When you are writing code In C# or any other programming languages
there will arise situations where you would want your program
to make decisions based on the criteria that you have predefined.
For Example,
When you are making a game of any genre, whether it be a Racing Game, an Adventure Game
or whatever crazy concoction of ideas in your head, you are making that Game out of
one thing is certain and that is
You as the God of Creation will have to make Arbitrary or Logical decisions
which will decide, how your Game World will function.
There are several ways using which you can make these decisions,
In today’s article, we will be going over, If Statements and all of it’s variants
which is one of the ways of doing so.
This Post is Part of : How To Make A Game Series where i’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
If you have are interested in Game Development, then you might also be interested in this article on 5 Ways To Develop Games On Low End / Low Spec PCs with Unity
in which i have given 5 Actionable ways using which you will be able to improve your Game Development Experience with Unity exponentially.
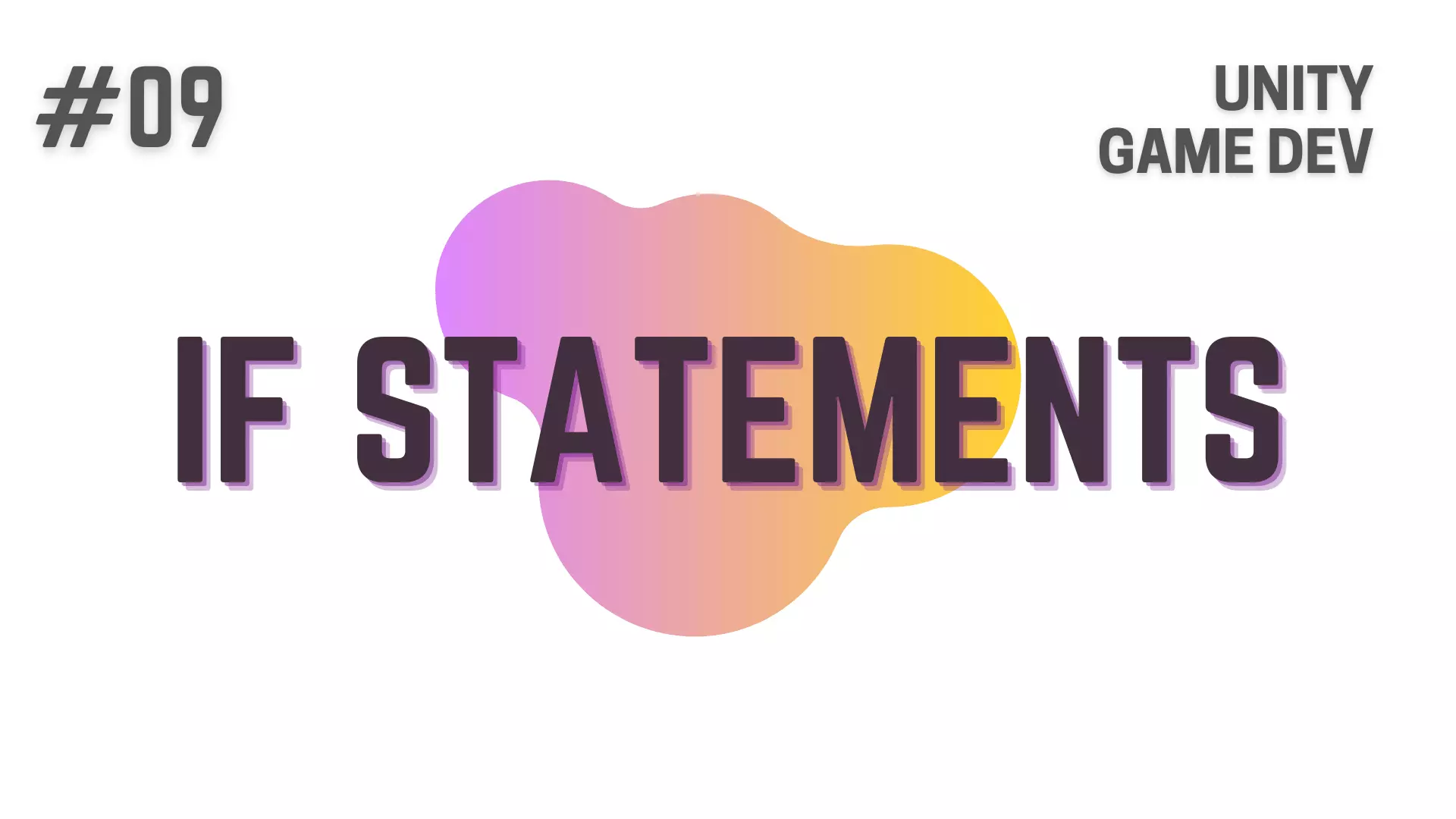
RPG Analogy To Explain What An If Statement / Conditional Statement / Decision Making Statement is
Let’s go to a RPG analogy and think about the Loot System in your game as an example.
Now for a while, think that your Game World is sentient and has it’s own thoughts.
So, watching your battle with the enemy
while reclining with a bowl of popcorn and a bottle of coke.
the Game World is thinking :
“Hmm! If The Player surmounts this battle,
then let me give him a million gold coins and some rare herbs
and maybe even that huge axe, that his enemy is wielding.”
Here, what the Game World is creating is what we call a conditional or a decision-making statement.
It has created an if statement with a condition that will decide
what will happen after the battle ends
and condition is based on whether the player wins or loses the battle
if the player defeats the enemy,
the Game World will magically drop some loot as a reward for winning the battle,
but if the player loses nothing will happen.
How To Write An If Statement?
Now let’s come back to reality where the Game World is not sentient
and you, the God of Creation will have to decide and write
this Arbitrary or Concrete Logical Condition
which needs to happen for the loot to drop.
So to start off,
let’s declare a boolean named _hasPlayerWon;
private bool _hasPlayerWon = true;
which will be true, if the player wins the battle
and false, if the player loses the battle
Next, in the Start() Method, let’s write the conditional
that will let our Game World know what to do
when the player wins the battle
To do that, we have to type
// Everything below is inside a Class.
private bool _hasPlayerWon = true;
void Start()
{
if(_hasPlayerWon is true)
{
Debug.Log("Player has won the battle and the gameworld will reward him with x amount of loot.");
}
}
That’s it.
Executed Code | If Statement
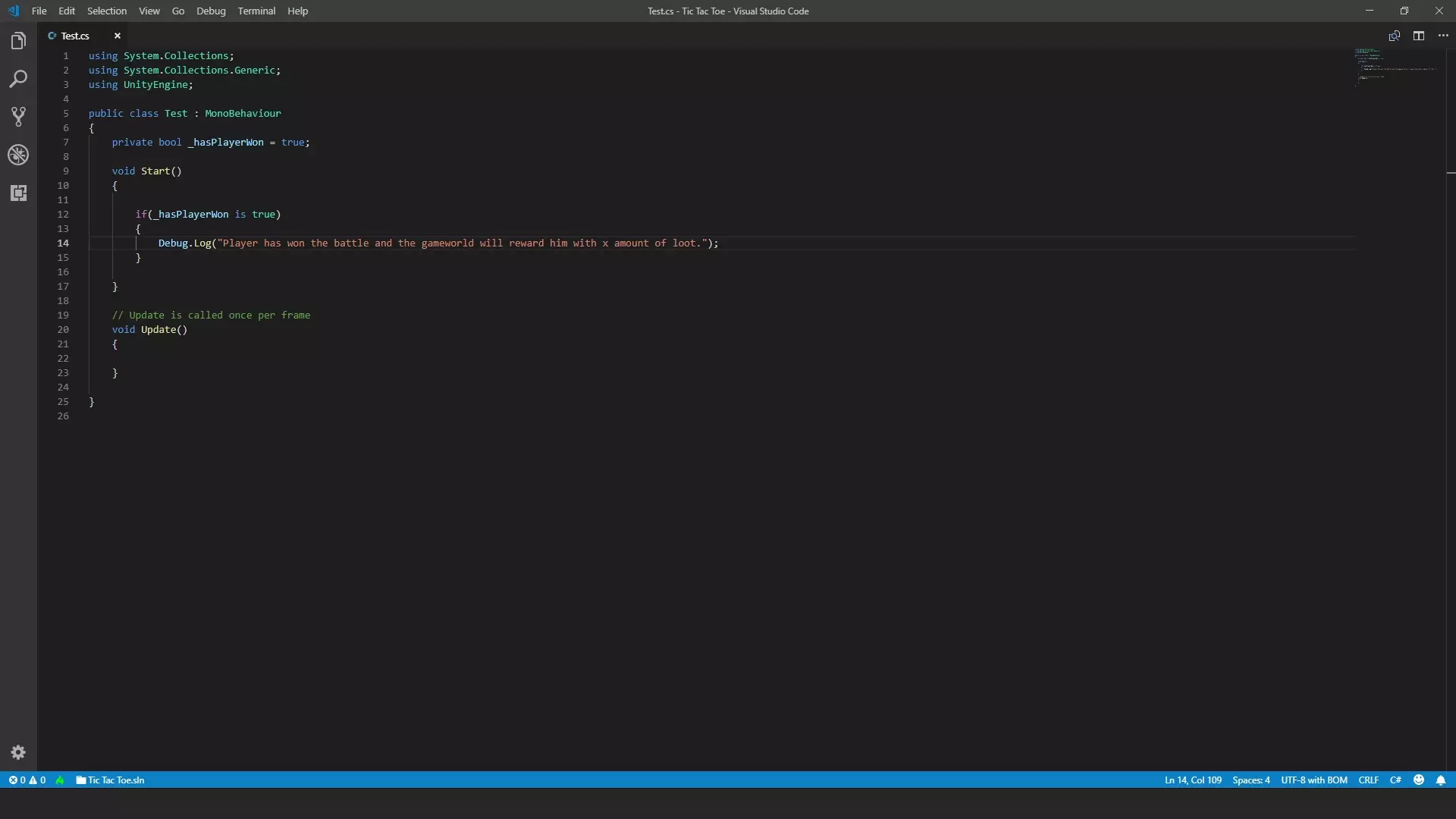
Now, let’s break down this block of code
just to make sure that you understand how this works.
First is, if and the two parenthesis which tells your compiler
that you are writing an if statement.
Then comes the condition between the two parenthesis i.e. _hasPlayerWon is true
if this condition is met,
then everything between the curly braces of the if statement will be executed,
and if the condition is not met
then everything between them will be ignored by the compiler.
Pretty Simple, isn’t it?
Now, Let’s execute this code in the Unity Game Engine and check the logs
Unity Log | If Statement
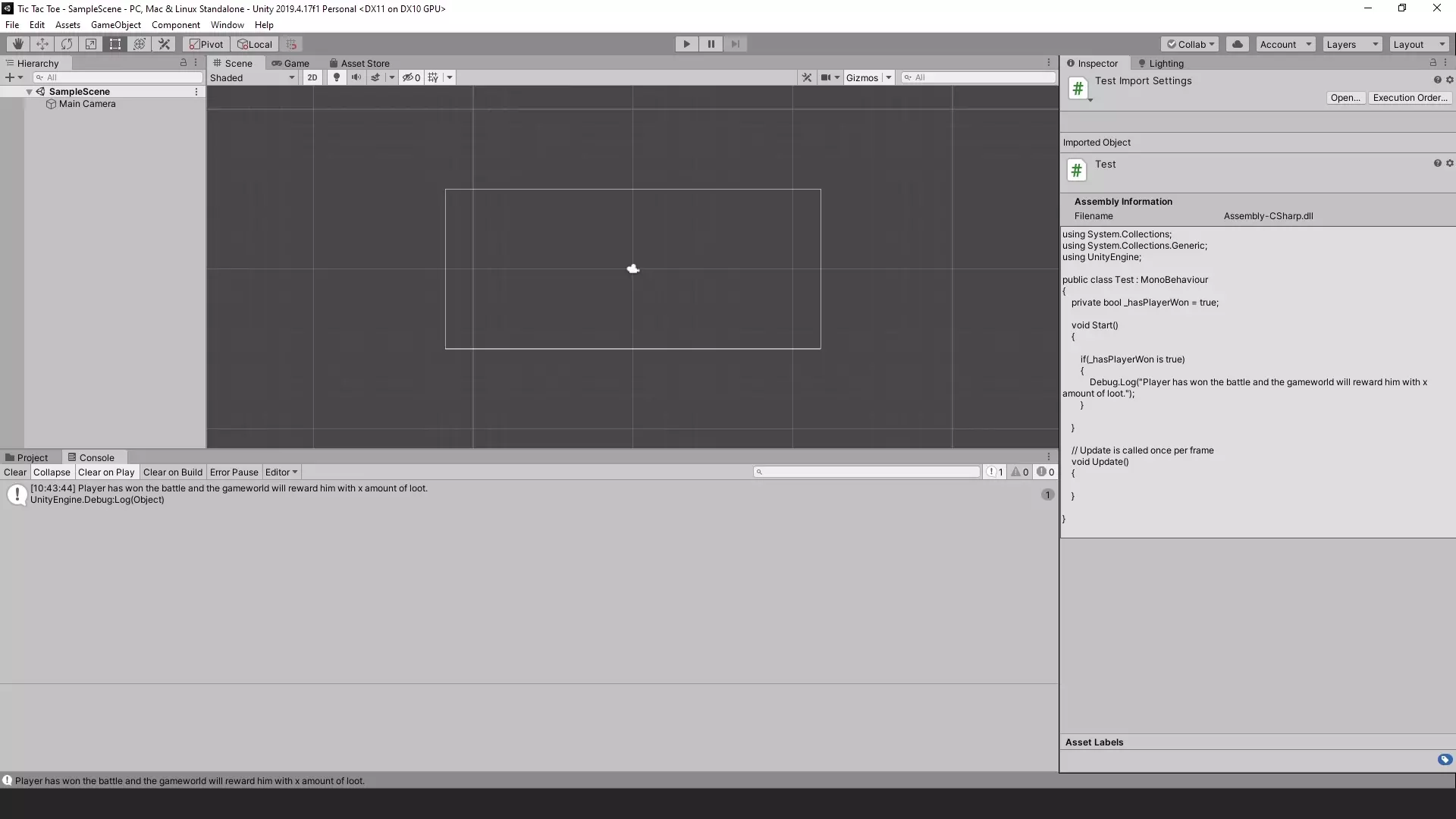
As you can see, it says
“Player has won the Battle and The gameworld will reward him with x amount of loot.”
Now let’s go back to the code and change the condition of if statement to
_hasPlayerWon to false, like this
// Everything below is inside a Class.
private bool _hasPlayerWon = false;
void Start()
{
if(_hasPlayerWon is true)
{
Debug.Log("Player has won the battle and the gameworld will reward him with x amount of loot.");
}
}
and look at the logs again in the Unity Console.
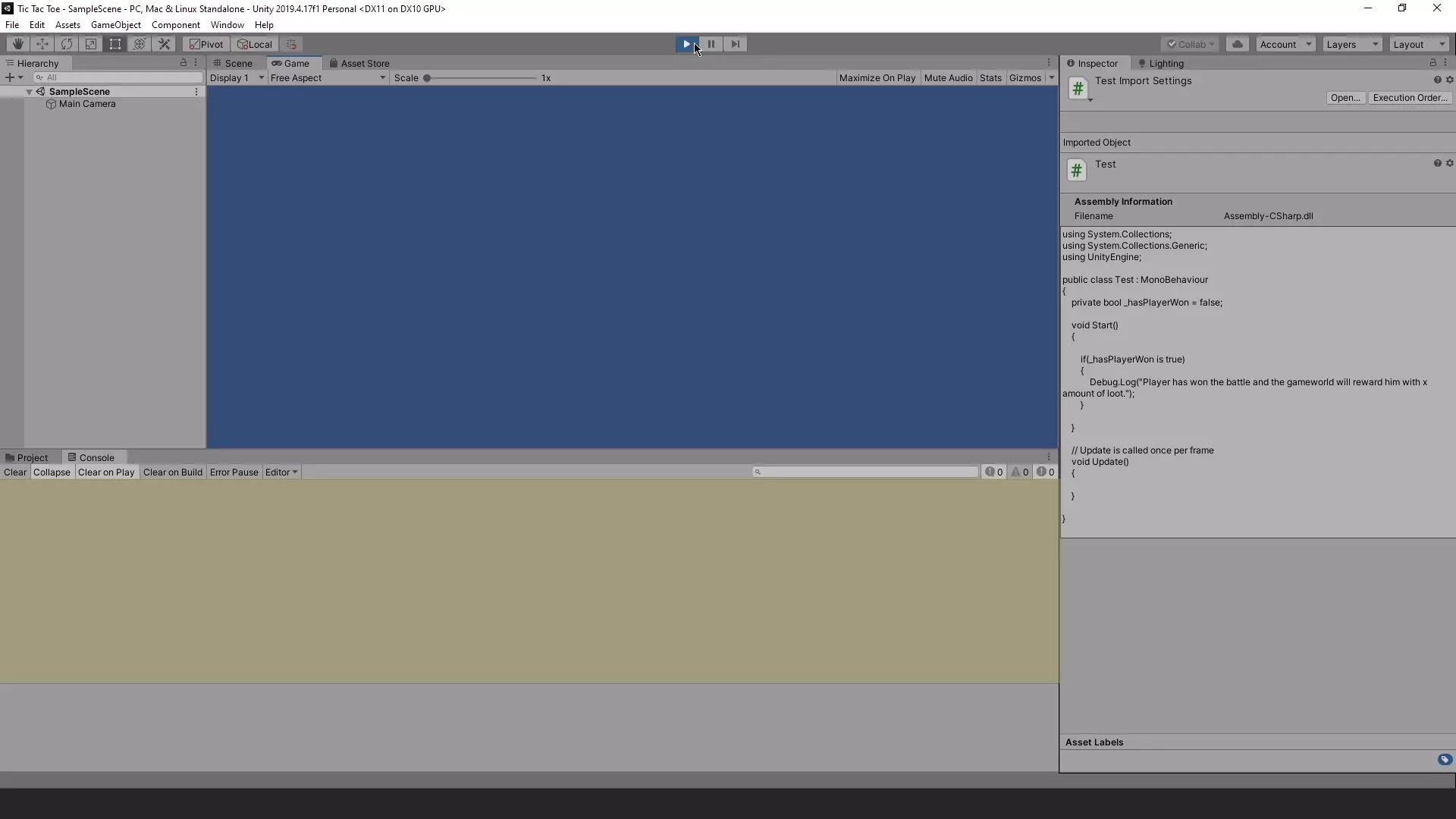
As you can see nothing was logged as that’s the whole point.
Because the condition of the if statement was not met,
the compiler ignored what was written inside the curly braces
and skipped ahead.
If Statement Variant | If – Else
By now, i think that you will have a good enough understanding
of how to use the basic if statements in your own projects.
so let’s move forward to the next variant of if statement, that is the if – else variant
In the last Unity Log, nothing was printed in the Unity Console
because the condition was not met.
but let’s say, what if, that instead of not printing anything,
we want to log a default statement inside the unity console if the condition was not met.
This is where the if else variant comes into play
The if else variant can be used to accomplish this,
by adding an else block right after the if statement,
like this
// Everything below is inside a Class.
private bool _hasPlayerWon = false;
void Start()
{
if(_hasPlayerWon is true)
{
Debug.Log("Player has won the battle and the gameworld will reward him with x amount of loot.");
}
else
{
Debug.Log("Player lost the battle.");
}
}
Here if the condition _hasPlayerWon is true returns true,
then it will print the log inside the curly braces of the if block
otherwise it will print the log inside the curly braces of the else block.
Thing To Remember When Using The If Else Statement
Just remember that, if the condition that governs the if block is not met
then the else block will be printed every single time
as there are no conditions attached to the else block.
For example,
let’s say that this piece of code was executed while the player was still battling the enemy
and the battle’s outcome has yet to be determined.
Even if that was the case,
because the condition for the if statement, i.e. _hasPlayerWon is true, was not met
The Log inside the else part will be printed and you will see :
“Player lost the battle.”
printed in the Unity Console
This is also why i said that
the else block is used to write default statements,
that will be executed, only if
no conditions before it were met.
Usually, my strategy of writing a conditional
is by making it so that
i will only enter the else block
if something went wrong in my logic
but I’m getting ahead of myself
For now, let’s execute this code in unity and check the logs.
Executed Code | If – Else
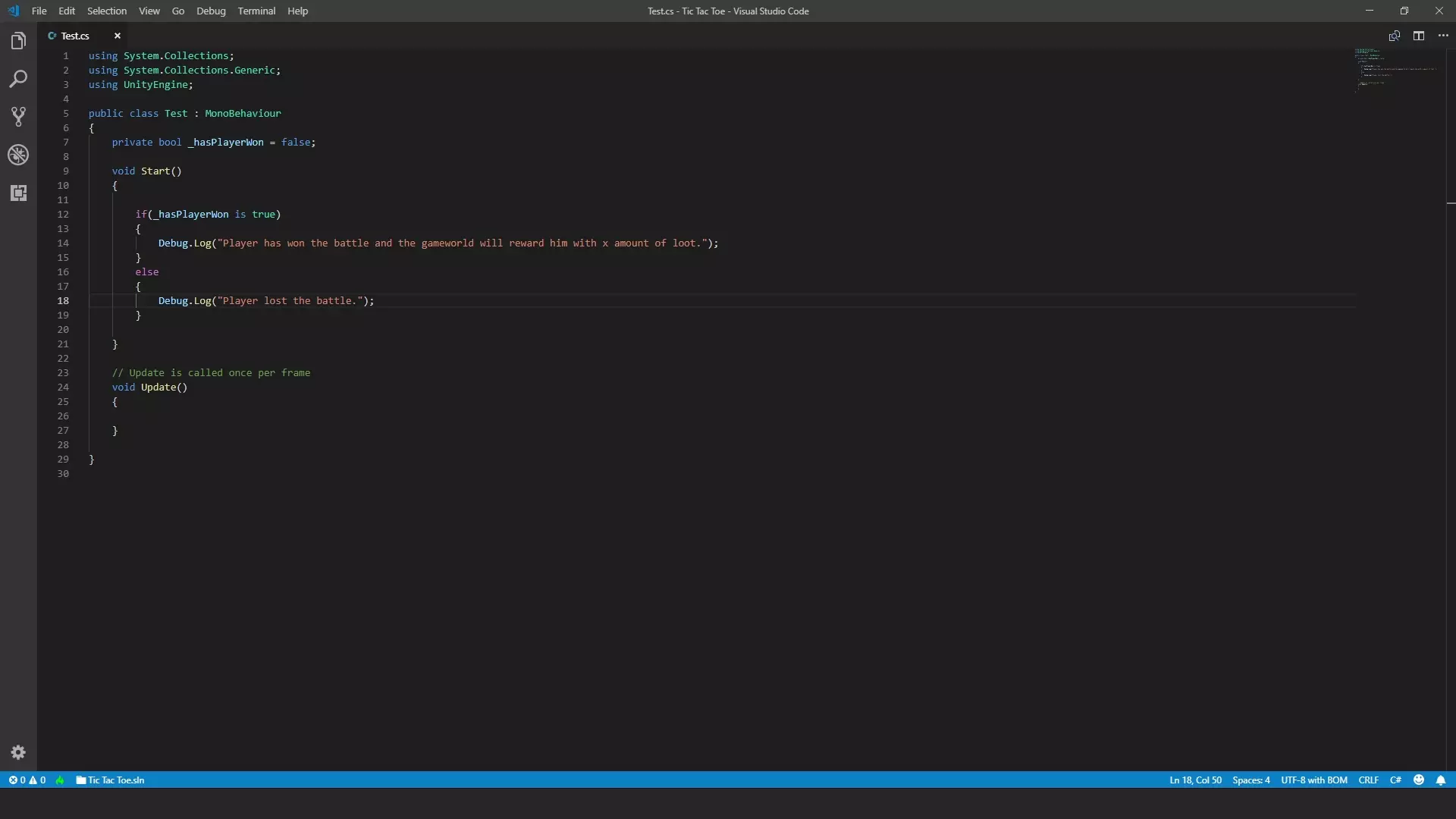
Unity Log | If – Else
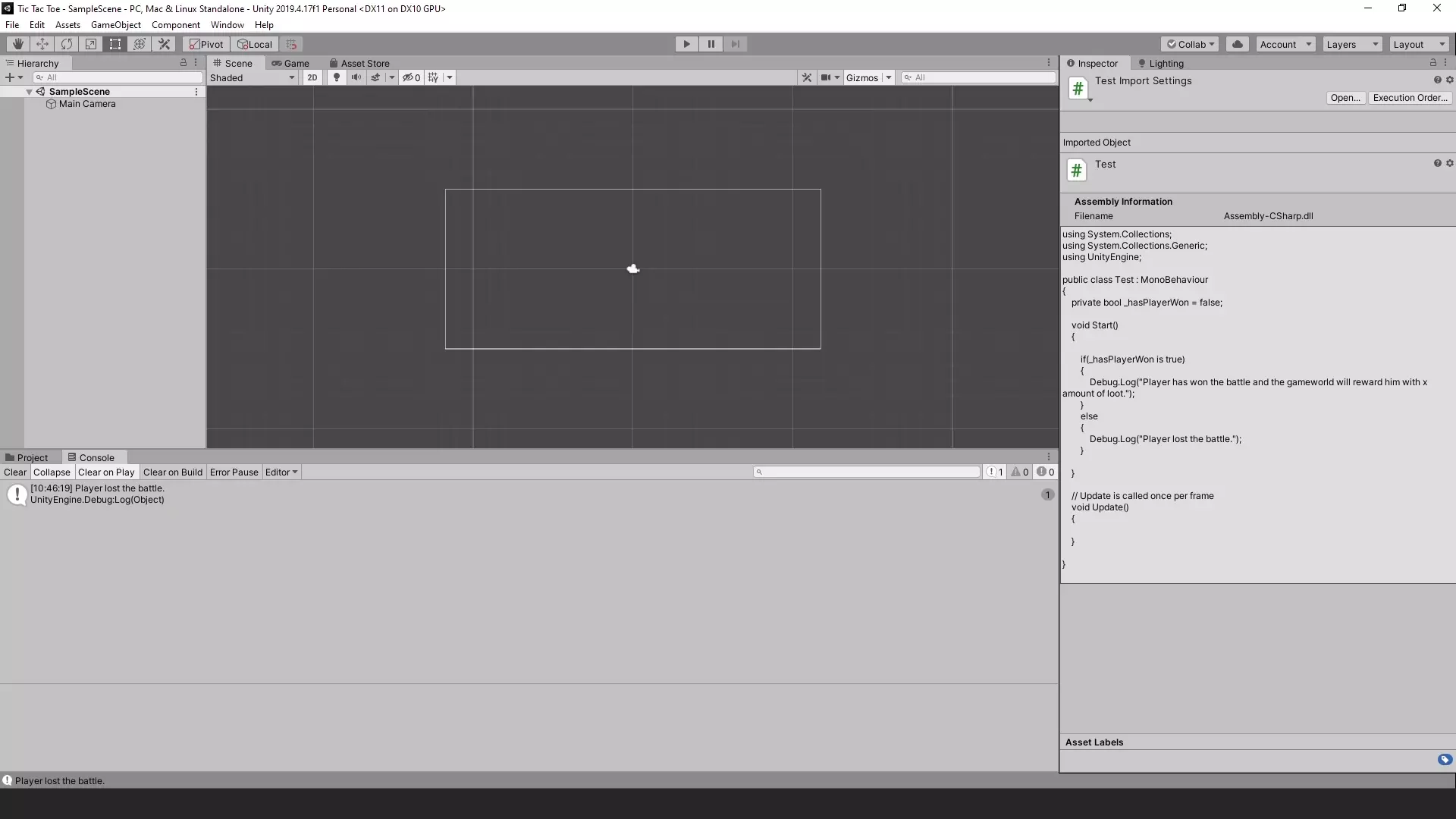
As you can see in the console, it says
“Player lost the battle.”
This means that our if else block is working as we expected.
If Statement Variant | If – Else If – Else
Going back to the code.
Now, let’s say that, you don’t want to print the log inside the else block
every single time the condition for the if statement was not met
but only want to print it
if the Player actually lost the battle.
Which is how it should be.
This sort of scenario is where the third variant of if statement
i.e. the if – else if – else variant comes into play.
If we take the existing code and turn else into else if like this by adding a condition
// Everything below is inside a Class.
private bool _hasPlayerWon = false;
void Start()
{
if(_hasPlayerWon is true)
{
Debug.Log("Player has won the battle and the gameworld will reward him with x amount of loot.");
}
else if(_hasPlayerWon is false)
{
Debug.Log("Player lost the battle.");
}
}
and then after the closing curly brace of else if, create an else block
and log “Something went wrong” inside it, like this
// Everything below is inside a Class.
private bool _hasPlayerWon = true;
void Start()
{
if(_hasPlayerWon is true)
{
Debug.Log("Player has won the battle and the gameworld will reward him with x amount of loot.");
}
else if(_hasPlayerWon is false)
{
Debug.Log("Player lost the battle.");
}
else
{
Debug.Log("Something went wrong.");
}
}
then now we have created an if – else if – else variant of the if statement.
You can stack as many else ifs as you want after the first if statement,
there is no limit to it that i know of.
Adding the else block is also optional
and you can just use if and multiple else if blocks
with no generalized else block.
Now let’s go to unity and execute this piece of code.
Executed Code | If – Else If – Else
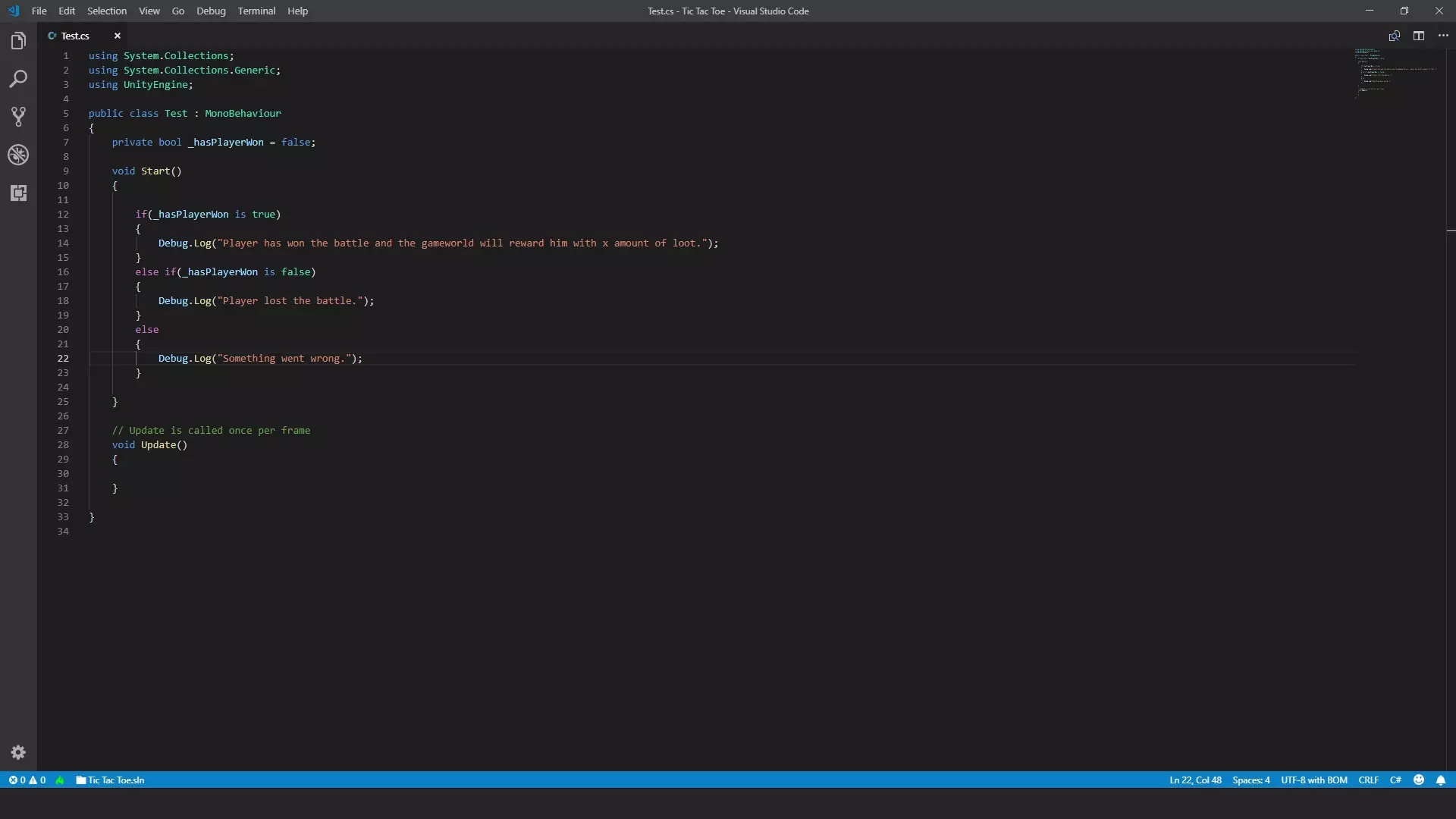
Unity Log | If – Else If – Else
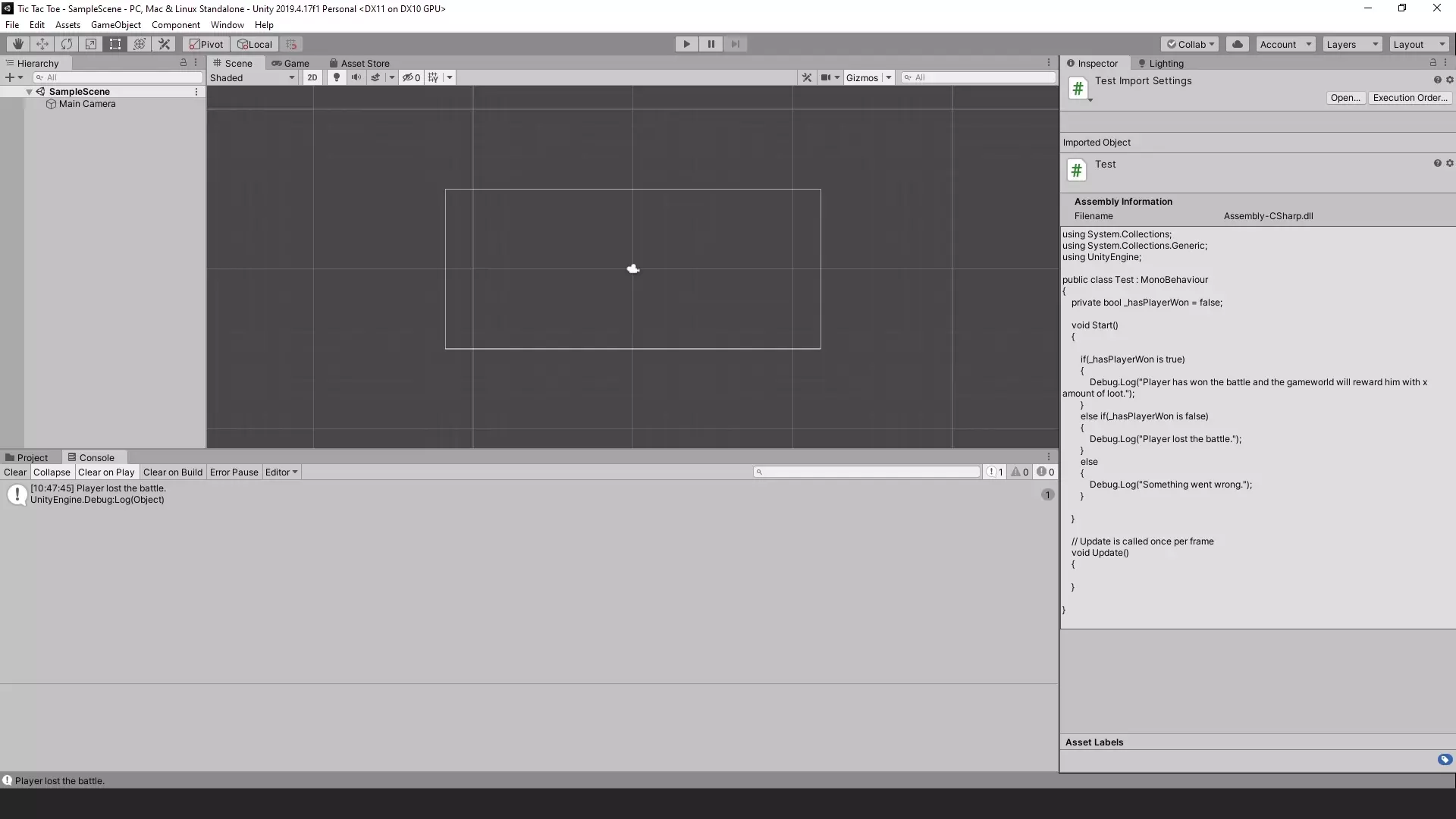
As you can see it says
“Player lost the battle.”
but this time this log was printed
not because it is the default log for the else block
but because it is a log for else if block
that would have only printed
if the player actually lost the battle
and would not print anything
if the player was still battling the enemy.
If Statement Variant | Nested If Statement
Back to the code,
now comes the last variant of if statement
which is called Nested if statement.
But before going into the code,
let’s continue the previous example.
We only want the code we wrote to be executed
if the player was battling the enemy
or the outcome of the battle has been decided.
and we don’t want this block of code to be executed
if the enemy doesn’t exist and the player was just having a dream.
So, to get something like this accomplished,
we will declare another boolean named _enemyTrulyExists and set it to false.
then we will take the code that we have already written
and put it inside a new if block, like this
where the condition of the new if statement is _enemyTrulyExists is true
// Everything below is inside a Class.
private bool _hasPlayerWon = false;
private bool _enemyTrulyExists = false;
void Start()
{
if(_enemyTrulyExists is true)
{
if(_hasPlayerWon is true)
{
Debug.Log("Player has won the battle and the gameworld will reward him with x amount of loot.");
}
else if(_hasPlayerWon is false)
{
Debug.Log("Player lost the battle.");
}
else
{
Debug.Log("Something went wrong.");
}
}
else
{
Debug.Log("Player is having a dream.");
}
}
and as you can see
just for fun I’ve also added an else block, which will log that
“Player is having a dream”
if the condition of the it’s if statement was not met.
That’s it.
You take an if statement of any variant
and put it inside another if statement
and BAM!
You have a Nested if statement.
Now as we have set _enemyTrulyExists to false,
when we execute this code in Unity
we should get a log in the Unity Console saying that
“Player is having a dream.”
So let’s go to Unity and check the logs
Executed Code | Nested If Statement
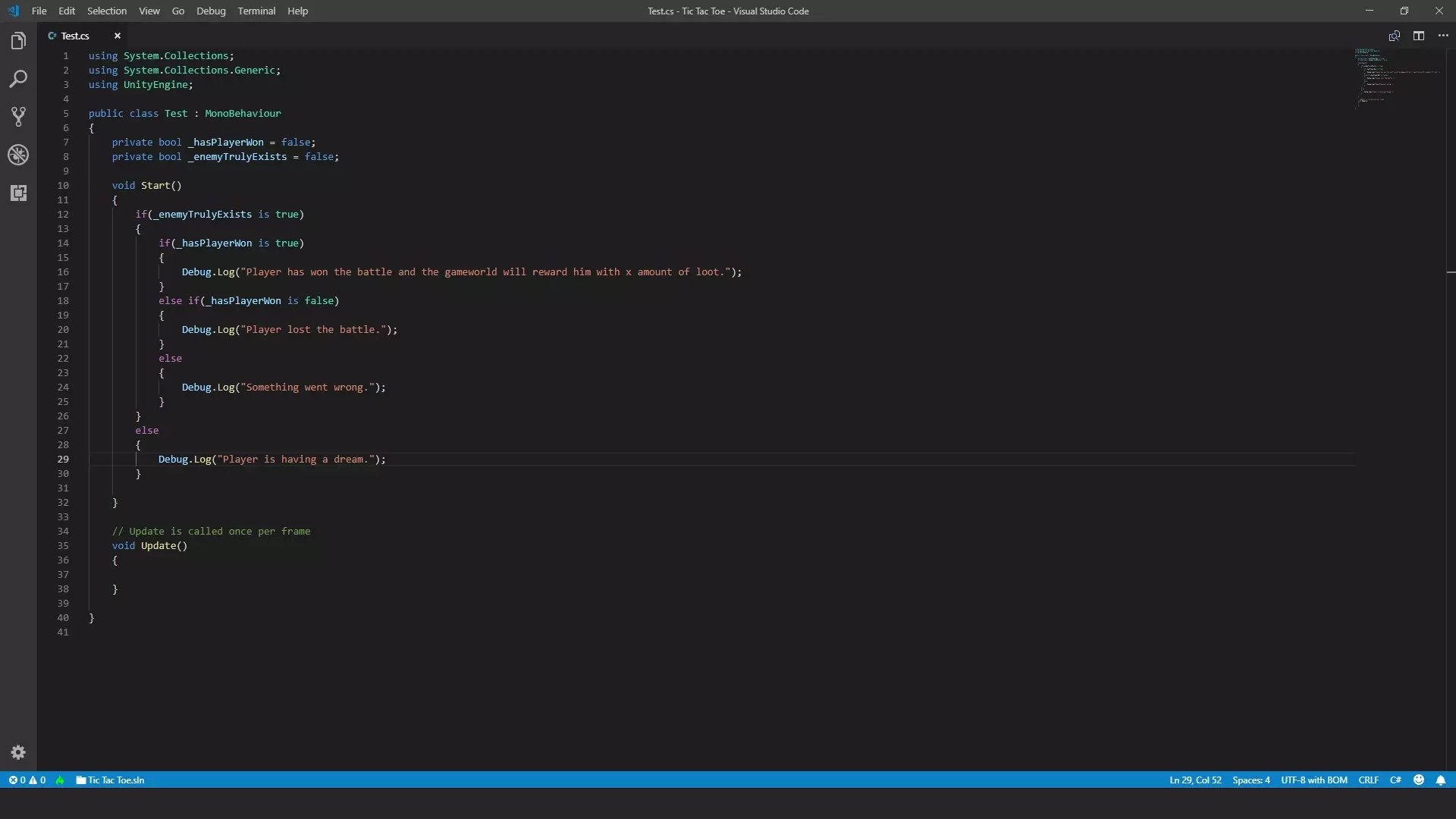
Unity Log | Nested If Statement
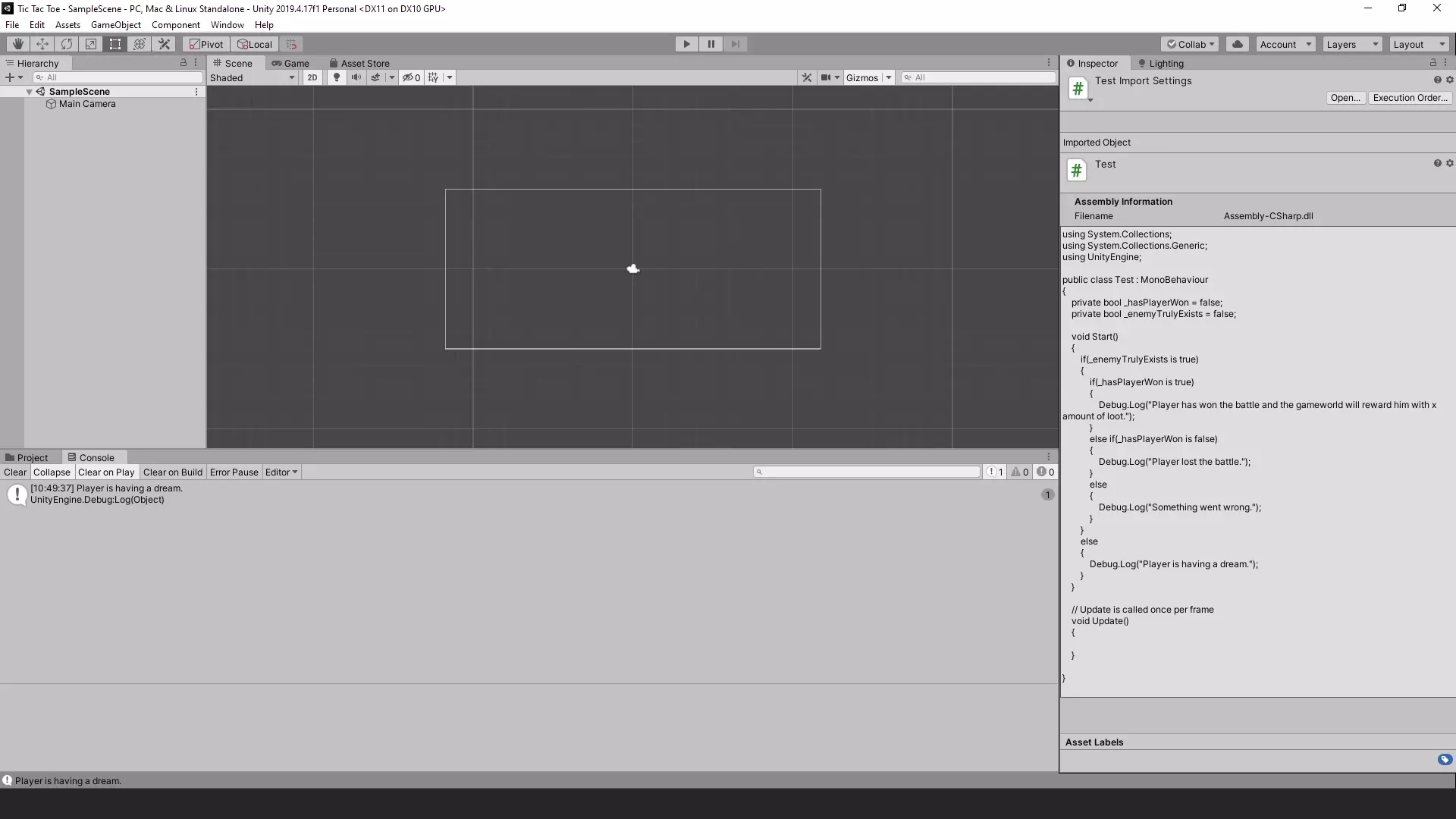
And as we expected, the idiot was really just dreaming.
In What Precedence Should You Write Conditions For An If Statement ?
One last thing i want to cover is
in what precedence, you should write these conditions.
So, the condition that you put at the top of the block
should be the most important condition in your conditional block.
This is because the compiler will evaluate the conditions, from top to bottom.
While, the succeeding conditions, should be in decreasing order of importance
with the last condition or the else block
having the least importance
being executed only if
nothing above it has met the conditions defined.
Taking the example of the player dreaming about the enemy,
the most important condition is whether the enemy truly exists or not.
Only if the enemy exists
do we want to move ahead and think about
whether the player won the battle or lost the battle.
If we wrote a code
where the evaluation of player winning or losing
is done before we check whether the player was dreaming
at best we would just lose processing power
because the code block which otherwise did not need to be executed
has to be executed now.
While at worst,
we will have an insufferably buggy game
that not even you, the developer, will wanna play.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords