There are a whole lot of Keywords in C# that are reserved and are used for specific purpose when coding and the static Keyword is one of those keywords.
In this Article, I’m going to talk about the static modifier / keyword and am going to cover
- Static keyword and what it represents when writing code in C#
- What are Instance Variables & Class Variables and the difference between them.
- What are Instance Methods & Class Methods and the difference between them.
- After that, we will go to the code and see how you can use the static modifier / keyword in your C# Scripts
- While doing that we will also see, how you will need to modify your code, to make the static variables and methods work correctly without Compile Time Errors.
So, without further ado, let’s get on with it!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Predictability and Unpredictability are two opposing forces that can lead to you game becoming a major hit or a failure
and it is also something that most of the Game Developers forget to think about when they are Designing and Developing their Games.
Most of the Game Developers consciously or subconsciously lean towards making their games easily predictable
but they fail to realize that, a highly predictable game will become stale for their players pretty quickly
as their is nothing new and exciting happening in your game.
So to help you in understanding the importance of adding Unpredictability to your Games,
I’ve written this article on Add Some Unpredictability To Your Games
in which I’ve talked about Unpredictability with Real Game Examples to make you better understand Unpredictability.
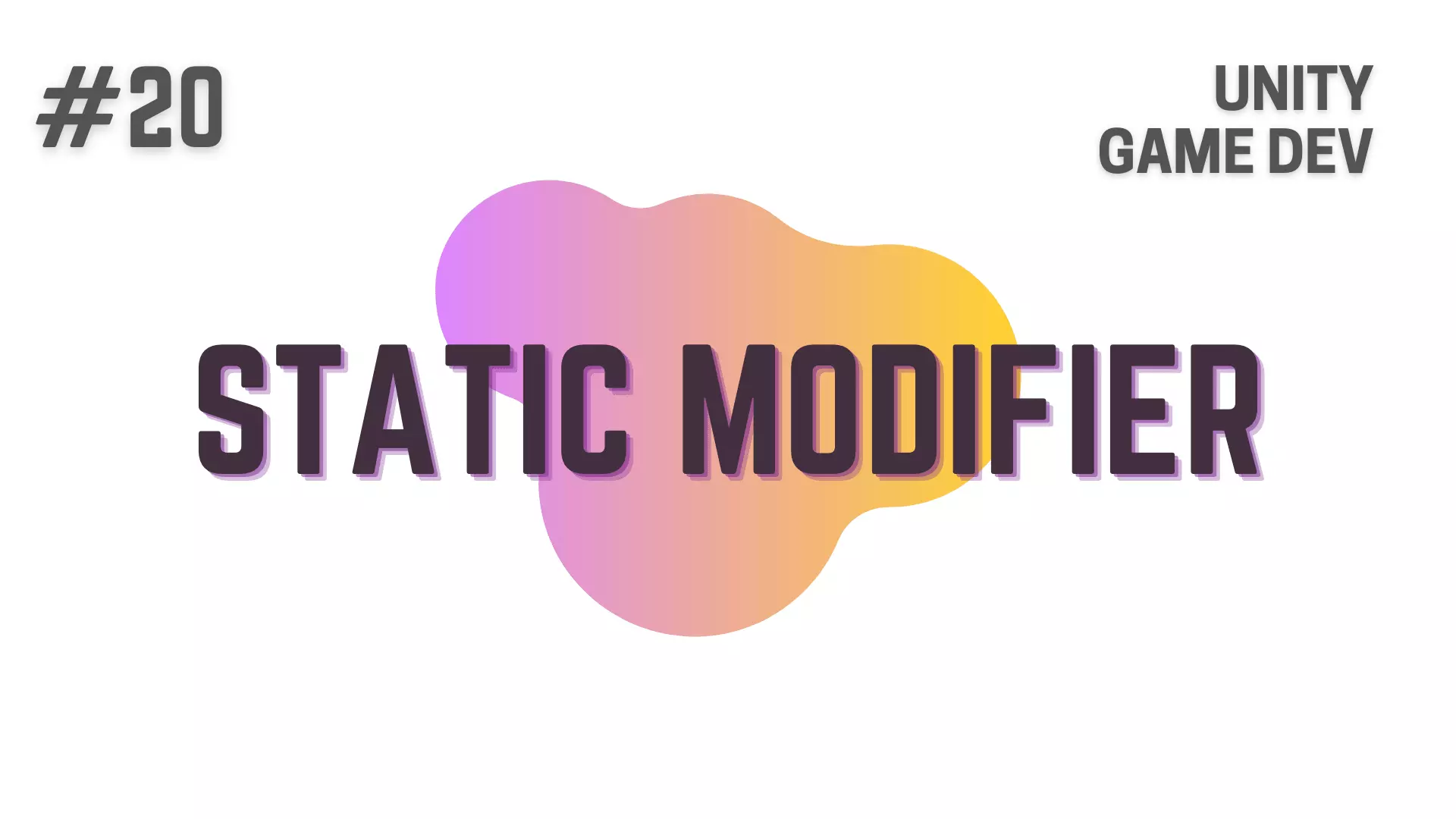
Instance Variables and Instance Methods V/S Class Variables and Class Methods in C#
Now before we go to the C# Script and start coding using the static modifier you need to understand what the static modifier represents
and to understand that you will need to understand the difference between
Instance Variables and Instance Methods V/S Class Variables and Class Methods
So, when you declare a variable inside the class as shown below
private string _swordName;
what you are doing is, you are declaring an Instance Variable.
Every time you create an instance of a class, it will creates a copy of these instance variables for the new instance.
While when a Class Variables is declared the runtime system allocates Class Variables only once per Class
regardless of the number of instances created of that Class.
The system allocates memory for Class Variables the first time it encounters the Class and after the allocation
All the instances of the class will share the same copy of the Class’s Class Variables.
Methods are similar i.e. you can declare Instance Methods and Class methods.
Instance Methods can operate on the Current Object’s Instance Variables and they also have access to the Class Variables.
Class Methods, on the other hand, can only access Class Variables and will not be able to access the Instance Variables declared within the Class,
unless you create a new object or pass an object as an argument to the class method and then access the instance variable through this object.
By default, a member declared within a class is an Instance Member.
To declare a Class Variable or a Class Method, you can use the static modifier when defining them.
Also, you don’t need an instance to make a Method Call to a Class Method.
How To Use Static Modifier in C# | Unity Game Development Tutorial
Using Static Modifier To Make A Class Variable in C#
To show how to use static modifier within your C# Scripts,
First let’s copy and paste some code from the article about Classes, Objects and Constructors as shown below
// Everything below is inside the template_sword Class
private string _swordName;
private byte _piercingDamage;
private byte _slashingDamage;
private byte _bleedingDamage;
private byte _elementalDamage;
private byte _manaConsumption;
private short _weaponHP;
// Start is one of the inbuilt methods in Unity
public void Start()
{
template_sword Rapier001 = new template_sword();
template_sword WarSword001 = new template_sword();
Rapier001._swordName = "Rapier001";
Rapier001._piercingDamage = 5;
Rapier001._slashingDamage = 1;
Rapier001._bleedingDamage = 3;
Rapier001._elementalDamage = 0;
Rapier001._manaConsumption = 0;
Rapier001._weaponHP = 100;
WarSword001._swordName = "WarSword001";
WarSword001._piercingDamage = 1;
WarSword001._slashingDamage = 5;
WarSword001._bleedingDamage = 3;
WarSword001._elementalDamage = 0;
WarSword001._manaConsumption = 0;
WarSword001._weaponHP = 150;
Debug.Log("Sword Name : " + Rapier001._swordName + " Piercing Damage : " + Rapier001._piercingDamage + " Slashing Damage : " + Rapier001._slashingDamage + " Bleeding Damage : " + Rapier001._bleedingDamage + " Elemental Damage : " + Rapier001._elementalDamage + " Mana Consumption : " + Rapier001._manaConsumption + " Weapon HP : " + Rapier001.weaponHP);
Debug.Log("Sword Name : " + WarSword001._swordName + " Piercing Damage : " + WarSword001._piercingDamage + " Slashing Damage : " + WarSword001._slashingDamage + " Bleeding Damage : " + WarSword001._bleedingDamage + " Elemental Damage : " + WarSword001._elementalDamage + " Mana Consumption : " + WarSword001._manaConsumption + " Weapon HP : " + WarSword001.weaponHP);
WarSword001.Attack();
Rapier001.Parry();
}
public void Attack()
{
Debug.Log("Player Attacked Using: " + _swordName);
}
public void Parry()
{
Debug.Log("Enemy Parried Using: " + _swordName);
}
public void Defend()
{
Debug.Log("Player Defended Using: " + _swordName);
}
Now, let’s try to convert the _swordName Variable which is right now an Instance Variable into a Class Variable, by using the static modifier.
To do this, we will add the static modifier after the Access Modifier where we are declaring the _swordName variable as shown below.
private static string _swordName;
Now when you do this you will get a couple of red underlines in VS Code, signifying that you have Compile Time Errors
as show in the image below
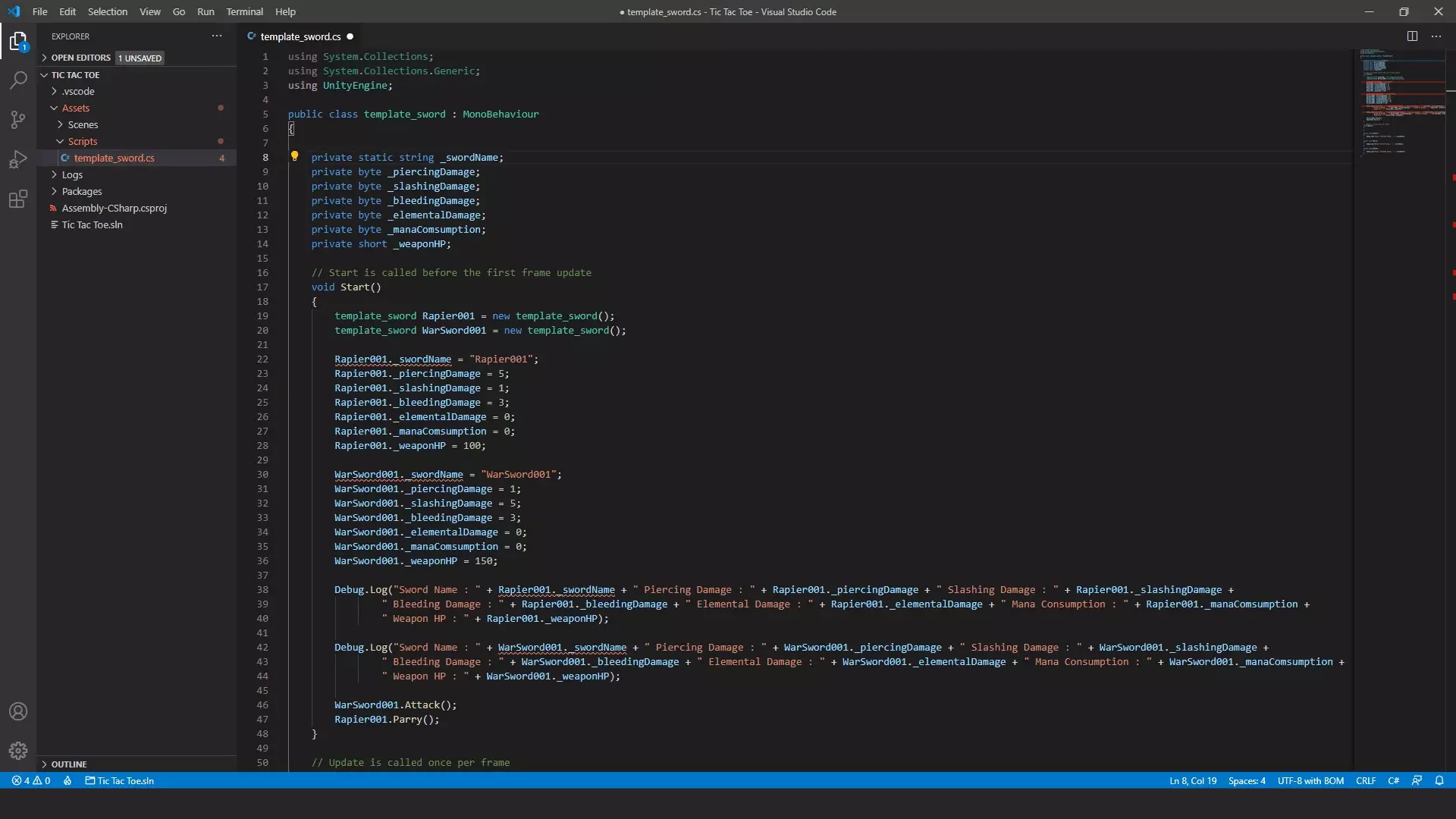
We are getting these Compile Time Errors because now _swordName is a Class Variable,
and you can’t access it like you would an Instance Variable.
To access the _swordName Class Variable, you can’t go through an instance of the Class anymore
instead you have to access it directly by Removing the Name of the Instance i.e. the Object Name and the dot ( . ) before it
By doing this the red underlines will go away as shown in the image below.
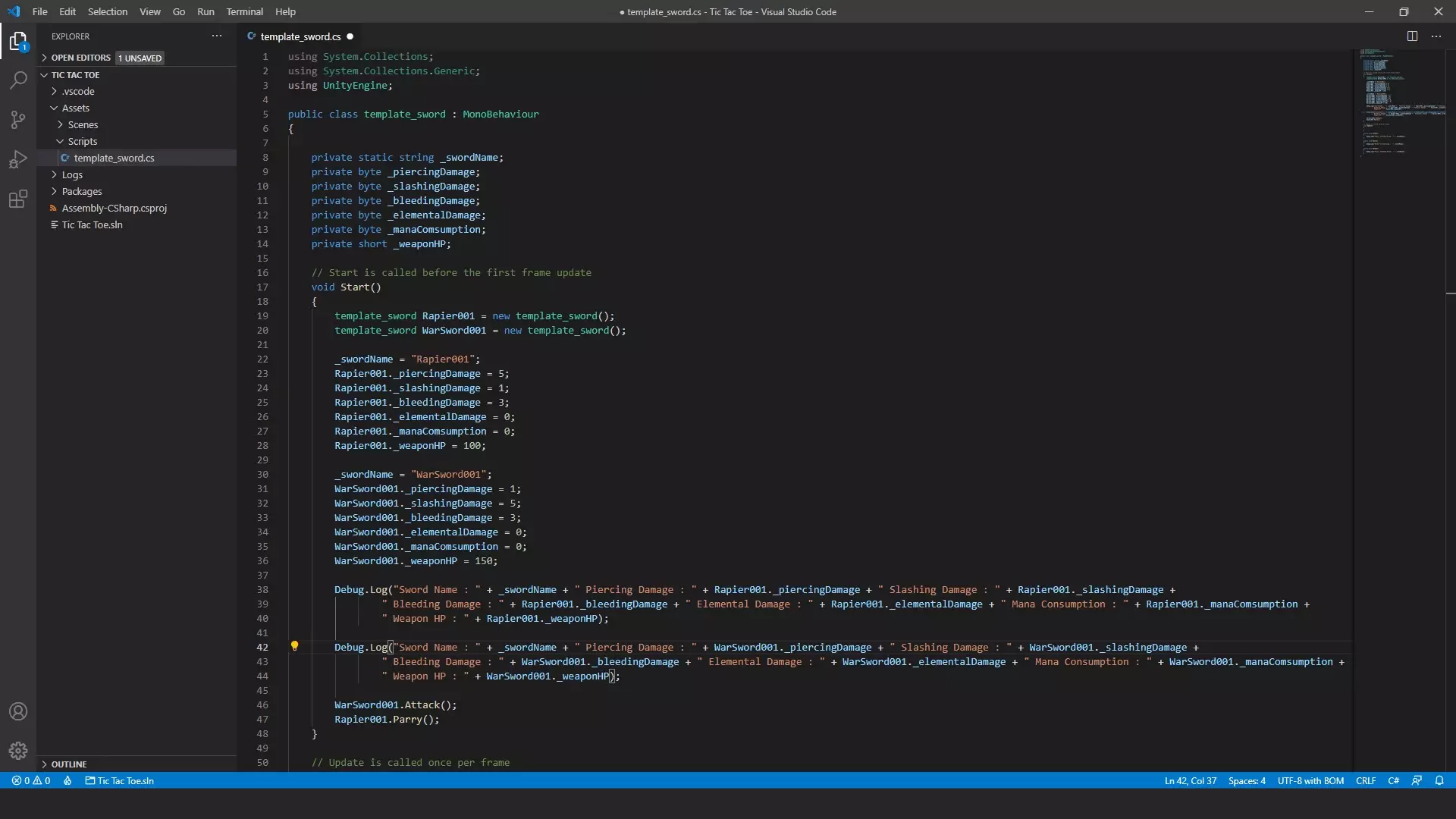
Now making _swordName a static variable i.e. a class variable, will cause another problem
and that is, since both the instance of the swords are now sharing the _swordName variable
if we execute this piece of code in Unity and check the logs
both the swords will have the same name i.e. WarSword001
as in our code we are first setting _swordName to Rapier001
and then overwriting it’s value to WarSword001.
So, let’s execute this piece of code in Unity and check the logs
to make sure that the _swordName variable with the static modifier works as we are expecting.
Executed Code | Using Static Modifier To Make A Class Variable in C#
// Everything below is inside the template_sword Class
private static string _swordName;
private byte _piercingDamage;
private byte _slashingDamage;
private byte _bleedingDamage;
private byte _elementalDamage;
private byte _manaConsumption;
private short _weaponHP;
// Start is one of the inbuilt methods in Unity
public void Start()
{
template_sword Rapier001 = new template_sword();
template_sword WarSword001 = new template_sword();
_swordName = "Rapier001";
Rapier001._piercingDamage = 5;
Rapier001._slashingDamage = 1;
Rapier001._bleedingDamage = 3;
Rapier001._elementalDamage = 0;
Rapier001._manaConsumption = 0;
Rapier001._weaponHP = 100;
_swordName = "WarSword001";
WarSword001._piercingDamage = 1;
WarSword001._slashingDamage = 5;
WarSword001._bleedingDamage = 3;
WarSword001._elementalDamage = 0;
WarSword001._manaConsumption = 0;
WarSword001._weaponHP = 150;
Debug.Log("Sword Name : " + _swordName + " Piercing Damage : " + Rapier001._piercingDamage + " Slashing Damage : " + Rapier001._slashingDamage + " Bleeding Damage : " + Rapier001._bleedingDamage + " Elemental Damage : " + Rapier001._elementalDamage + " Mana Consumption : " + Rapier001._manaConsumption + " Weapon HP : " + Rapier001.weaponHP);
Debug.Log("Sword Name : " + _swordName + " Piercing Damage : " + WarSword001._piercingDamage + " Slashing Damage : " + WarSword001._slashingDamage + " Bleeding Damage : " + WarSword001._bleedingDamage + " Elemental Damage : " + WarSword001._elementalDamage + " Mana Consumption : " + WarSword001._manaConsumption + " Weapon HP : " + WarSword001.weaponHP);
WarSword001.Attack();
Rapier001.Parry();
}
public void Attack()
{
Debug.Log("Player Attacked Using: " + _swordName);
}
public void Parry()
{
Debug.Log("Enemy Parried Using: " + _swordName);
}
public void Defend()
{
Debug.Log("Player Defended Using: " + _swordName);
}
Unity Log | Using Static Modifier To Make A Class Variable in C#
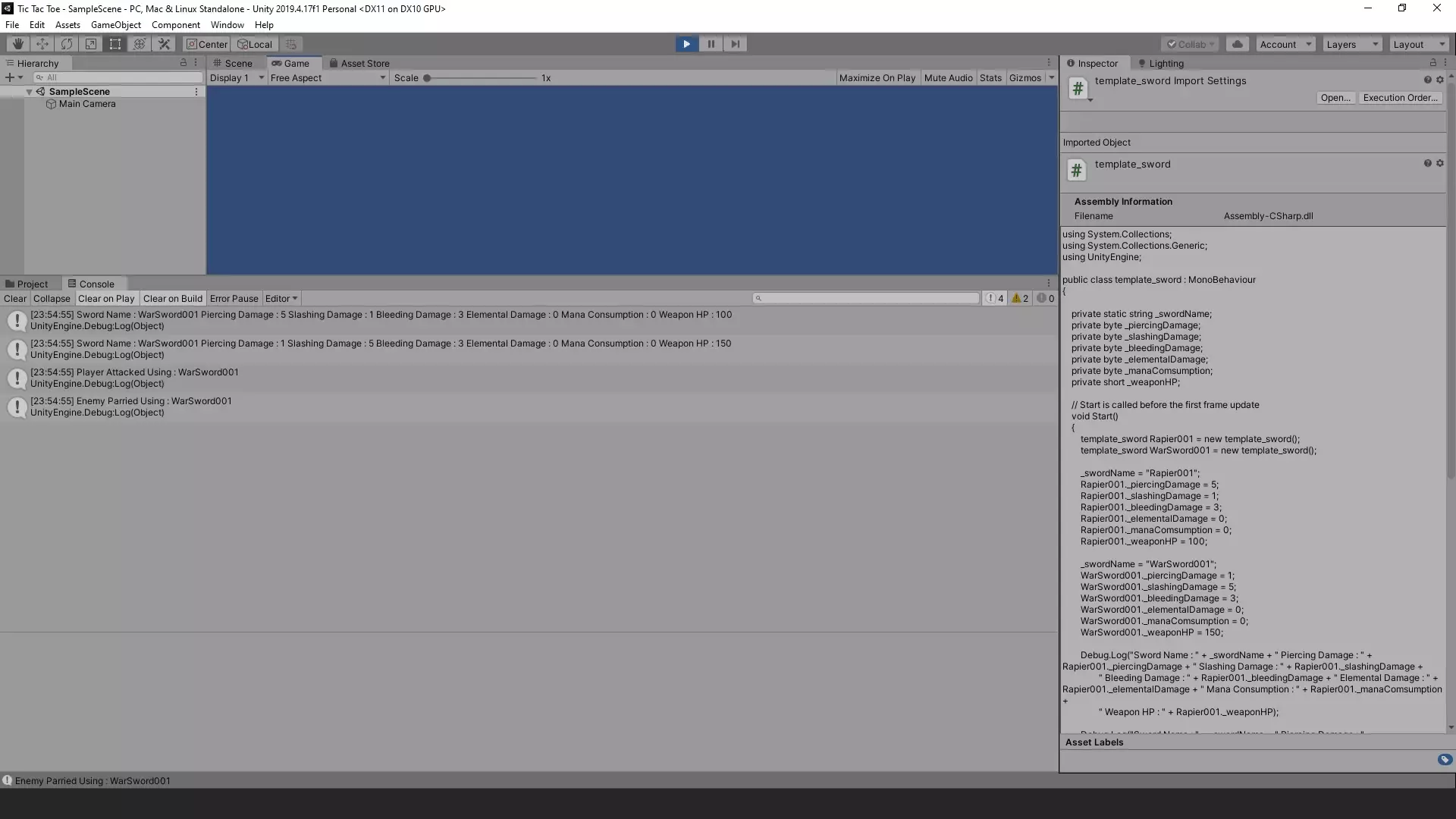
As you can see both the swords have the same name i.e. WarSword001
while the other variables were set the same as before.
So everything worked just as we expected.
Using Static Modifier To Make A Class Method in C#
Back to the code, now let’s convert the Attack Method from being an Instance Method into a Class Method by using the static modifier.
To do this, we will add the static modifier to the Instance Method that we are trying modify
just like how we added the static modifier after the Access Modifier when we are declaring an Instance Variable.
// Not showing the other code.
public static void Attack()
{
Debug.Log("Player Attacked Using: " + _swordName);
}
Now when we do this, we will get a red underline beneath WarSword001 where we were making a Method Call to the Attack Method
as you can see in the image below
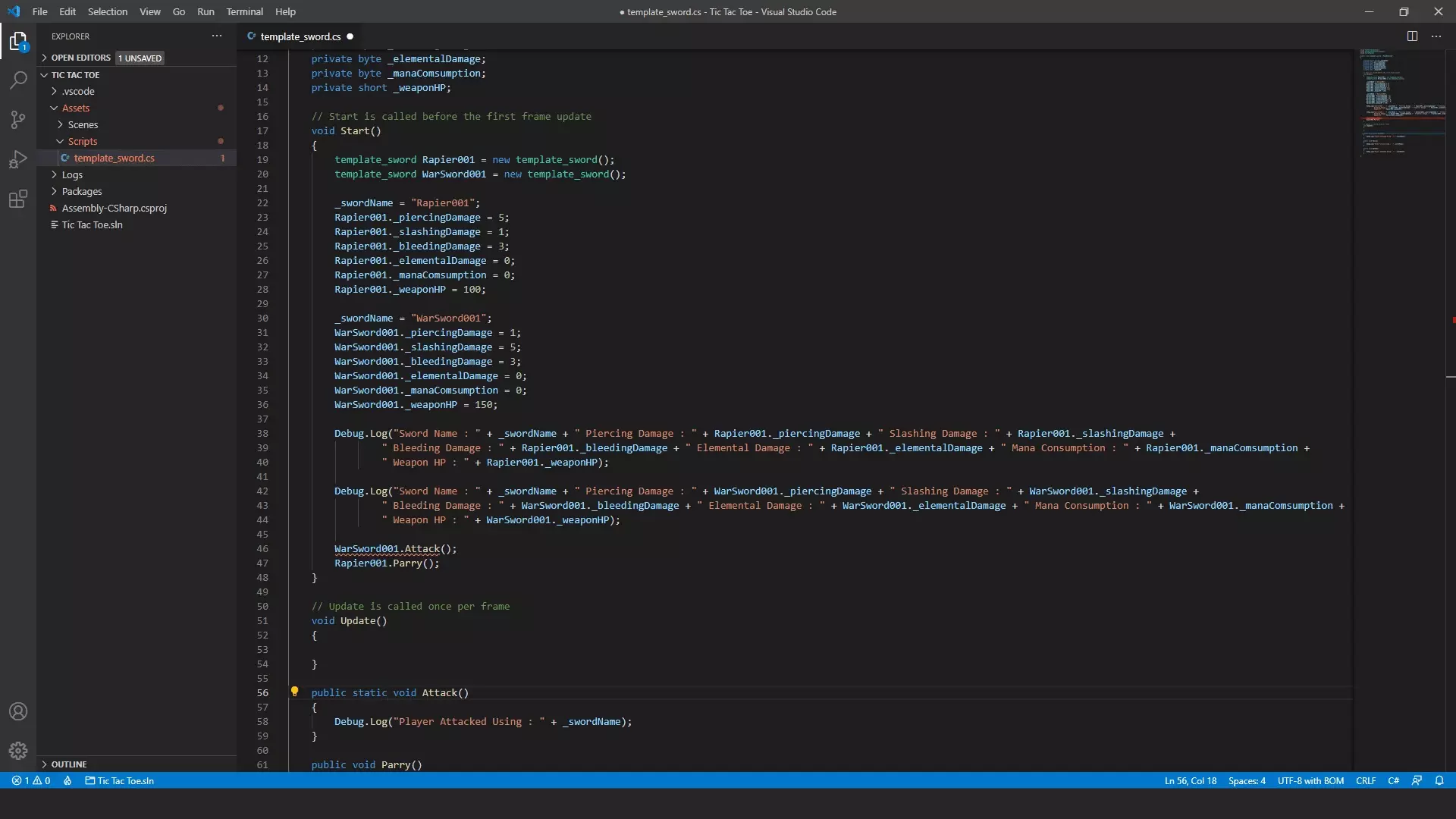
We are getting this Compile Time Error because after the modifications that we did above
we are now trying to call a Class Method like we would call an Instance Method
and we can’t do that.
To make a method call to a Class Method i.e. static method
we don’t need to go through an instance of the class i.e. an Object like WarSword001,
instead we can make a method call to it directly
so if we remove WarSword001 and dot ( . ) before the Method Call where we are getting the error,
the error will be gone as you can see below
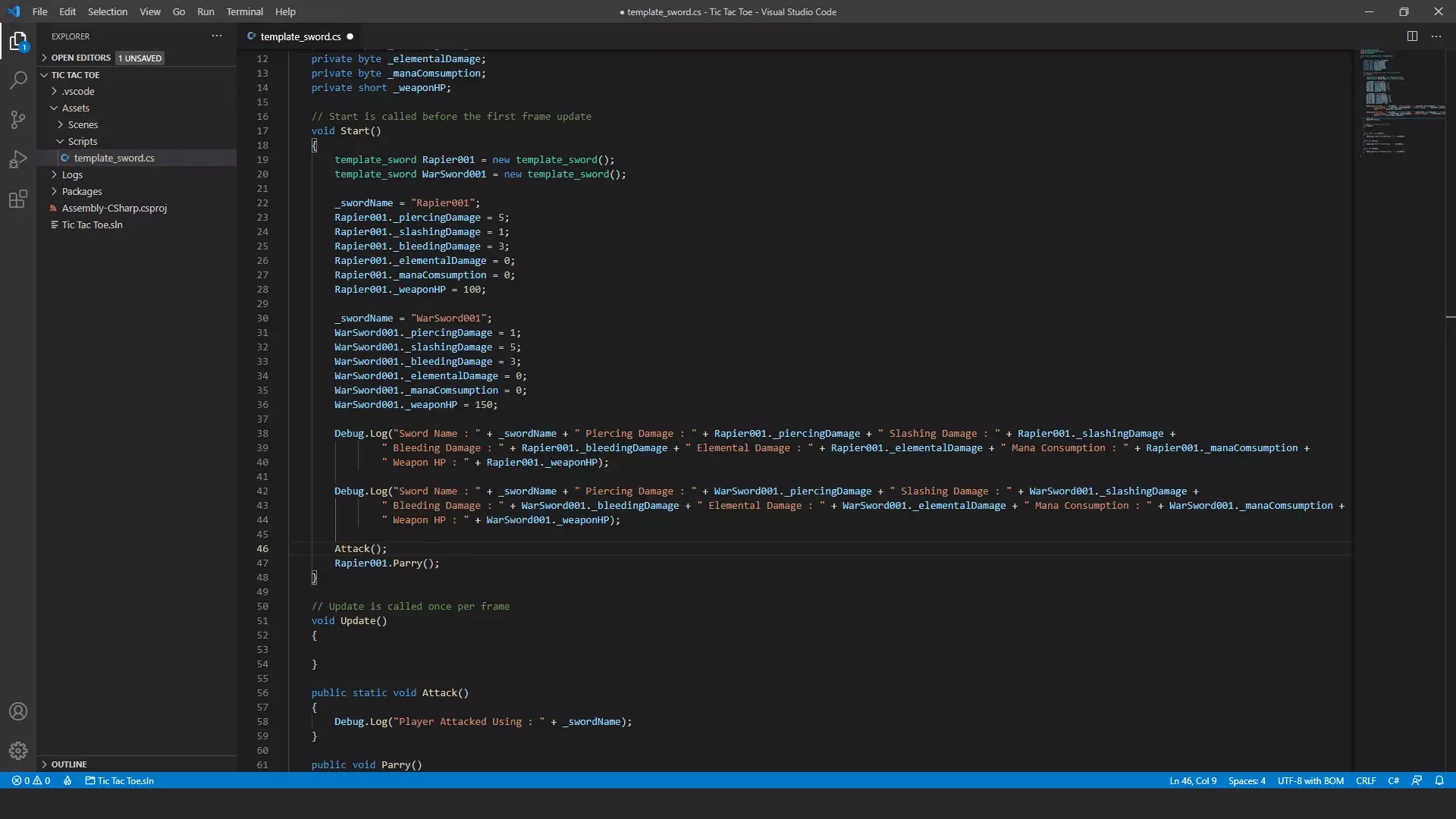
Now, we are able to access the _swordName variable inside this static method i.e. Attack Method, because _swordName is also a static variable
if we remove the static modifier from _swordName and convert it back to an Instance Variable,
then as you can see in the image below, we start getting Compile Time Error inside the Attack Method’s code block.
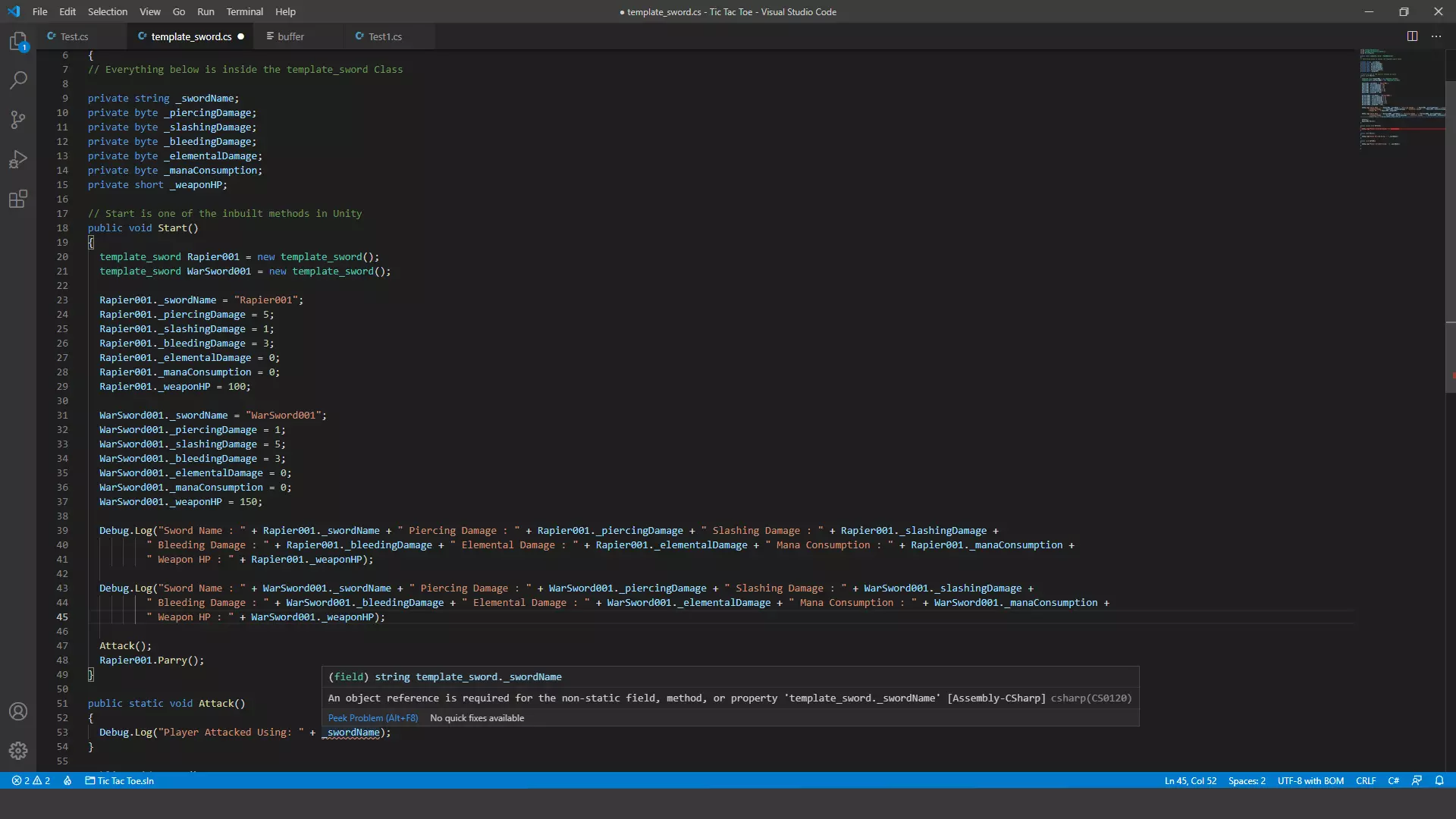
This happens because a Class Methods can’t access an Instance Variable directly, as i had explained at the start of the article.
If you need access to the data stored inside this variable within the Attack Method
then you will either have to make _swordName a Class Variable or you will need to pass it as an Argument to the Attack Method.
With that, i think you would have a good enough understanding
about how to use the static modifier in your own C# Scripts when developing your games
and that you would be able to understand the errors if and when you get them.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords