Overload Resolution is a concept that comes into play because of Optional Parameters that we learned how to use in the last article.
For those who have not been following this article series,
In the last article, we went over everything related to various important types of Parameters and Arguments available to you when developing games using C# and Unity,
if you don’t know anything about methods, then i suggest that you start from the first article
I’ve divided my explanation of Methods in 4 different articles as otherwise the articles would be too long.
List Of All Articles To Master Methods In C#
- Part 1 – Basics Of Methods
- Part 2 – Multiple, Required and Optional Parameter + Named Arguments
- Part 3 – Overload Resolution <— Currently You Are Here! :p
- Part 4 – In, Out and Ref Keywords
In this article, I’ll will be covering the concept of Overload Resolution and will go over
- How your program uses the concept of Overload Resolution to resolve the ambiguity of calling Overloaded Methods and decide on which of the Overloaded Method to call.
- We will see how the behavior of your program changes based on the Type of Parameters you have defined when defining the Overloaded Method.
- We will also see the change in behavior based on the Arguments we supply when making the call to the Overloaded Method.
- While going through all these points we will also have Code Examples and Unity Console Logs for all of them based on an RPG Analogy
So, without further ado, let’s dive directly into it!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Game Development is one of those professions that needs a couple of different professions to work in conjunction to be done right.
If you are someone completely new to Game Development and don’t know much about it,
then you might be interested in the these articles on Intro To Game Development and Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
in which i have talked about the Art of Game Development, Various Roles in Game Dev, Softwares you will need based on the different categories of Game Development
and about the Recipe of Success that will help you in having the right mindset as you get into Game Dev.
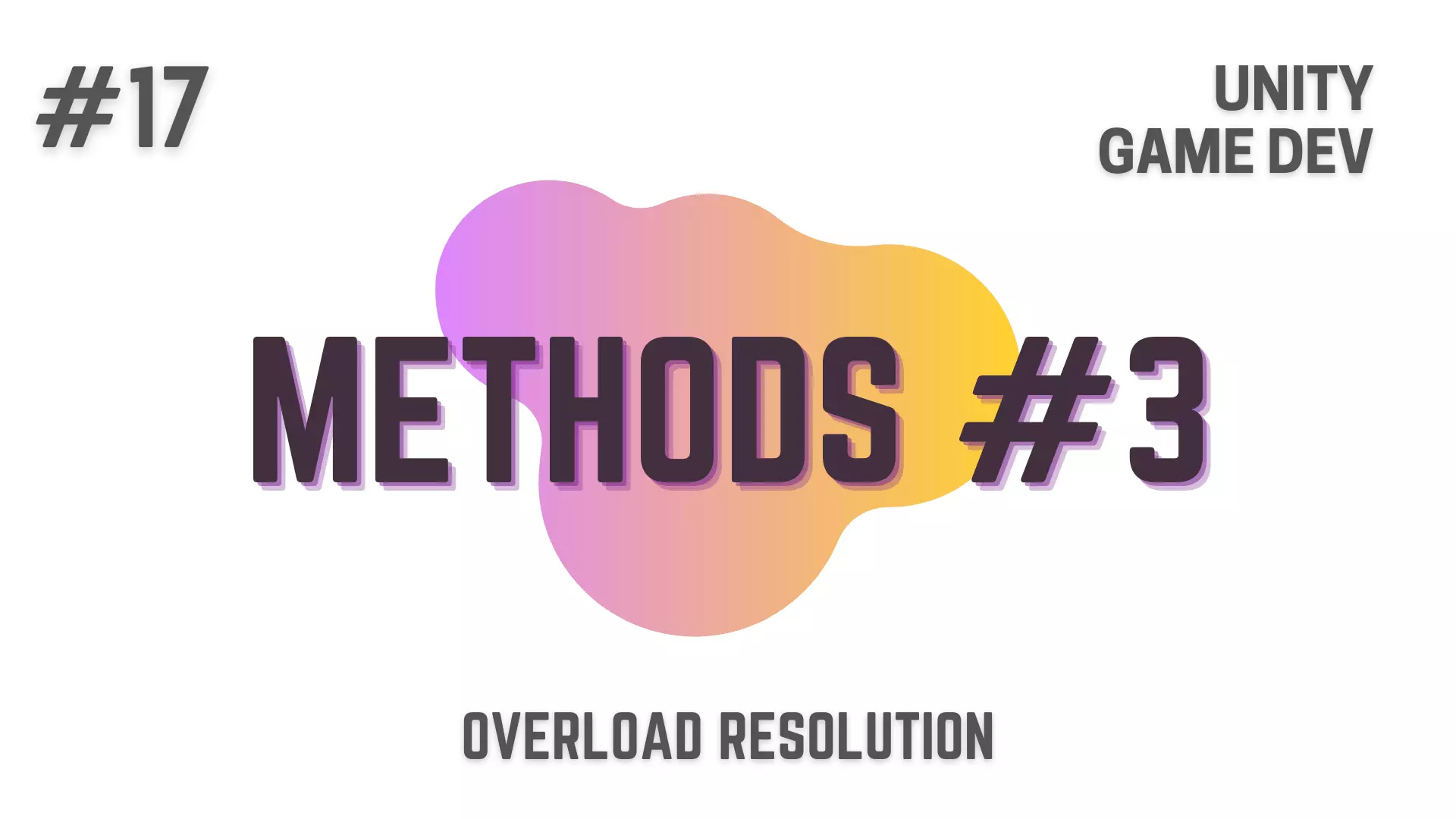
Problem With Overloaded Methods
Overloaded Methods are those methods where we use the same Method Name but have different versions of the methods based on the Parameters and Type of Parameters.
Now, when we use Overloaded methods there comes the question of
to which of the multiple methods, will the Method Call go to?
because now two or more methods have the same method name
So to kinda of give you a context, let’s go to the code.
Now this is a bit important so pay attention.
Let’s first copy and paste code from the Methods in C# | Part 2 – Multiple, Required and Optional Parameter + Named Arguments article
then make a duplicate of the carMovement method,
remove the Optional Parameters from it’s method definition
and also modify it’s Debug.Log as shown below.
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, useBoost: false);
carMovement(1f, 1f, 180f);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f, float boostLevel = 0f, bool useBoost = false)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed)
{
Debug.Log("Second Method - Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed);
}
Now as you can see above,
After the modification these two methods have identical Required Parameters
while only one of them has Optional Parameters
Some of you might already have realized that this would be a huge problem
As, if you make a method call right now
with Arguments that only fulfill the required parameters
you don’t know which of these two methods will be called
and that sort of ambiguity in your code is never good.
Solving The Said Problem Using Overload Resolution
This is where the concept of Overload Resolution comes into play
and will help you understand
how the compiler will decide which of the two methods to use for this method call.
So in a case like this, where the compiler is faced with a choice
where one method does not have any Optional Parameters
while the other method contains one or more Optional Parameters
and both of these methods are otherwise completely identical
then, the compiler will select the method that does not have any optional parameters.
Now let’s execute this piece of code in Unity and check the logs.
Unity Log | Overload Resolution in C# | Same Required Parameters But One Method With No Optional Parameters
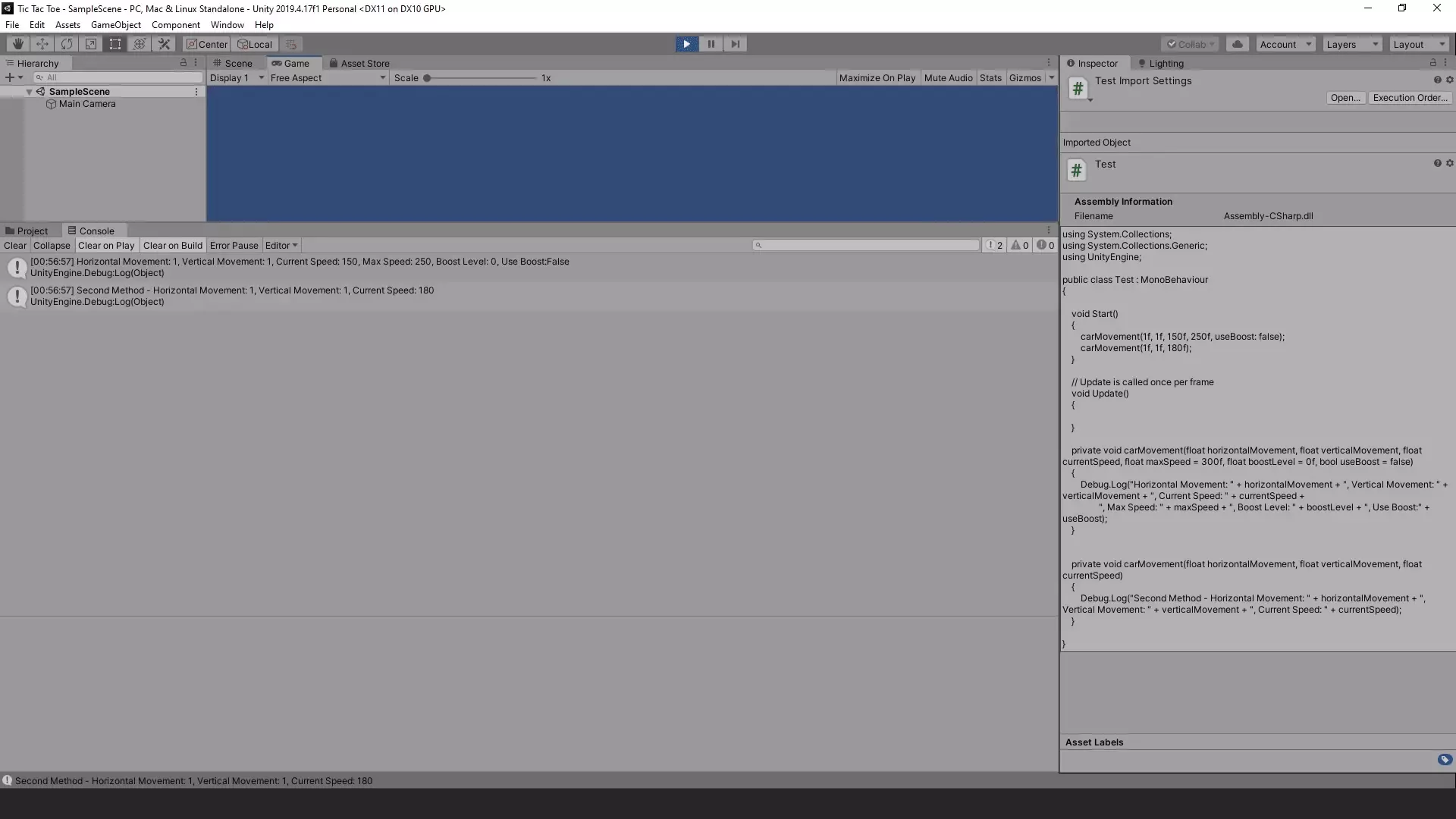
As you can see we have printed the Debug.Log in the method Without Optional Parameters.
How Will The Compiler Resolve The Method Overload If Both Methods Contain Identical Required Parameters And Contains One Or More Identical Optional Parameters?
Back to the code, now in the method without any optional parameters
let’s consider adding one optional parameter.
So let’s add maxSpeed as an Optional Parameter like show below
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, useBoost: false);
carMovement(1f, 1f, 180f);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f, float boostLevel = 0f, bool useBoost = false)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f)
{
Debug.Log("Second Method - Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed);
}
Now some of might be thinking that
the compiler will pick the method with one optional parameter
because it has less number of optional parameters then the other method
but if you think so, then you would be wrong.
In this case, as both the methods contain optional parameters
and require default values to be filled in by the compiler.
And As, they are otherwise identical
the compiler will raise an error.
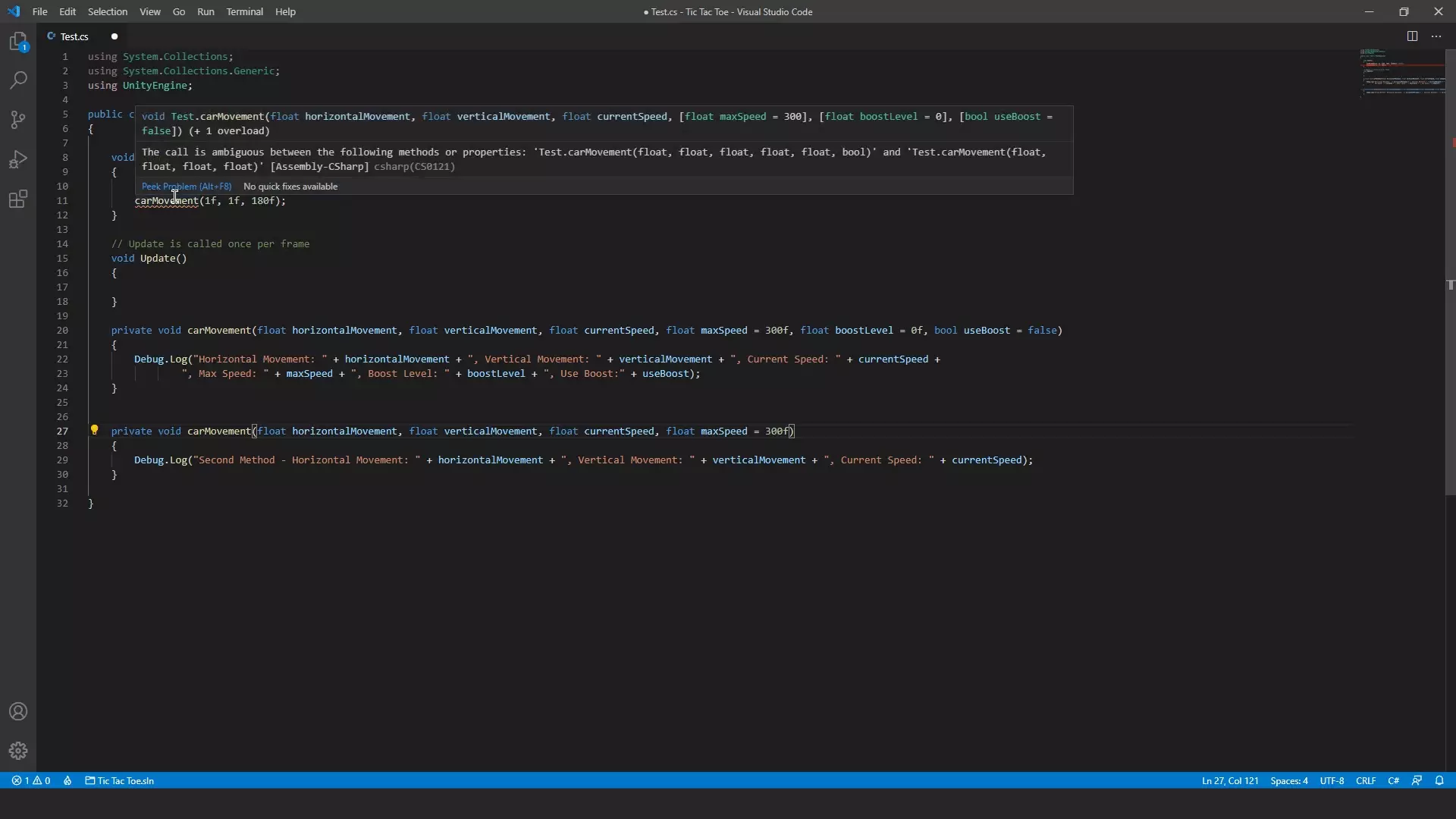
The fact that, one of the methods only requires a single optional parameter
while the other method requires three optional parameters
is completely irrelevant.
Overload Resolution in C# | Passing Argument For maxSpeed Parameter
Now, if in the Start Method,
we pass an argument for maxSpeed
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, useBoost: false);
carMovement(1f, 1f, 180f, 280f);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f, float boostLevel = 0f, bool useBoost = false)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f)
{
Debug.Log("Second Method - Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed);
}
then the error will disappear
and if you hover over the method call
you will be able to see that it is calling the second method
as you can see in the image below
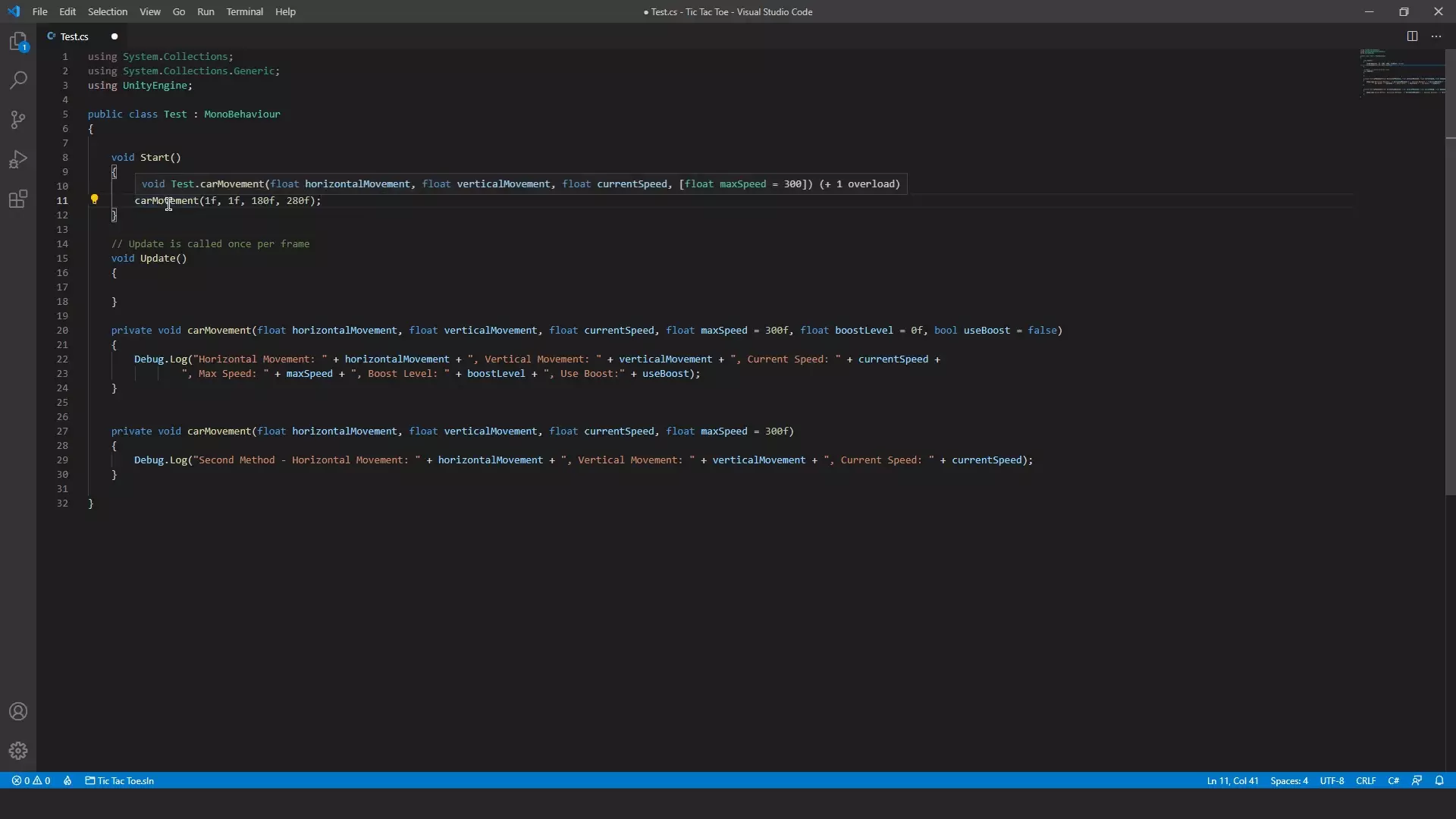
The reason i believe this is happening is because
all the parameters for the second method had arguments passed to them
while in the first method there are still two optional parameters
that needs the compiler to fill in default values.
Overload Resolution in C# | What Will Happen If We Now Add boostLevel Parameter To The Parameter List
if the second method also had Another Optional Parameter i.e. boostLevel Parameter
for which we did not pass an argument in the method call
like shown below
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, useBoost: false);
carMovement(1f, 1f, 180f, 280f);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f, float boostLevel = 0f, bool useBoost = false)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f, float boostLevel = 0f)
{
Debug.Log("Second Method - Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed);
}
then we would get an error again
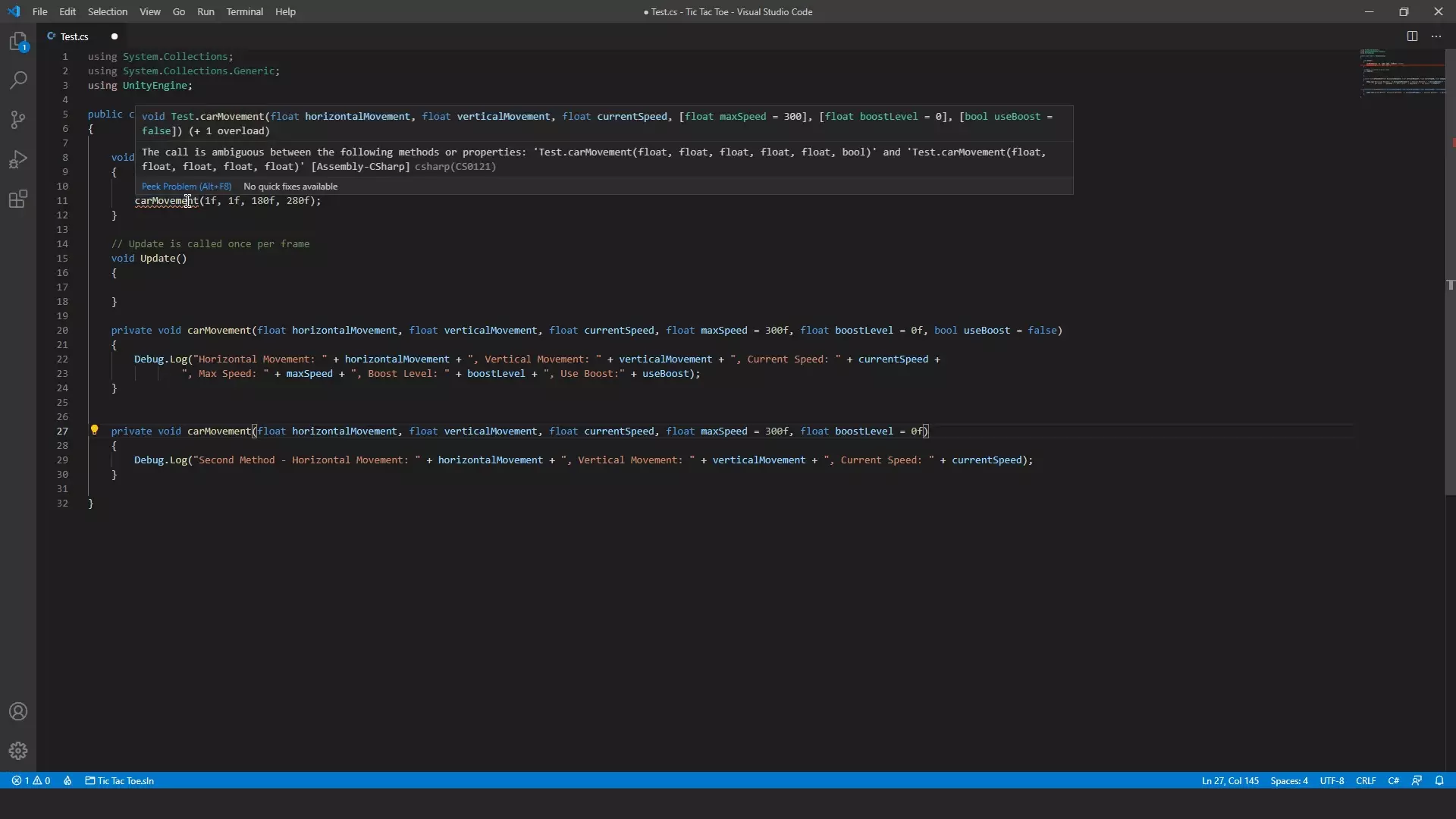
as now both the methods need the compiler to fill in default values to the optional parameters
Again, the fact that the second method requires less number of optional parameters
is completely irrelevant here.
If you are really interested in this topic, then i would suggest that you read the official MSDN documentation on Methods in C#
which contains some parts related to how Overload Resolution Works in C#
as this topic is quite important to understand.
Also this does not end our journey with methods in C#
because there are still things to cover about methods that are pretty important
but again just like the previous article
as this article is already more than a bite size
I’ll be covering all the remaining things about methods in the next article.
List Of All Articles To Master Methods In C#
- Part 1 – Basics Of Methods
- Part 2 – Multiple, Required and Optional Parameter + Named Arguments
- Part 3 – Overload Resolution <— Currently You Are Here! :p
- Part 4 – In, Out and Ref Keywords
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords