An Array can be considered as one of the most fundamental parts of programming and you should learn about what Arrays are and get a thorough understanding of how they work before starting to create your own games.
So in today’s article I’m going to walk you through all the things related to Arrays and all the concepts related to it with real world RPG Analogies.
We will go through everything about Arrays from:
- What an Array is?
- Why do we use Arrays?
- Syntax for defining and initializing an Array in C#
- Overwriting / Modifying a value at an Array Index
- Finding the Size of an Array
- Common Error When Using Arrays | Index Out Of Range Exception
So, let’s dive directly into it.
This Post is Part of : How To Make A Game Series where i’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
If you have not read the article on Intro To Game Development in which i introduce the Art of Game Development, Roles in Game Development and your Recipe for Success as a Game Developer to you,
you can read that article next as i can’t emphasize how necessary it is and how helpful it will be for you to have the right mindset,
required to learn how to make a game and become an exceptional Game Developer.
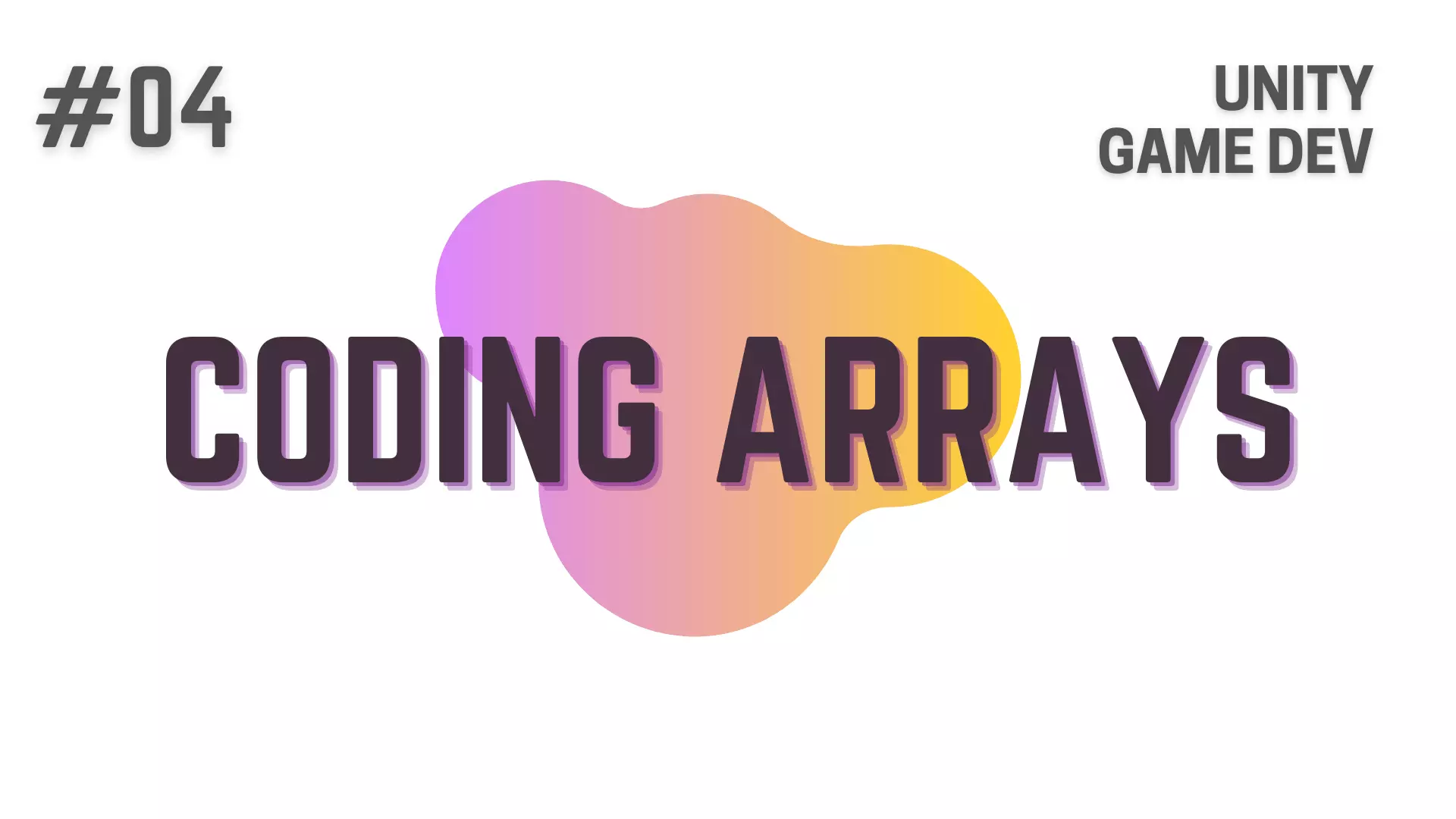
What is an Array?
In the last article we talked about Variables and the various Data Types that we will be using when coding in C#.
If you don’t know what a Variable is or just want to refresh your memory on it, you can read this article on Variables and Data Types in C#.
Building on that article, it would be really easy to understand what an Array is.
You already know that every Variable has a Data Type and also that a Variable is like a container that can contain a value of that defined Data Type.
private int _playerHP = 100;
For Example, in the code above, we have declared and initialized a variable named _playerHP with it’s Data Type defined as int.
So, the _playerHP variable can only contain values that are whole numbers between the ranges allowed by the int Data Type.
Now, Arrays can be thought of as a crate in which these container like variables that have the same Data Type are placed and then that crate is given a name.
RPG Analogy
Going to the RPG analogy, think about the numerous NPCs in the game and you having to store the names of those NPCs (NPC – Non Player Characters).
Now lets say that you don’t have any knowledge of Arrays and only know what a Variable is,
then you would probably declare and set values for these Variables like this.
private string _npc1 = "Zane";
private string _npc2 = "Connor";
private string _npc3 = "Delilah";
...
and so on.
And if you don’t know what an array is then this is completely fine,
but you might also have noticed that doing it like this is quite redundant.
Why Do We Use Arrays?
If you look at this piece of code and use a little brain, you will know two things,
First, you will know that, all these 3 variables contain names of NPCs in the game
and Second, all these 3 variables have the same Data Type, that is string.
Arrays are a great way to categorize this sort of data, so that we can remove this copious amount of unnecessary redundancy.
Using Arrays makes it a lot easier to maintain and access the data when we so require.
So, let’s solve this redundancy by declaring an array for this scenario.
Syntax For Defining An Array in C#
private string[] _npcNames = new string[3]{"Zane", "Connor", "Delilah"};
Now to some of you the line of code above would have made complete sense,
While some of you might be thinking, VP what sort of chimera monstrosity have you created.
Well, let’s break this little monster down to pieces and understand the syntax.
Left Side Of C# Array Defining Syntax
private string[] _npcNames = new string[3]{"Zane", "Connor", "Delilah"};
First as you already know is the Access Modifier i.e private
Then we have the Data Type of the Variable i.e. string,
but as you can see, we have a pair of opening and closing Square Brackets right after the Data Type.
This pair of opening and closing Square Brackets is what is used to signify that we are creating an Array.
And the Data type before the Square Brackets will be the Data Type of this defined Array i.e. _npcNames.
Right Side Of C# Array Defining Syntax
private string[] _npcNames = new string[3]{"Zane", "Connor", "Delilah"};
Next is = new string[3], which is us basically saying that,
make a new array of type string, that has 3 members in it at index 0, 1 and 2.
You can replace 3 inside the Square Brackets, which is the Size of the Array, with any whole number.
You can even use a variable which has a whole number datatype i.e. byte, short, int, long as the argument in place of 3.
After that, we have everything inside the Curly Braces, which is us setting values to our array,
Zane at index 0, Connor at index 1 and Delilah at index 2.
Remember an Array and most things in programming start from 0 and not at 1.
That mean that the first element of an Array, in this case Zane, is stored at the 0th index of the _npcNames Array and not at the 1st index.
Another Way Of Defining An Array In C#
private string[] _npcNames = new string[3]{"Zane", "Connor", "Delilah"};
In this piece of code, we have declared and initialized the Array all in one line,
but most of the time, you will not be doing it like this
and instead you will probably be separating the Array Declaration and Initialization into multiple steps, like this
// All The Code Below Is Inside The Class
// Array Declaration.
private string[] _townLocations;
// Predefined Method In Unity. Will Talk About It In Another Article.
void Start()
{
int totalLocationsInGame = 2;
// Array Initialization.
_townLocations = new string[totalLocationsInGame];
_townLocations[0] = "Dragon Cave";
_townLocations[1] = "Mayor's House";
// Logging Array Index Values In The Unity Console.
Debug.Log("Index 0 : " + _townLocations[0]);
Debug.Log("Index 1 : " + _townLocations[1]);
}
In the code above, as you can see, we first declare the Array, _townLocations but we don’t Initialize it.
After that in the Start Method we are initializing _townLocations using an int variable totalLocationsInGame as argument.
And then we are Setting Values Dragon Cave at 0th index and Mayor’s House at the 1st index of the _townLocations array.
The two Debug.Logs are what we use to log anything in the Unity Console for Testing & Debugging purposes when we are working with unity.
What we want to log, is the data that is stored in the _townLocations array,
at 0th index which is at _townLocations[0]
and at the 1st index which is _townLocations[1].
What we expect from this piece of code is that,
When we compile and run it, in the Unity Console it will show,
Index 0 : Dragon Cave
Index 1 : Mayor’s House
That will mean that we have successfully Declared, Initialized and Assigned values for the _townLocations array.
Now, Let’s Go into Unity and check the logs in the Unity Console.
Complete Piece Of Code That I Executed
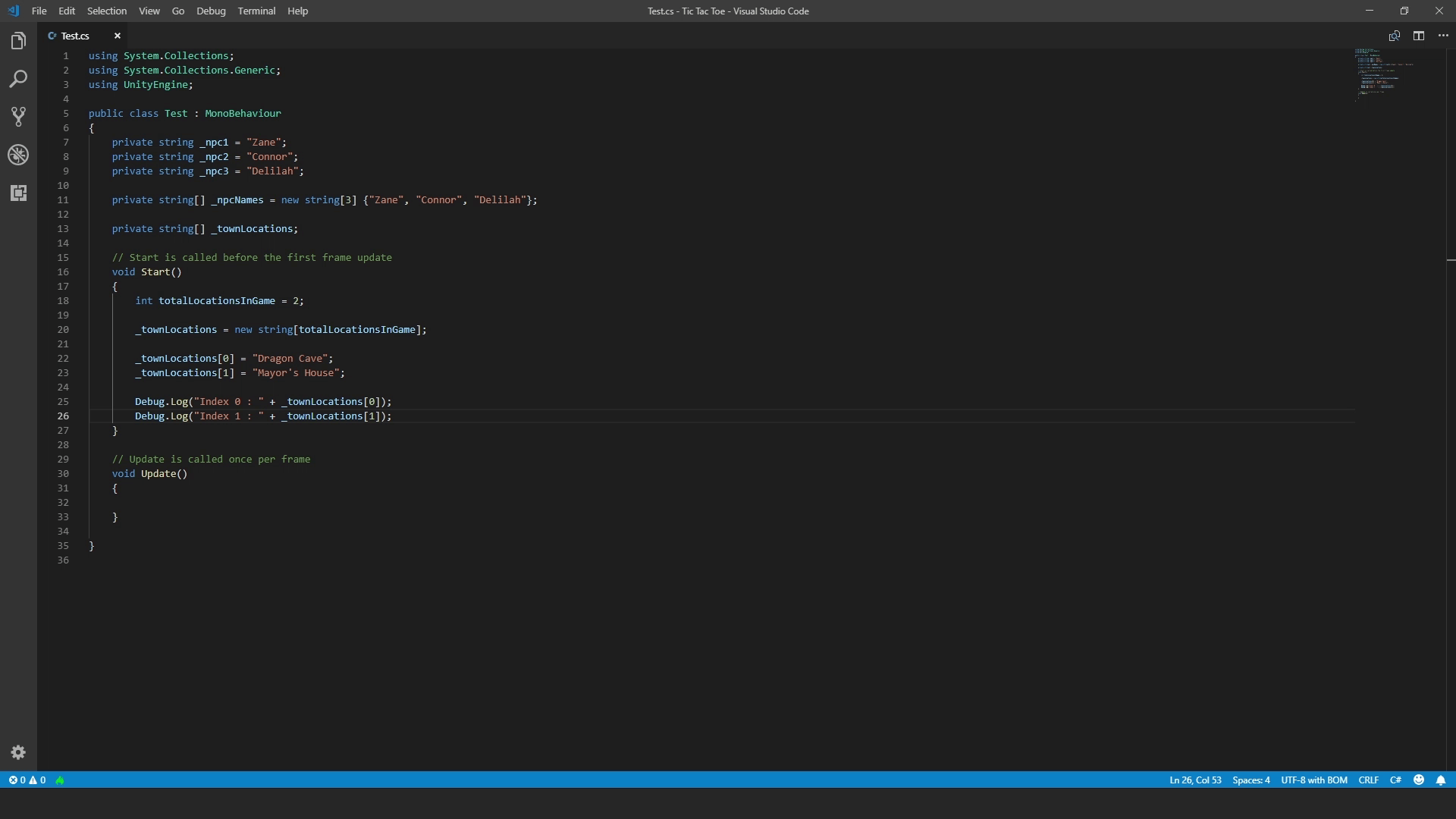
Unity Log
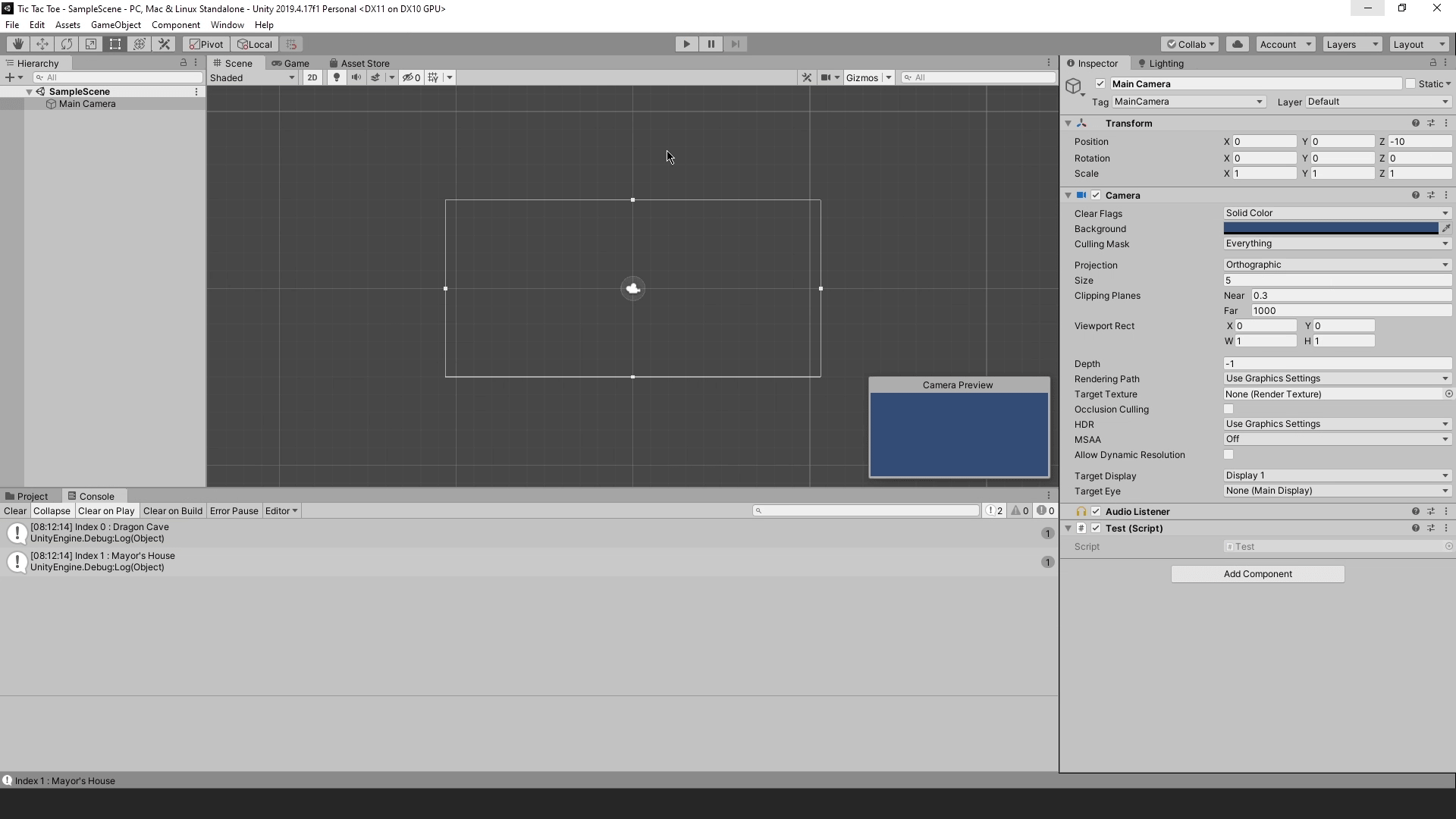
As you can see in the Unity Log above, we have our Logs saying
Index 0 : Dragon Cave
Index 1 : Mayor’s House
Great, so now we at least know that i have not been wasting your time.
Overwriting / Modifying A Value At An Array Index
Coming back to the Code, You can use the same syntax that you use for setting the value, to overwrite a value at a certain index.
For example, if you want to overwrite the 0th index that right now contains Dragon Cave and change it to Lake House, you can do it like
_townLocations[0] = "Lake House";
And then you can Debug.log it’s value in the Unity Console like this.
Debug.Log("Index 0 Changed To : " + _townLocations[0]);
So, if you add these 2 Lines of code after the 2 Debug.Logs in the full code image above, we should get an output that look like this in the Unity Console.
Index 0 : Dragon Cave
Index 1 : Mayor’s House
Index 0 Changed To : Lake House
Finding The Size Of An Array
Now, Let’s Talk about how you can find the size of an array.
This is something that you need to know because you would be using this quite extensively when working with various sorts of loops.
So, to find the size of _npcNames array and _townLocations array and then log it in the unity console, you need to type
Debug.Log("Total NPCs : " + _npcNames.Length);
Debug.Log("Total Town Locations : " + _townLocations.Length);
So, you type the name of the array, in this case _npcNames & _townsLocation.
After that you add a dot (.) and then type Length.
If we add this 2 Lines to the code that we already have then in the Unity Console you will see these logs for these 2 Lines :
Total NPCs : 3
Total Town Locations : 2
As when we declared and initialized both these arrays, we set their lengths to these sizes respectively.
Common Error When Using Arrays | Index Out Of Range Exception
Moving forward, there is one final thing that i want to cover before wrapping this article up and that is one of the most Common Errors you will encounter when you work with arrays.
So, let’s generate an error intentionally by adding the line below to our code.
Debug.Log("Name of the Third Location : " + _townLocations[2]);
Now this line of code should give us an error because when we initialized the _townLocations array, we stated that it only has 2 members
and now we are trying to access the 2nd index, which is the 3rd member of the array, which does not exist. (Reminder: Array Index Starts at 0.)
If we go to Unity and execute this code we will get a big red exclamation mark in the Unity Console as shown in the image below.
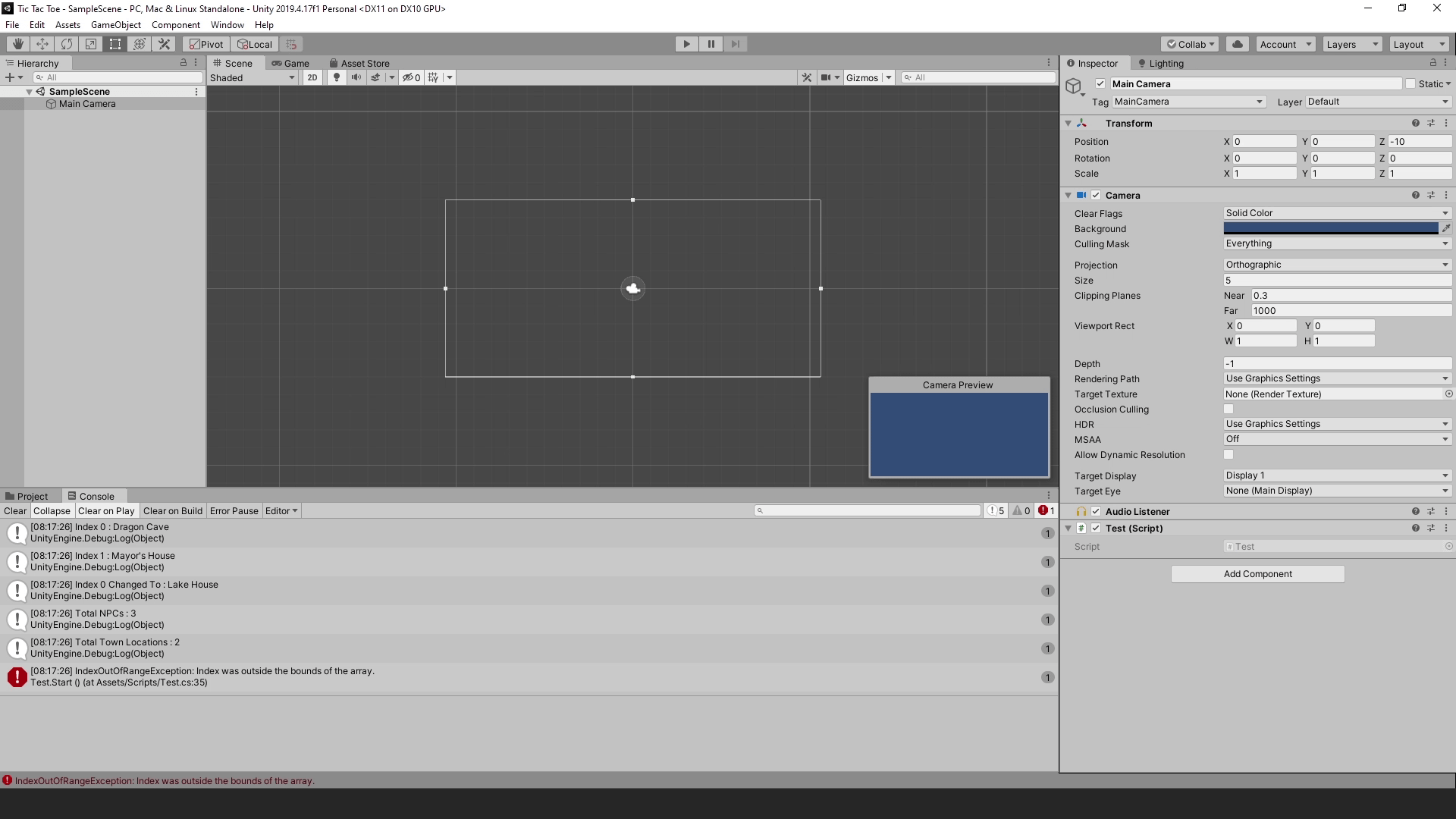
The error says IndexOutOfRangeException and also tells you more details about where this error originated from.
Now, let me explain what’s going on.
if you try to read a value at an index that is greater than the size of the array minus 1,
then you will get a runtime error saying that Index was outside the bounds of the array.
Which is basically programming lingo for your program saying, dude where’s the index, there ain’t nothing there.
In our case, length of the _townLocations array is 2,
So if you use a number larger than, size of the array – 1 i.e. 2 – 1, which is 1, then you will get this error.
This type of errors are what we call Runtime Errors and they happen when the program is running.
They can’t be caught by the compiler when you are compiling the code and will only be noticed when you are running the program and things start going downhill.
To stop getting this error all i can say is, you are trying to access something that doesn’t exist and that means that there is some logical error on your part when you wrote the code.
Just remember that an Array starts at 0 and the last element of an Array is at -> size of the array – 1.
Conclusion
Well folks, that does it for this article.
There is more to arrays then what i have shown in this article, like multidimensional arrays and jagged arrays but they are separate topic of their own and will have their dedicated articles.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords