When you are coding a Game there will come times when you would want to repeat the same piece of code again and again
like when showing Items on your Menu or spawning the Player and NPCs in your Game World.
Loops in programming languages like C# can be used to do just that.
Now, there are various kinds of loops in C# like For Loop, Foreach Loop, While Loop and Do While Loop
in this article, we will take a closer look at For Loops and understand all the key details about it.
So, let’s get started with it.
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Game Development can be a really computer intensive profession when you are trying to develop good looking games
and that can be a huge problem as not a lot of people doing it have really powerful computer.
If you are one of these people you should take a look at this article on 5 Ways To Develop Games On Low End / Low Spec PCs with Unity
where i have noted down 5 actionable steps that you can take
to make your game development experience using the Unity Game Engine a lot smoother on Low End PCs.
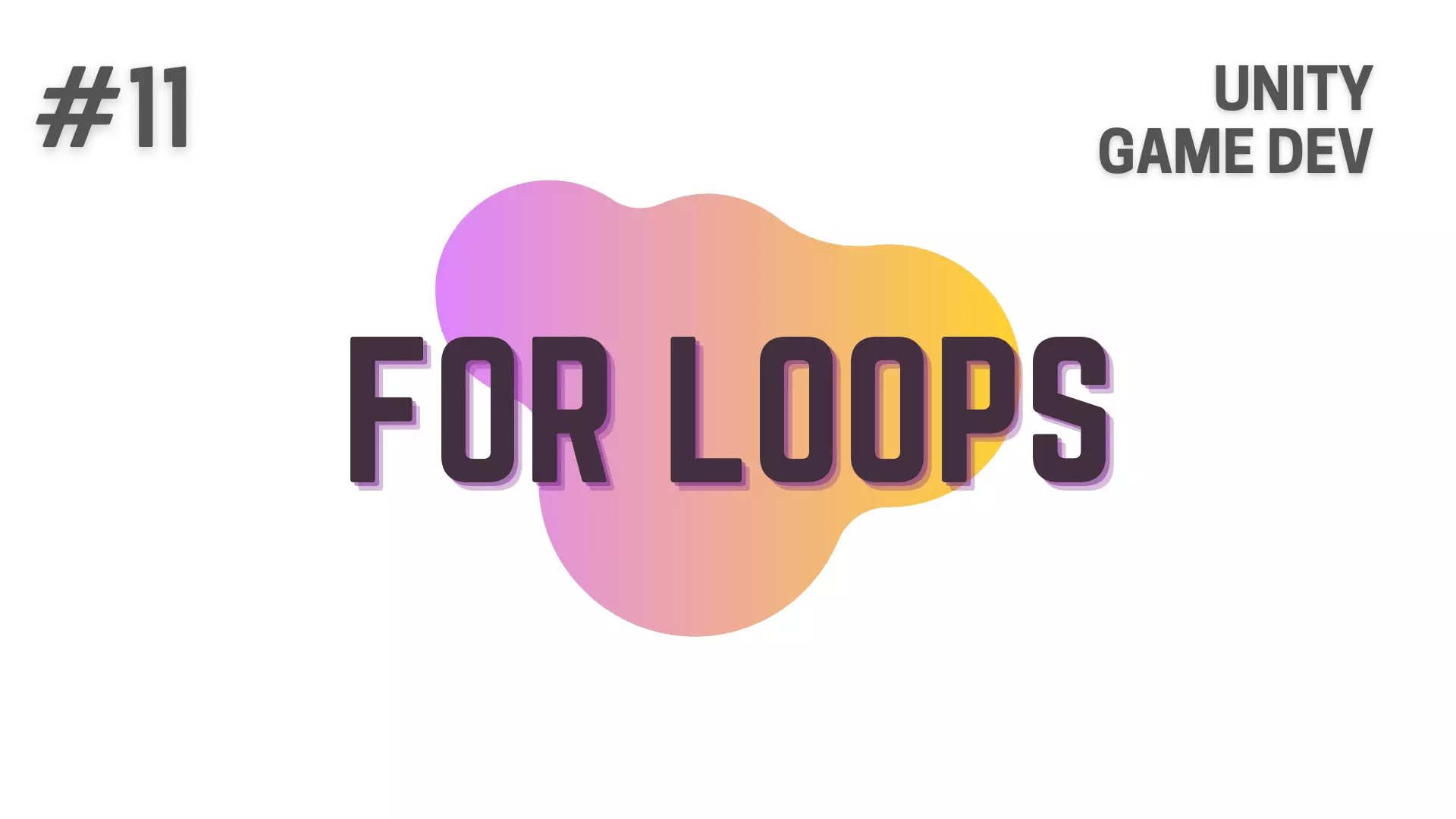
List Of Other Articles on Loops In C#
What Is A For Loop And In What Sort Of Game Development Scenario Would Your Use For Loops?
Loops are a fundamental part of programming and they are used to execute a statement or a block of code repeatedly until the specified condition return false.
Now to give more context as to why you would need to repeatedly execute a piece of code
Let’s go to the RPG analogy and think about the Inventory System in your game.
Now let’s say the inventory system is slot based and your bag has 10 slots which are stackable up to 99 items per slot.
which means that you can store 990 items in your bag at the maximum.
I hope that you are clear till here.
Now let’s say that the player is playing the game and has items inside his inventory
with some items being stackable, while some not being stackable.
What we need to do now is show these items correctly to the player when he/she opens the inventory.
That is if the items are not stackable, then we have to show unstacked
while if they are stackable, then we have to show them as stacked
with the number of stacked items shown in the top right corner of the slot.
This sort of scenario is pretty common in RPG games
and is one of the scenarios where you would be using loops.
Syntax To Write / Code A For Loop In C#
// Everything below is just a placeholder code to understand syntax.
// For working example go further down in the article.
for(Initializer ; Condition ; Iterator)
{
// Code Block To Be Executed
}
// Loop below will log 0 & 1 in console
for(int i = 0; i < 2; i++)
{
Debug.Log(i);
}
Now the Syntax for the For Loop can be divided into 5 parts
First is the for Keyword and the two Opening and Closing Parenthesis which will tell the compiler that you are writing a For Loop.
Second, inside the parenthesis we have the Initializer ( int i = 0 ),
which is used to initialize a variable that will be local to the for loop and cannot be accessed outside loop.
Third, is the Loop Condition Which will return either true or false.
If the condition return true, then the program will execute the loop again
while if the condition returns false, the loop will be stopped and the program will move forward.
Then for the Fourth Part, we have the Iterator ( i++ )
which will let you increase or decrease the value of variables
after each time that the code block inside the for loop is executed.
And Finally in the Fifth Part, we have everything inside the curly braces
which is the block of code that will be executed repeatedly
while the condition for the for loop returns true.
Simple For Loop Code Example With Unity And C#
Now, we are not going to write the whole inventory system in this article
but what we are going to do instead is
write a block of code which will test our Logic.
So first of all, let’s write a simple For Loop to log the Names of all the Items in the Player’s Inventory.
To do that let’s first declare and initialize a string array named _itemNames
that will contain Names of Items in the Player’s Inventory
and then in the Start() method write a For Loop that will iterate through each item in the _itemNames array
and log the name of each of the iterated item in the Unity Console.
// Everything Below is inside a Class.
private string[] _itemNames = new string[5] {"Sword", "Apple", "Apple", "Potion", "Dagger"};
void Start()
{
for(int i = 0; i < _itemNames.length; i++)
{
Debug.log("Item " + i + " : " + _itemNames[i]);
}
}
Breaking Down And Understand The Piece Of Code
Now let’s break down and understand this piece of code.
Here, first of all in the initializer part we initialized a new local variable i and set it’s value to 0.
then we wrote the Loop Condition which is that
the newly initialized i is less than _itemNames.Length which is 5.
and finally in the iterator part,
we increased the value of i by 1 after each loop cycle.
That means that, the code will start with i set at 0
and will loop 5 times
until the iterator increases the value of i to 5
which will result into the condition returning false
because we have set the condition as
i should be less than _itemNames.length which is 5
and 5 is not less than 5.
and when the condition returns false
the loop will be stopped.
Inside the code block, with each loop cycle
we have debug.logged the value at different indexes of _itemNames array
So when we execute this code in Unity,
we should see the logs inside the console saying
Item 0 : Sword
Item 1 : Apple
Item 2 : Apple
Item 3 : Potion
Item 4 : Dagger
Now let’s execute this code in unity and check the logs.
Executed Code
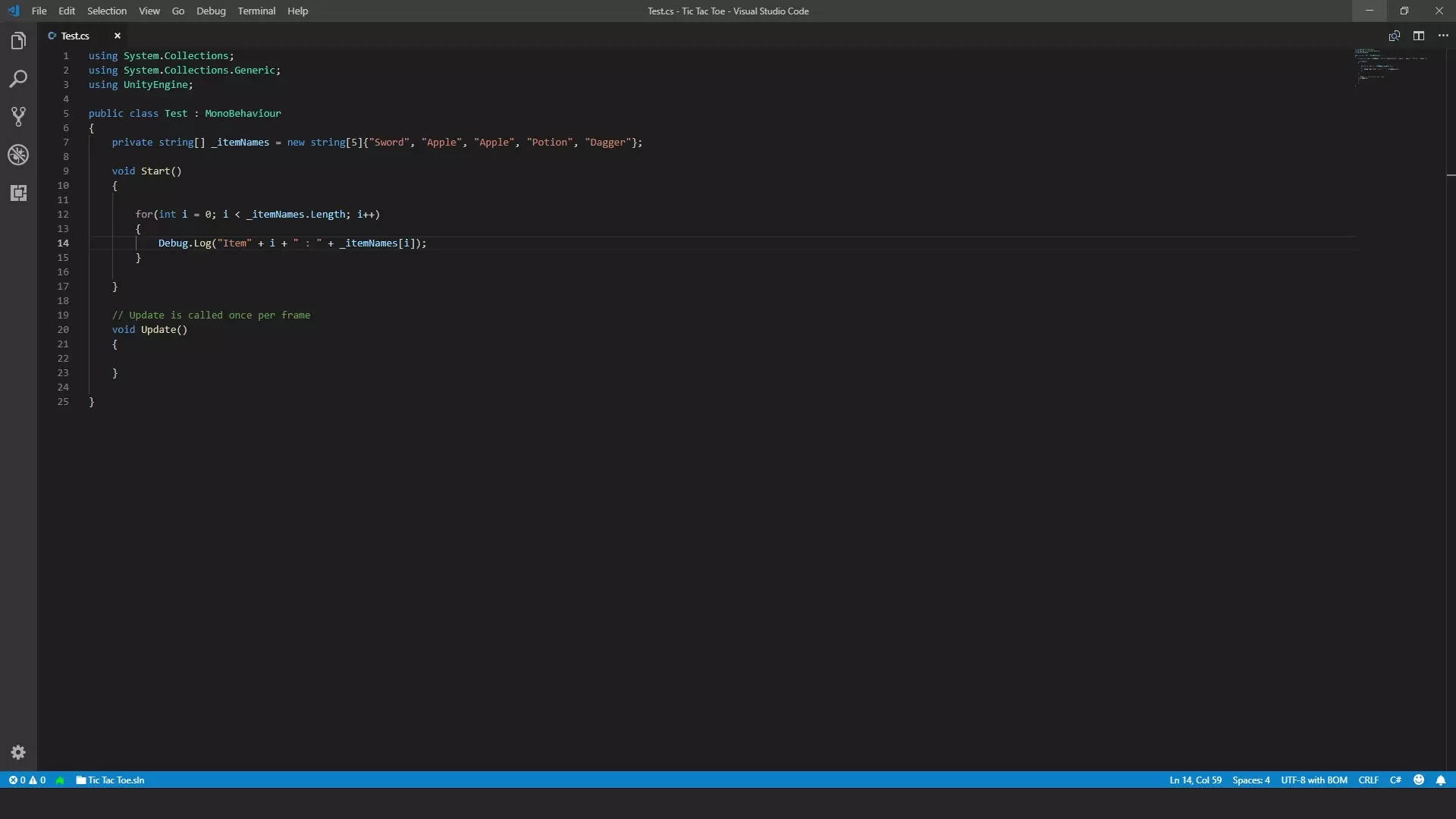
Unity Log
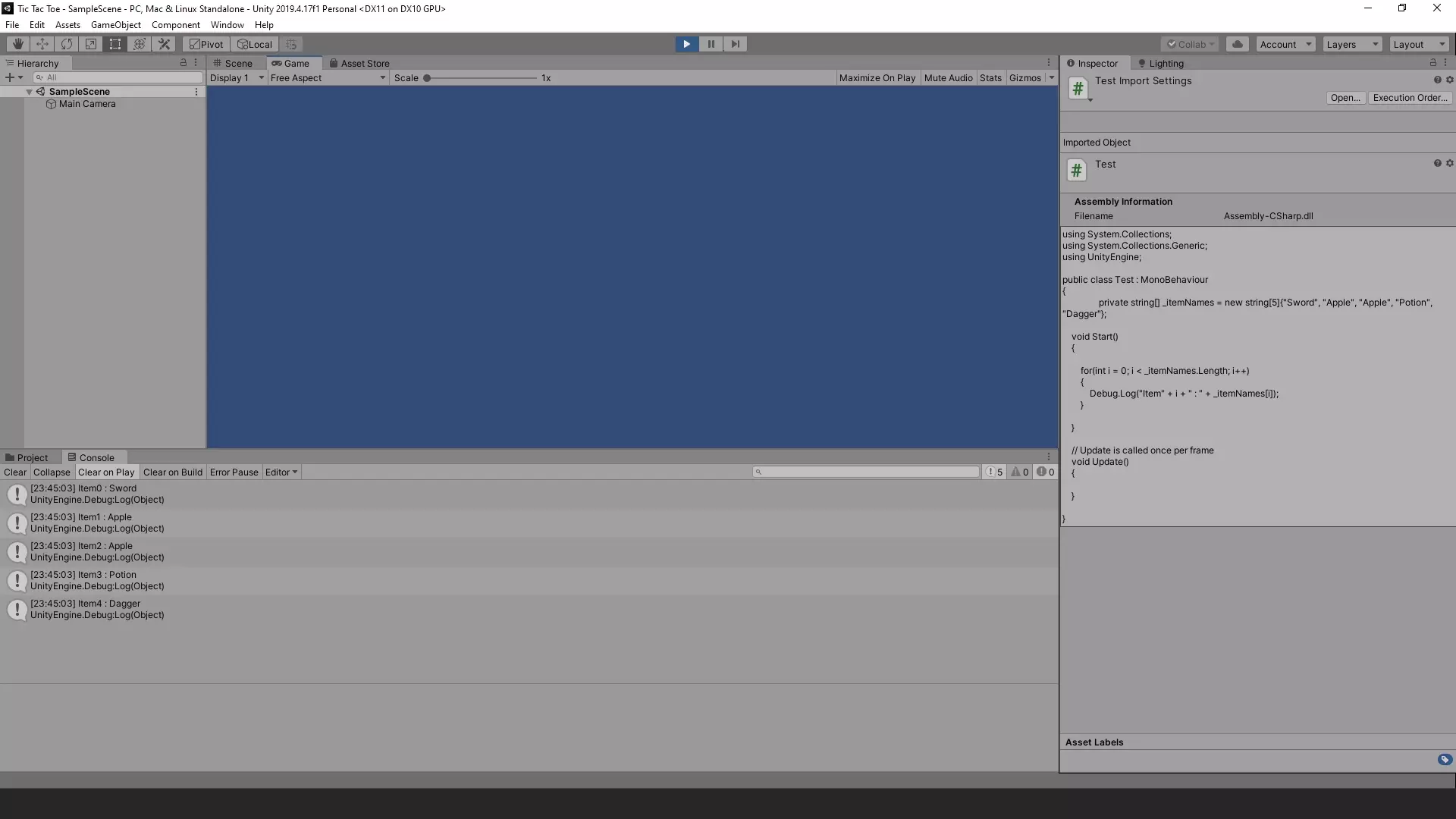
As you can see it displays all the 5 items correctly.
Separating Stackable And Non-Stackable Items Using If Statement And For Loop in C#
Back to the code, Now let’s declare another array
but this time of data type Boolean named _isStackable
with size of array set to : 5
and the members set to : false, true, true, true, false
Then we are going to do next
is to modify the piece of code that we have already written
so that it will log the Names of Stackable and Non-Stackable Items separately.
To accomplish this,
inside the for loop, we will create an if statement with the condition
_isStackable[i] == false
and debug.log the name of the item inside it.
and After that we will attach an else if block with the condition
_isStackable[i] == true
and debug.log the iterating item name inside here too.
And That it.
// Everything Below is inside a Class.
private string[] _itemNames = new string[5] {"Sword", "Apple", "Apple", "Potion", "Potion"};
private bool[] _isStackable = new bool[5]{false, true, true, true, false};
void Start()
{
for(int i = 0; i < _itemNames.length; i++)
{
if(_isStackable[i] == false)
{
Debug.Log("Item " + i +" Is Not Stackable, Name : " + _itemNames[i]);
}
else if(_isStackable[i] == true)
{
Debug.log("Item " + i + " Is Stackable, Name: " + _itemNames[i]);
}
}
}
Now let’s execute this piece of code in Unity and check to logs
Executed Code
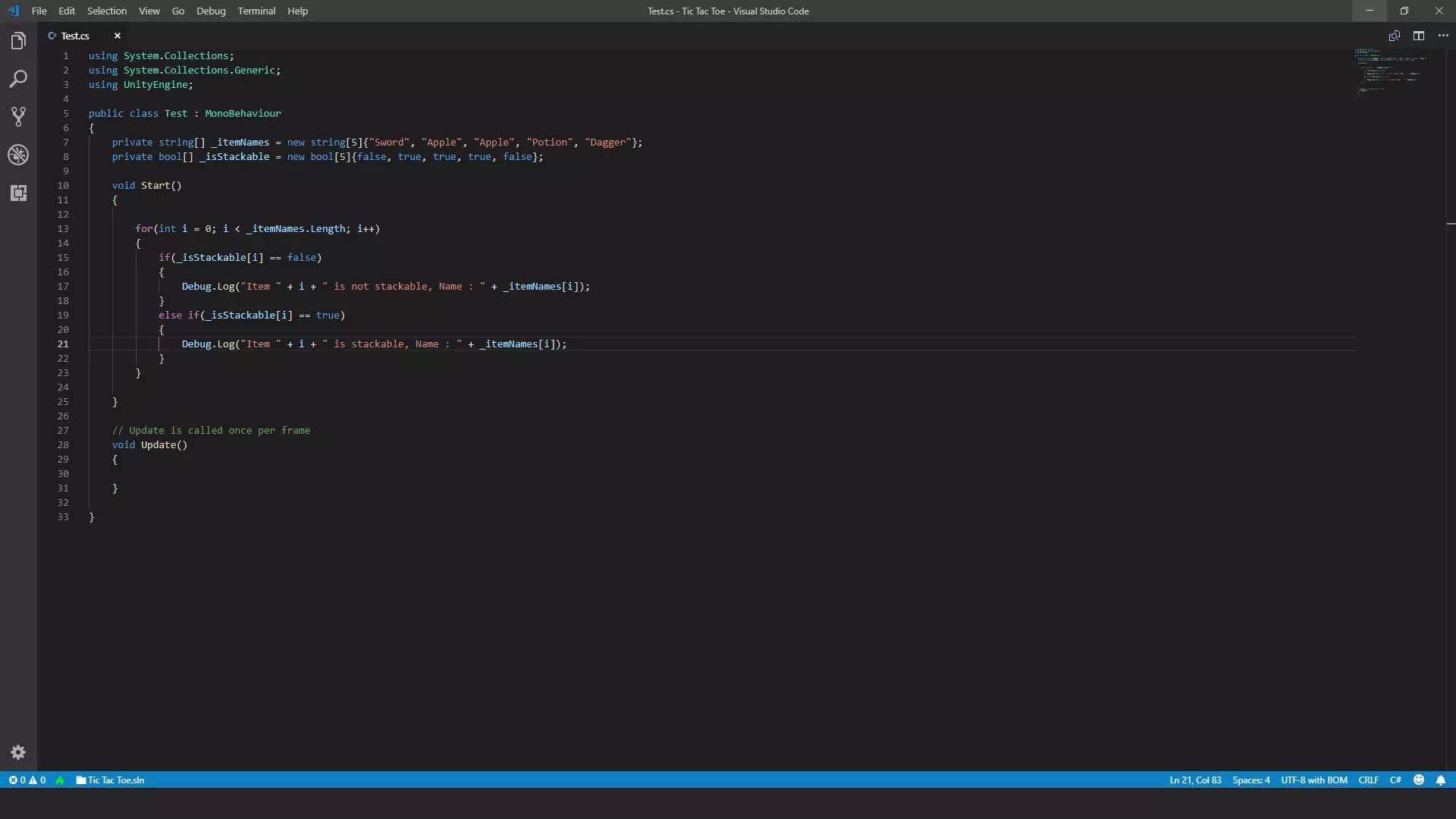
Unity Log
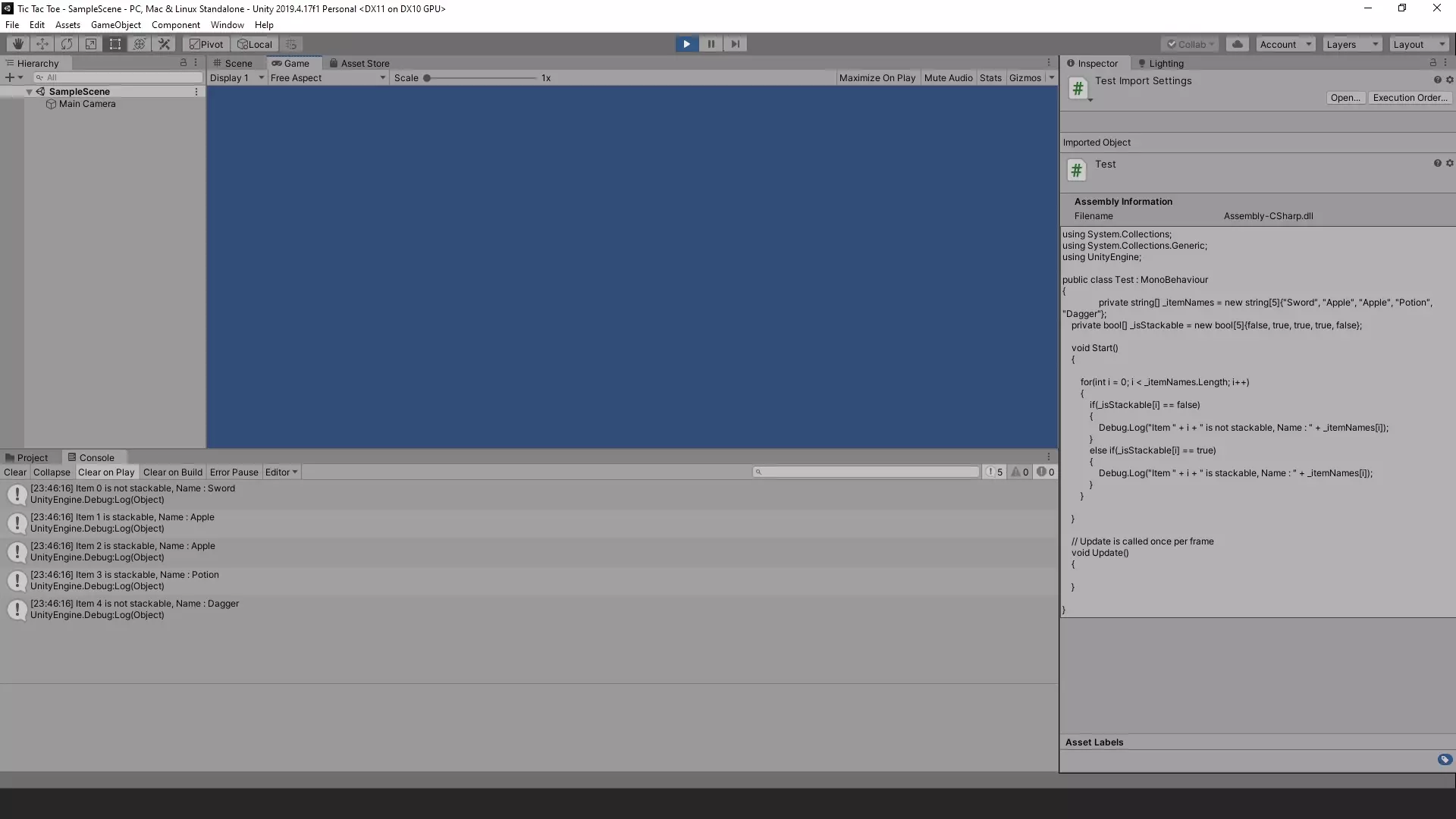
And as you can see it divided the items based on whether they are Stackable or Non-Stackable.
Solve Problems & Don’t Be Afraid Of The Size Of Code; It Won’t Bite
Now just to give a heads up, as most of you readers are beginners to programming,
it is possible that some of you might get lost in the next part
because the code is going to get a bit larger than in the previous articles, about 70 – 80 lines
but don’t rush it, if you understand everything that I’m doing then great
if not, then don’t worry, just stick around as i will slowly explain everything.
The reason why i am writing this relatively larger piece of code to explain for loop is because
i don’t want to give you a senseless example with no context
like just iterating the value of i or making a pattern with stars or that sort of thing that would be of no use to you.
but instead explain it from the perspective of real life problem solving
using only the things that i have made articles and videos on until now
which i think is a much better way of explaining any concept.
and second reason is that i want you to get used to seeing large pieces of code
because if you are serious about developing a game
then you will be dealing with thousands of lines of code in each script.
And Finally, another point i want to say is that the length of the code is of little importance
and what you should be focusing on is to understand the the problem you are trying to solve and the underlying system that you are creating solve it.
that is also the reason why i have been creating these RPG scenarios and analogies
to get you into that perspective of solving problems, instead of just typing a plus b = magic.
Well with that out of the way let’s continue.
Simple Code To Stack Inventory Items Using For Loops & Arrays in Unity with C#
Declaring And Setting Values To Stacked Item Names & Stacked Item Count Arrays
Now the purpose of the code that we are writing is to display the items in form of stacks
with the right amount of stacked value assigned to the slot.
To accomplish this we will need to declare two more arrays.
First, a string array named _inventoryItemNames
and Second an int array named _stackedItemCount
_inventoryItemNames will contain the names of stacked items.
and _stackedItemCount will contain the stacked item count for the items in _inventoryItemNames array.
Now in the Start() method
before the for loop, we will initialize these two arrays like this using the length property.
// Everything Below is inside a Class.
private string[] _itemNames = new string[5] {"Sword", "Apple", "Apple", "Potion", "Potion"};
private bool[] _isStackable = new bool[5]{false, true, true, true, false};
private string[] _inventoryItemNames;
private int[] _stackedItemCount;
void Start()
{
_inventoryItemNames = new string[_itemNames.Length];
_stackedItemCount = new int[_inventoryItemNames.Length];
for(int i = 0; i < _itemNames.length; i++)
{
if(_isStackable[i] == false)
{
Debug.Log("Item " + i +" Is Not Stackable, Name : " + _itemNames[i]);
}
else if(_isStackable[i] == true)
{
Debug.log("Item " + i + " Is Stackable, Name: " + _itemNames[i]);
}
}
}
We are doing this using the length property
because we know that even if none of the items are stackable,
the members needed to contain all the items in the _itemNames array
will not be larger than the size of the _itemNames array.
Instead of referencing using the length property,
You can try hard coding this instead
but it will be really difficult to maintain it once your project starts getting even slightly large.
So, just don’t hard code it.
moving forward, I only declared the two arrays but did not initialize them while declaring
because we would get an error saying that
“a field initializer cannot reference a non-static field, method or property.”
And as we are trying to use the Length Property of the two Arrays
which is not a Static Property
we can’t initialize the two Arrays while declaring them outside a method.
Coding For Loop & If Statement To Count Non-Stackable Items
Next, inside the for loop we have already made the if condition that separates Stackable and Non-Stackable Items.
So, inside the first conditional block where the non stackable items will go
we will add this incoming non stackable item to the _inventoryItemNames array
and at the same index set the number of stacked items in the _stackedItemCount array.
We can do this by creating a nested for loop to go through the _inventoryItemNames array
and find the index at which we have not stored an item yet by using an if condition
where we are checking the value at the current index and seeing if it’s null
If this condition returns true, then that means that we have found an empty slot
and next thing to do is to assign the name of the current item iterating in the larger for loop
to the empty slot that we just found by typing
_inventoryItemNames[j] = _itemNames[i];
and after that setting _stackedItemCount at index j to 1
as even though this item cannot be stacked,
your itemCount can’t be 0,
because the item does exist.
Next, we need to add break
because we want to assign this item only to the first empty slot that we find in the _inventoryItemNames array
and not to all the empty slots that we find.
and to stop that from happening
after we have set the values correctly in the first empty slot
we need to break the for loop and and get out it
// Everything Below is inside a Class.
private string[] _itemNames = new string[5] {"Sword", "Apple", "Apple", "Potion", "Potion"};
private bool[] _isStackable = new bool[5]{false, true, true, true, false};
private string[] _inventoryItemNames;
private int[] _stackedItemCount;
void Start()
{
_inventoryItemNames = new string[_itemNames.Length];
_stackedItemCount = new int[_inventoryItemNames.Length];
for(int i = 0; i < _itemNames.length; i++)
{
if(_isStackable[i] == false)
{
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_inventoryItemNames[j] == null)
{
_inventoryItemNames[j] = _itemNames[i];
_stackedItemCount[j] = 1;
break;
}
}
}
else if(_isStackable[i] == true)
{
Debug.log("Item " + i + " Is Stackable, Name: " + _itemNames[i]);
}
}
}
Coding For Loop & If Statement To Count Stackable Items
With that done, now let’s move on the else if code block where the Stackable Items will enter.
Inside here, the first thing we will do is
declare a boolean named _foundIdenticalItem and set it to false.
after that we will create a for loop and iterate through the _inventoryItemNames array
to find if an identical item already exists in the array.
if such an identical item exists then we will set the _foundIdenticalItem boolean to true.
and increase the _stackedItemCount variable by 1
at the index that we found the identical item
like this.
// Everything Below is inside a Class.
private string[] _itemNames = new string[5] {"Sword", "Apple", "Apple", "Potion", "Potion"};
private bool[] _isStackable = new bool[5]{false, true, true, true, false};
private string[] _inventoryItemNames;
private int[] _stackedItemCount;
void Start()
{
_inventoryItemNames = new string[_itemNames.Length];
_stackedItemCount = new int[_inventoryItemNames.Length];
for(int i = 0; i < _itemNames.length; i++)
{
if(_isStackable[i] == false)
{
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_inventoryItemNames[j] == null)
{
_inventoryItemNames[j] = _itemNames[i];
_stackedItemCount[j] = 1;
break;
}
}
}
else if(_isStackable[i] == true)
{
bool _foundIdenticalItem = false;
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_itemNames[i] == _inventoryItemNames[j])
{
_foundIdenticalItem = true;
_stackedItemCount[j] = _stackedItemCount[j] + 1;
break;
}
}
}
}
}
With that done, after the for loop we will check if _foundIdenticalItem is false.
If this returns true, it will mean that
we went through all the items in the _inventoryItemNames array
and did not find a single identical item.
Which means that, we will need to find an empty slot
and assign the currently iterating item of _itemsNames array to it.
just like in the code for non-stackable items above.
So instead of writing that code again
we can just copy and paste that piece of code here.
and with that, we have completed the code that will take the items from _itemNames array
and use the _isStackable array to stack the items correctly
After that place them inside the _inventoryItemNames array
and set the correct number of stacked items in the _stackedItemCount array
// Everything Below is inside a Class.
private string[] _itemNames = new string[5] {"Sword", "Apple", "Apple", "Potion", "Potion"};
private bool[] _isStackable = new bool[5]{false, true, true, true, false};
private string[] _inventoryItemNames;
private int[] _stackedItemCount;
void Start()
{
_inventoryItemNames = new string[_itemNames.Length];
_stackedItemCount = new int[_inventoryItemNames.Length];
for(int i = 0; i < _itemNames.length; i++)
{
if(_isStackable[i] == false)
{
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_inventoryItemNames[j] == null)
{
_inventoryItemNames[j] = _itemNames[i];
_stackedItemCount[j] = 1;
break;
}
}
}
else if(_isStackable[i] == true)
{
bool _foundIdenticalItem = false;
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_itemNames[i] == _inventoryItemNames[j])
{
_foundIdenticalItem = true;
_stackedItemCount[j] = _stackedItemCount[j] + 1;
break;
}
}
if(_foundIdenticalItem == false)
{
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_inventoryItemNames[j] == null)
{
_inventoryItemNames[j] = _itemNames[i];
_stackedItemCount[j] = 1;
break;
}
}
}
}
}
}
Logging The Correctly Stacked Items In The Unity Console
Now the final thing that we need to do is
we will Debug.Log the correctly stacked items in the Unity Console
so let’s do that
To do this we will create another for loop inside the Start() method
right after the largest for loop ends
and inside it, we will create an if condition
which will stop the program from printing the Item slots that are empty by checking for null slots
and then logging the Stacked Item Name and Stacked Item Count of Currently iterating item, like this.
// Just Writing Code For The Current For Loop
for(int i = 0; i < _inventoryItemNames.Length; i++)
{
if(_inventoryItemNames[i] != null)
{
Debug.Log("Item " + i + " : " + _inventoryItemNames[i]);
Debug.Log("Item Count : " + _stackedItemCount[i]);
}
}
And That’s it!
The code for today’s article is complete.
Man that took a long time.
Now let’s go and execute this code in Unity to check the logs
Executed Code | For Loop Code Example Unity C#
Code is too long for a single image :p.
// Everything below is inside a class.
private string[] _itemNames = new string[5] {"Sword", "Apple", "Apple", "Potion", "Potion"};
private bool[] _isStackable = new bool[5]{false, true, true, true, false};
private string[] _inventoryItemNames;
private int[] _stackedItemCount;
void Start()
{
_inventoryItemNames = new string[_itemNames.Length];
_stackedItemCount = new int[_inventoryItemNames.Length];
for(int i = 0; i < _itemNames.length; i++)
{
if(_isStackable[i] == false)
{
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_inventoryItemNames[j] == null)
{
_inventoryItemNames[j] = _itemNames[i];
_stackedItemCount[j] = 1;
break;
}
}
}
else if(_isStackable[i] == true)
{
bool _foundIdenticalItem = false;
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_itemNames[i] == _inventoryItemNames[j])
{
_foundIdenticalItem = true;
_stackedItemCount[j] = _stackedItemCount[j] + 1;
break;
}
}
if(_foundIdenticalItem == false)
{
for(int j = 0; j < _inventoryItemNames.Length; j++)
{
if(_inventoryItemNames[j] == null)
{
_inventoryItemNames[j] = _itemNames[i];
_stackedItemCount[j] = 1;
break;
}
}
}
}
}
for(int i = 0; i < _inventoryItemNames.Length; i++)
{
if(_inventoryItemNames[i] != null)
{
Debug.Log("Item " + i + " : " + _inventoryItemNames[i]);
Debug.Log("Item Count : " + _stackedItemCount[i]);
}
}
}
Unity Log | For Loop Code Example Unity C#
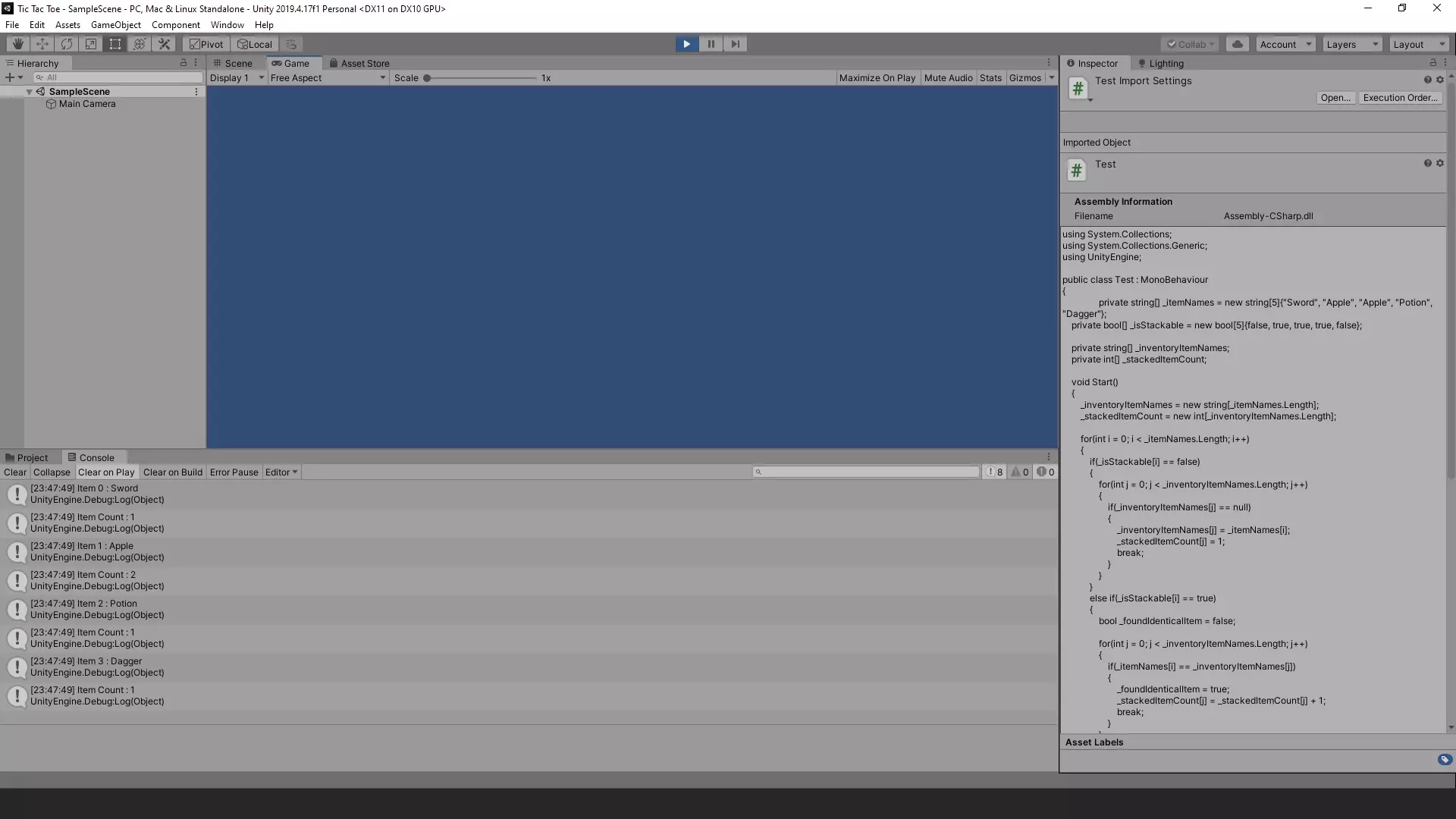
As you can see, our items were stacked correctly based on the data inside the _isStackable array.
Important Points About For Loops Before Wrapping Up This Article
Now, if this was a legit project then i would not be using arrays to store this sort of data.
Instead what i would be doing is that
i will probably create a struct or class that will contain stats of these items
and then use Dictionaries to maintain this sort of data.
Using Dictionaries will make your life much easier but that’s a topic for another article.
Next there are 4 points about For Loops that you should know.
First is that, you can initialize more than one local variable in the initializer section of the for loop
by separating the declarations with comma, like this
for(int i = 0, int j = 8; i < 10; i++){}
Second is that The control variable which is the variable you are using to control the condition of for loop
can be of any numeric data type, such as short, int, float, double, decimal, etc.
for(short i = 7; i < 10; i++){}
// OR
for(float i = 10f; i >= 0; i-2.5){}
Third is that, in this article we have looped in the positive direction from 0 to the length of an array
but you can also loop in the reverse direction by iterating the control variable negatively, like this
for(int i = array.Length; i >= 0; i--){}
// OR
for(double i = 10d; i >= 0; i-0.5){}
and the final point is that
just like you can initialize multiple variables in the initializer section,
you can iterate multiple variables in the iterator section
in whichever direction you want after every loop cycle, like this
for(int i = 0, int j = 8; i < 10; i++, j--){}
// OR
for(int i = 0, float j = 8f; i < 10; i++, j-0.25){}
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords