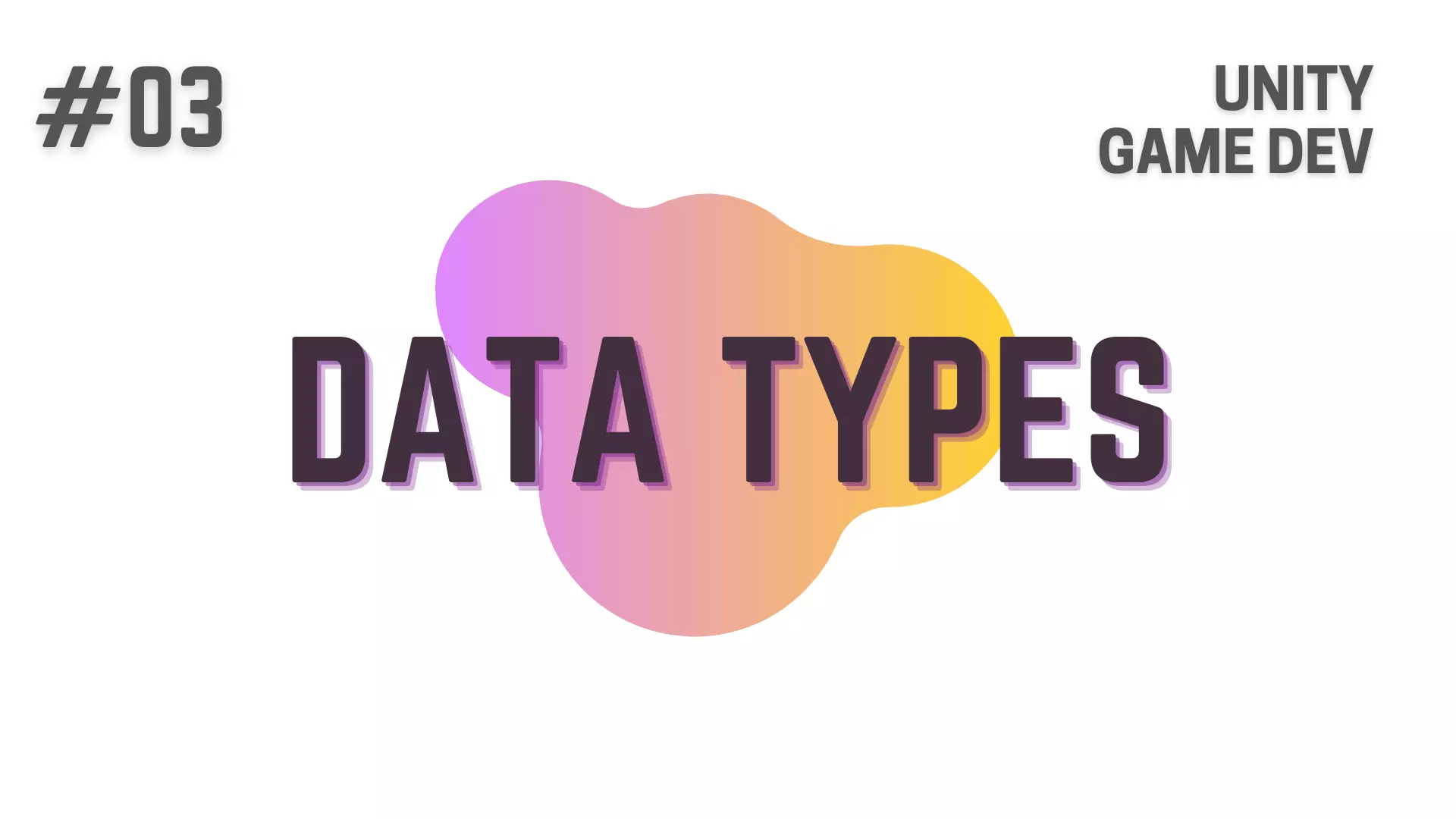
In this article, I’ll be talking about Variables and Data Types in C# Programming Language that you will be using while developing games using the Unity Game Engine.
So, let’s directly get to the point.
Breaking Down The Base Structure Of A Unity C# Script
To explain everything simply and break down the base structure of a Unity C# Script, without going into details about Classes, Methods or Namespaces.
Let’s look at this piece of code.
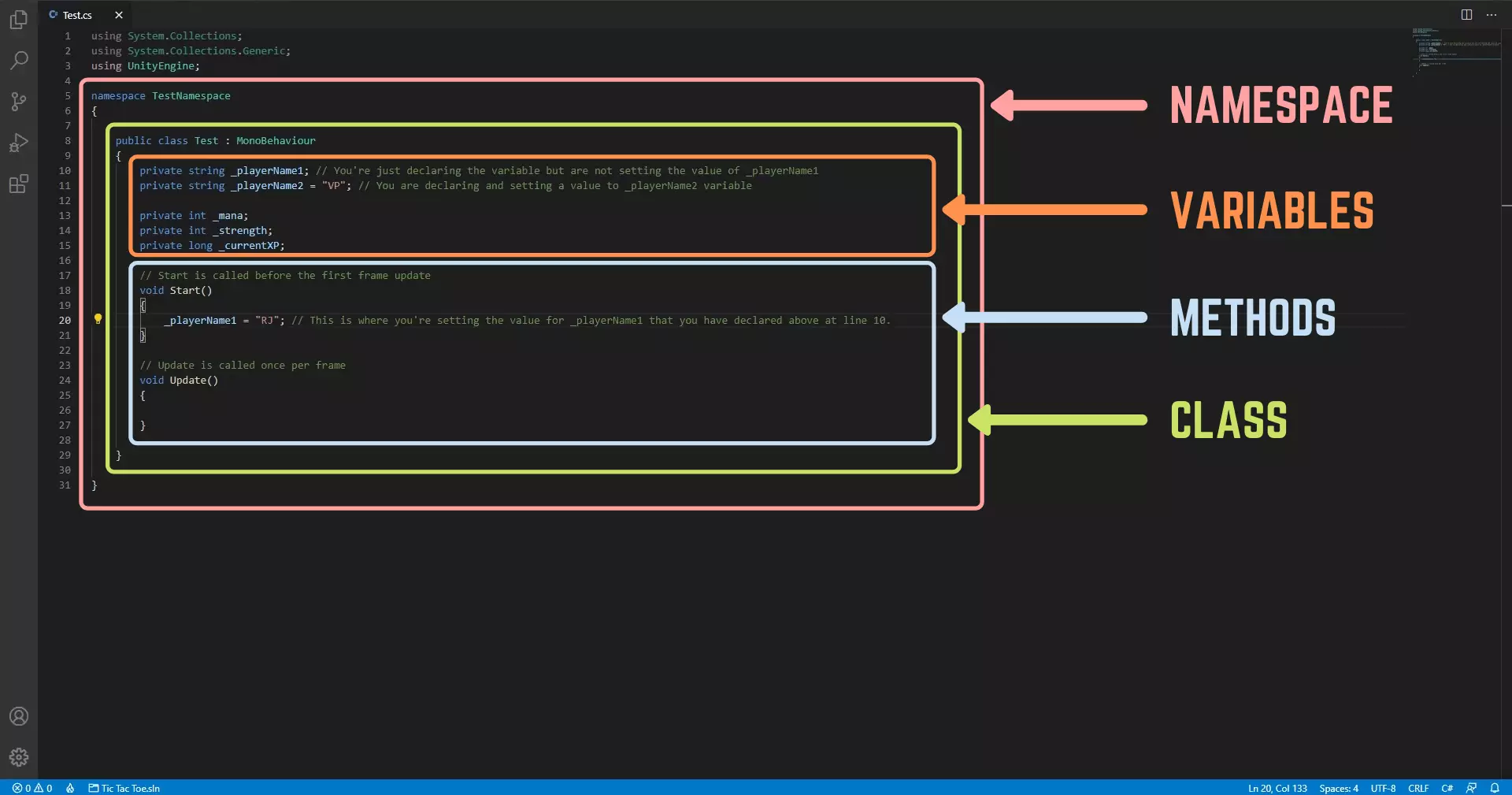
Here, the Largest part, that is bordered Pink is the Namespace.
The name of the namespace is TestNamespace and everything between the first and last curly braces in the Pink bordered box is considered as the part of the TestNamespace namespace.
Now inside TestNamespace, we have the Class called Test and inside this class we have the Variables and Methods declared which are bordered in Orange and Blue respectively.
Don’t worry about what a Namespace, Class or a Method is right now as we will have dedicated Articles and Video Tutorials on each of them later.
what is a variable in programming
So, finally having dug deep enough into the base structure of a Unity C# Script, we have reached the topic of today’s article, that is Variables.
You can consider variables as Data Containers that will be used throughout your program to contain data. In your case the program refers to the Game you are making or a particular C# Script in the Unity Project of your Game.
Explaining Use Of Variables With A RPG Analogy
To give a real world context, suppose you’re making a RPG (Role Playing Game).
Now in this RPG, your Player Character will have Stats, like Name, Strength, Endurance, Mana, Charm etc that will be used by you to display and calculate various things in your game.
Your game might also have various sort of items and weapons that will all have their own stats and buffs.
All these different stats need to be stored somewhere when your game is running and that place is a variable.
This Post is Part of : How To Make A Game Series where i’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
If you have not read the first article in which i introduce the Art of Game Development, Roles in Game Development and your Recipe for Success as a Game Developer to you, you can click here to read that article first as i can’t emphasize how necessary it is and how helpful it will be for you to have the right mindset required to learn how to make a game and become an exceptional Game Developer.
How To Declare A Variable & Assign a Value to it
Now coming towards the coding part, let me show you the syntax that will be used to Declare a variable and Assign a value to it.
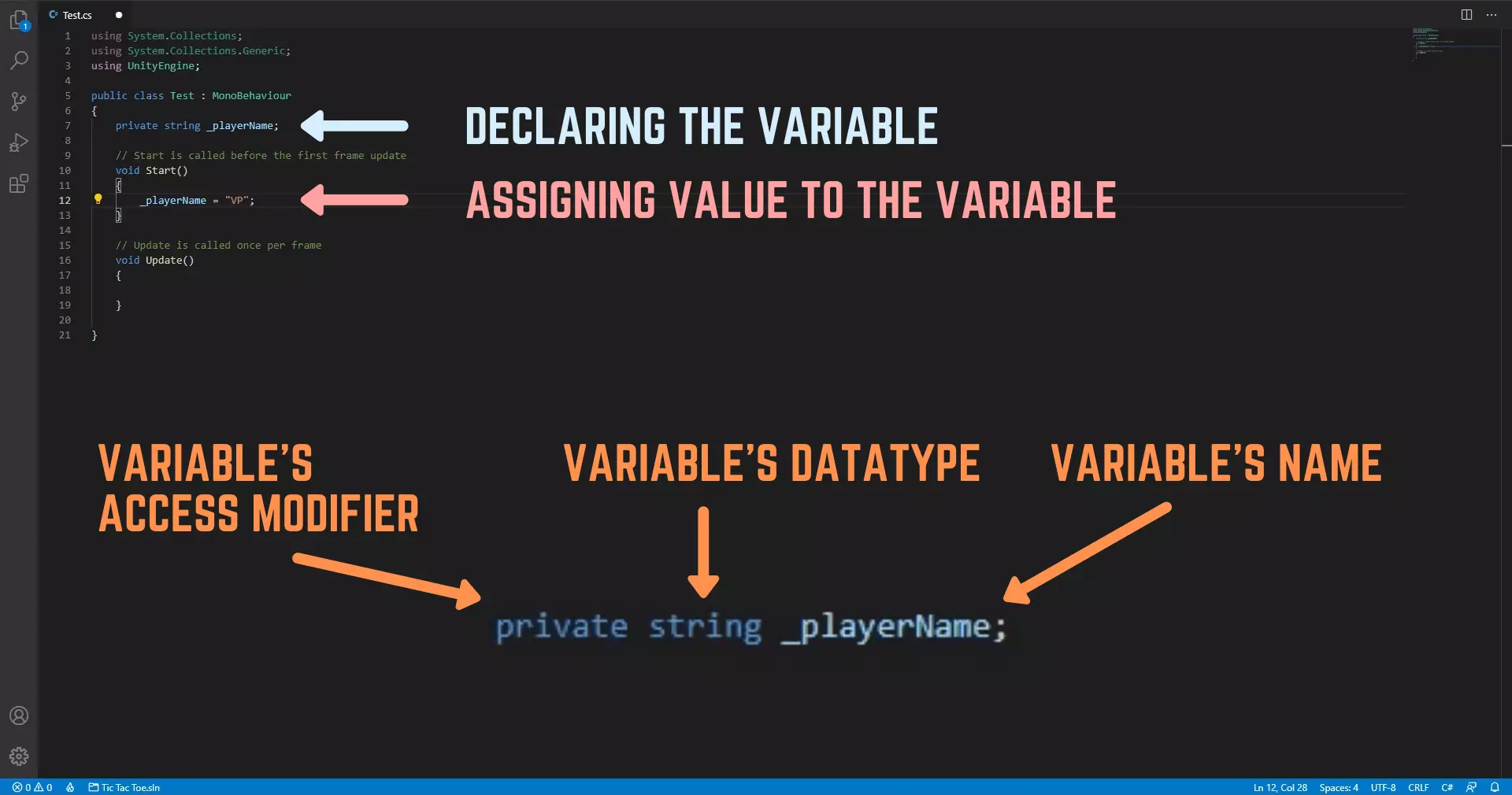
If you look at the image above, you can see that the syntax for Declaring a variable can be divided into 3 different parts
- Access Modifier
- Data Type
- Variable Name
#1 – Access Modifier
Access Modifier will decide the visibility of your variable to other parts of your program. That is, it will decide from where you will be able to access and modify the declared variable.
Defining the access modifier is considered as being optional but if you ask my opinion do it every single time as it will make your code much more readable.
If you don’t define an access modifier, then the variable if it is a class member i.e. if it is part of a class, will be private by default.
Now again don’t worry about how Access Modifiers actually works, we will have dedicated Articles & Videos Tutorials about that later.
In this Articles, just pay attention to what a variable is and how you can use variables when you are making a game with C# and the Unity Game Engine.
#2 – Data Type
Next comes the Data Type of your variable, which will decide what kind of data you can put inside the variable.
If you try to put data inside your variable that is not compatible with the Defined Data Type of your variable, then it will result into you getting an error.
For example, if your try putting Text into a variable that is defined to contain only Whole Numbers then you will get a compile time error.
We will talk more about the various Data Types in C# below after we talk about the third and the final point.
#3 – Variable Name
And Finally, we have the Variable Name.
This is the name using which we will be accessing this variable when we want to get the data stored inside this variable or when we want to set or modify the data stored inside this variable.
For Example, if we want to get the Stats of the Player Character like Strength, Mana, Charm, etc when the player opens his Stats Window then in the code inside the C# Script we will use the Variable Name to get the data stored inside this variable.
Now, the final point before moving forward is that, just like when you use a full stop ( . ) to end a sentence in English, whenever you write a statement in C#, like when you declare a method, set a value to a variable, or basically do anything in your code, you have to end your line with a Semi-Colon ( ; ) as you can see in the image above.
Data Types in C# Programming Language
Now, lets look at the different Data Types available to you when you are coding in C#.
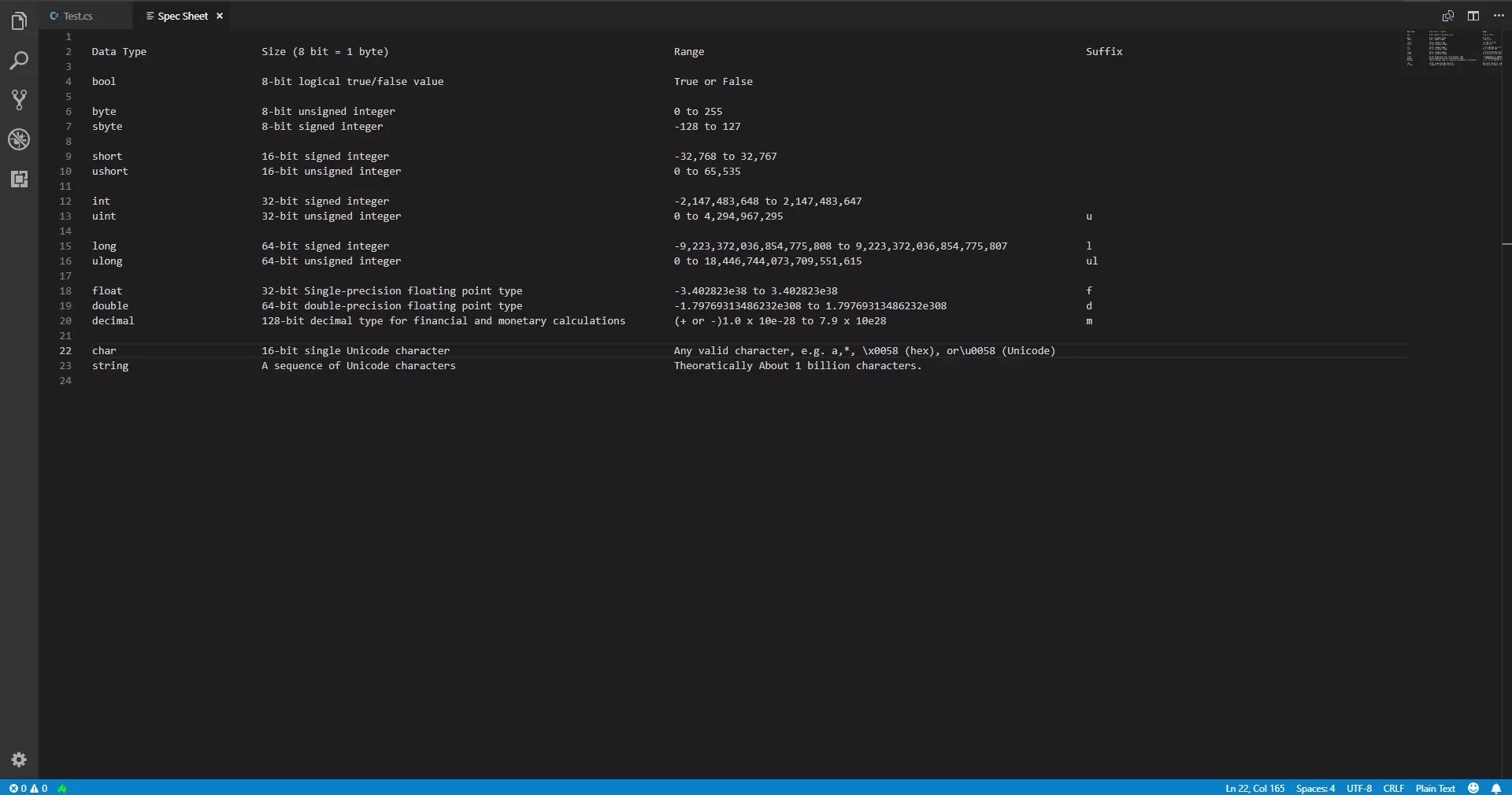
#1 – Boolean Data Type
A Boolean can store true or false values. You can use this to store data that can only have two sides.
To give more context, lets go back to the RPG analogy.
Think about a town in this game, with houses that the player can go in an out of during a certain period of time, lets say from 9 am to 5 pm.
So, to get this scenario working in your game, you will need the houses with doors that open and close at correct time.
Now, a Door can either be Open or it can be Closed which is perfect for us to use a Boolean.
So first of all you will declare a Boolean called _isDoorOpen in your script like
private bool _isDoorOpen;
After that what you can do is, in the script which manages game world time and day night cycle, when the time hits 9 am you open the house doors by setting _isDoorOpen to true like
_isDoorOpen = true;
and at 5pm you can close the house doors by setting _isDoorOpen to false like
_isDoorOpen = false;
But just setting the boolean values will do nothing as you still need to code the game logic of what will happen when _isDoorOpen is set to true or false.
So, in the script that allows the player to move in and out of the house, you can use the _isDoorOpen boolean to create a condition which will only allow the player to enter the house if _isDoorOpen is true and will stop the player from entering if _isDoorOpen is false.
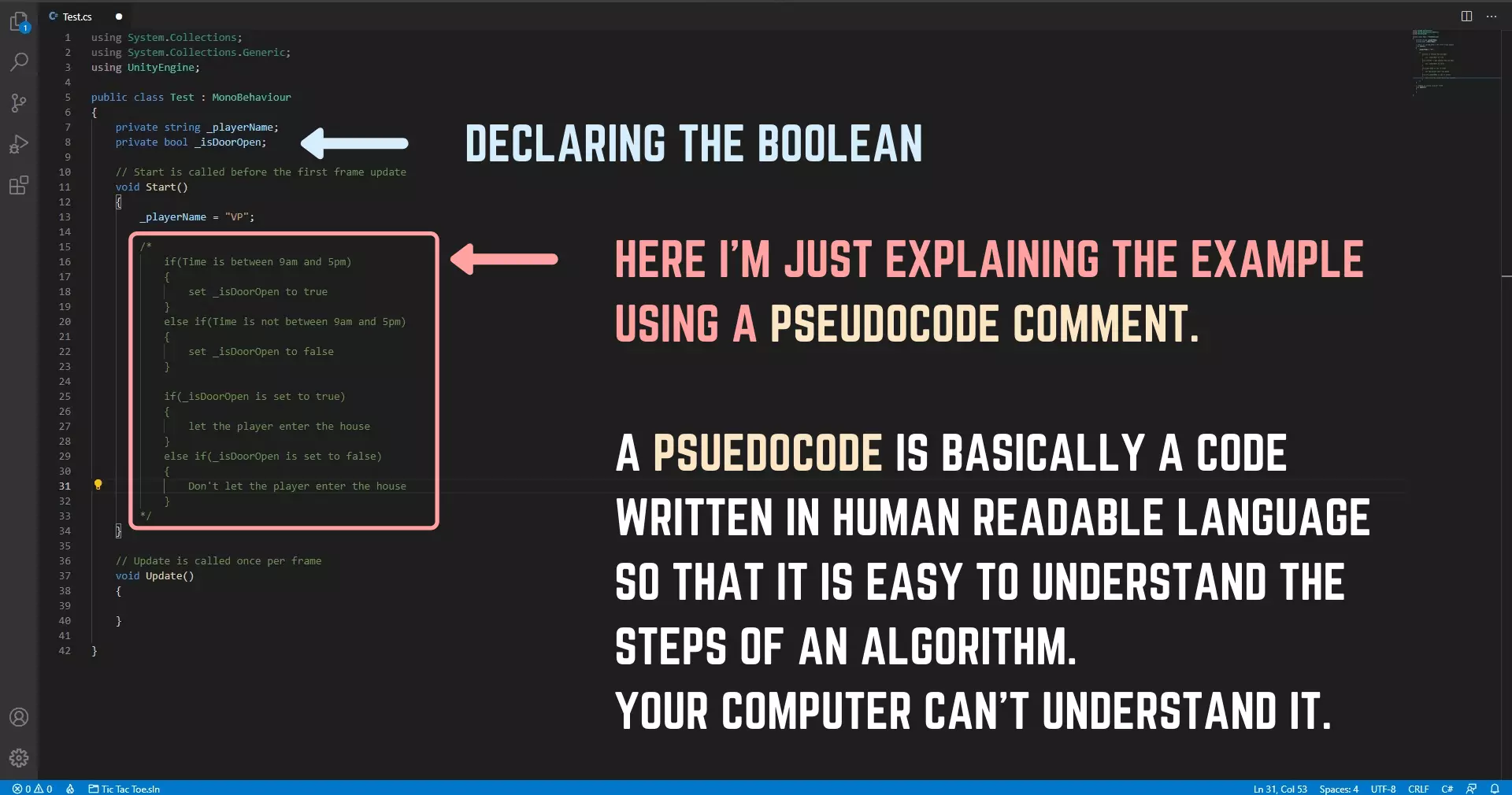
There are a lot of ways to make this into a much more flexible system, but this is just a basic example to explain in what sort of situation you would use Booleans.
#2 – Whole Number Data Types – Byte, Short, Int, Long
Moving Forward, let’s talk about the four Whole Number data types that is Byte, Short, Int and Long.
These four data types can be used to store whole numbers with varying amount of ranges with
- byte : 0 to 255
- short : -32768 to 32767
- int : -2,147,483,648 to 2,147,483,647
- long : -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
You can find all this information in the Spec Sheet image at the start of this section.
In the spec sheet you can also see that each of these data types will take varying amount of space in memory with
- byte : 1 Byte (8 Bits)
- short : 2 Bytes (16 Bits)
- int : 4 Bytes (32 Bits)
- long : 8 Bytes (64 Bits)
You have to make sure that the Data Type you are using for the variable has sufficient range for the purpose it was made for.
Again going back to the RPG analogy, we talked about you having to store attribute data for your player character like Strength, Mana, Endurance, Charm, etc.
Now we can use all these Data Types to store something like that by doing
private byte _strength = 5;
private short _endurance = 3;
private int _mana = 3;
private long _playerMoney = 3474674747738L; // L Suffixed
But, if you know that your Character’s Strength, Endurance, Mana, etc are always going to be Positive Values and let’s say are never going to be above 25 then it would be Waste of Memory to use anything other than Byte data type to store this sort of data.
My reason for this lengthy explanation is that it is really easy to default to Int data type for storing any whole numbers and to be completely honest i do that a lot.
Making a habit of only using what you need from the beginning, might save you some trouble down the line and will also make you a better Game Programmer.
Moving forward, as you might have noticed, we don’t use any suffix when setting a value for Byte, Short or Int data types but when you are setting a value for Long data type you have to suffix the value with the letter L.
#3 Whole Number Data Types – Sbyte, Ushort, Uint, Ulong
There is another concept that needs to be covered before moving to Fractional data typesand that is the Concept of Signed and Unsigned when it comes to these four Whole Number Data Types.
So, when you are creating a variable using Short, Int or Long Keyword, what you would be creating will be a Signed Variant of Short, Int, or Long as the ranges of these data types covers both negative numbers and positive numbers.
If you know that the variable that you are creating will only contain positive values, then you can declare this variable using the Unsigned Variants.
The ranges of the Unsigned Variants are as follows
- ushort : 0 to 65,535
- uint : 0 to 4,294,967,295
- ulong : 0 to 18,446,744,073,709,551,615
and you can declare them like this
private ushort _weaponWeight = 25454;
private uint _playerMoney = 536344U; // U Suffixed
private ulong _maxXP = 79697621896798UL; // UL Suffixed
In case of byte it is opposite as by default byte is unsigned.
The range of Signed Variant of byte
- sbyte : -128 to 127
and you can declare a Signed Variant of byte i.e. sbyte like this
private sbyte _charm = 5; // Signed Variant of Byte as Byte inherently is unsigned
Another point that you might have noticed is that the uint values need to be suffixed with the letter U, while ulong values need to be suffixed with UL.
#4 Fractional Data Types – Float, Double, Decimal
Next, we have the Fractional data types, that is Float, Double and Decimal which can contain Numbers with a floating point.
All these 3 data types will store fractional values in the ranges as stated in the Spec Sheet image above. Although they might seem really similar, there is a difference between them that you need to know of.
float and double data types both are not “decimally accurate” because they stores values as a Binary Floating Point Type.
While decimal on the other hand is “decimally accurate” and has much higher precision because it stores the values as a Decimal Floating Point Type.
For this reason, though you will take a performance hit when using decimal data type, you definitely want to use it when doing critical tasks where precision is absolutely necessary like when doing financial calculation.
You can do more research on that yourself but as you’re reading this article on game development, i will take it as you are developing a game and for that purpose you don’t need such high precision and using float or double is just fine.
Now going to the code again, you can declare these Fractional Data Types like this
private flaot _floatExample = 23.45f; // F Suffixed
private double _doubleExample = 3434345345.2323d; // D Suffixed
private decimal _decimalExample = 3.7527365236273623776m; // M Suffixed
# 5 -Text Based Data Types – Char, String
Moving forward, let’s talk about the data types that you would be using to store Text based data, that is Char and String.
Char data type can be used to store a single character whether it be in form of
- Unicode – eg. \u0058
- Hex – eg. \x0058
- Just a character – eg. a, *
String can be thought of as a sequence of characters and can be used to store any length of text with the theoretical upper limit of 2GB per string or about 1 billion characters.
Going to the code you can declare and initialize char using single quotes like this
private char _charExample = 'a';
and String can be declared and initialized using double quotes like this
private string _stringExample = "This is your host VP.";
Going to the RPG analogy again, you will be using Strings a lot for displaying Dialogues, using it in your Game Menus and your HUD.
Basically any text you see in your game will be using string in one form of another.
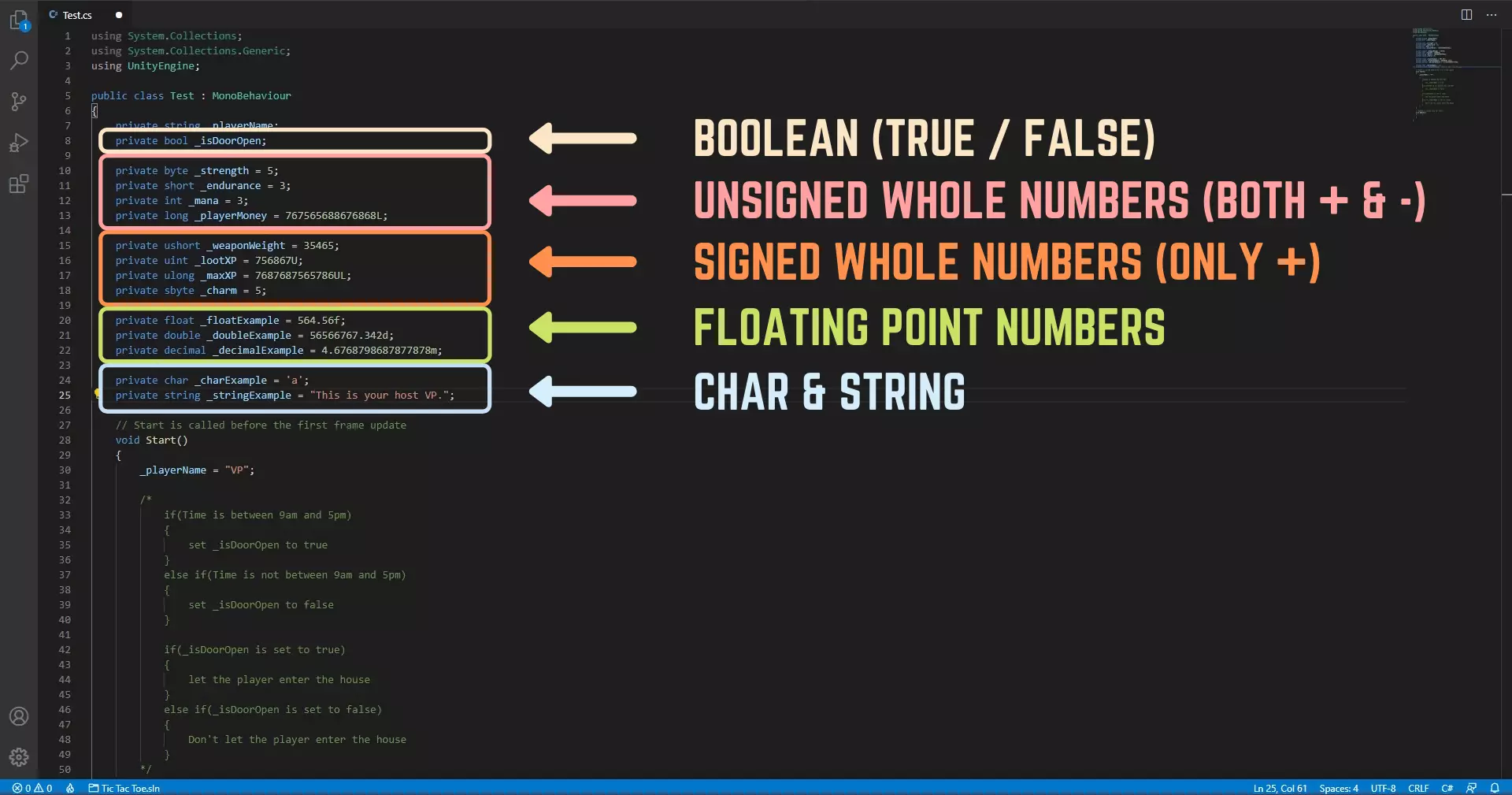
Now, that does it for all the C# Language Specific Data Types that you needed to know to get started in Game Development but that does not mean you know all the Data Types you will be using when developing your own games.
When you start working with Unity you will encounter various Unity Specific Data Types that you will need to work with but you don’t need to start panicking as with these C# Data Types as the base you will be able to understand how they work pretty easily.
If you think you will have difficulty understanding the Unity Specific Data Types and how they work you can continue following this article series as I’ll be explaining these Data Types as we keep encountering them.
Conclusion
Well folks, i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords