict damage on them
while not inflicting any damage to neutrals and allies.
That’s it.
What Will We Need To Code This RPG Analogy?
Now to test this scenario, we would first need an array that contains names of the factions
then another array which will contain all the npcs
We will giving the npcs unique names here and will suffix the names with their factions.
By doing this we won’t need to create another array
to store whether the npc is an ally or enemy or neutral.
next we will create another array that will store whether that npc is within the AOE attacks range or not
With just this three array and a couple of variables we should be able to code this AOE Attack.
So, let’s get started with the code.
Displaying Names Of The 3 Factions Using Foreach Loop
First of all let’s display the names of the 3 factions.
To do that, let’s declare a string array named _factions with 3 members
and set the values to .Ally, .Enemy and .Neutral, like this
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
After that let’s go the the Start() Method and create a Foreach Loop.
inside the brackets we will use the var keyword to initialize a local variable named faction
that will contain the currently iterating member data from _factions array
Now inside the curly braces, let’s create a decision making block using an if statement,
with the condition being
faction == “.Ally”
if this returns true
then we’ll log : “Ally Faction.”, in the Unity Console
Next is the else if block with the condition being that
faction == “.Enemy”
if this returns true
then Debug.Log : “Enemy Faction.”
And the last else if block with the condition being
faction == “.Neutral”
if this returns true
then Debug.log : “Neutral Faction.”
And that’s it
Now let’s execute this piece of code in Unity and check the logs
Executed Code | Displaying Faction Names Using Foreach Loops In C#
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
void Start()
{
foreach(var faction in _factions)
{
if(faction == ".Ally")
{
Debug.Log("Ally Faction.");
}
else if(faction == ".Enemy")
{
Debug.Log("Enemy Faction.");
}
else if(faction == ".Neutral")
{
Debug.Log("Neutral Faction.");
}
}
}
Unity Log | Displaying Faction Names Using Foreach Loops In C#
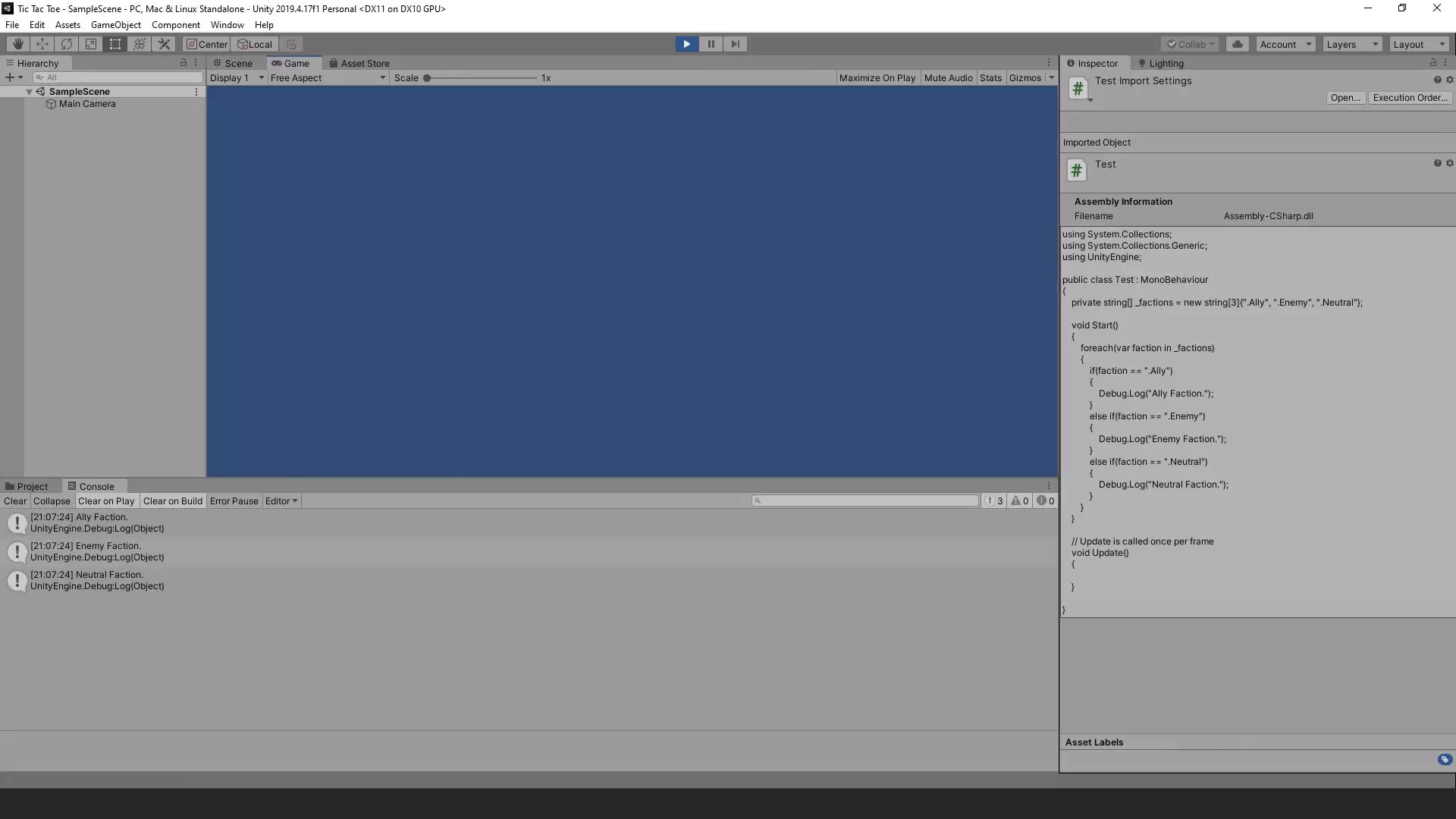
As you can see we logged the 3 factions as expected.
Foreach Loop Code Example For A Sentient AOE Attack
Declaring And Initializing _npcs and _withinAORRange Arrays
Now i don’t think that i need to break this piece of code down as it’s pretty simple
so let’s move forward and complete the whole code for our AOE Attack.
To do this, first we need to create a string array named _npcs
and a bool array named _withinAOERange both with 7 members
and set values to those members, like this
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
private string[] _npcs = new string[7]{"Zane.Ally", "Connor.Enemy", "Delilah.Neutral", "Deborah.Ally", "Seth.Enemy", "Alex.Neutral", "Wolf.Enemy"};
private bool[] _withinAOERange = new bool[7]{true, true, false, false, false, true, true};
void Start()
{
foreach(var faction in _factions)
{
if(faction == ".Ally")
{
Debug.Log("Ally Faction.");
}
else if(faction == ".Enemy")
{
Debug.Log("Enemy Faction.");
}
else if(faction == ".Neutral")
{
Debug.Log("Neutral Faction.");
}
}
}
Coding A Nested Foreach Loop To Iterate Over The Array Containing All THe NPCS
Now in the Start() Method lets create 3 variables all of data type int
with names allyFound, enemyFound and neutralFound.
and set all of their values to 0.
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
private string[] _npcs = new string[7]{"Zane.Ally", "Connor.Enemy", "Delilah.Neutral", "Deborah.Ally", "Seth.Enemy", "Alex.Neutral", "Wolf.Enemy"};
private bool[] _withinAOERange = new bool[7]{true, true, false, false, false, true, true};
void Start()
{
int allyFound = 0;
int enemyFound = 0;
int neutralFound = 0;
foreach(var faction in _factions)
{
if(faction == ".Ally")
{
Debug.Log("Ally Faction.");
}
else if(faction == ".Enemy")
{
Debug.Log("Enemy Faction.");
}
else if(faction == ".Neutral")
{
Debug.Log("Neutral Faction.");
}
}
}
With that done inside the if block of Ally Faction,
create another int named i and set it to 0.
after that create a foreach loop to iterate over _npcs array
and inside the curly braces create an if statement with a compound condition
where the first condition is
npc.EndsWith(faction)
this means that,
if the string inside the npc variable has dot Ally at it’s end, then it will return true
otherwise, it will return false
and the second condition is
_withinAOERange[i] == true
which is us checking whether the npc is within the AOE attack’s range or not
Now To make this Compound condition
we will use the logical AND operator to join both of these conditions
so that only if both of these conditions return true
will the code within this if block be executed
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
private string[] _npcs = new string[7]{"Zane.Ally", "Connor.Enemy", "Delilah.Neutral", "Deborah.Ally", "Seth.Enemy", "Alex.Neutral", "Wolf.Enemy"};
private bool[] _withinAOERange = new bool[7]{true, true, false, false, false, true, true};
void Start()
{
int allyFound = 0;
int enemyFound = 0;
int neutralFound = 0;
foreach(var faction in _factions)
{
if(faction == ".Ally")
{
Debug.Log("Ally Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
// Next we will Code This.
}
}
}
else if(faction == ".Enemy")
{
Debug.Log("Enemy Faction.");
}
else if(faction == ".Neutral")
{
Debug.Log("Neutral Faction.");
}
}
}
now if we are inside the if block
that means that we have found an ally
so let’s increase the value of allyFound by 1
and then Debug.Log the Found Ally in the Unity Console
and after the if statement,
increase the value of i by 1
so that we would be able to get
the correct data from _withinAOERange array
for the next npc in the next loop cycle
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
private string[] _npcs = new string[7]{"Zane.Ally", "Connor.Enemy", "Delilah.Neutral", "Deborah.Ally", "Seth.Enemy", "Alex.Neutral", "Wolf.Enemy"};
private bool[] _withinAOERange = new bool[7]{true, true, false, false, false, true, true};
void Start()
{
int allyFound = 0;
int enemyFound = 0;
int neutralFound = 0;
foreach(var faction in _factions)
{
if(faction == ".Ally")
{
Debug.Log("Ally Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
allyFound = allyFound + 1;
Debug.Log("Ally " + allyfound + " " + npc + "HP : 100 - 0 = 0");
}
i++;
}
}
else if(faction == ".Enemy")
{
Debug.Log("Enemy Faction.");
}
else if(faction == ".Neutral")
{
Debug.Log("Neutral Faction.");
}
}
}
With that done,
Now let’s copy and paste everything that we did inside the Ally Faction’s if block
into the Neutral and Enemy Faction’s if block
and change every place that we have written ally
to neutral in the neutral faction’s if block
and to enemy in the enemy faction’s if block, like this
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
private string[] _npcs = new string[7]{"Zane.Ally", "Connor.Enemy", "Delilah.Neutral", "Deborah.Ally", "Seth.Enemy", "Alex.Neutral", "Wolf.Enemy"};
private bool[] _withinAOERange = new bool[7]{true, true, false, false, false, true, true};
void Start()
{
int allyFound = 0;
int enemyFound = 0;
int neutralFound = 0;
foreach(var faction in _factions)
{
if(faction == ".Ally")
{
Debug.Log("Ally Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
allyFound = allyFound + 1;
Debug.Log("Ally " + allyFound + " " + npc + "HP : 100 - 0 = 0");
}
i++;
}
}
else if(faction == ".Enemy")
{
Debug.Log("Enemy Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
enemyFound = enemyFound + 1;
// This Debug.Log will be modified next as the Enemy will suffer damage.
Debug.Log("Enemy " + enemyFound + " " + npc + "HP : 100 - 0 = 0");
}
i++;
}
}
else if(faction == ".Neutral")
{
Debug.Log("Neutral Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
neutralFound = neutralFound + 1;
Debug.Log("Neutral " + neutralFound + " " + npc + "HP : 100 - 0 = 0");
}
i++;
}
}
}
}
We are able to do this because
the only difference that will be between the factions
will be inside the innermost if block
and the code structure will remain the same before it
with only the names of member counting variables and Debug.log statements
being different between the factions.
Next, inside the innermost if block of the enemy faction’s if block
we have incremented the enemyfound variable by 1.
Now we need to apply damage to the enemies HP
So, let’s create another integer variable named randomDamage
and use Random.Range() to generate a random number between 10 and 100.
After that we will also modify the Debug.log
so that it will show the applied damage to Enemy HP, like this
And with that the code for our AOE attack is finally complete.
// Everything below is inside a Class.
private string[] _factions = new string[3]{".Ally", ".Enemy", ".Neutral"};
private string[] _npcs = new string[7]{"Zane.Ally", "Connor.Enemy", "Delilah.Neutral", "Deborah.Ally", "Seth.Enemy", "Alex.Neutral", "Wolf.Enemy"};
private bool[] _withinAOERange = new bool[7]{true, true, false, false, false, true, true};
void Start()
{
int allyFound = 0;
int enemyFound = 0;
int neutralFound = 0;
foreach(var faction in _factions)
{
if(faction == ".Ally")
{
Debug.Log("Ally Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
allyFound = allyFound + 1;
Debug.Log("Ally " + allyFound + " " + npc + "HP : 100 - 0 = 0");
}
i++;
}
}
else if(faction == ".Enemy")
{
Debug.Log("Enemy Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
enemyFound = enemyFound + 1;
int randomDamage = Random.Range(10, 100);
Debug.Log("Enemy " + enemyFound + " " + npc + "HP : 100 - " + randomDamage + " = " + (100 - randomDamage));
}
i++;
}
}
else if(faction == ".Neutral")
{
Debug.Log("Neutral Faction.");
int i = 0;
foreach(var npc in _npcs)
{
if(npc.EndsWith(faction) && _withinAOERange[i] == true)
{
neutralFound = neutralFound + 1;
Debug.Log("Neutral " + neutralFound + " " + npc + "HP : 100 - 0 = 0");
}
i++;
}
}
}
}
Breaking Down And Understanding The Complete Piece Of Code
Now let’s break down this piece of code
First, we declared and initialized 3 arrays and set their values
Then in the Start() Method,
we created 3 member counting variables and set them to 0
After that we have the foreach loop which is looping over the _factions array
Actually we could have done this without using the factions array
but i wanted to show you nested foreach loops so we did this extra steps.
After that inside the foreach loop,
we created an if condition to separate the npcs by faction
Next, inside each faction
we initialized a variable i and set it to 0
then we created a Nested Foreach Loop inside each faction
by creating another Foreach Loop to loop over the _npcs array
and for each npc that we looped over, we checked two things
First, if the data inside the npc variable ends with the correct faction name
and Second, if the npc is within the range of the AOE attack.
If both these conditions returned true
then based on the faction we increased the member counting variable by 1.
After that, for the factions that will not be affected by the AOE attack i.e. the Ally and Neutral faction
we directly logged that there was no damage inflicted
while for the enemy faction, we generated a randomDamage value
and used it to simulate that the enemy was hit with the attack
and logged the newly calculated HP for the enemy.
With that done, let’s execute this piece of code in Unity and check the logs
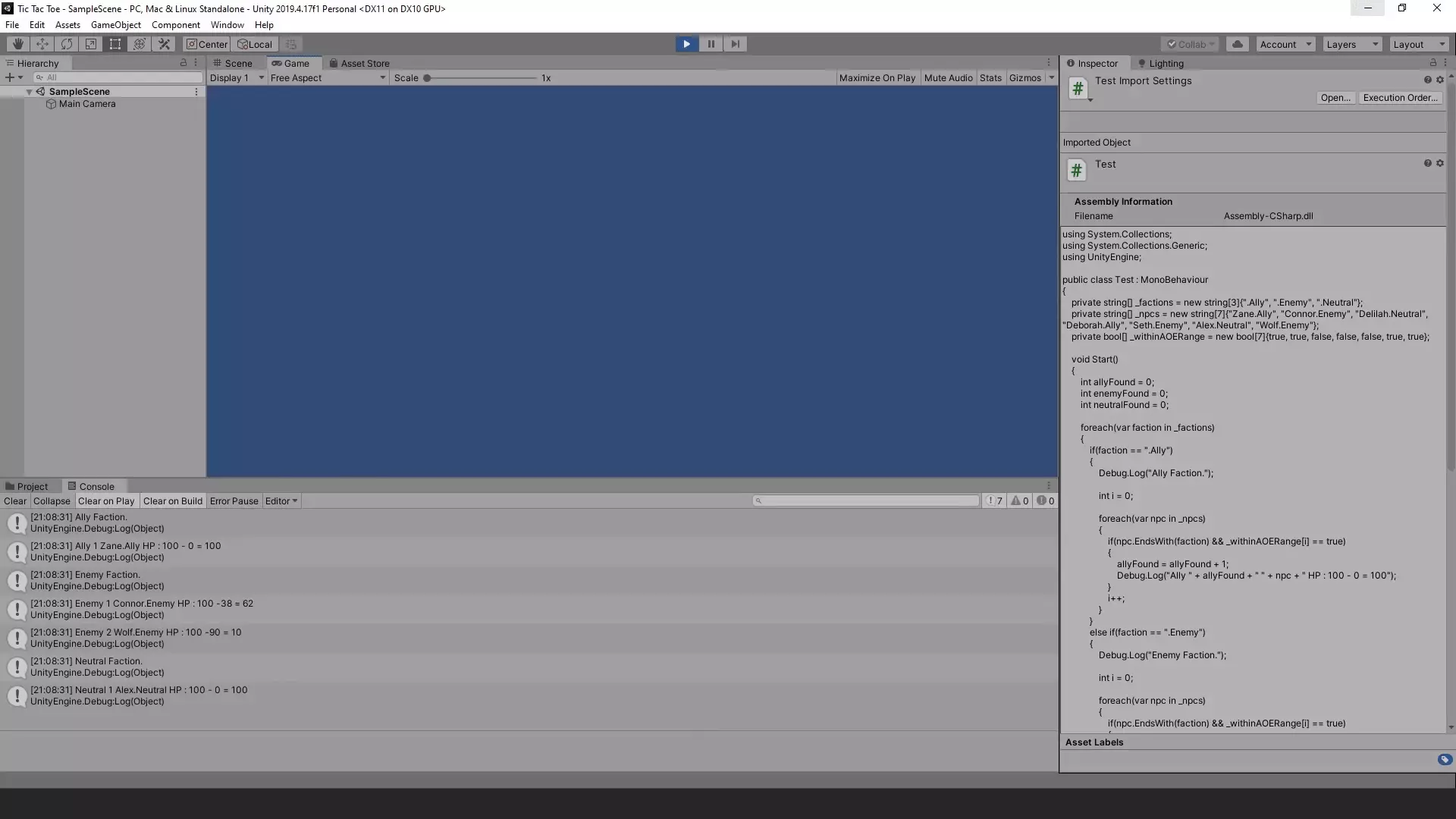
As you can see all the logs are showing correctly based on the factions
and are only logging the npcs that are within the range of the AOE attack.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords