In C# and all the other programming languages, there are special symbols and combination of symbols which which we use to Compare data stored inside a variable with another variables or with a hard-coded value.
These symbols and combination of symbols are what we call Comparison Operators in programming.
In articles before, we have already talked about Arithmetic Operators & Assignment Operators.
In this article, we are going to cover Comparison Operators and then in subsequent articles we will cover the Logical Operators.
This Post is Part of : How To Make A Game Series where i’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
If you are interested in Game Development then you might like this article on 3 Points To Consider Before Committing To A Game Idea.
in which i talk about the things i personally think about when I’m evaluating whether a Game Idea is worth pursuing or not.
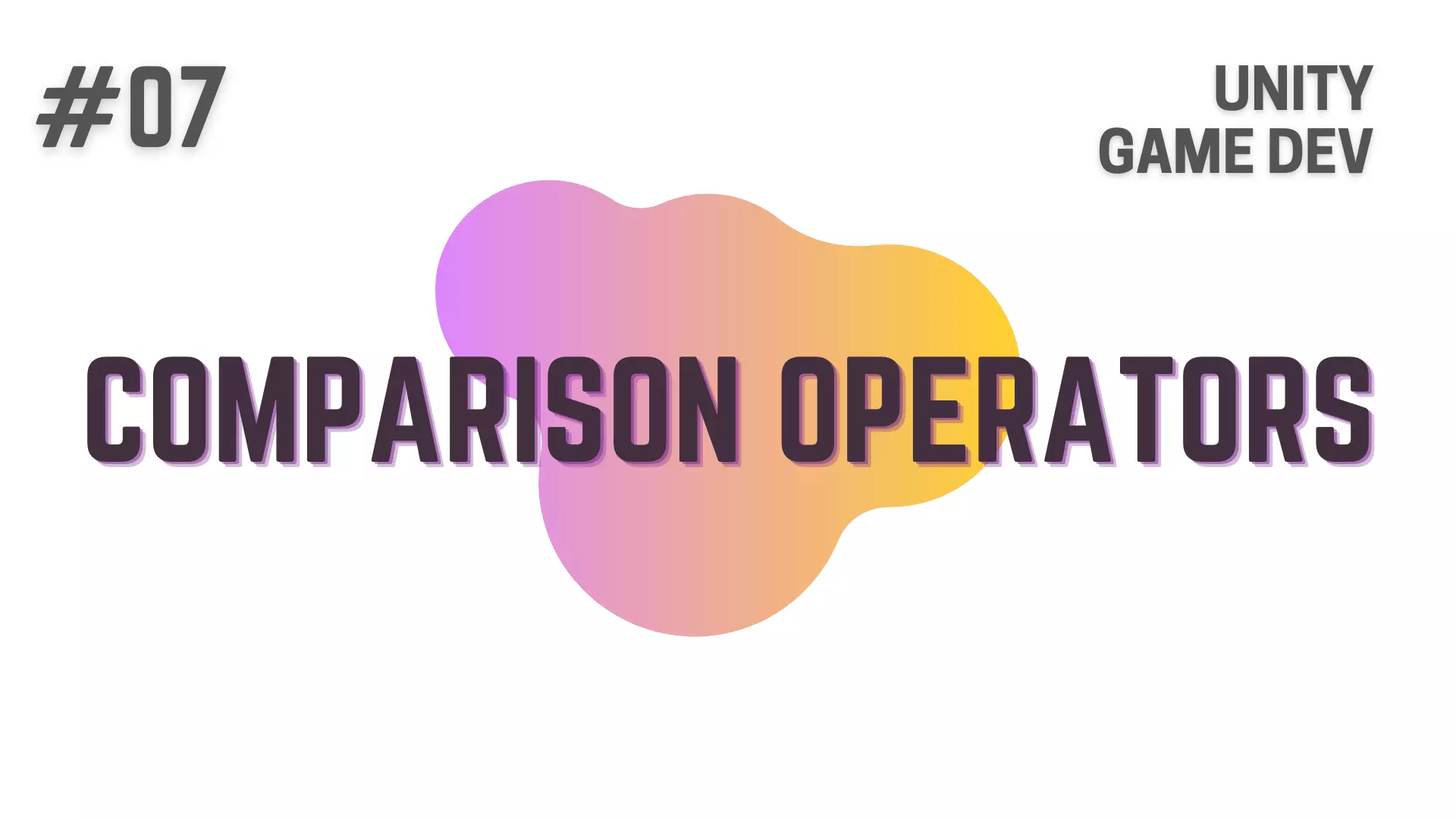
Articles Related To Operators in C#
Comparison Operators
Moving Forward, now let’s talk about Comparison Operators
As i said above, Comparison Operators are used to compare data inside a variable with other variables or with a hard-coded value.
In C#, there are 6 Comparison Operators that you will find useful as a Game Developer :
- Double Equal To Operator ( == )
- Not Equal To Operator ( != )
- Greater Than Operator ( > )
- Less Than Operator ( < )
- Greater Than Equal To Operator ( >= )
- Less Than Equal To Operator ( <= )
Comparison operator | Double Equal To ( == )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(_playerHP == 300)
{
// This Log will be printed.
Debug.log("Player HP : " + _playerHP);
}
}
Using the Double Equal To Comparison Operator ( == )
you can check if the variable or value that is on the left side of the Operator
is the same
as the variable or value on the right side of the Operator.
If both the values are same than the Operator will return true
while if both the values are different then it will return false.
Comparison operator | Not Equal To ( != )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(_playerHP != 300)
{
// This Log will not be printed.
Debug.log("Player HP : " + _playerHP);
}
}
Using the Not Equal To Comparison Operator ( != )
you can check if the variable or value that is on the left side of the Operator
is not the same
as the variable or value on the right side of the Operator.
If both the values are different than the Operator will return true.
while if both the values are the same then the Operator will return false.
Comparison operator | Greater Than ( > )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(_playerHP > 300)
{
// This Log will not be printed.
Debug.log("Player HP : " + _playerHP);
}
}
Using the Greater Than Comparison Operator ( > )
you can check whether the variable or value that is on the left side of the Operator
is Greater Than
the variable or value on the right side of the Operator.
Comparison operator | Less Than ( < )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(_playerHP < 300)
{
// This Log will not be printed.
Debug.log("Player HP : " + _playerHP);
}
}
Using the Less Than Comparison Operator ( < )
you can check whether the variable or value that is on the left side of the Operator
is Less Than
the variable or value on the right side of the Operator.
Comparison operator | Greater Than Equal To ( >= )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(_playerHP >= 300)
{
// This Log will be printed.
Debug.log("Player HP : " + _playerHP);
}
}
Using the Greater Than Equal To Comparison Operator ( >= )
you can check whether the variable or value that is on the left side of the Operator
is Greater Than or Equal To
the variable or value on the right side of the Operator.
Comparison operator | Less Than Equal To ( <= )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
if(_playerHP <= 300)
{
// This Log will be printed.
Debug.log("Player HP : " + _playerHP);
}
}
Using the Less Than Equal To Comparison Operator ( <= )
you can check whether the variable or value that is on the left side of the Operator
is Less Than or Equal To
the variable or value on the right side of the Operator.
RPG Analogy
Going to the RPG Analogy,
Think about a town in your game, with houses that the player can go in an out of during a certain period of time,
lets say from 9 am to 5 pm.
Now to do this, you can define a boolean to set the state of the door like
private bool _isDoorOpen;
Next, what you can do is,
in the script which manages game world time and day night cycle,
when the time hits 9 am you will set _isDoorOpen to true.
and at 5 pm you will set _isDoorOpen to false.
And in the script that allows the player to move in and out of the house,
you can use this boolean and create a condition
which will only allow the player to enter the house if _isDoorOpen is true
and will not allow the player to enter the house if _isDoorOpen is false.
Now, we are not going to make a day and night cycle script right now
what we are going to instead is we are going to put all the code in a single C# script
and hard code the current game time in it,
so that we can test out our logic.
Code Example
Note: In the code below I’m using the 24 Hour Time cycle.
// Everything Below is inside a Class.
private bool _isDoorOpen;
private byte _currentGameHour = 10;
private byte _houseOpeningHour = 9;
private byte _houseClosingHour = 17;
// This method is called on every single frame.
void Update()
{
// Section 1.
if(_currentGameHour >= _houseOpeningHour)
{
if(_currentGameHour < _houseClosingHour)
{
_isDoorOpen = true;
}
else
{
_isDoorOpen = false;
}
}
// Section 2
if(_isDoorOpen == true)
{
Debug.Log("The Player Is Allowed To Enter The House");
}
else if(_isDoorOpen != true)
{
Debug.log("The Player Is Not Allowed To Enter The House");
}
else
{
Debug.log("Something Went Wrong.");
}
}
Now, this piece of code can be divided into two parts
Section 1 evaluates whether to set the _isDoorOpen boolean to true or false based on what the _currentGameHour is.
Here we are using the Comparison operators and two if statements to make decision on whether the _isDoorOpen variable is set to true or false.
if the _currentGameHour is between _houseOpeningHour & _houseClosingHour
which are set to 9 ( 9 AM ) & 17 ( 5 PM ) respectively.
then _isDoorOpen variable will be set to true, else it will be set to false.
If we use Logical Operators, then instead of having two if statements, we could have done this if block using a single if statement
by using Logical AND Operator to make a compound condition.
if you want to learn about it go to this article on Logical Operators & Compound Conditions.
Section 2 evaluates based on the result of Section 1
whether we will allow the player to enter the house or will we stop the player.
if _isDoorOpen boolean was set to true in Section 1
then the player will be allowed to enter the house
otherwise he will be stopped from entering the house.
And for some unknown reason, if _isDoorOpen is undefined
then we will get a log saying that “Something Went Wrong.”
Now as we have hard-coded _currentGameHour to 10.
if you execute this code in Unity then you will get a log every frame saying that
The Player Is Allowed To Enter The House.
You Should not be Hard-coding the Variables
Now this piece of code is simple and is working fine.
but just to be sure that you understand,
You should not be hard-coding the _houseOpeningHour and _houseClosingHour variables like this
when you are developing a legit project
because if you are making something like an RPG (Role Playing Game.)
you would have a lot of different kinds of shops and houses in your game
And your town people will have their own schedules that they would be following
So some shops will open at let’s say 9 am while some might open at noon.
or maybe you have a night market in your game which will only be functional at night.
There will also be days where the shops will be closed because of a festival
or maybe because it’s a Sunday.
The same goes when we are talking about town houses
as if no one is home, you might want to close the door
and not allow the player to enter the house.
My point is that you should never hard-code this sort of things
and instead make a system based on your criteria,
which will manage this aspect of the game world for you
in a way that is more modular and easy to manage.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords