In C# and all the other programming languages, there are special symbols and combination of symbols which which we use to Assign values to variables.
These symbols and combination of symbols are what we call Assignment Operators in programming.
In an article before, we have already talked about Arithmetic Operators.
In this article, we are going to cover Assignment Operators and then in subsequent articles we will cover the Comparison Operators and Logical Operators.
This Post is Part of : How To Make A Game Series where i’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic. If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
If you are interested in Game Development then you might also be interested in this article on Understanding The Target Player Base Of Your Game
in which i have listed down some question that will help you better determine what your Player Base wants
and will help you make better Game Design, Game Development and Sales Decisions.
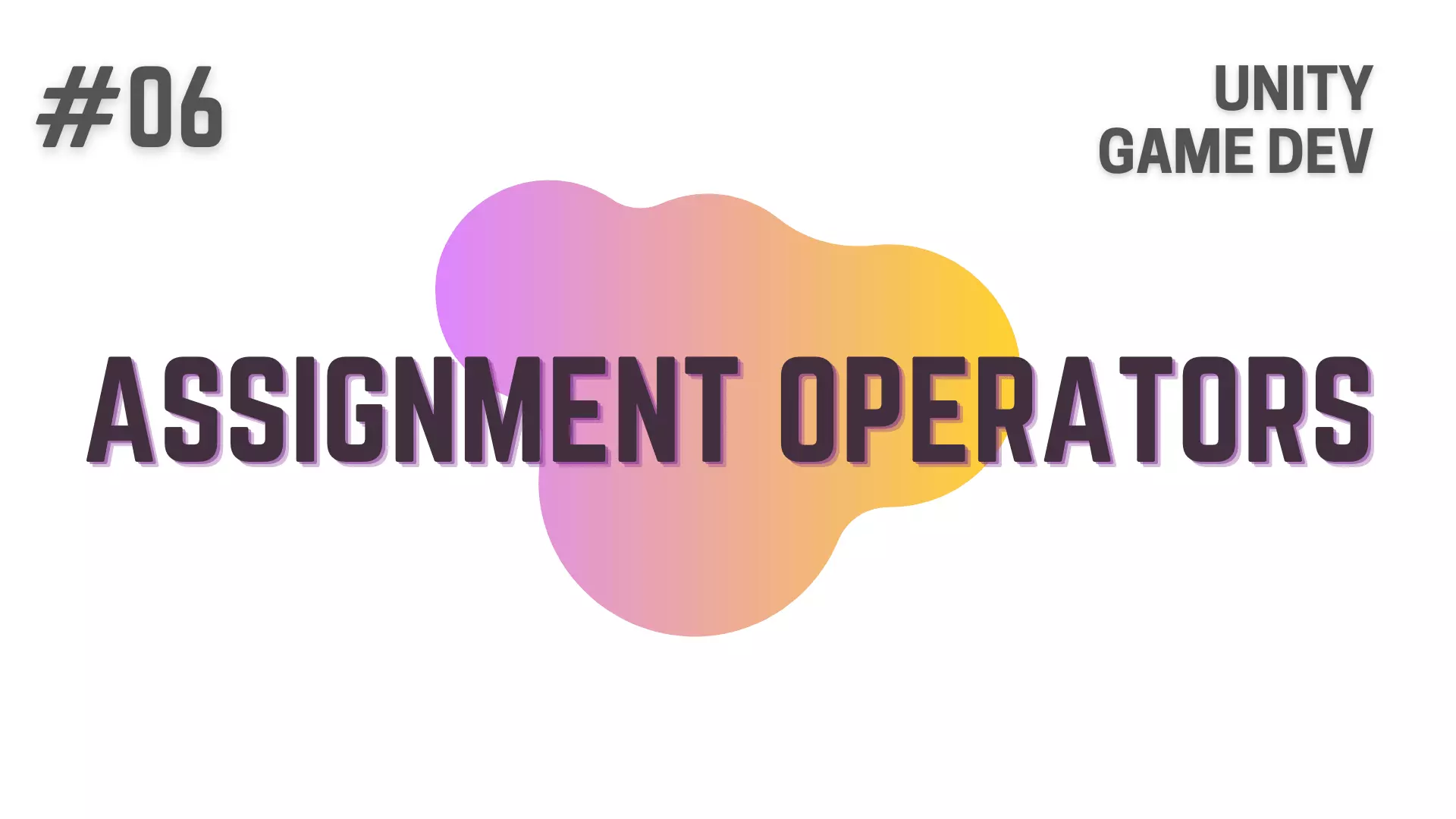
Related Articles On Operators in C#
Assignment Operators
Moving Forward, now let’s talk about Assignment Operators which are used to assign values to the variables.
There are 6 Assignment Operators that you will find useful as a Game Developer :
- Equal To Operator ( = )
- Addition Operator ( += )
- Subtraction Operator ( -= )
- Multiplication Operator ( *= )
- Division Operator ( /= )
- Modulus Operator ( %= )
assignment operator | Equal To ( = )
// Everything Below is inside a Class.
private int _playerHP = 300;
void Start()
{
_playerHP = 250 + 10; // Now _playerHP is 250 + 10 = 260.
_playerHP = _playerHP + 50; // Now _playerHP is 260 + 50 = 310.
}
Using the Equal To Assignment Operator ( = ) you can assign value that is on the right side of the Operator to the variable on the left side of the Operator.
In the code above, we are performing addition twice and are using the Equal To ( = ) Assignment Operator
to set the resulting values of those Arithmetic Operations to _playerHP variable.
assignment operator | Addition ( += )
// Everything Below is inside a Class.
private int _enemyHP = 100;
void Start()
{
_enemyHP += 30; // This Basically means _enemyHP = _enemyHP + 30.
}
Using the Addition Assignment Operator ( += ) you can perform addition between the variable on the left side of the Operator with the values or variables on the right side of the Operator.
After that Addition is done, the resulting value will be assigned to the variable on the left side of the Operator.
In the code above, we first set _enemyHP to 100 and then in the Start() Method
we are using the Addition Assignment Operator ( += ) to perform addition between _enemyHP and 30
which gives us 130, that we then set to the _enemyHP variable.
assignment operator | Subtraction ( -= )
// Everything Below is inside a Class.
private int _weaponHP = 150;
void Start()
{
_weaponHP -= 45; // This Basically means _weaponHP = _weaponHP - 45.
}
Using the Subtraction Assignment Operator ( -= ) you can perform subtraction between the variable on the left side of the Operator with the values or variables on the right side of the Operator.
After that Subtraction is done, the resulting value will be assigned to the variable on the left side of the Operator.
In the code above, we first set _weaponHP to 150 and then in the Start() Method
we are using the Subtraction Assignment Operator ( -= ) to perform subtraction between _weaponHP and 45
which gives us 105, that we then set to the _weaponHP variable.
assignment operator | Multiplication ( *= )
// Everything Below is inside a Class.
private int _weaponDamage = 10;
void Start()
{
_weaponDamage *= 4; // This Basically means _weaponDamage = _weaponDamage * 4.
}
Using the Multiplication Assignment Operator ( *= ) you can perform multiplication between the variable on the left side of the Operator with the values or variables on the right side of the Operator.
After that Multiplication is done, the resulting value will be assigned to the variable on the left side of the Operator.
In the code above, we first set _weaponDamage to 10 and then in the Start() Method
we are using the Multiplication Assignment Operator ( *= ) to perform multiplication between _weaponDamage and 4
which gives us 40, that we then set to the _weaponDamage variable.
assignment operator | Division ( /= )
// Everything Below is inside a Class.
private int _attackDamage = 125;
void Start()
{
_attackDamage /= 5; // This Basically means _attackDamage = _attackDamage / 5.
}
Using the Division Assignment Operator ( /= ) you can perform division between the variable on the left side of the Operator with the values or variables on the right side of the Operator.
After that Division is done, the resulting value will be assigned to the variable on the left side of the Operator.
In the code above, we first set _attackDamage to 125 and then in the Start() Method
we are using the Division Assignment Operator ( /= ) to perform division between _attackDamage and 5
which gives us 25, that we then set to the _attackDamage variable.
assignment operator | Modulus ( %= )
// Everything Below is inside a Class.
private int _moduloExample = 13;
void Start()
{
_moduloExample %= 3; // This Basically means _moduloExample = _moduloExample % 3.
}
Using the Modulus Assignment Operator ( %= ) you can perform modulo calculation between the variable on the left side of the Operator with the values or variables on the right side of the Operator.
Note : Modulus is finding the remainder of a division calculation between two numbers.
After that modulo calculation is done, the resulting value will be assigned to the variable on the left side of the Operator.
In the code above, we first set _moduloExample to 13 and then in the Start() Method
we are using the Modulus Assignment Operator ( %= ) to perform modulo calculation between _moduloExample and 3
which gives us 1, that we then set to the _moduloExample variable.
Code Without Assignment Operators
// Everything Below is inside a Class.
private int _weaponHP;
void Start()
{
_weaponHP = 0;
_weaponHP = _weaponHP + 20;
Debug.Log("_weaponHP 0 + 20 = " + _weaponHP);
_weaponHP = _weaponHP - 16;
Debug.Log("_weaponHP 20 - 16 = " + _weaponHP);
_weaponHP = _weaponHP * 4;
Debug.Log("_weaponHP 4 * 4 = " + _weaponHP);
_weaponHP = _weaponHP / 4;
Debug.Log("_weaponHP 16 /4 = " + _weaponHP);
_weaponHP = _weaponHP % 4;
Debug.Log("_weaponHP 4 % 4 = " + _weaponHP);
}
Same Code But With Assignment Operators
// Everything Below is inside a Class.
private int _weaponHP;
void Start()
{
_weaponHP = 0;
_weaponHP += 20;
Debug.Log("_weaponHP 0 + 20 = " + _weaponHP);
_weaponHP -= 16;
Debug.Log("_weaponHP 20 - 16 = " + _weaponHP);
_weaponHP *= 4;
Debug.Log("_weaponHP 4 * 4 = " + _weaponHP);
_weaponHP /= 4;
Debug.Log("_weaponHP 16 /4 = " + _weaponHP);
_weaponHP %= 4;
Debug.Log("_weaponHP 4 % 4 = " + _weaponHP);
}
If you ask me i like the verbose method more as it is really easy to read through
but of course there are people that like using these assignment operators instead.
Now, let’s execute the code in the image below and check the Unity Console Logs.
Executed Code
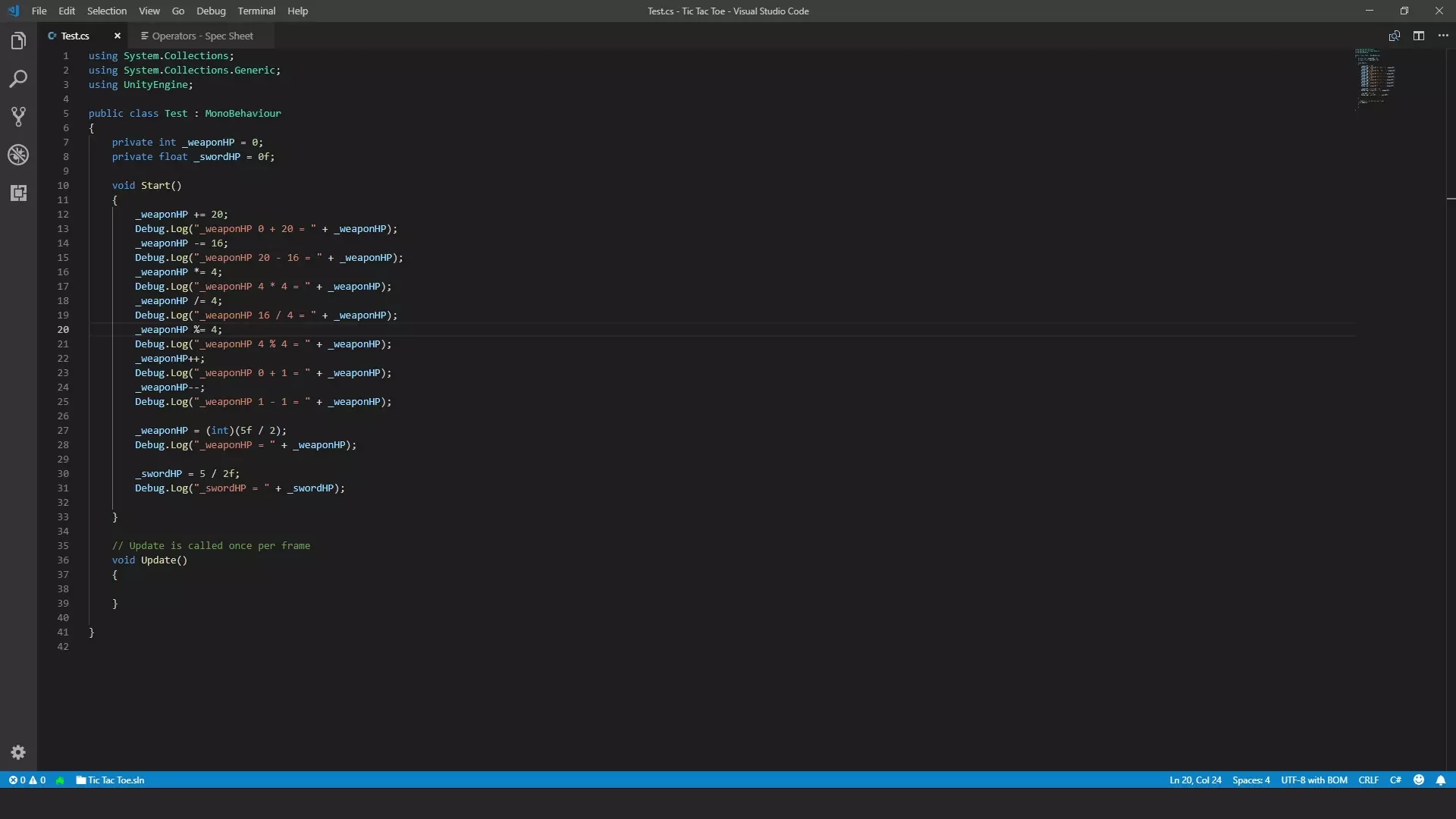
Unity Log
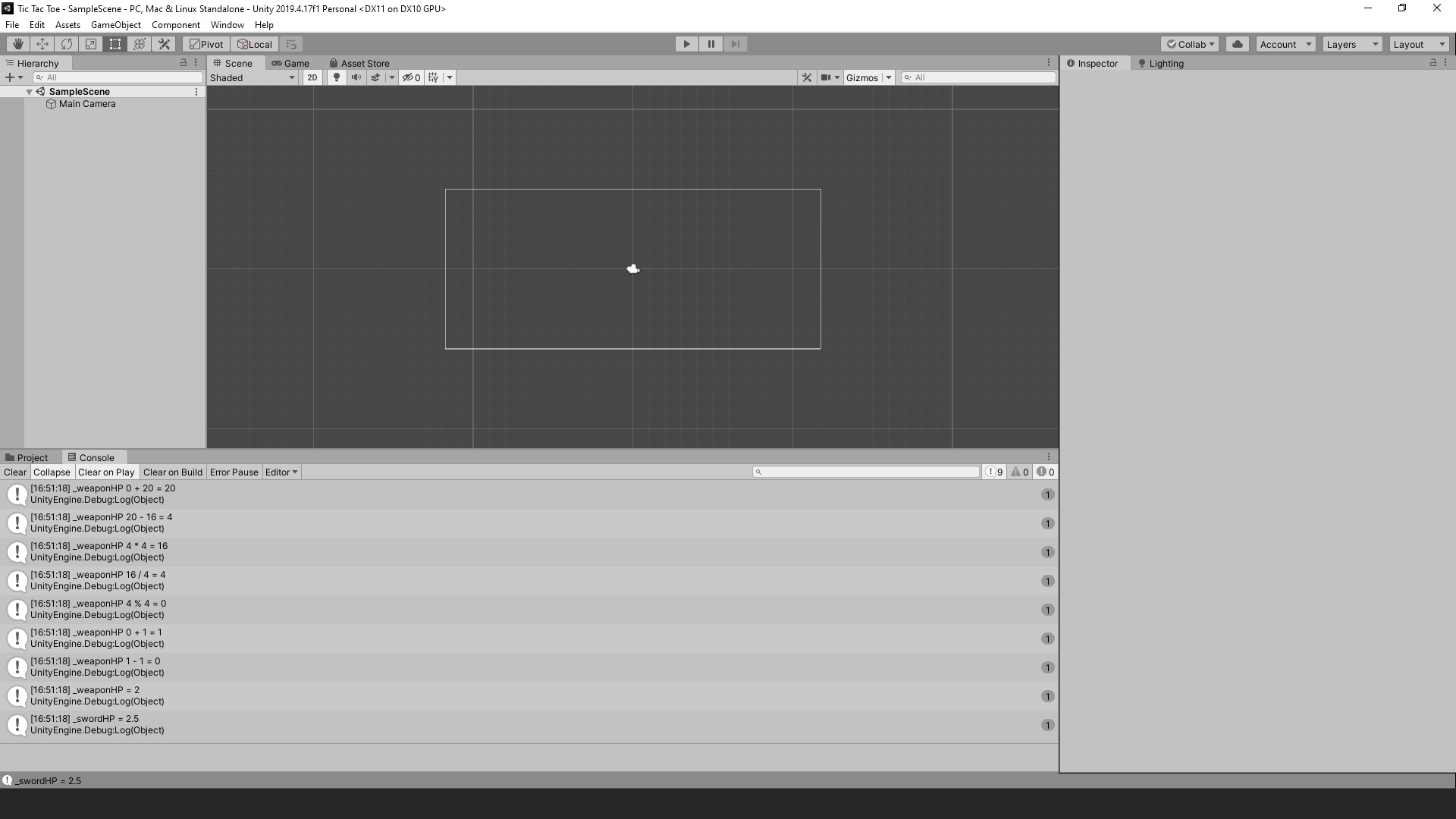
As you can see all the Arithmetic Calculations done using the Assignment Operators gave the expected output.
This means that, if you so wish,
you can use the Assignment Operators Instead of the Arithmetic Operators where it makes sense in your code.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords