While Loop just like For Loop and Foreach Loop is used to repeatedly execute a piece of code based on the condition that you have predefined.
If you have been following along with the whole series, you should already be pretty clear about what loops are and how and why you would use them.
To those who are directly starting at this article and don’t have a clear understanding of what a loop is
Loops are special way of writing code using which you can loop through all the elements in an Array, Dictionary, List or Collection.
If you are new to programming and Game Development then i suggest that you go to articles on For Loops and Foreach Loops and continue from there
as in these two articles, I’ve talked about the basics of loops pretty extensively
and they be really helpful in making a good base for you.
So, let’s dive directly into it.
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Game Development takes a long time and it is really easy to lose focus on what’s important as you give more and more time to your game.
This can lead to you using your limited and valuable time on things that would not be really meaningful for your particular game,
So, to stop that from happening to you, I’ve written this article on 3 Points To Focus On When Designing A Game
in which I’ve highlighted 3 Points that will help you stay focused on things that are important for your games while you are developing them.
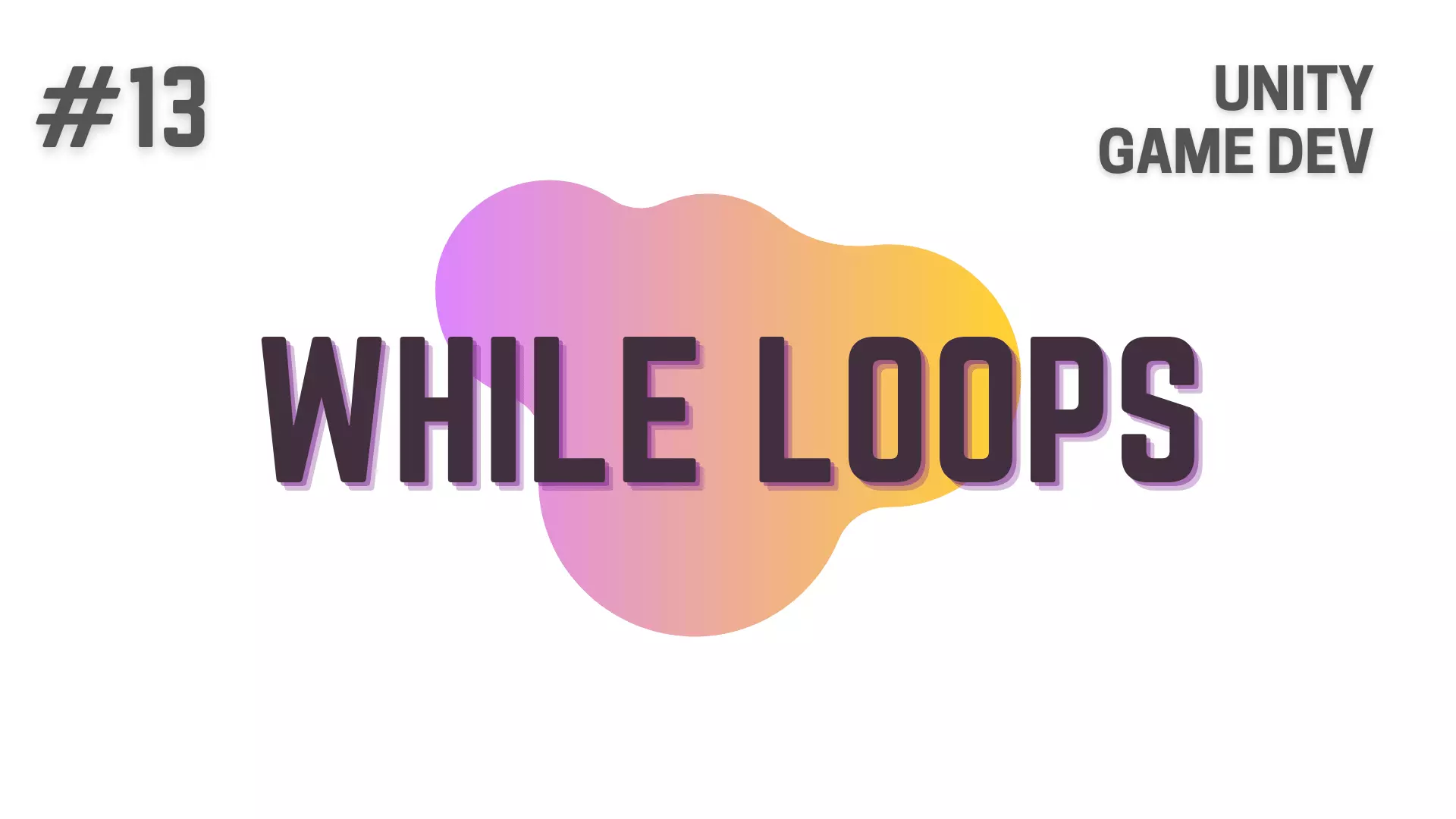
List Of Other Articles on Loops In C#
Syntax Of A While Loop in C# | Unity Game Development
// Everything below is inside a Class.
// The code below is just to understand the Syntax.
// For working example move further down in the Article.
void Start()
{
int i = 0;
while (condition) // Something like i < 10
{
// Code Block
i++; // Increment or Decrement Step
}
}
Now let’s directly go to the Syntax of a While Loop.
The While Loop starts with the While Keyword and the two Opening and Closing Parenthesis
which tell the compiler that you are writing a While Loop,
Next, inside the parenthesis we have the Condition that when evaluated
will return either true or false.
If the condition returns true, then the code block inside the curly braces of the While Loop will be executed
and if it returns false, then the code block inside the curly braces of the While Loop will not be executed
The For Loop contains the initialization and increment/decrement parts inside it’s loop setup.
On the other hand with While Loop,
initialization of the variables should be done before the While Loop Starts,
and the increment or decrement part should be inside the While Loop’s code block.
RPG Analogy For While Loops in C# | Unity Game Development
With that done, let’s move forward to the RPG analogy.
Think about you having various status effects in your game
that can be inflicted on the player like in the Pokemon games
where your Pokemon can be Burnt, Paralyzed, Poisoned, etc.
Now this effect will be sustained for various turns
or a certain amount of in game minutes
or whatever your criteria is.
So, let’s try to recreate this scenario
where for a couple of turns the player will not have any status effects
then the enemy will attack and the player will be inflicted with let’s say poisoning
And after that this status effect will last for 2 more turns after the current turn
that is for 3 total turns
before reverting back to normal.
That’s it.
Now let’s dive into the code and get this done.
Code Example For While Loops | Unity C# Game Development
Declaring Required Variables For While Loop Code Example
First of all lets declare and initialize a bool variable named _statusEffect and set it to false.
_statusEffect will be true or false based on whether the player is poisoned or not
Next, declare and initialize an int variable named _poisonedTurns and set it to 2
_poisonedTurns will contain how many turns the player will be poisoned for after the current turn.
After that, declare and initialize another int variable named _lastTurn and set it to 8.
_lastTurn as it name suggests, contains data regarding how long the battle will last for
In this case it will be for 8 total turns.
Next, inside the Start() Method,
we will declare and initialize two other int variables.
first named i and set it to 1;
and the second one being named _playerEffectedAtTurn
and set it to a Random Number between 1 and 9 using Random.Range()
with 1 being inclusive and 9 being exclusive.
This variable contains data about at which turn our player will be poisoned.
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
}
Coding Your First While Loop
With that done, now let’s create a While Loop with condition being
i <= _lastTurn
which means that, we will enter the while loop as long as the battle has started
and will keep on looping until the battle is finished
which in this case is at the 8th Turn.
Now inside the while loop,
the first thing we will do is increment i by 1
I’m doing this right away because if i forget to do it later,
it will create an infinite loop.
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
// Code will be added here in next part.
i++;
}
}
What Is An Infinite Loop?
For those who don’t know what an infinite loop is
it happens when the programmer does not put the correct exit statements
and increment or decrement logic in the loop
because of which the loop just keeps repeating itself
with no way for the program to move forward.
When a programmer does this the program will freeze or crash
and in the Task Manager on Windows,
it will show as “Not Responding”.
You can try creating an infinite loop yourself
and watch as it makes your unity editor become Not Responding in the Task Manager.
Check If Player Is Suffering From A Status Effect Or Not Inside The While Loop
Now inside While Loop’s curly braces but above the increment statement ( i++ )
we will create an if condition with the condition being
_statusEffect == false
and then below it, we will create an else if block, with the condition being
_statusEffect == true
So, if our player is currently not poisoned,
then the program will enter the if block
while if the player is currently poisoned,
then the program will enter the else if block
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
if(_statusEffect == false)
{
}
else if(_statusEffect == true)
{
}
i++;
}
}
If Player is Not Suffering From A Status Effect
Now inside the if block with the condition _statusEffect == false,
we will create another if condition where i == 1
and inside it’s curly braces, we will debug.log “Battle Started”.
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
if(_statusEffect == false)
{
if(i == 1)
{
Debug.Log("Battle Started.");
}
}
else if(_statusEffect == true)
{
}
i++;
}
}
After that, we will create another if condition
where i == _playerEffectedAtTurn
after that we will create an else if block
with the condition being
i == _lastTurn
and after that an else block,
like this.
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
if(_statusEffect == false)
{
if(i == 1)
{
Debug.Log("Battle Started.");
}
if(i == _playerEffectedAtTurn)
{
}
else if(i == _lastTurn)
{
}
else
{
}
}
else if(_statusEffect == true)
{
}
i++;
}
}
Now, the first if condition ( i.e. i == _playerEffectedAtTurn ) means that
this is the random turn when the player will get poisoned.
if this happens, then we will set the _statusEffect boolean to true
marking that the player has be poisoned
and then Debug.Log the turn on which the player got poisoned.
after the debug.log, we will write an if statement
where i == _lastTurn
and inside it’s curly braces, we will debug.log “Battle Ended”.
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
if(_statusEffect == false)
{
if(i == 1)
{
Debug.Log("Battle Started.");
}
if(i == _playerEffectedAtTurn)
{
_statusEffect = true;
Debug.Log("Battle Turn : " + i + "Player got poisoned.");
if(i == _lastTurn)
{
Debug.Log("Battle Ended.");
}
}
else if(i == _lastTurn)
{
}
else
{
}
}
else if(_statusEffect == true)
{
}
i++;
}
}
With that done, now inside the second condition i.e ( i == _lastTurn )
we will Debug.log the current Battle Turn
and then write another Debug.log saying “Battle Ended”.
Next inside the else block,
we will debug.log the current Battle Turn.
like this.
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
if(_statusEffect == false)
{
if(i == 1)
{
Debug.Log("Battle Started.");
}
if(i == _playerEffectedAtTurn)
{
_statusEffect = true;
Debug.Log("Battle Turn : " + i + "Player got poisoned.");
if(i == _lastTurn)
{
Debug.Log("Battle Ended.");
}
}
else if(i == _lastTurn)
{
Debug.Log("Battle Turn : " + i);
Debug.Log("Battle Ended.");
}
else
{
Debug.Log("Battle Turn : " + i);
}
}
else if(_statusEffect == true)
{
}
i++;
}
}
If Player Is Suffering From A Status Effect
That does it for the if block where the condition is
_statusEffect == false
Now moving forward to the else if block where
_statusEffect == true
we will create another if condition where
_poisonedTurns > 0
and Debug.log the current battle Turn
and the the fact that the Player is Currently Poisoned inside it.
After that, we will decrease _poisonedTurns by 1
as a turn has just passed since the player got poisoned.
With that done we will create an if statement where i == _lastTurn
and Debug.Log “Battle Ended” inside the curly braces.
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
if(_statusEffect == false)
{
if(i == 1)
{
Debug.Log("Battle Started.");
}
if(i == _playerEffectedAtTurn)
{
_statusEffect = true;
Debug.Log("Battle Turn : " + i + "Player got poisoned.");
if(i == _lastTurn)
{
Debug.Log("Battle Ended.");
}
}
else if(i == _lastTurn)
{
Debug.Log("Battle Turn : " + i);
Debug.Log("Battle Ended.");
}
else
{
Debug.Log("Battle Turn : " + i);
}
}
else if(_statusEffect == true)
{
if(_poisonedTurns > 0)
{
Debug.Log("Battle Turn : " + i + "Player is still poisoned.");
_poisonedTurns = _poisonedTurns + 1;
if(i == _lastTurn)
{
Debug.Log("Battle Ended.");
}
}
}
i++;
}
}
Now outside the if condition, let’s create an else if block
which has a condition of _poisonedTurns == 0
and set the _statusEffect boolean to false
marking that the player is no longer poisoned
and then Debug.log the current Battle Turn and the fact that the Player has Recovered in the Unity Console.
After that, just like above,
create an if condition where i == _lastTurn
and if it evaluates to true, Debug.log “Battle Ended”.
And with that done, the code is complete
Now let’s go and execute this code block in Unity and check the logs
Executed Code | While Loop Code Example in C# | Unity Game Development
// Everything below is inside a Class.
private bool _statusEffect = false;
private int _poisonedTurns = 2;
private int _lastTurn = 8;
void Start()
{
int i = 1;
int _playerEffectedAtTurn = Random.Range(1, 9);
while(i <= _lastTurn)
{
if(_statusEffect == false)
{
if(i == 1)
{
Debug.Log("Battle Started.");
}
if(i == _playerEffectedAtTurn)
{
_statusEffect = true;
Debug.Log("Battle Turn : " + i + "Player got poisoned.");
if(i == _lastTurn)
{
Debug.Log("Battle Ended.");
}
}
else if(i == _lastTurn)
{
Debug.Log("Battle Turn : " + i);
Debug.Log("Battle Ended.");
}
else
{
Debug.Log("Battle Turn : " + i);
}
}
else if(_statusEffect == true)
{
if(_poisonedTurns > 0)
{
Debug.Log("Battle Turn : " + i + "Player is still poisoned.");
_poisonedTurns = _poisonedTurns + 1;
if(i == _lastTurn)
{
Debug.Log("Battle Ended.");
}
}
else if()
{
Debug.Log("Battle Turn : " + i + "Player has recovered.");
_statusEffect = false;
if(i == _lastTurn)
{
Debug.Log("Battle Ended.");
}
}
}
i++;
}
}
Unity Logs | While Loop Code Example | Unity Game Development
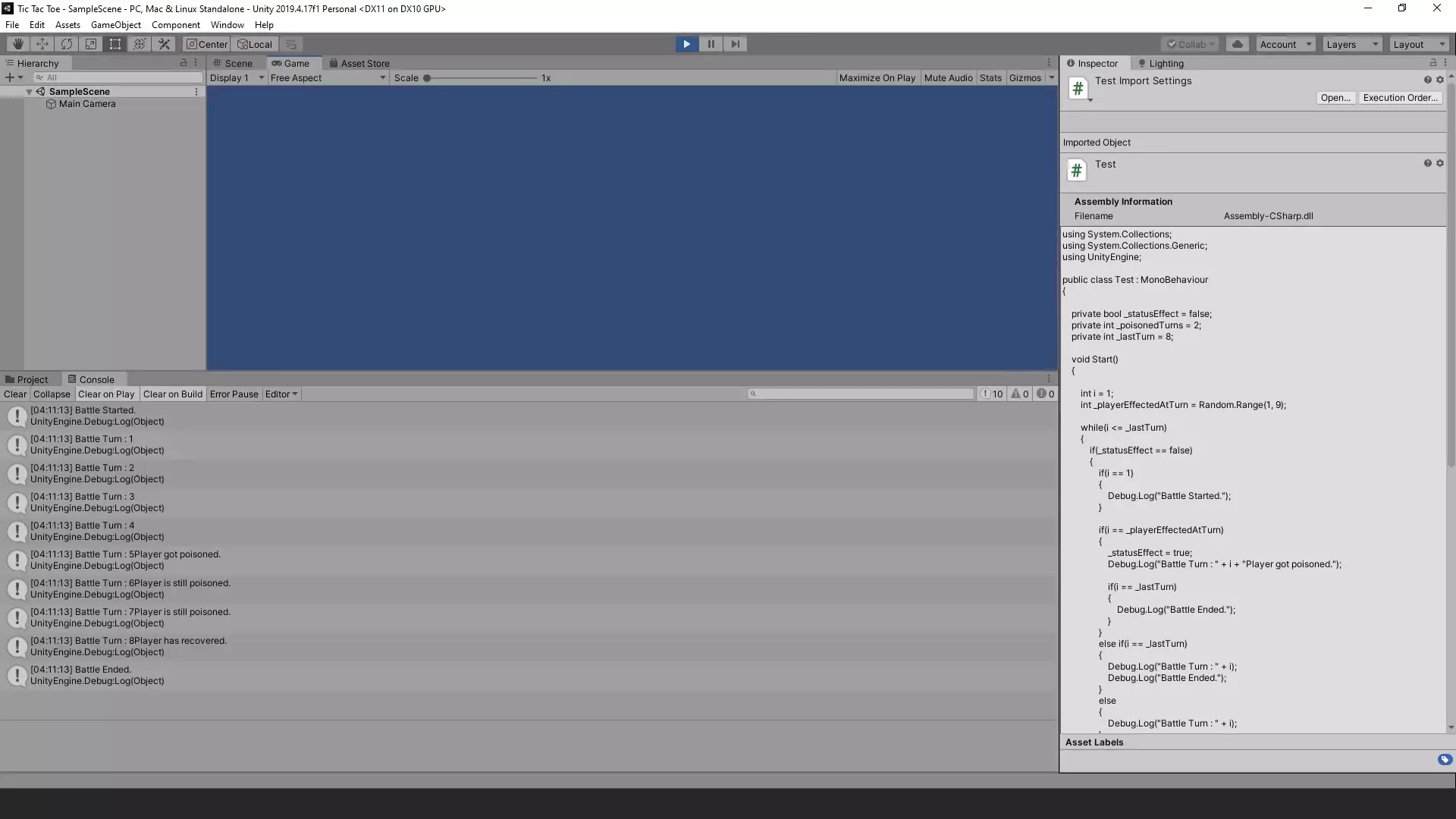
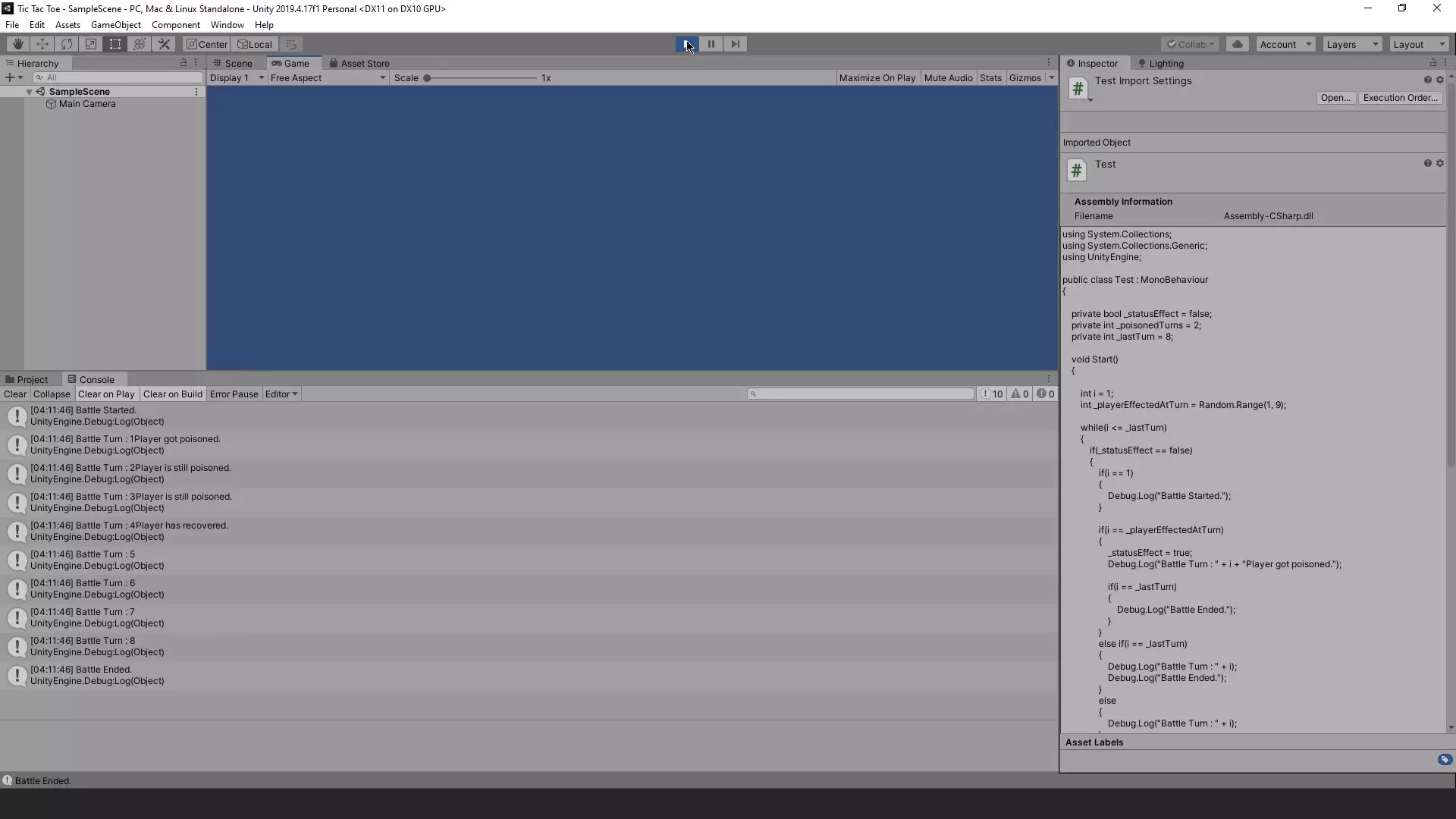
As you can see the logs are showing correctly
and are also reflecting that we used Random.Range()
to randomize on which turn the player would be poisoned.
Some Points On While Loops Before Wrapping This Article Up
Now, a couple of things before wrapping this article up.
First, you can created a Nested While Loop
just like with any other loops
by encapsulating a while loop inside another while loop.
i have already shown how to do this in the articles on For Loop and Foreach Loop
So, I’m not going to show it again in this article
and keep this article relatively short.
and Second, just like you use break keyword to exit For Loops and Foreach Loops,
you can do the same with While Loops.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords