Inheritance is one of the fundamental concepts of OOPS (Object Oriented Programming System)
and one that we will talk about today.
In this article, we will go over everything about Inheritance and will cover,
- What is Inheritance in C# and how using it can help reduce the amount of code and increase code manageability when developing your games.
- After that, we will look at Inheritance practically by creating C# Scripts in Unity and coding them based on an RPG Analogy & the concept of Inheritance in C#.
- With that done, we will talk about Multiple Inheritance in C#
- and finally we will talk about Sealed Classes / Sealed Modifier in C# and how it can be used to stop a Type or Member in C# from being Inherited.
So, without further ado, let’s get started!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Designing a good game is no way an easy task and is one where as Game Developers you can easily lose focus of what is important for your game.
With a ton of ideas floating through your head when designing every little aspect of your game,
if you lose focus, it can lead to you committing to and putting your efforts on things that will not add a lot of value to your game.
So to help your in making sure you are putting your efforts in the right place, I’ve written this article on 3 Points To Focus On When Designing A Game
in which I’ve highlighted some points that will help you evaluate a game design idea
and will help you stay focused on the what is important for your particular game.
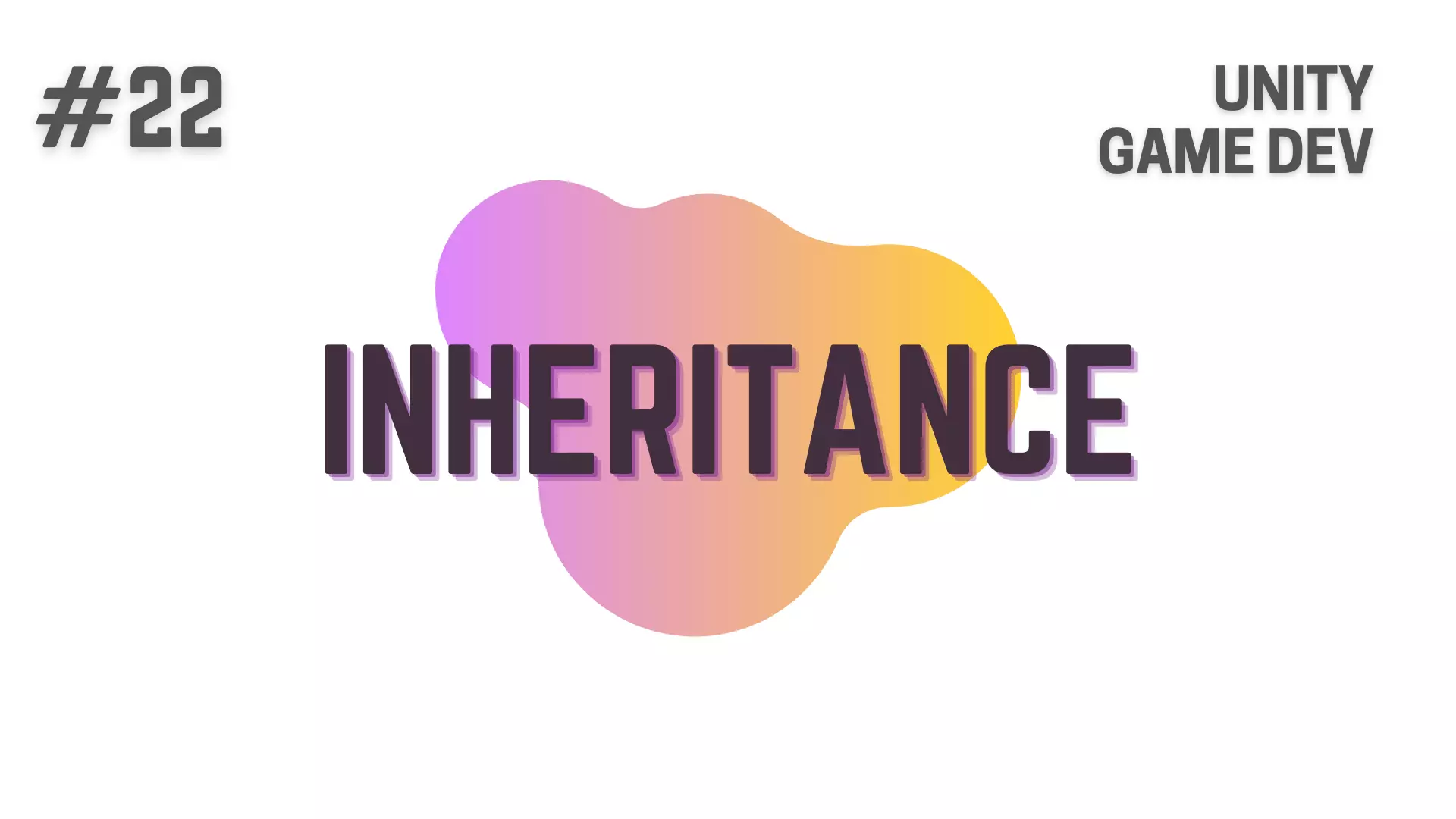
What is Inheritance in C#? | Unity Game Development Tutorial
In real world, children inherit a lot of stuff from their parents,
Just like that In C#, it is possible to inherit variables and methods from one class to another.
The concept goes like this, you have a Parent Class also know as a Base Class
which has Variables and Methods declared in it.
Then you have another class, that inherits these Variables and Methods from the Base Class.
This class is called a Child Class or a Derived Class.
To give more context, let’s let’s go to the RPG Analogy.
Let’s say that your RPG (Role Playing Game) has a lot of different types of weapons like
- Swords
- Spears
- Guns
- Axes
- Bows and Arrows
- etc
Now all these weapons will have stats like
- Piercing Damage
- Slashing Damage
- Bleeding Damage
- Weapon HP
- and what not
which all these weapons will have in common, just like in the article about Classes, Objects and Constructors
So in a scenario like this, if we were not using Inheritance, Interfaces or something like that
we would probably declare each of these variables in all the relevant scripts that you create.
Now the problem with this approach is that,
you will now have to maintain the same variables, with the same functionality,
in all the weapon scripts that you create,
which is not good, as this is highly prone to user errors
and will also increase the amount of code that you have to manage, exponentially.
Solving Redundancy & Increasing Code Manageability using Inheritance in C#
So to solve this problem we can use inheritance
what we will do is, we will create a Base Class named weapon_base
and then create a Derived Class for each weapon type, i.e
- weapon_sword
- weapon_spear
- weapon_gun
- etc
you get the point
What this will do is, it will create a central location i.e. weapon_base
where you will define the common Variables and Methods between all these weapons.
And then all the Derived Classes of this Base Class will be able to have these common Variables and Methods,
without having to define them in each of the Derived Classes.
So the only variables and methods that you will define in the Derived Classes themselves
will be the variables and methods that are specific to each of the Derived Class.
This will be really helpful as your game code grows in size
because you will only need to manage these common variables and methods in weapon_base.
and any changes that you make to them here, will directly reflect in all the Derived Classes.
To give even more context, look at the image below,
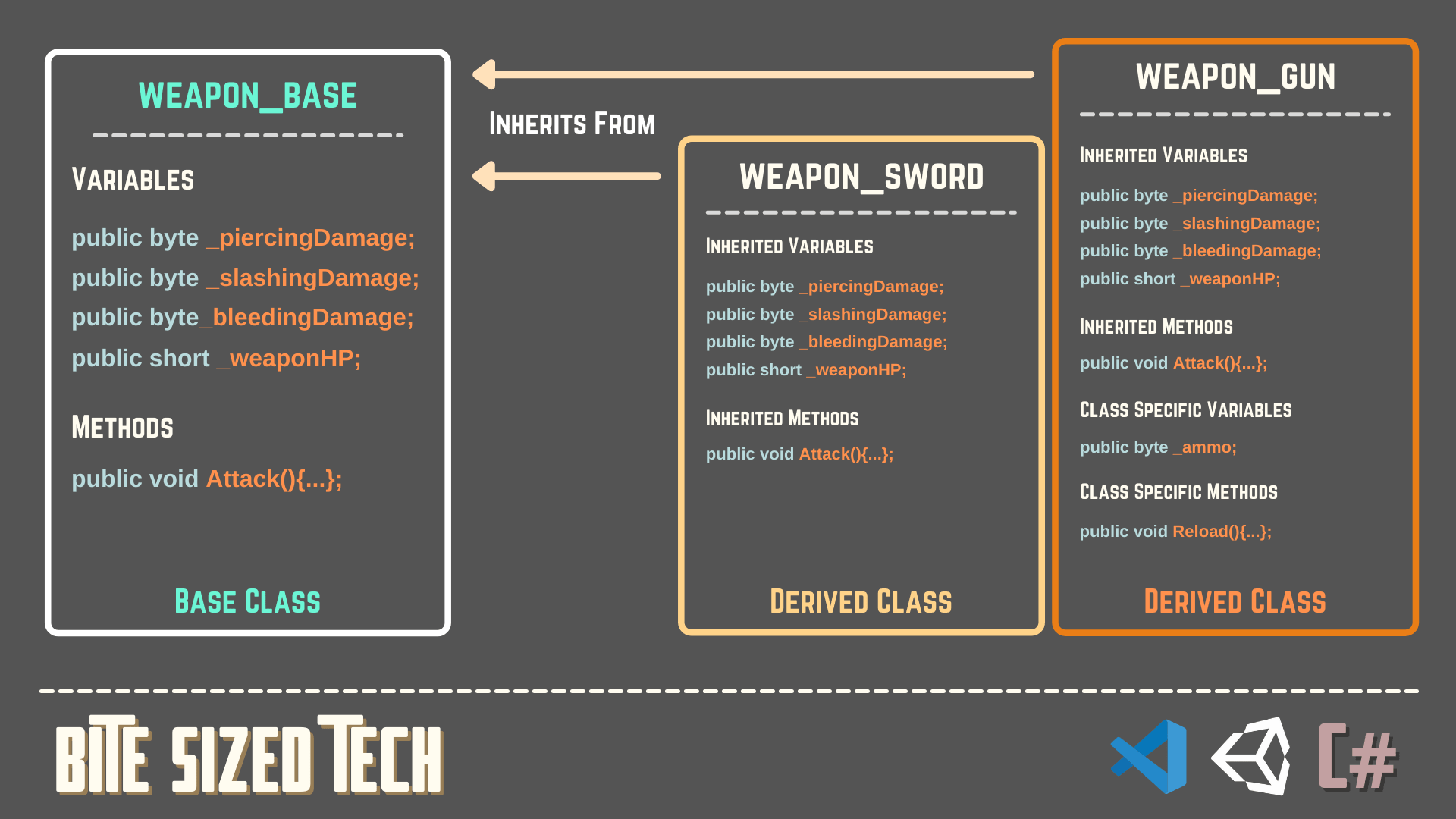
On the left side, you can see our Base Class weapon_base
and on it’s right side you have two Derived Classes, weapon_sword & weapon_gun
that inherits from the weapon_base Base Class
Now as you can see we have 4 Variables i.e. _piercingDamage, _slashingDamage, _bleedingDamage & _weaponHP and 1 Method i.e. Attack
defined and implemented in the Base Class weapon_base
which we think will be common in all the weapons.
and then through inheritance, we are bringing these variables and methods to the Derived Classes
without copying and pasting these same variables and methods in all of them.
Other than this, the Derived Classes also contain their own variables and methods, that are specific to their particular weapon.
i.e weapon_gun will have variables and methods like _ammo and Reload,
while weapon_sword won’t have these variables and methods.
Some of you might already be understanding, how this can be really helpful
when the classes and inheritance are structured and used correctly.
Implementing Inheritance in Unity | Unity Game Development Tutorial
So let’s go to Unity and in the Assets Directory create a Sub-Directory named Scripts
and then inside it let’s create our Base class named weapon_base
and also create the Derived Classes weapon_sword & weapon_gun, which will inherit from the Base Class weapon_base
With that done, now let’s open the weapon_base Class in VS Code and declare some variables and methods.
First of all, let’s declare the common stats for weapons using Variables
and then let’s declare a method named Attack and just log something inside it so that we can see it in the Unity Console.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_base : MonoBehaviour
{
public byte _piercingDamage;
public byte _slashingDamage;
public byte _bleedingDamage;
public short _weaponHP;
public void Attack()
{
Debug.Log("Attack Method Called!");
}
}
With that done, now let’s go to the weapon_sword C# Script
and modify it so that it will inherit from the weapon_base class
We can do this by using, colon ( : ) space and then the Name of the Base Class after the Name of the Current Class as shown below.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_sword : weapon_base
{
}
With that done, now let’s move to the weapon_gun C# Script and do the same
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_gun : weapon_base
{
}
Next we will declare Gun specific Variable and Method that is _ammo and Reload in the weapon_gun Class
and after that, in the Start Method let’s set values to all the Inherited and Specific Variables for weapon_gun Class, as shown below.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_gun : weapon_base
{
public byte _ammo;
void Start()
{
this._piercingDamage = 5;
this._slashingDamage = 0;
this._bleedingDamage = 5;
this._weaponHP = 160;
this._ammo = 6;
}
public void Reload()
{
Debug.Log("Gun Reloaded!");
}
}
With that done, let’s Debug.Log the values of these Variables so that we can see if they were set as we intended in the Unity Console.
Also, make a call to the Reload Method that we have declared in this Class
and the Attack Method that we have inherited from the weapon_base Class.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_gun : weapon_base
{
public byte _ammo;
void Start()
{
this._piercingDamage = 5;
this._slashingDamage = 0;
this._bleedingDamage = 5;
this._weaponHP = 160;
this._ammo = 6;
Debug.Log("Piercing Damage : " + this._piercingDamage + ", Slashing Damage : " + this._slashingDamage + ", Bleeding Damage : " + this._bleedingDamage + ", Weapon HP : " + this._weaponHP + ", Ammo : " + this._ammo);
Reload();
Attack();
}
public void Reload()
{
Debug.Log("Gun Reloaded!");
}
}
Now, let’s go back to the weapon_sword Class
and set values to the inherited variables & log them to the Unity Console as shown below
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_sword : weapon_base
{
void Start()
{
this._piercingDamage = 3;
this._slashingDamage = 5;
this._bleedingDamage = 3;
this._weaponHP = 200;
Debug.Log("Piercing Damage : " + this._piercingDamage + ", Slashing Damage : " + this._slashingDamage + ", Bleeding Damage : " + this._bleedingDamage + ", Weapon HP : " + this._weaponHP);
}
}
In the above code we have not set value for _ammo as it is a specific variable for weapon_gun Class
and we don’t have access to it in weapon_sword class.
With that done, let’s go to Unity and check the logs.
Don’t forget to attach the weapon_sword & weapon_gun C# Scripts to Game Objects in your Unity Scene.
Unity Log | Inheritance in C# | Unity Game Development Tutorial
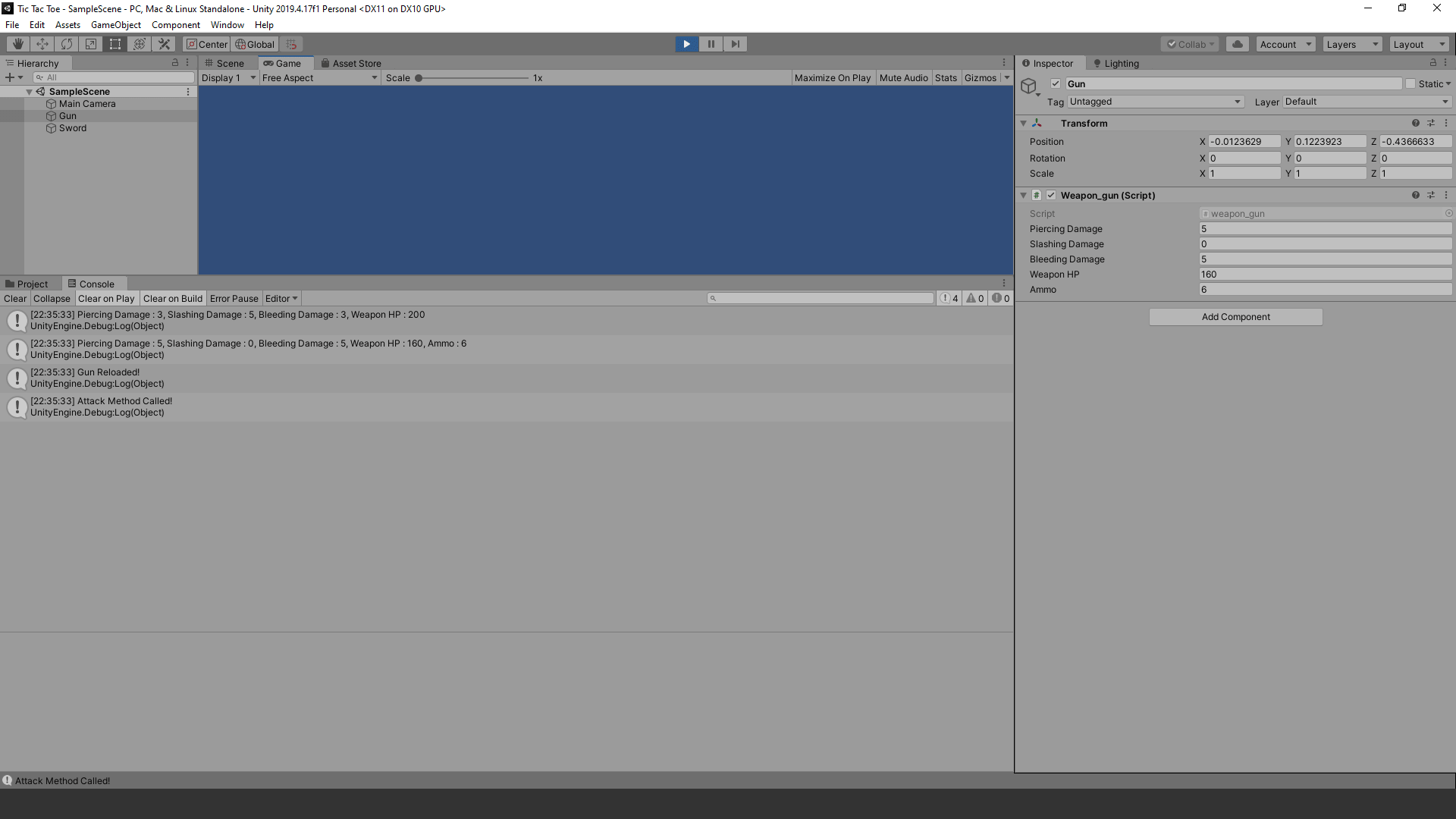
As you can see, in the First log being done from weapon_sword Class,
we are logging the values for all the Inherited Variables
In the Second Log which is being done from weapon_gun Class,
we are logging values for both the Inherited and Specific Variables
In the Third Log, we are calling the Gun Specific Method i.e Reload from weapon_gun Class
and in the Fourth Log we are calling the Inherited Attack Method from weapon_gun Class,
which we Inherited From weapon_base Class.
Inheritance Issues When Using Inappropriate Access Modifiers in C# | Unity Game Development Tutorial
Back to the code,
Now the thing to keep in mind is what we talked about in the article about Access Modifiers.
Right now, all the variables that we defined in weapon_base class are set to public
so if we change one of them to private like this
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_base : MonoBehaviour
{
private byte _piercingDamage;
public byte _slashingDamage;
public byte _bleedingDamage;
public short _weaponHP;
public void Attack()
{
Debug.Log("Attack Method Called!");
}
}
Then As you can see in the image below, we start getting Compile Tile Errors in the Derived Classes
where we are trying to access this variable saying that
“The variable is inaccessible due to it’s protection level.”
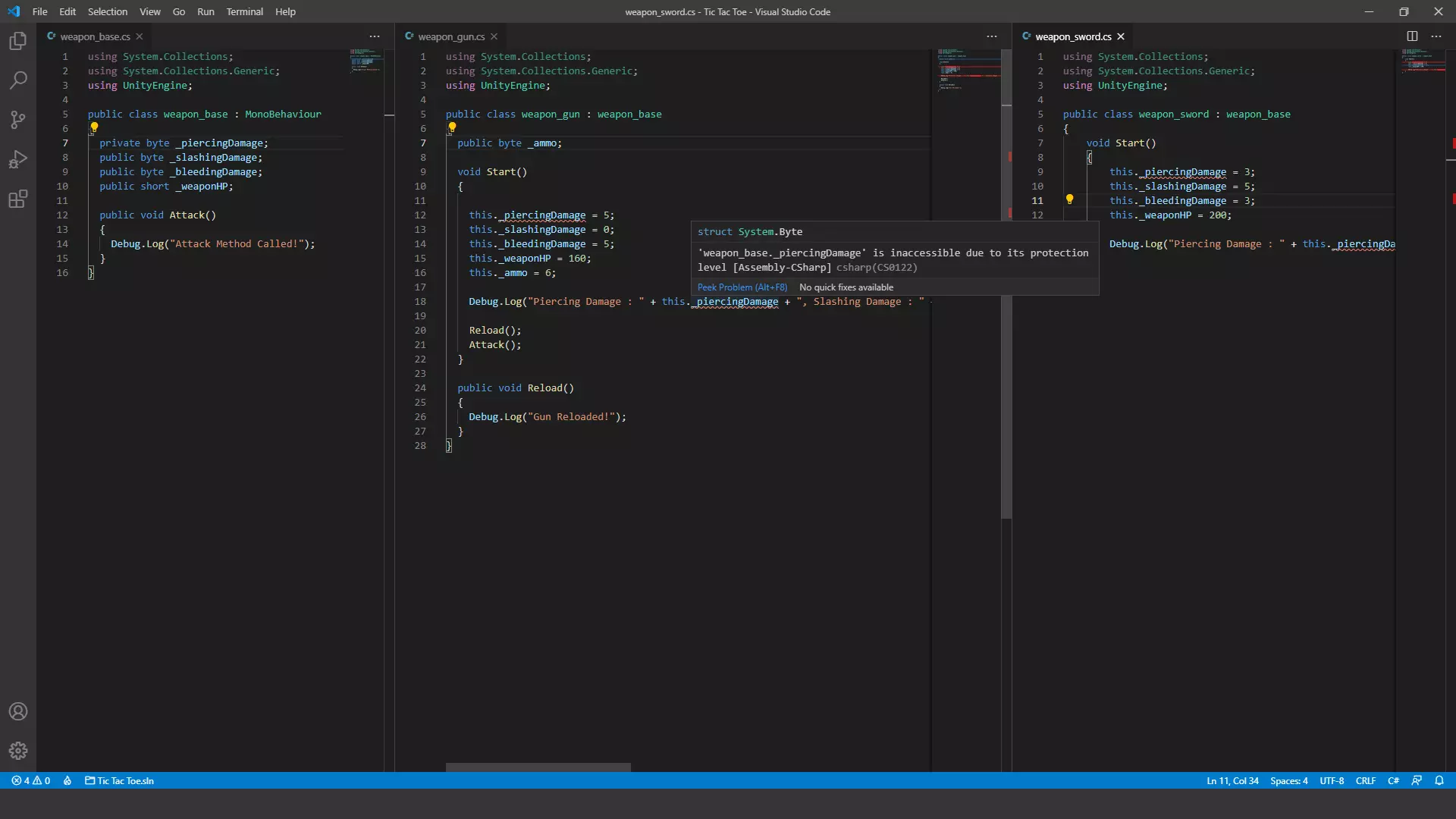
So just remember to use the correct Access Modifiers with the variables and methods that you define,
so that you have appropriate access to them.
Also as i said in the article about Access Modifiers, you should try keeping your variables private,
to do that in a situation like this, you can use Getter Setter Properties
So that you can still have access to these variables in a structured way, while still keeping them private.
So you should definitely look into that and understand how Getter Setter property works.
I’ll be going through that in one of the upcoming articles
so if you are interested, keep an eye out for that.
Multiple Inheritance in C# | Unity Game Development Tutorial
Next, some of you, who have worked with a language other than C#
might know of a concept in programming called Multiple Inheritance
which lets a Class inherit from more than one Base Class.
This is not supported in C# and if you do try to inherit from multiple base classes
than you will get an error stating that
“a class cannot have multiple base classes.”
as show in the image below
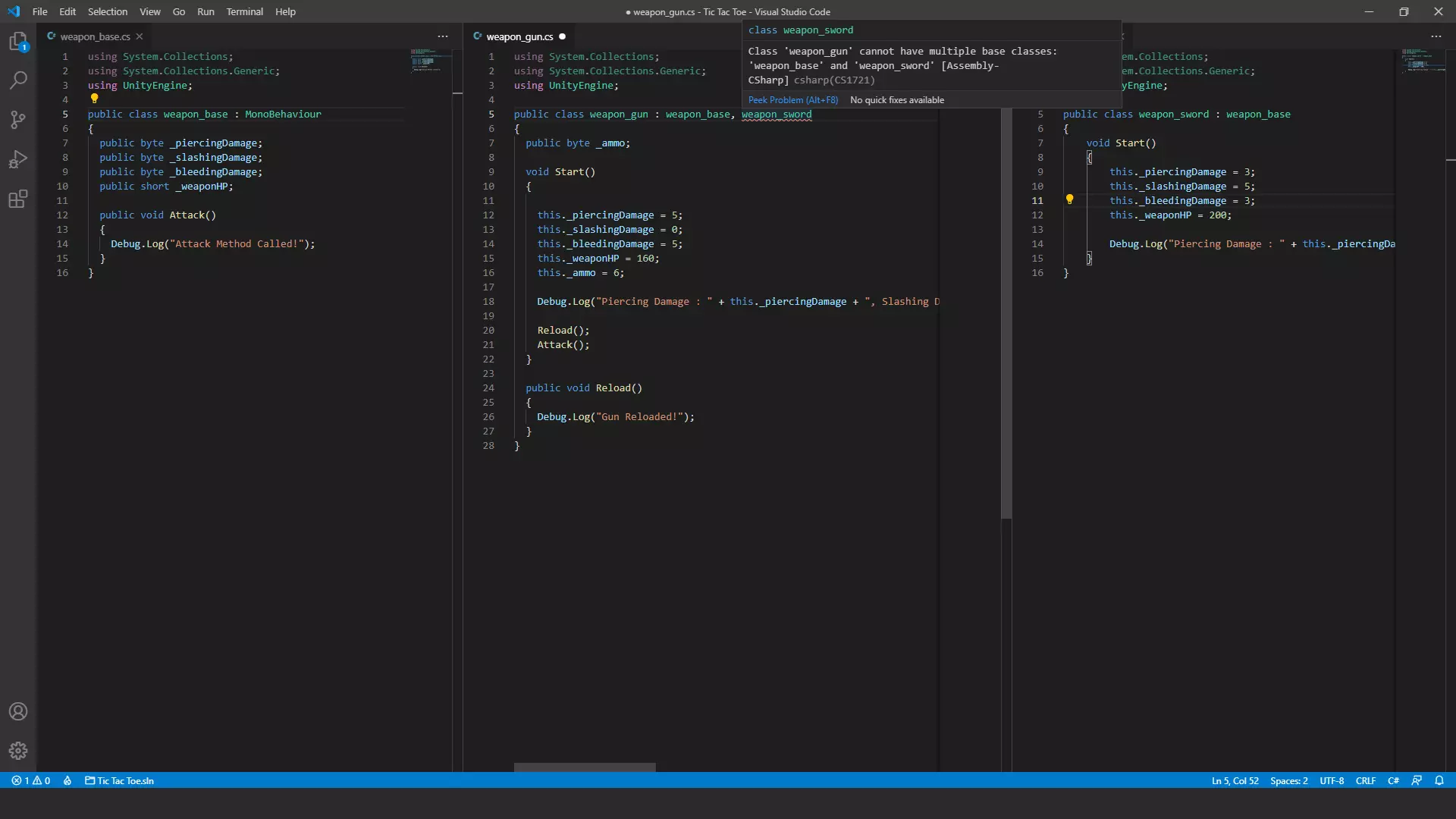
Sealed Class / Sealed Modifier in C# | Unity Game Development Tutorial
With that done, let’s talk about, how you can stop a class from being inherited.
To do this, you can use the sealed modifier before the class definition
so let’s make weapon_base a sealed class as shown below
public sealed class weapon_base : MonoBehaviour
{
//...
}
Doing this will stop the weapon_base class from being inherited
which also means that, we will start getting errors in the weapon_sword and weapon_gun Classes
because they were inheriting from weapon_base Class as shown in the image below.
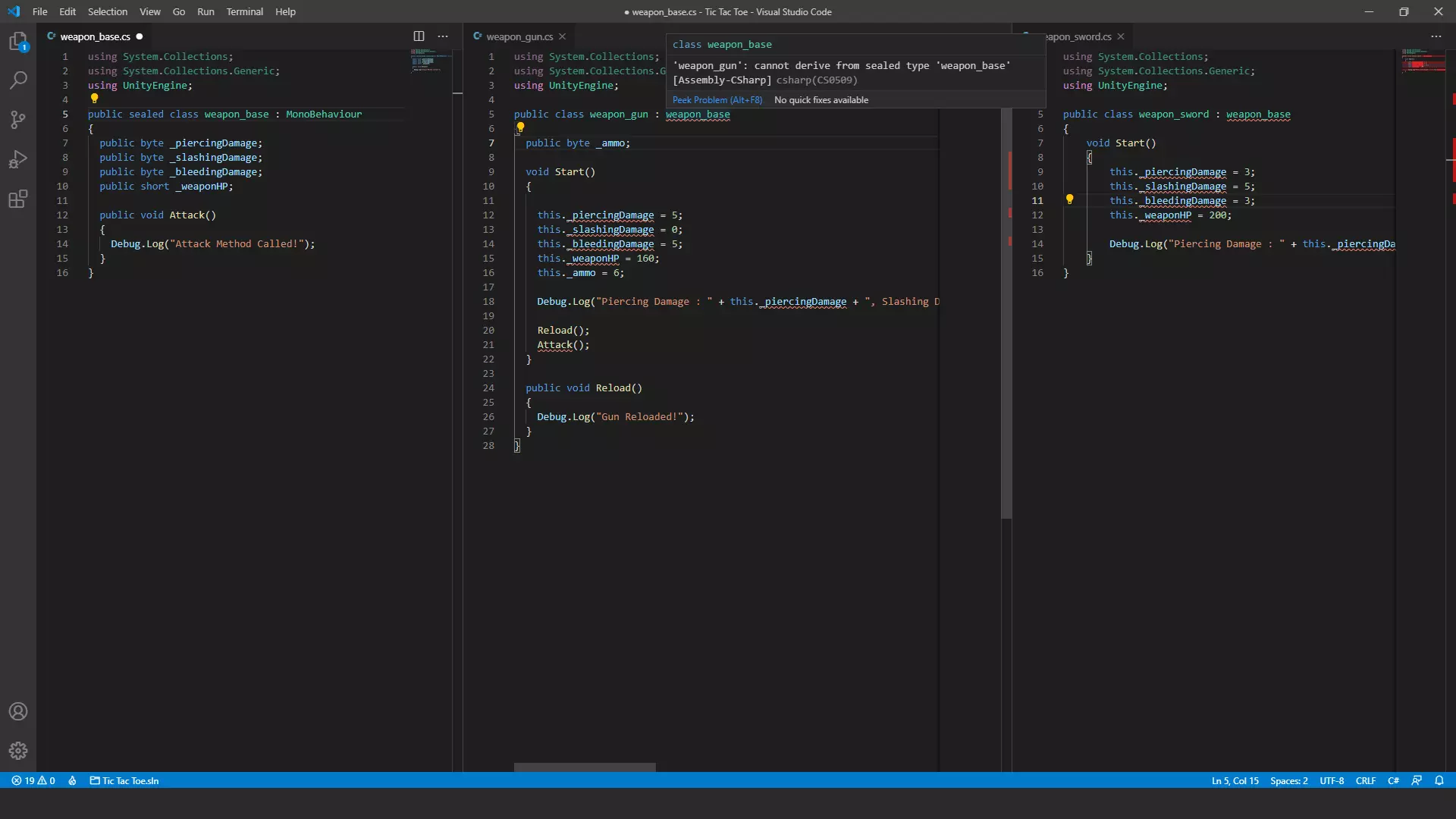
This means that a class with the sealed modifier can never be used as a Base Class.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords