In the last article, we went over everything related to the Basics of Methods,
if you don’t know anything about methods and have not read that article i suggest that you read it first!
I’ve divided my explanation of Methods in 4 different articles as otherwise the articles would be too long.
List Of All Articles To Master Methods In C#
- Part 1 – Basics Of Methods
- Part 2 – Multiple, Required and Optional Parameter + Named Arguments <— Currently You Are Here! :p
- Part 3 – Overload Resolution
- Part 4 – In, Out and Ref Keywords
In this article, I’ll will be covering the different sorts of Parameters and Arguments that you can use when Defining and Making Calls to Methods in C#, like
- How To Define Methods with Multiple Parameters?
- What are Named Arguments and how can you use then when Making Calls to Methods in C#?
- What are Required and Optional Parameters? and how can you use them while Defining Methods in C#
- Code Examples for all of them based on an RPG Analogy
So, without further ado, let’s dive directly into it!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
Before you start Designing and Developing a Game, you have to take some time to research what softwares you are going to use to develop your game.
Now, this can take a long time, so to make your job a bit easier, I’ve written this article on Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
in which I’ve talked about all the major categories of softwares that you will require to develop your game
and have give multiple options for each game development category, so that you are literally spoiled for choice.
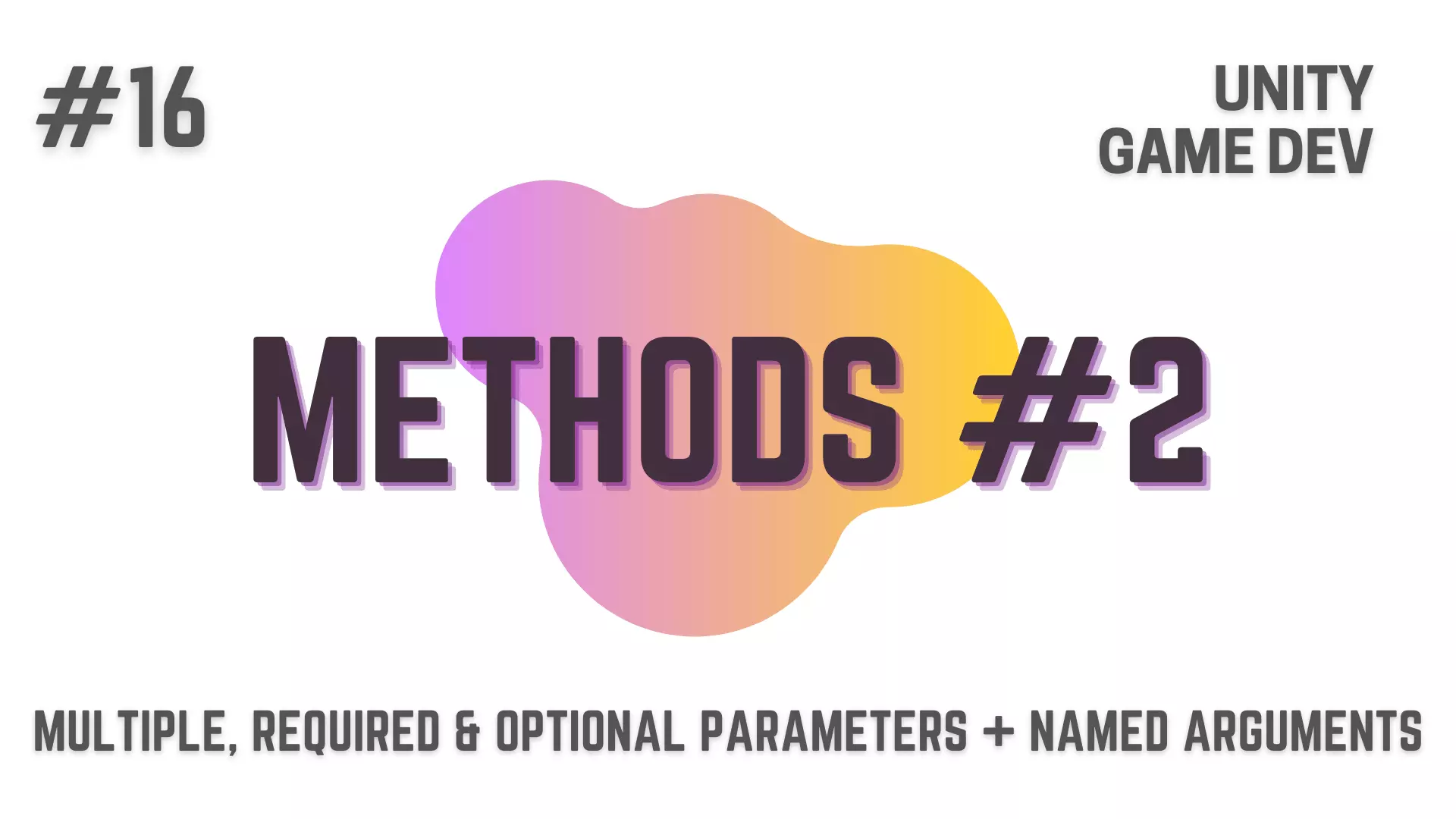
Methods With Multiple Parameters | Unity C# Game Development Tutorial
We have already talked about what Methods are
and how you can create methods with and without parameters in the last article.
Now let’s move on and directly go to the RPG analogy
so that we can write some methods with Multiple Parameters.
Think about a scenario in the game where the player is driving a car.
Now for the player to drive this car,
you the god of creation will have to write the movement script for the car
which would be different from the script that the player uses to control the main character
Now the Movement Script of the Car will take Multiple Parameters like
- Horizontal Movement
- Vertical Movement
- Current Speed
- Max Speed
- Boost Level
- Use Boost
- etc
So, let’s first create this Method with Multiple parameters and Debug.Log them in the Unity Console by typing
// Everything below is inside a Class.
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed, float boostLevel, bool useBoost)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
How Are The Arguments Evaluated Inside A Method Call in C#?
Okay, so lets stop here for a second and talk about how Arguments that you pass inside the Method Call are evaluated.
The program evaluates the Arguments based on the Position that the Argument has in the Parameter List
i.e. in the case for our carMovement Method,
the program will assign the first argument to horizontalMovement
then the next argument will be assigned to verticalMovement
and so on.
Now, some of you might already be thinking that,
some methods that you will create might have a lot of parameters
and remembering the order of these parameters will become impossible
if you are not using something like intelliSense i.e An intelligent Code Completion Software.
So, to remedy this problem in C#,
you can use something called Named Arguments.
What Are Named Arguments? And How Can You Use Them In Method Calls?
Named Arguments free you from matching the order of parameters in the parameter list of called method.
The argument for each parameter can be specified by the parameter name
and they will be evaluated in the order in which they appear in the Argument List
instead of the order that they come in the Parameter List.
So let’s go to the Start Method
and first make a Method Call using the Position in the Parameter List by typing
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, 0.8f, false);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed, float boostLevel, bool useBoost)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
and with that done, let’s make a Method Call using the Named Argument
but in no particular order like in the code below
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, 0.8f, false);
carMovement(currentSpeed: 180f, horizontalMovement: 1f, useBoost: true, verticalMovement: 0f, maxSpeed: 250f, boostLevel: 0.3f);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed, float boostLevel, bool useBoost)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
And this works too!
but what you need to make sure is that
you use Named Arguments for all the arguments as above
because if you are mixing and matching Positional and Named Arguments
you have be sure that the position at which you are placing the Named Argument
is before it’s position in the Parameter List
and to be honest you would be defeating the whole purpose of Named Arguments
while also increasing your work in that case
so just don’t do that.
Now if used correctly, it should be easy for you to understand,
how this can be really helpful.
The funny thing is that, I have never needed to use Named Arguments
as intellisense in VS Code is quite good
and it makes it really simple to pass the arguments correctly without this extra step.
But i can definitely understand that if i was using an editor without good intellisense
how Named Arguments will be a lifesaver.
Now, let’s go and execute this piece of code in Unity to check the logs.
Unity Log | Named Arguments In C# – Code Example | Unity Game Development Tutorial
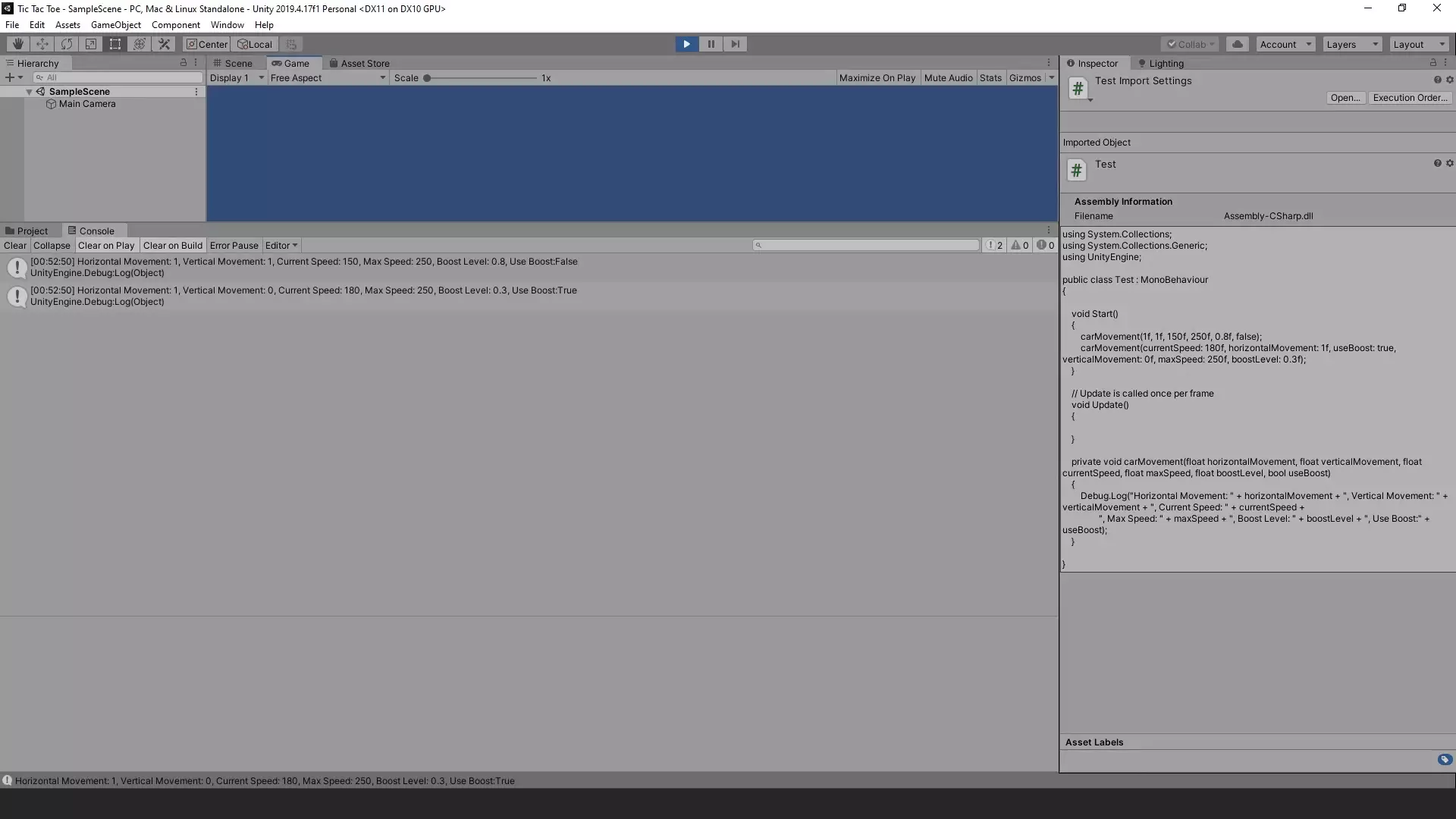
As you can see both the methods are showing the logs correctly
and that means that the Named Arguments are working as intended.
What are Required Parameters & Optional Parameters?
Now, let’s talk about what Optional Parameters are and how you can specify them when you define a method.
As it’s name suggest, any method call must provide arguments for all Required Parameters,
but can omit Arguments for Optional Parameters.
Each Optional Parameter has a default value as part of its definition.
and if we don’t pass an argument for that Optional Parameter when the method is called
then the program will use the default value in this place.
How To Use Required and Optional Parameters in C# and Unity?
To use Optional parameters we have to define them at the end of the Parameter List, after all the Required Parameters have been listed.
So, going back to the code, let’s modify the carMovement method
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, 0.8f, false);
carMovement(currentSpeed: 180f, horizontalMovement: 1f, useBoost: true, verticalMovement: 0f, maxSpeed: 250f, boostLevel: 0.3f);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f, float boostLevel = 0f, bool useBoost = false)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
As you can see here, just to show what this all means,
we have changed maxSpeed, boostLevel and useBoost parameters into Optional Parameters
by setting a default value for them in the method definition.
If there are multiple optional parameters like we have in our method
and the caller provides an argument for any one of these Optional Parameters ( Without using Named Arguments ),
then the caller must also provide arguments for all Preceding Optional Parameters that are before it in the Parameter List.
i.e. if we pass an argument for boostLevel,
then you also need to pass an argument for maxSpeed.
but it will be okay to not pass an argument for useBoost.
carMovement(1f, 1f, 150f, 250f, , false);
You can’t use Comma-separated gaps in the Argument List as in the code above
because they are not supported and will cause Compile Time Error.
However, if you know the name of the parameter,
then you can use a named argument to accomplish this task
and not give arguments for the Preceding Parameters, like this.
carMovement(1f, 1f, 150f, 250f, useBoost: false);
Summarizing Optional Parameters
So to reaffirm again simply,
Optional Parameters are parameters with default values assigned to them when you define them in the Method.
and if you pass an argument for this optional parameters when you make the call to the method
then the program will use the passed arguments instead of the default values inside these parameters
and if you don’t pass an argument for these optional parameters
then the program will use the default values when you use these variables inside method’s code block.
Another thing is that, if you have intellisense working in VS Code
and you hover over the method call
you will see a dialogue box like this appear
that shows you your method definition as shown below
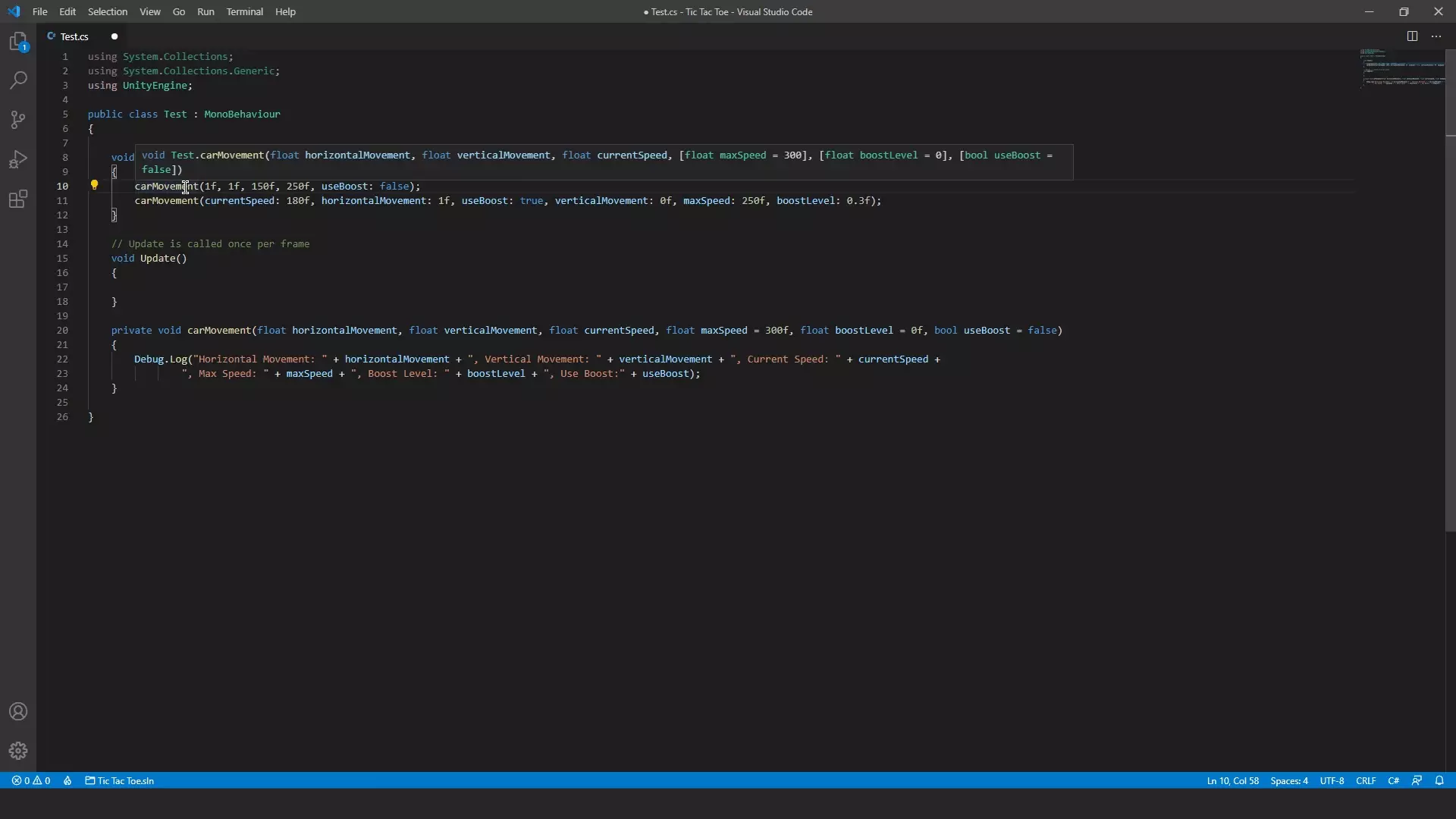
Here the parameters that are within Square Brackets are Optional Parameter.
Modifying The Code Example
Now let’s modify one of the method calls, so that it will only pass the Required Arguments like in the code below
// Everything below is inside a Class.
void Start()
{
carMovement(1f, 1f, 150f, 250f, useBoost: false);
carMovement(1f, 1f, 180f);
}
private void carMovement(float horizontalMovement, float verticalMovement, float currentSpeed, float maxSpeed = 300f, float boostLevel = 0f, bool useBoost = false)
{
Debug.Log("Horizontal Movement: " + horizontalMovement + ", Vertical Movement: " + verticalMovement + ", Current Speed: " + currentSpeed + ", Max Speed: " + maxSpeed + ", Boost Level: " + boostLevel + ", Use Boost: " + useBoost);
}
and we already have the method call above it which uses Named and Optional Parameters
So, now let’s go to Unity and check the logs.
Unity Log | Required Parameters and Optional Parameters in C# – Code Example
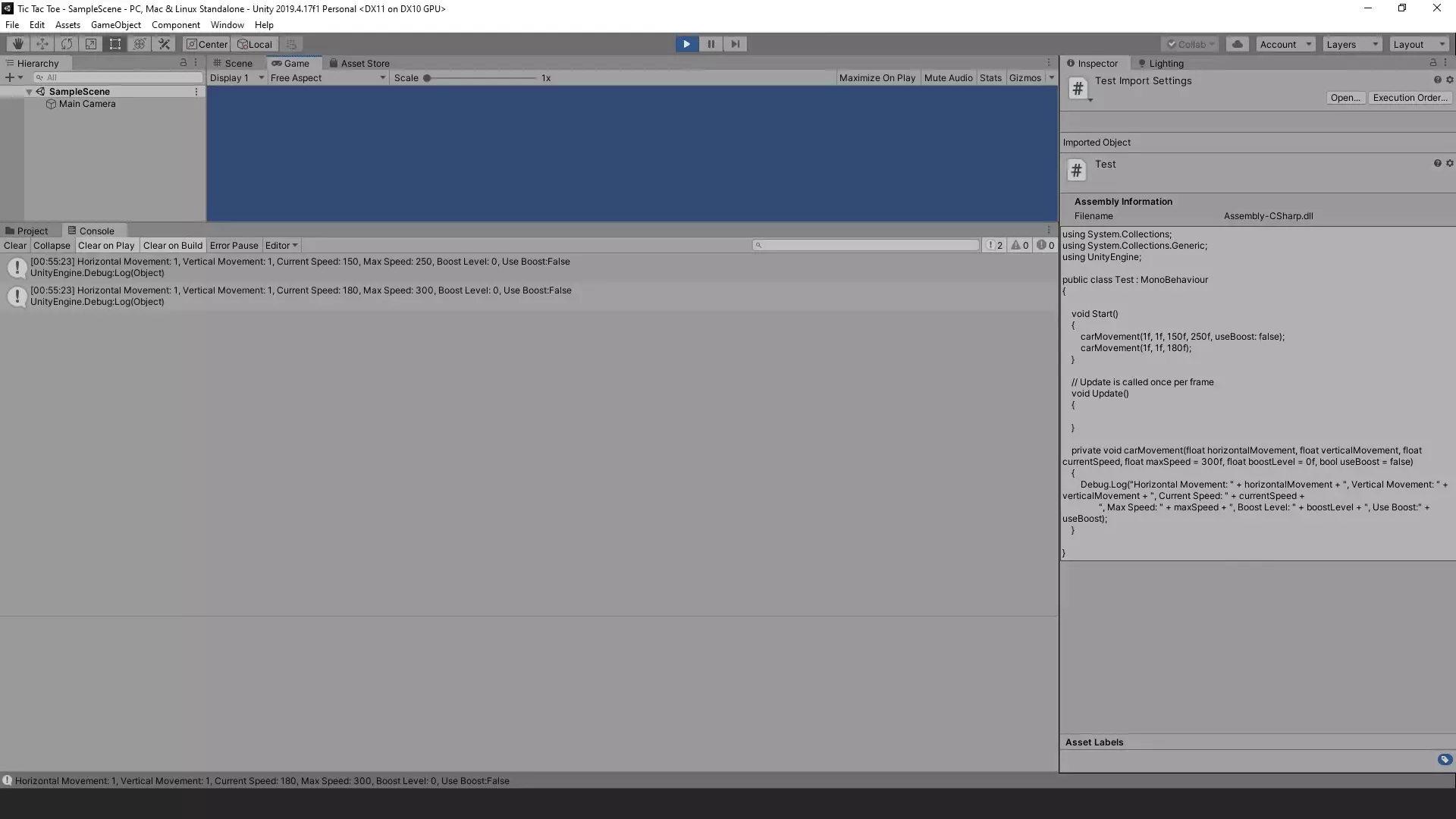
As you can see, in the first Debug.Log, we are both the Arguments passed in the Method Call and the Default Values in the Method Definition
while in the second Debug.Log, we are using default values for all optional parameters.
With that, i think i have covered everything regarding Methods with Multiple Parameters, Named Arguments, Required Parameters and Optional Parameters
In the Next article, I’ll be going over how Overload Resolution works when Methods are Overloaded in C#
It is a really important concept to understand, so do look at that article!.
List Of All Articles To Master Methods In C#
- Part 1 – Basics Of Methods
- Part 2 – Multiple, Required and Optional Parameter + Named Arguments <— Currently You Are Here! :p
- Part 3 – Overload Resolution
- Part 4 – In, Out and Ref Keywords
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords