We have got in touch of Access Modifiers since the article about Variables & Data Types
and since then they have made quite a few appearances in the succeeding articles
but till now other than just giving you a textbook definition about it,
i have not actually explained to you what Access Modifiers are and why they are necessary.
In this Article, I’m going to talk in depth about all the different Access Modifiers available to you when developing your games with Unity and C# and am going to cover
- What are Access Modifiers and what they represent when writing code in C#
- Overview the Concept of Assemblies and how Access Modifiers are connected to Assemblies in C#.
- After that, we will talk about the different Access Modifiers i.e. Public, Private, Protected, Internal, Private Protected, Protected Internal.
- While doing that, we will also understand the difference between each of these Access Modifiers in C#.
So, without further ado, let’s get started!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Predictability and Unpredictability are two opposing forces that can lead to you game becoming a major hit or a failure
and it is also something that most of the Game Developers forget to think about when they are Designing and Developing their Games.
Most of the Game Developers consciously or subconsciously lean towards making their games easily predictable
but they fail to realize that, a highly predictable game will become stale for their players pretty quickly
as their is nothing new and exciting happening in your game.
So to help you in understanding the importance of adding Unpredictability to your Games,
I’ve written this article on Add Some Unpredictability To Your Games
in which I’ve talked about Unpredictability with Real Game Examples to make you better understand Unpredictability.
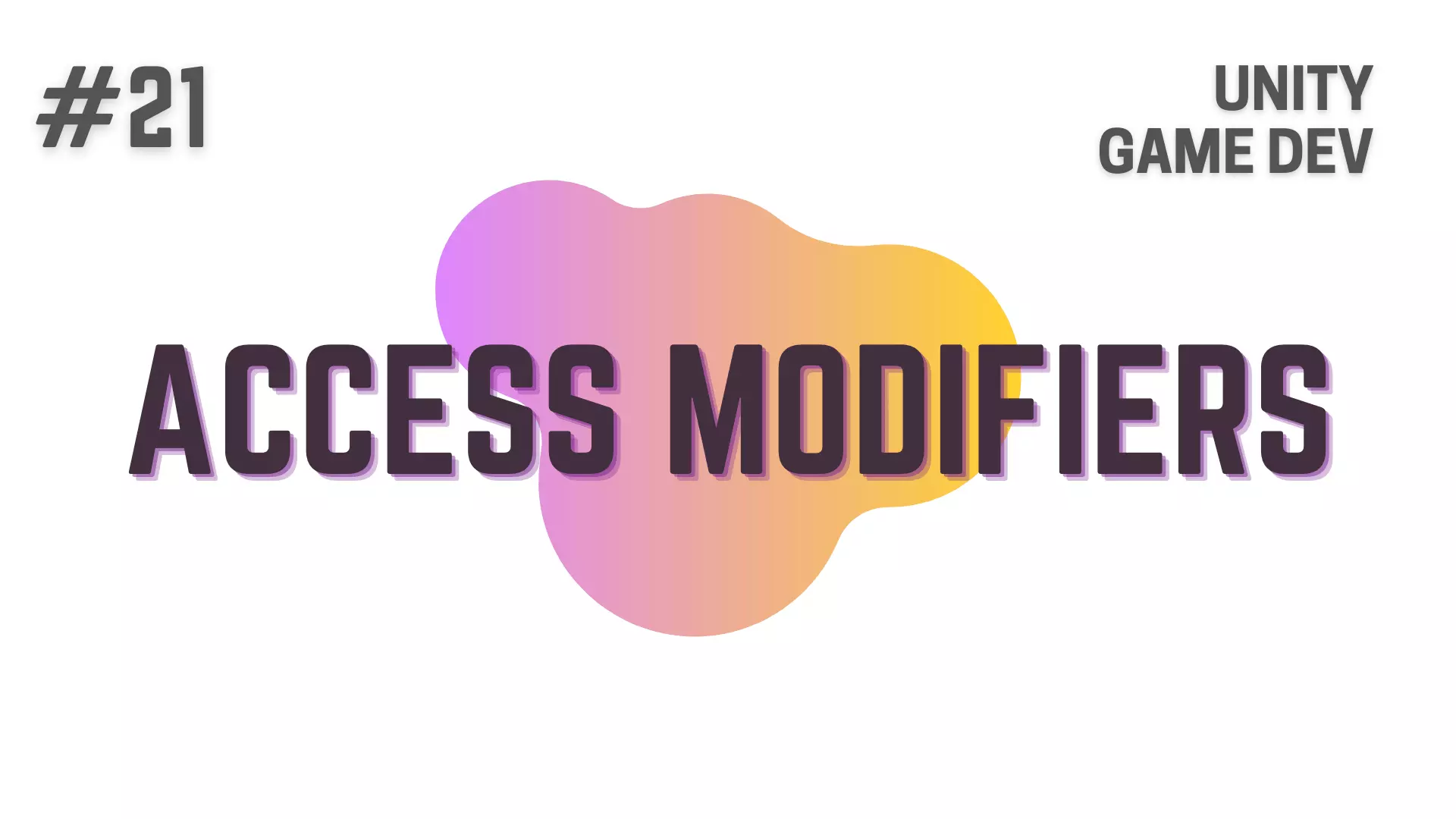
What Are Access Modifiers in C# & Reason For Their Use | Unity Game Development Tutorial
As i have said before in some of the articles, Access Modifiers are used to control the visibility of your Types and Members to other parts of your code.
This means that, Access Modifiers decide where you will be able to access these Types and Members ( For example : Variables )
and be able to read or modify the data stored inside them.
In C#, you have 6 Access Modifiers namely
- Public
- Private
- Protected
- Internal
- Private Protected
- Protected Internal
That lets you access the Types or Members at different scopes.
What are Assemblies in C# & How will They Affect Your Access Modifiers
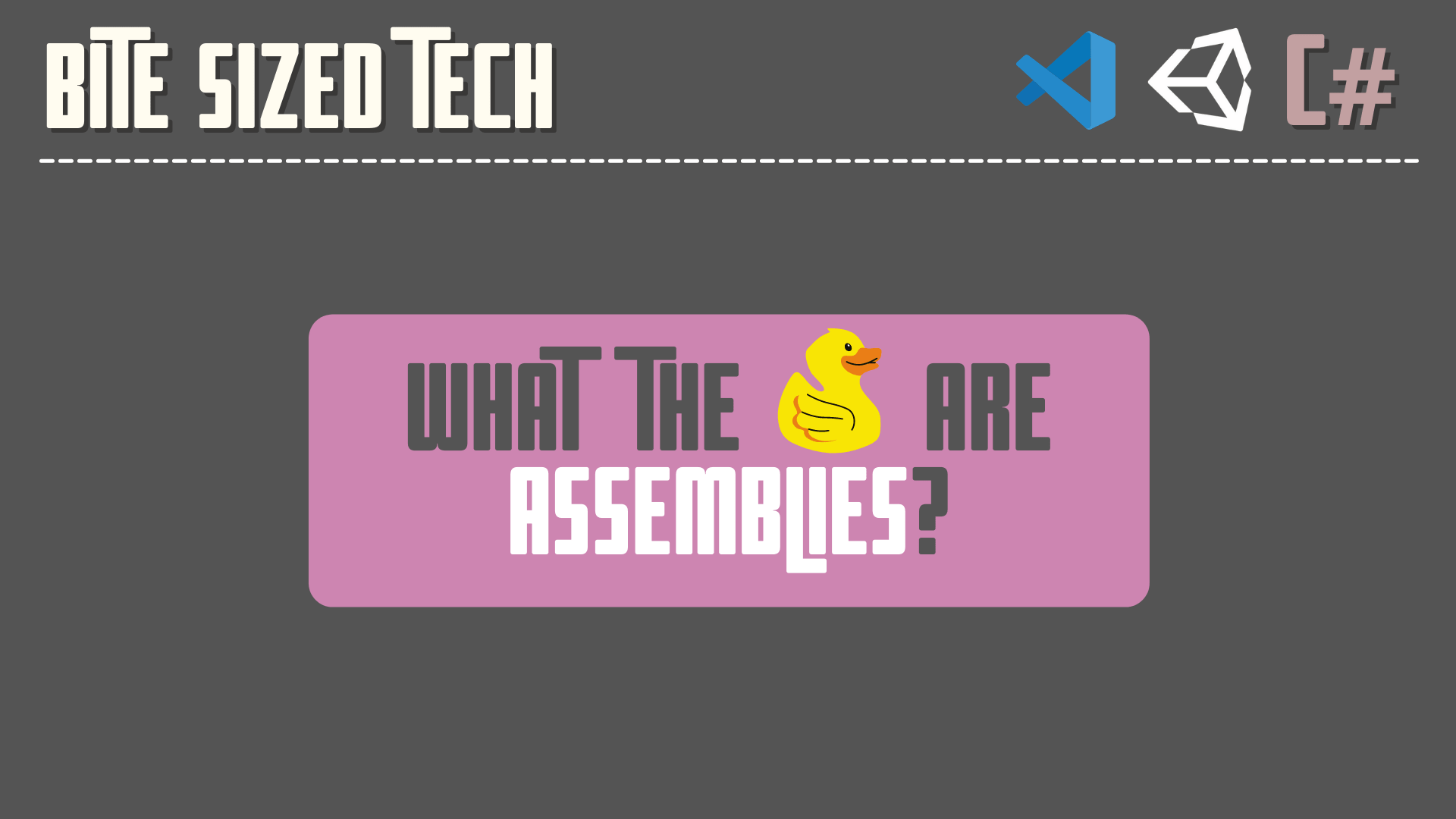
Now before moving forward to explaining each of these Access Modifier, what we need to talk about is Assemblies in C#.
But sadly, even i don’t have a working knowledge of assemblies
so i am not a good resource to learn about it, in any regards.
But, what i could extrapolate from doing a bit of research in the Unity documentation is that
An Assembly is a C# Code Library that contains the compiled classes and structs
that are defined by your various scripts and which also define references to other assemblies.
By default, Unity compiles almost all of your game scripts into a predefined assembly called Assembly-CSharp.dll.
as you can see in the image below.
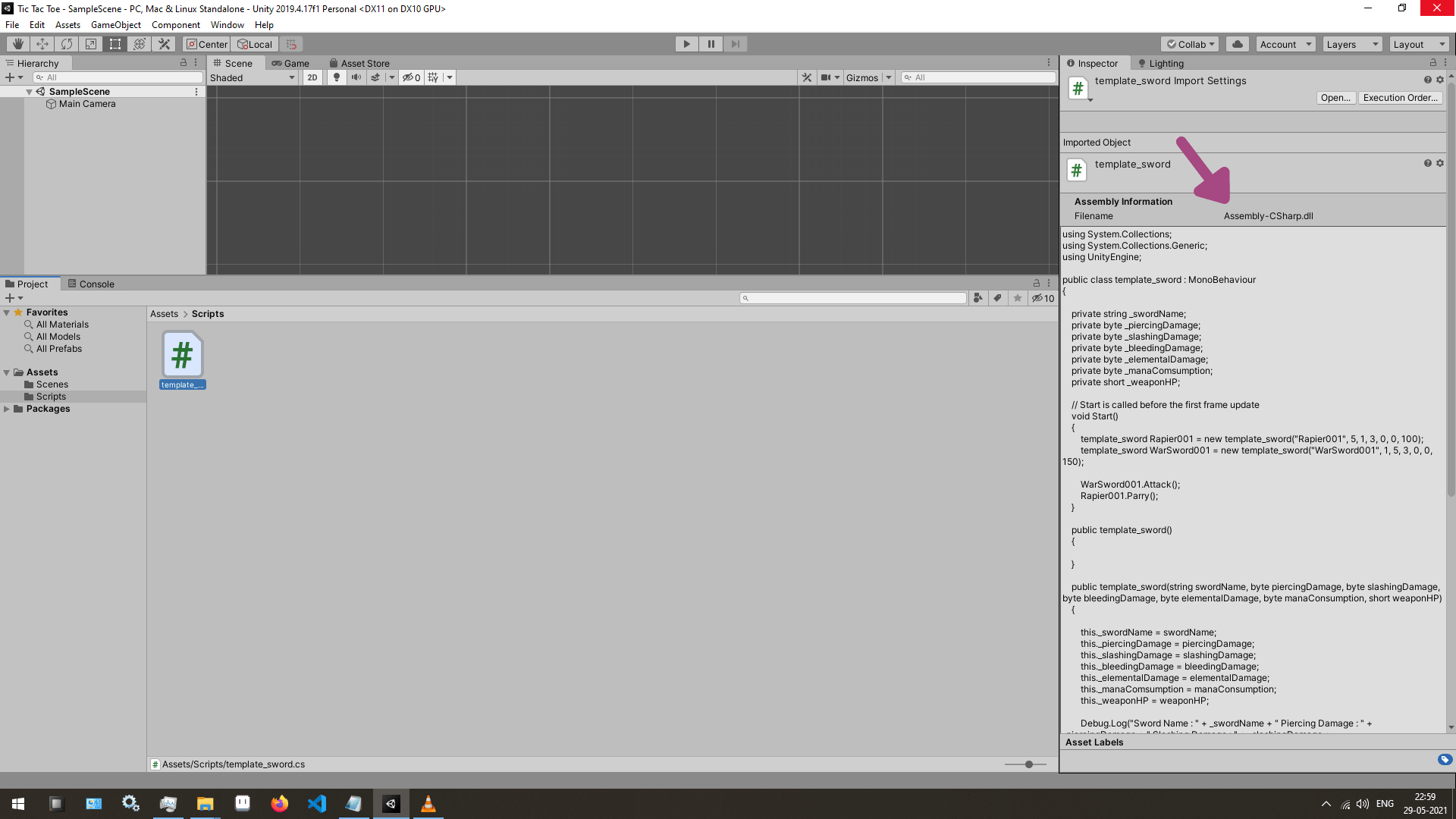
and Unity also creates a few smaller, specialized predefined assemblies.
What this tells me is that, by default, most of the scripts that we will write for our games will all be part of Assembly-CSharp.dll
and we won’t be talking between assemblies most of the time.
I know that as a complete beginner, you might be feeling that VP, what the duck is your point and i can empathize with that.
but the reason I’m going through all these trouble is that
if you go the MSDN page to look at the Specifications for Access Modifiers in C#,
the definitions for them will all be from the perspective of assemblies.
So, before i start talking about Access Modifiers, you needed to know that
by default most of your scripts will be part of the same assembly when you are working with Unity.
With that out of the way, let’s move forward and talk about each of the Access Modifiers one by one.
C# Public Access Modifier | Unity Game Development Tutorial
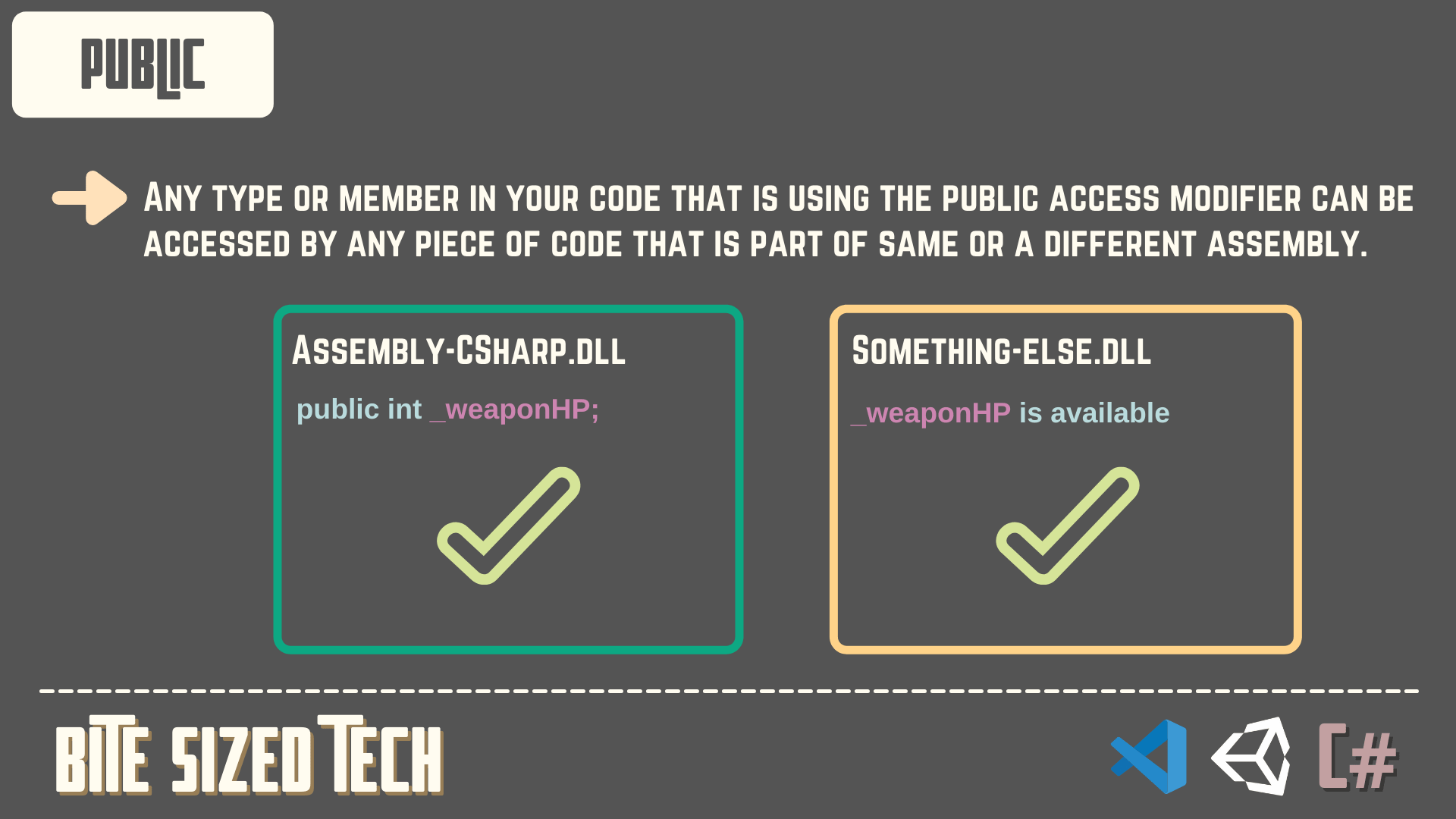
First is the Public Access Modifier,
Any type or member in your code that is using the public access modifier can be accessed by any piece of code that is part of same or a different assembly.
In a Practical sense what this means is, if you have a method that is declared as public
you will be able to access that method across multiple different scripts that are part of the Same Assembly or Different Assemblies.
In case of Unity, as by default all your game scripts will be part of Assembly-CSharp.dll
you will basically be able access this variable or method that you have defined as public from anywhere in your code.
C# Internal Access Modifier | Unity Game Development Tutorial
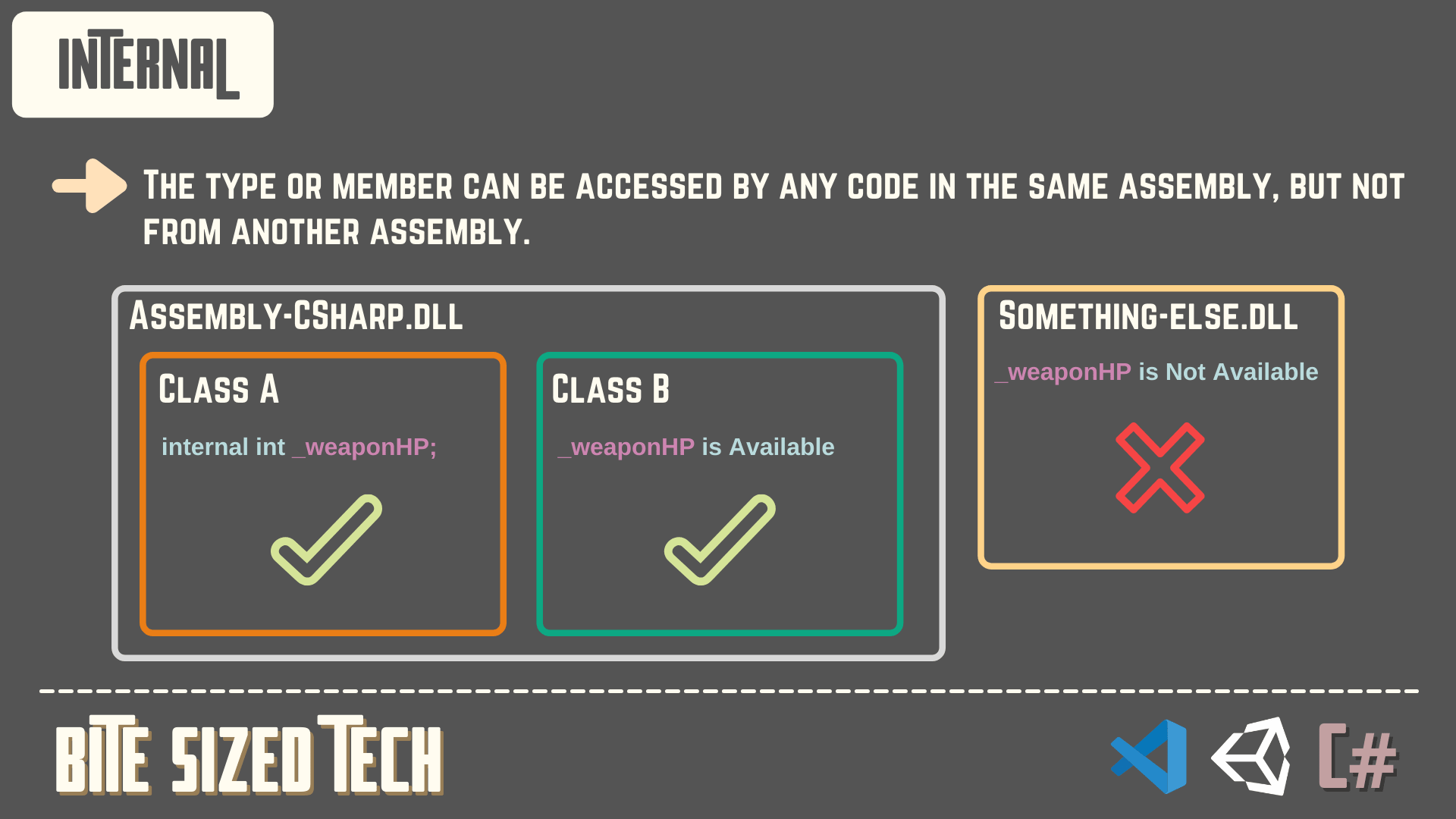
Next is the Internal Access Modifier,
which will allow the Type or Member to be accessed by any piece of code that is part of the same assembly
but will not allow the code from scripts that are part of a different assemblies to access this Type or Member.
If you are using the default settings in Unity,
then in practice you will see no difference between public and internal Access Modifiers as by default all you game scripts will be part of the same assembly.
C# Private Access Modifier | Unity Game Development Tutorial
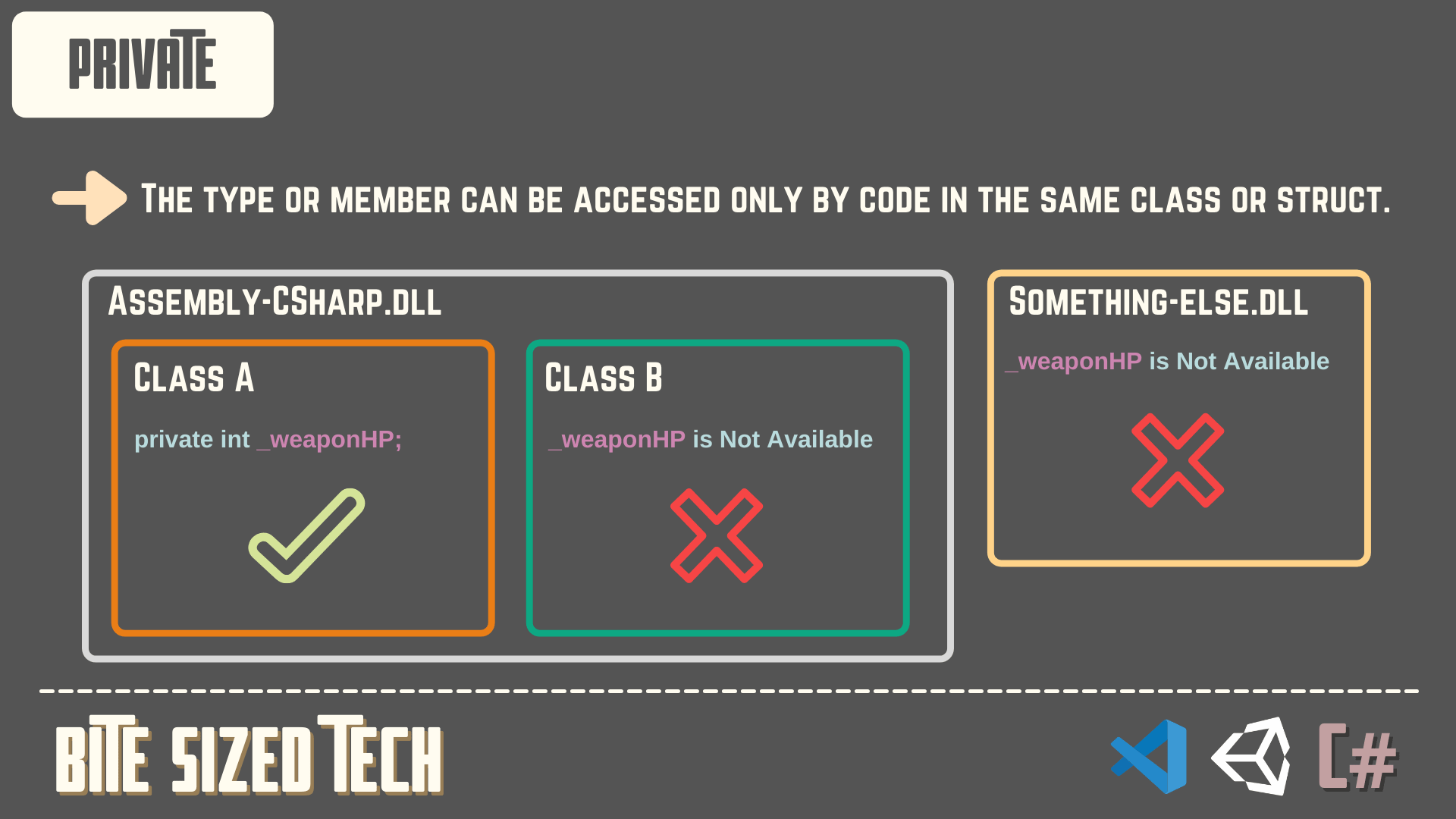
After that we have the Private Access Modifier,
If a Type or Member in your code is defined as Private then you will only be able to access this Type or Member from within the Same Class or Struct.
What this means is if you have declared a variable or method as private in let’s say Script A,
then you would not be able to access this variable or method in Script B directly.
Or if you have a Unity C# Script with multiple classes defined within it for some reason
Let’s Call the Script Weapon_Base,
then you will not be able to access a Type or member defined within the Script Weapon_Base -> Class Weapon_Base
inside Script Weapon_Base -> Class Weapon_Sword
even though they are within the same C# Script File.
C# Protected Access Modifier | Unity Game Development Tutorial
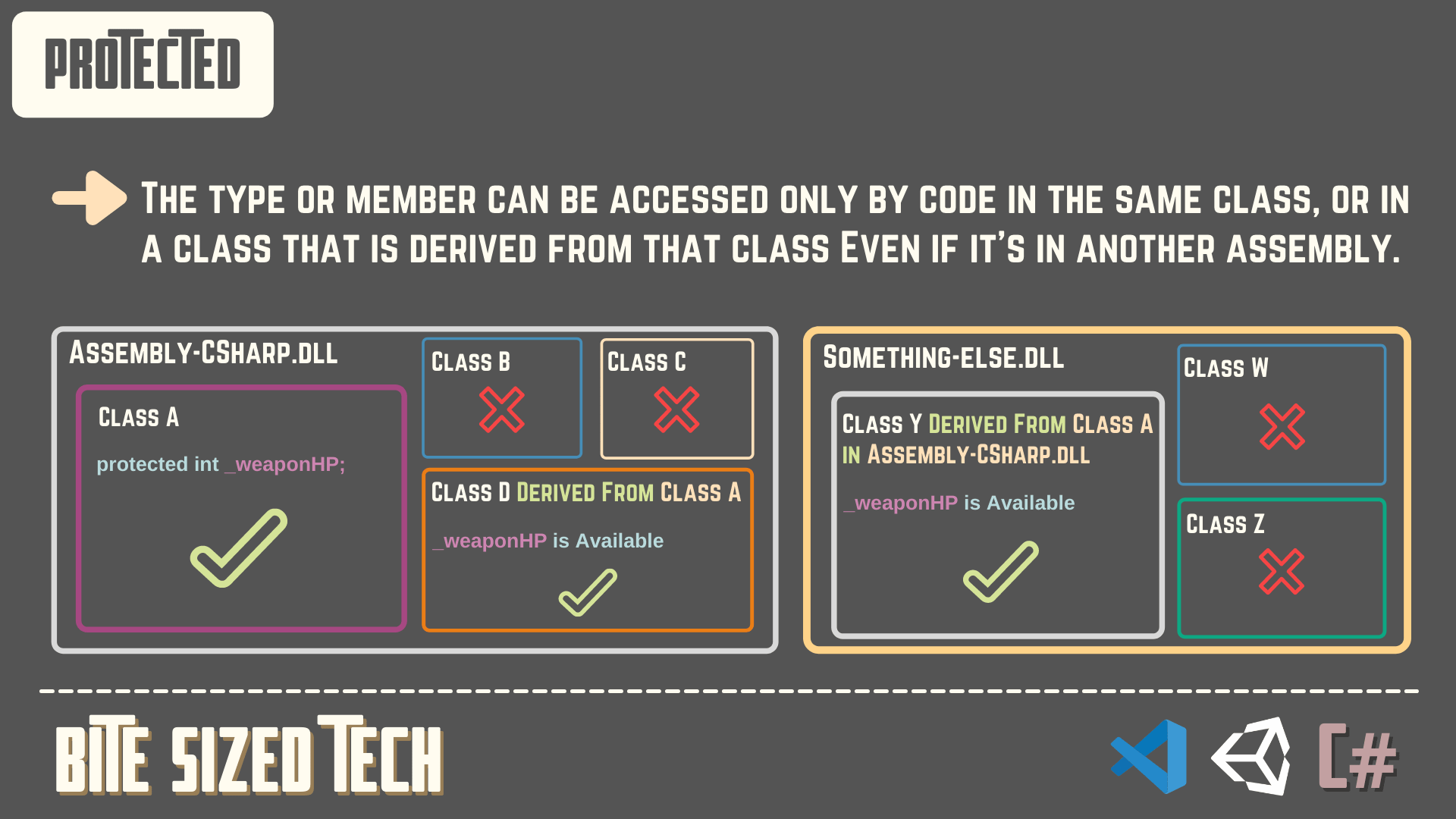
The 4th one is the Protected Access Modifier,
if a type or member is defined as protected then you will only be able to access them
within the Same Class or from within Classes Derived from this Class.
What this means is, suppose you have 3 Scripts -> ScriptA, ScriptB and ScriptC
and each of them have a Class X, Class Y and Class Z defined within them respectively
with Class Z in Script C actually being a Derived Class, with it’s Base Class being the Class X defined in Script A.
In this scenario, members defined as protected in Class X
will only be Accessible within Class X and Class Z
while these members would not be accessible within Class Y.
I have yet to cover the Concept of Inheritance in C#,
so some of you might be confused about Derived Classes
but for now don’t think about it too much as in the next article
I will be talking about inheritance and will explain everything related to it.
C# Protected Internal Access Modifier | Unity Game Development Tutorial
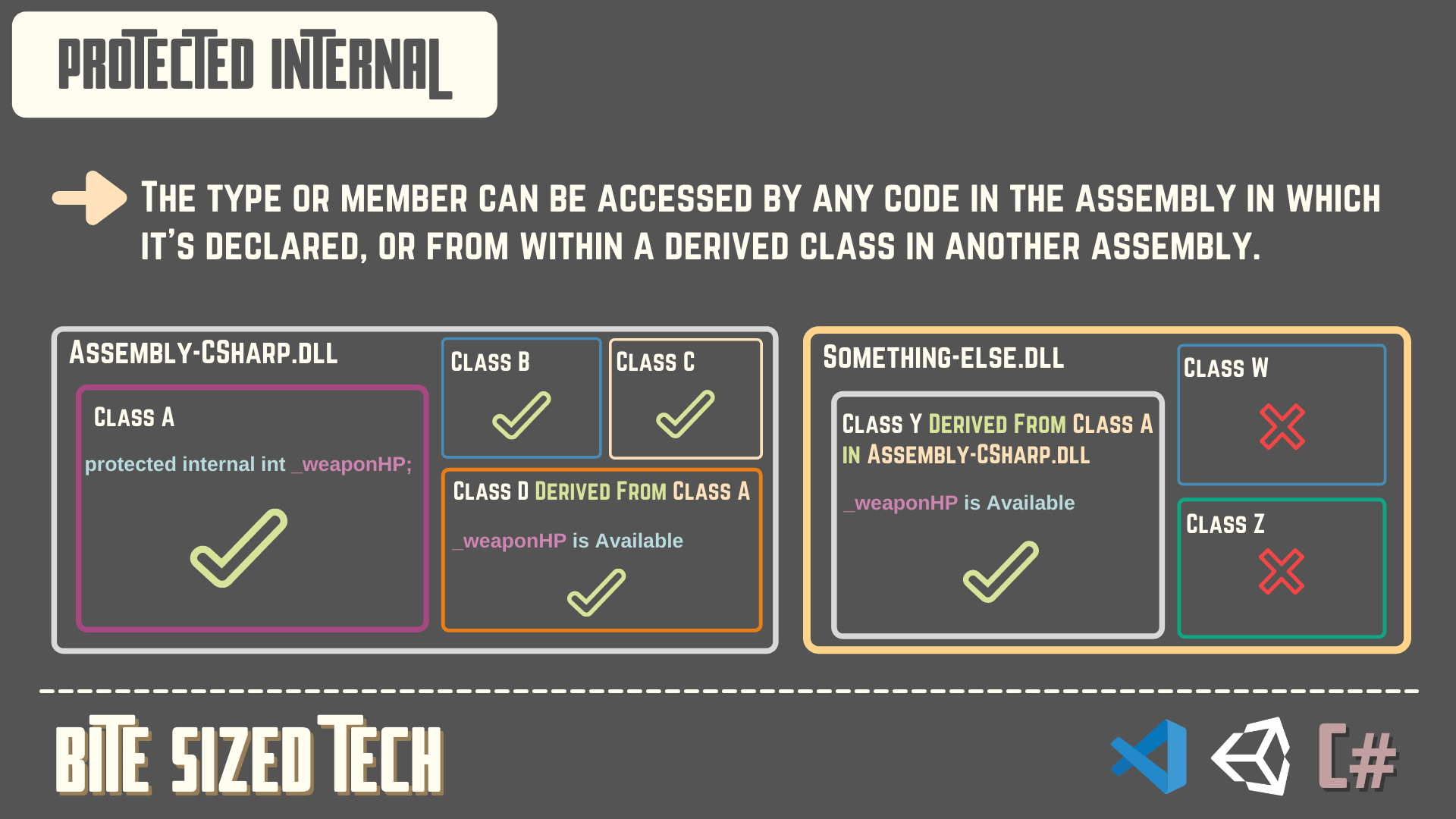
After that we have the Protected Internal Access Modifier.
Any type or member defined as protected internal will be accessible within the Same Assembly
while also being accessible within Derived Classes in other Assemblies.
C# Private Protected Access Modifier | Unity Game Development Tutorial
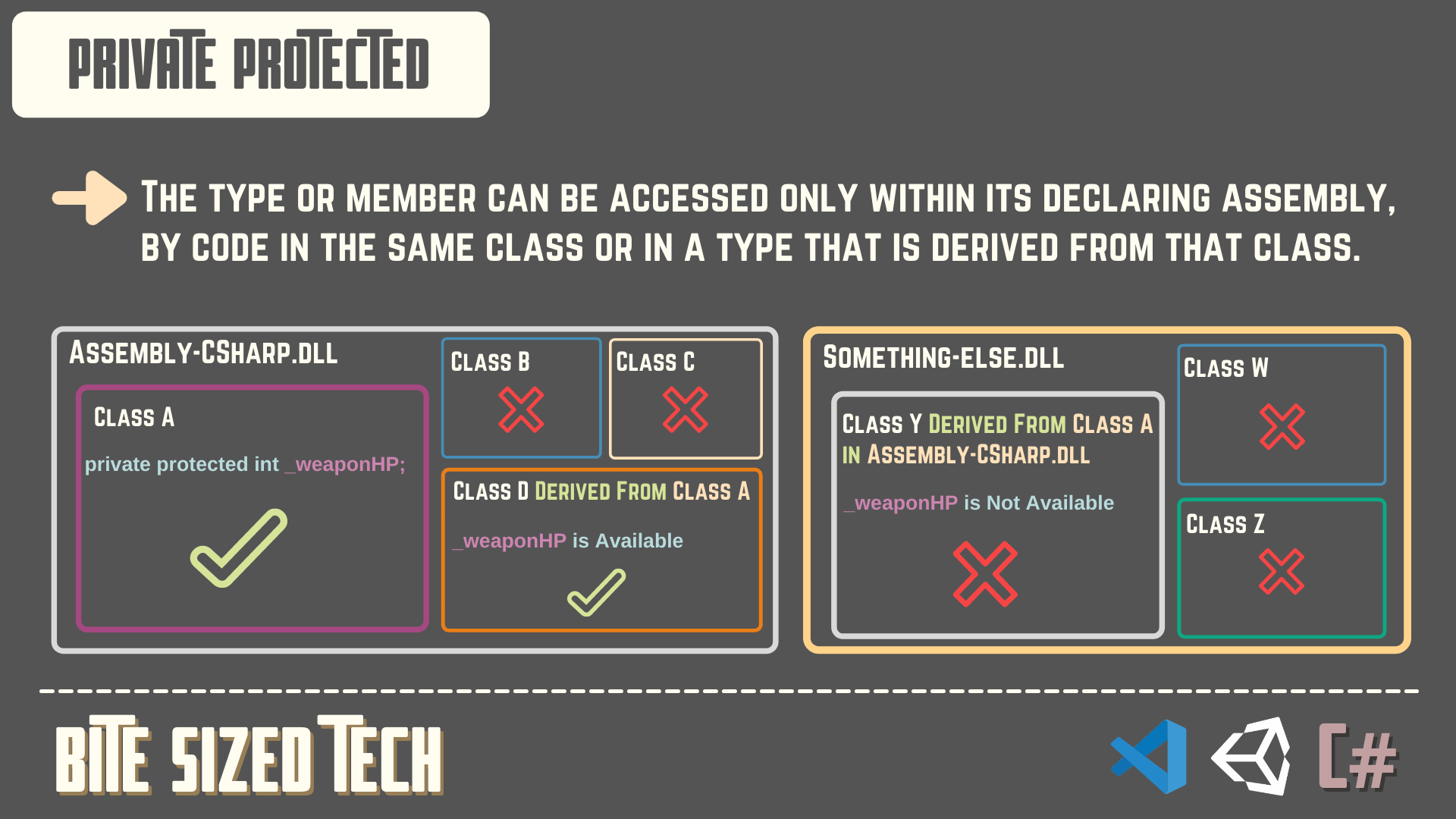
and the finally we have the last Access Modifier i.e. Private Protected,
Any type or member defined as Private Protected will be accessible
only within the code which is in the Same Class or a Derived Class in the same assembly.
These final two access modifiers i.e. Protected Internal and Private Protected
are in practice basically combination of two separate Access Modifiers,
which gives you a bit more control over the visibility of your type or member.
Some Useful Tips About Access Modifiers in C# | Unity Game Development Tutorial
Now with all that explained, when you are writing a script you should try making all the variables of the class private
as you don’t want to give direct access to these variables
to a piece of code which is not part of the class in which these variables are defined,
unless it is absolutely necessary.
But as you might still want to access these variables outside the class in which they were defined,
what you can do is define a Public Method or a Getter Setter Property
which will allow you to access the data stored within these private variables in a Structured and Controlled way.
I’ll link some resources such as the Unity Documentation and the MSDN page about Assemblies and Access Modifiers, after this section.
So that if you are so inclined, you can study it yourself.
but, although I’m sure it would be great if you understood the knowledge about Assemblies
and how you can use them to make a better game.
I suggest that you at least wait until you have a good enough understanding of the basics of programming
and then try and delve into the nitty gritties like this.
Links To Resources
- MSDN Page (Assembly)
- MSDN Page (Access Modifiers in C#)
- Unity Documentation (Assembly)
- MSDN Page (Getter Setter Properties)
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords