In C# and all the other programming languages, we use special symbols for performing Mathematical / Arithmetic Calculations.
These symbols are what we call Arithmetic Operators in programming and you can basically consider them staples of the programming world.
In this article, we are going to cover Arithmetic Operators and then in subsequent articles we will cover the Assignment Operators, Comparison Operators and Logical Operators.
This Post is Part of : How To Make A Game Series where i’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
If you are interested in Game Development then you might like this article on 3 Points To Consider Before Committing To A Game Idea.
in which i talk about the things i personally think about when I’m evaluating whether a Game Idea is worth pursuing or not.
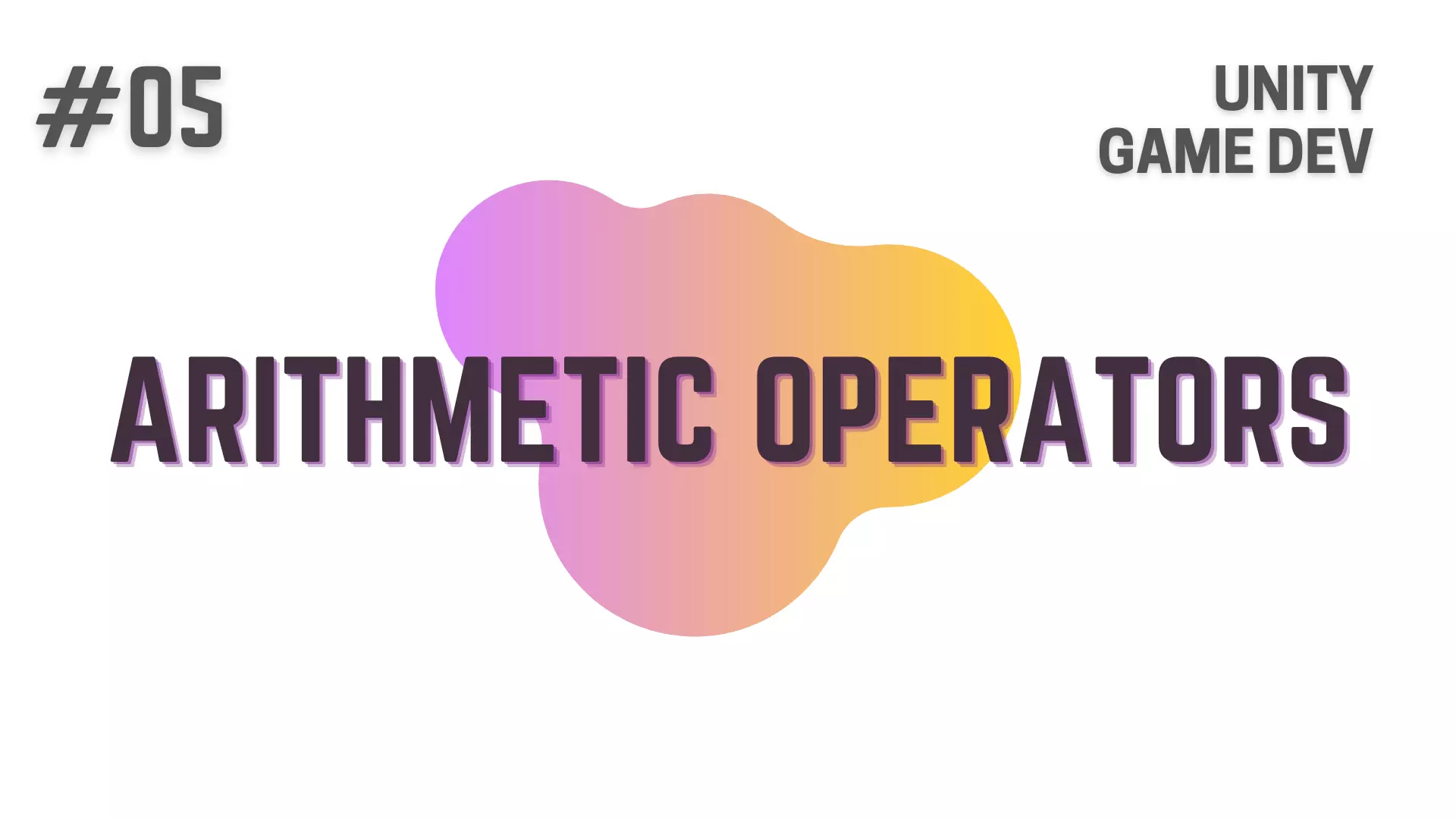
Related Articles On Operators in C#
Arithmetic Operators
So, Let’s get started with Arithmetic Operators.
In C# there are 7 Arithmetic Operators that you will find useful as a Game Developer :
- Addition Operator ( + )
- Subtraction Operator ( – )
- Multiplication Operator ( * )
- Division Operator ( / )
- Modulus Operator ( % )
- Increment Operator ( ++ )
- Decrement Operator ( ‐‐ )
Arithmetic Operator | Addition Operator ( + )
// Everything Below is inside a Class.
private int _playerHP = 250;
void Start()
{
_playerHP = 25 + 2; // Now _playerHP is 25 + 2 = 27.
_playerHP = _playerHP + 40; // Now _playerHP is 27 + 40 = 67.
}
Using the Addition Arithmetic Operator you can add two numbers as you can see in the Code example above.
In the code above, we are first setting _playerHP variable to 250, when we declare and initialize it.
After that, in the start method we overwrite 250 with 27, which is the output of Calculation 25 + 2.
Next, we use the data stored inside _playerHP which now is 27 and perform addition again with 40 giving us the result of 67, which we set to the _playerHP variable.
Arithmetic Operator | Subtraction Operator ( – )
// Everything Below is inside a Class.
private int _weaponHP = 100;
void Start()
{
_weaponHP = 100 - 8; // Now _weaponHP is 100 - 8 = 92.
_weaponHP = _weaponHP - 40; // Now _weaponHP is 92 - 40 = 52.
}
Using the Subtraction Arithmetic Operator you can subtract two numbers as you can see in the Code example above.
In the code above, we are first setting _weaponHP variable to 100, when we declare and initialize it.
After that, in the start method we overwrite 100 with 92, which is the output of Calculation 100 – 8.
Next, we use the data stored inside _weaponHP, which now is 92 and perform subtraction again with 40 giving us the result of 52, which we set to the _weaponHP variable.
Arithmetic Operator | Multiplication Operator ( * )
// Everything Below is inside a Class.
private int _swordDamage = 10;
void Start()
{
_swordDamage = 10 * 3; // Now _swordDamage is 10 * 3 = 30.
_swordDamage = _swordDamage * 2; // Now _swordDamage is 30 * 2 = 60.
}
Using the Multiplication Arithmetic Operator you can multiply two numbers as you can see in the Code example above.
In the code above, we are first setting _swordDamage variable to 10, when we declare and initialize it.
After that, in the start method we overwrite 10 with 30, which is the output of Calculation 10 * 3.
Next, we use the data stored inside _swordDamage, which now is 30 and perform multiplication again with 2 giving us the result of 60, which we set to the _swordDamage variable.
Arithmetic Operator | Division Operator ( / )
// Everything Below is inside a Class.
private int _attackDamage = 20;
void Start()
{
_attackDamage = 20 / 2; // Now _attackDamage is 20 / 2 = 10.
_attackDamage = _attackDamage / 10; // Now _attackDamage is 10 / 10 = 1.
}
Using the Division Arithmetic Operator you can divide two numbers as you can see in the Code example above.
In the code above, we are first setting _attackDamage variable to 20, when we declare and initialize it.
After that, in the start method we overwrite 20 with 10, which is the output of Calculation 20 / 2.
Next, we use the data stored inside _attackDamage, which now is 10 and perform division again with 10 giving us the result of 1, which we set to the _attackDamage variable.
Arithmetic Operator | Modulus Operator ( % )
// Everything Below is inside a Class.
private int _totalItems = 22;
private int _itemsToBeThrown = 0;
void Start()
{
_itemsToBeThrown = 21 % 2; // Now _itemsToBeThrown is 21 % 2 = 1.
_itemsToBeThrown = _totalItems % 2; // Now _itemsToBeThrown is 22 % 2 = 0.
}
Using the Modulus Arithmetic Operator you can find the remainder of division calculation between two numbers as you can see in the Code example above.
In the code above, we are first setting _totalItems variable to 22 & _itemsToBeThrown to 0 when we declare and initialize them.
After that, in the start method we overwrite _itemsToBeThrown which was 0 with 1, which is the output of Calculation 21 % 2.
Next, we use the data stored inside _totalItems, which is 22 and perform modulo calculation again with 2 giving us the result of 0, which we set to the _itemsToBeThrown variable.
Arithmetic Operator | Increment Operator ( ++ )
// Everything Below is inside a Class.
private int _npcHP = 100;
void Start()
{
_npcHP++; // Now _npcHP will be 101.
}
Using the Increment Arithmetic Operator you can increase the numerical data stored inside the variable by 1 as you can see in the Code example above.
In the code above, we are first setting _npcHP variable to 100, when we declare and initialize it.
After that, in the start method we overwrite _npcHP which was 100 with 101, which is the output of Calculation _npcHP++
_npcHP++ can be considered as a shorthand for _npcHP = _npcHP + 1
Arithmetic Operator | Decrement Operator ( ‐‐ )
// Everything Below is inside a Class.
private int _enemyHP = 200;
void Start()
{
_enemyHP--; // Now _enemyHP will be 199.
}
Using the Decrement Arithmetic Operator you can decrease the numerical data stored inside the variable by 1 as you can see in the Code example above.
In the code above, we are first setting _enemyHP variable to 200, when we declare and initialize it.
After that, in the start method we overwrite _enemyHP which was 200 with 199, which is the output of Calculation _enemyHP‐‐
_enemyHP‐‐ can be considered as a shorthand for _enemyHP = _enemyHP – 1
Code Example
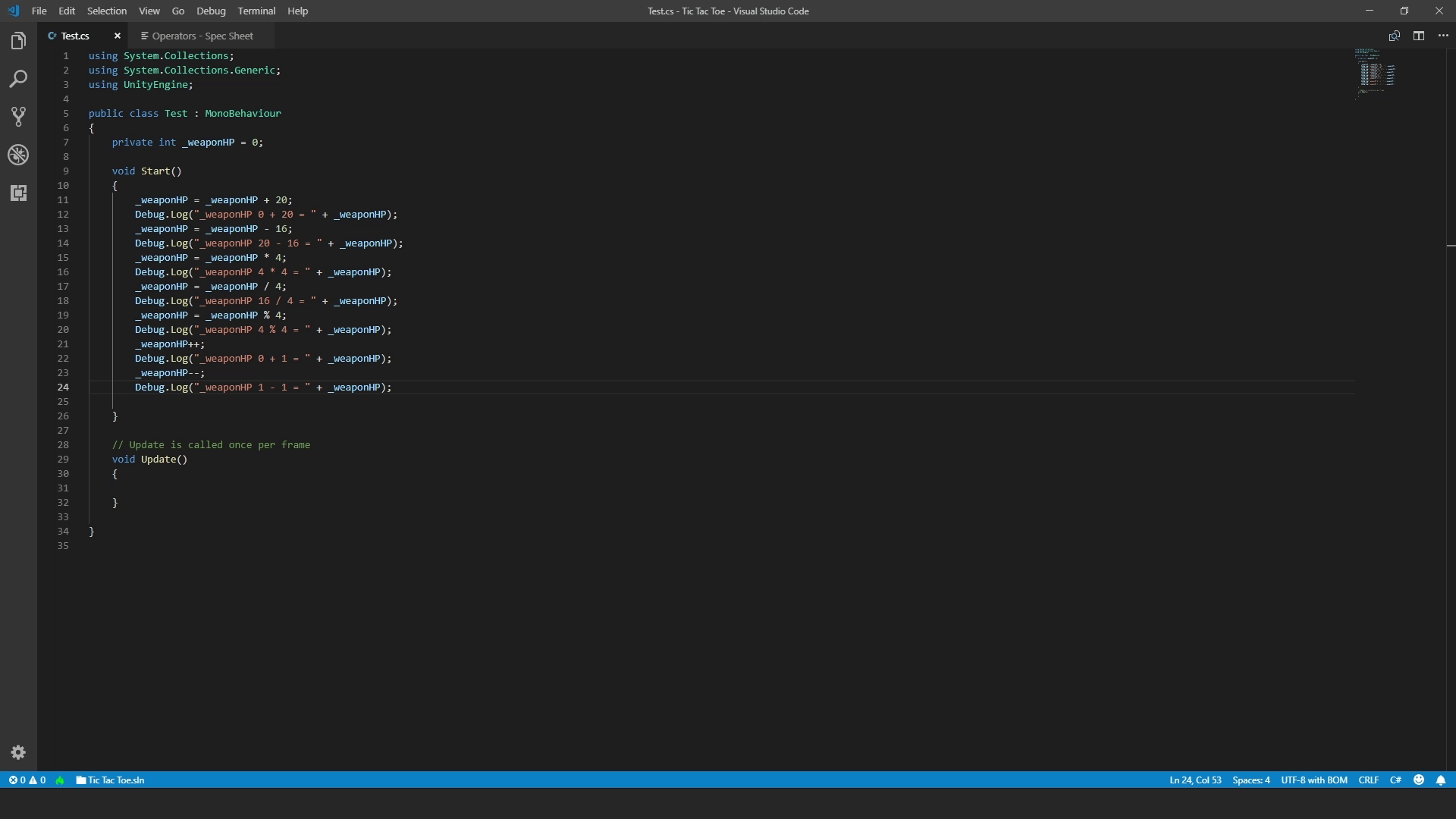
As you can see performing arithmetic operations on a variable is pretty simple.
Of course, when things are extremely simple it is easy to make silly mistakes.
But before telling you what to be cautious of, let’s execute this code in unity and look at the logs to confirm our outputs.
Unity Log
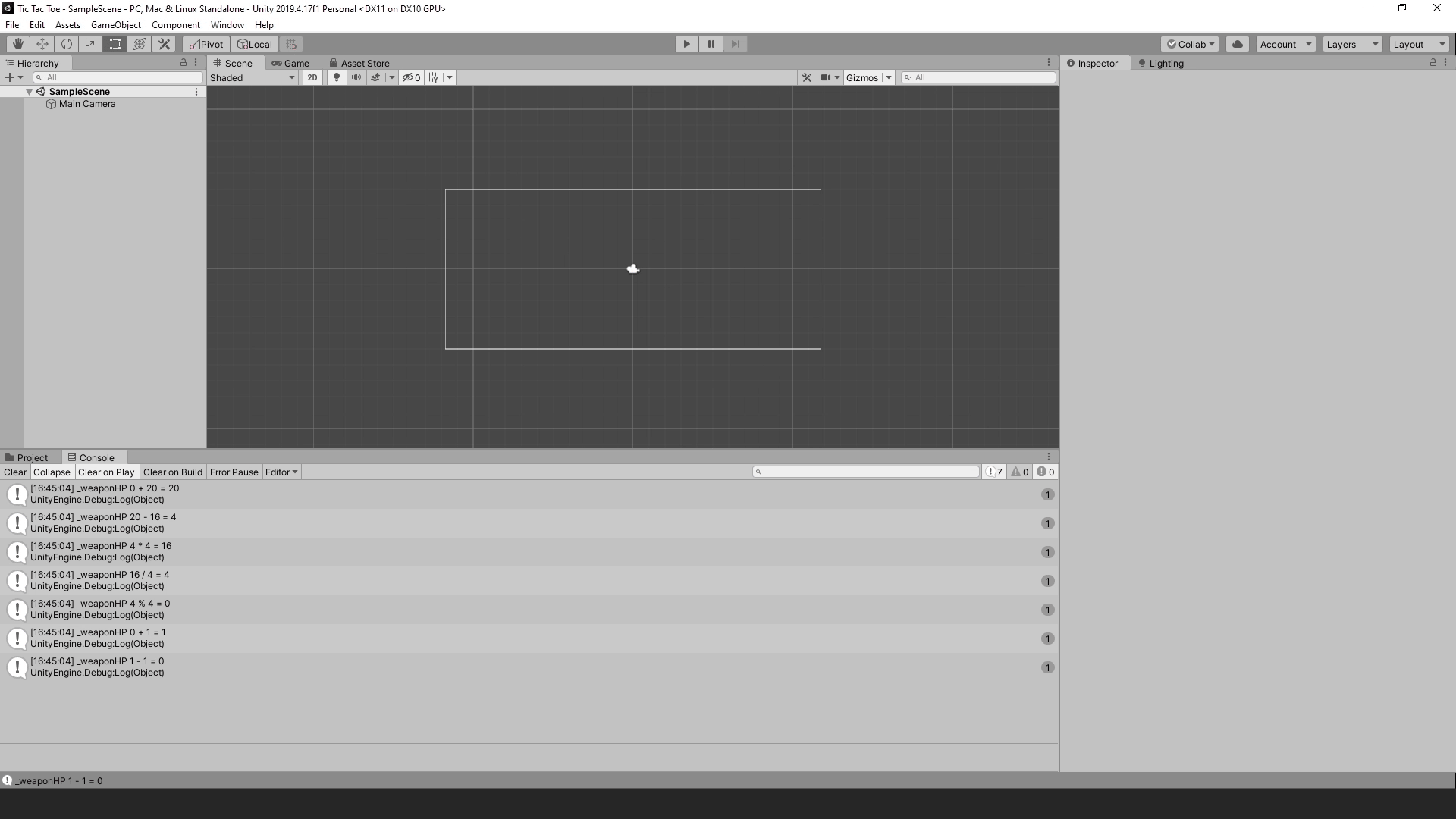
As you can see all the calculations in the code gave the expected output.
Things To Be Cautious About When Using Arithmetic Operators
#1 – Dividing An Int Value With Another Int Value
Now, back to the code, let me show you one of the mistakes you will make as a complete beginner, that at first sight, might seem correct.
So, in the Start method, let’s perform division again
// Everything Below is inside a Class.
private int _weaponHP = 0;
void Start()
{
//...
//... Not Showing The Code Above
//...
_weaponHP = 5 / 2;
Debug.Log("_weaponHP = " + _weaponHP);
}
Now looking at _weaponHP = 5 / 2, you would probably expect that the answer will be 2.5
but you would be wrong if you think that.
When you divide an int value with another int value, the resulting value will also be an int value,
which means that the resulting value will be a whole number, which 2.5 is not.
In this case, 5 / 2 should return 2
So, let’s execute this code in unity and check the logs.
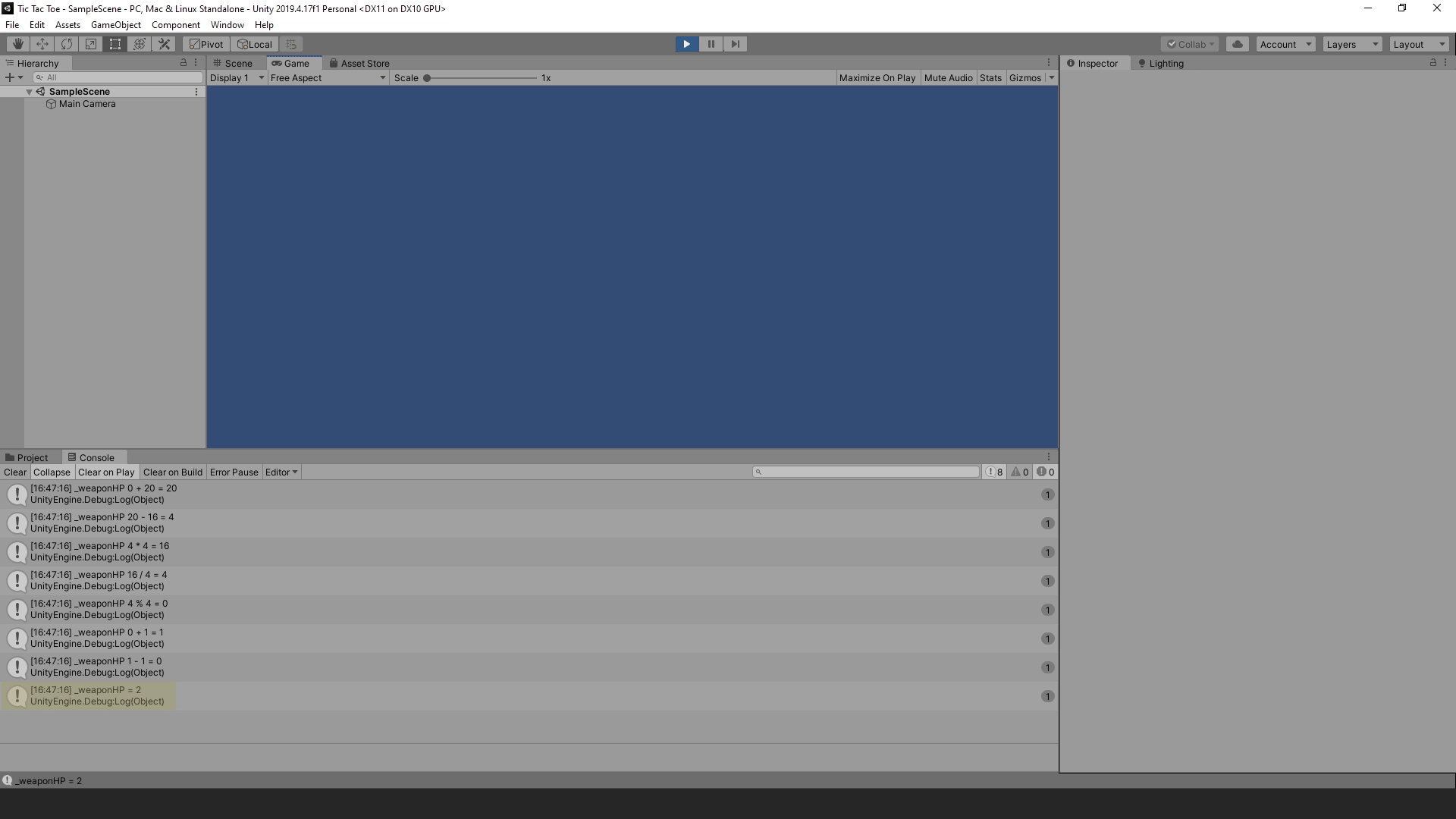
As you can see, the log says _weaponHP is 2.
Will This Behavior Change If We Store The Resulting Value Of An Arithmetic Operation in A Float Variable Instead Of An Int Variable?
Back to the code, now let’s initialize a float variable and do the same calculation.
// Everything Below is inside a Class.
private int _weaponHP = 0;
private float _swordHP = 0f;
void Start()
{
//...
//... Not Showing The Code Above
//...
_weaponHP = 5 / 2;
Debug.Log("_weaponHP = " + _weaponHP);
_swordHP = 5 / 2;
Debug.Log("_swordHP = " + _swordHP);
}
Now some of you might be expecting that because _swordHP is a variable of float data type,
when 5 is divided by 2, _swordHP will be assigned with 2.5 and not 2.
So, Let’s look at the Unity Logs again to see if you are correct.
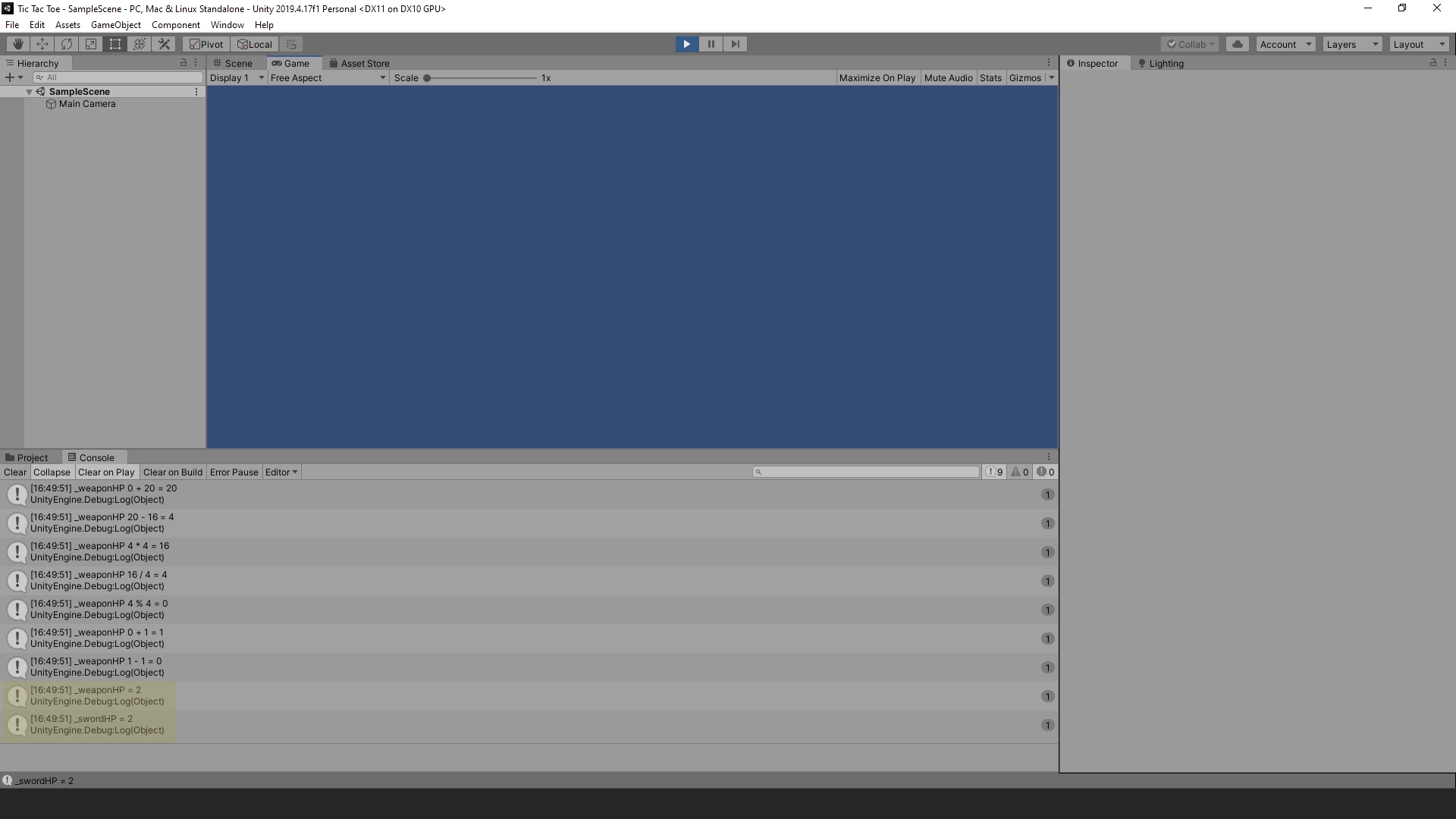
As you can see the logs says that _weaponHP and _swordHP are both 2
Explanation On Why This Is Happening
Now let me explain what is going on.
As i said before when you divide an int value by another int value, the resulting value will also be an int value.
So, you can take it as, the only thing that matters before the assignment is done is the Data Types of the Operands being operated on.
That is the Data Types of Operands 5 and 2, that are on the right side of equal to ( = ).
#2 – Dividing An Int Value With A Float Value
Now, if we convert one of these operands to float instead of int by suffixing f after it, like this,
// Everything Below is inside a Class.
private int _weaponHP = 0;
private float _swordHP = 0f;
void Start()
{
//...
//... Not Showing The Code Above
//...
_weaponHP = 5f / 2;
Debug.Log("_weaponHP = " + _weaponHP);
_swordHP = 5 / 2f;
Debug.Log("_swordHP = " + _swordHP);
}
then as you can see in the image below,
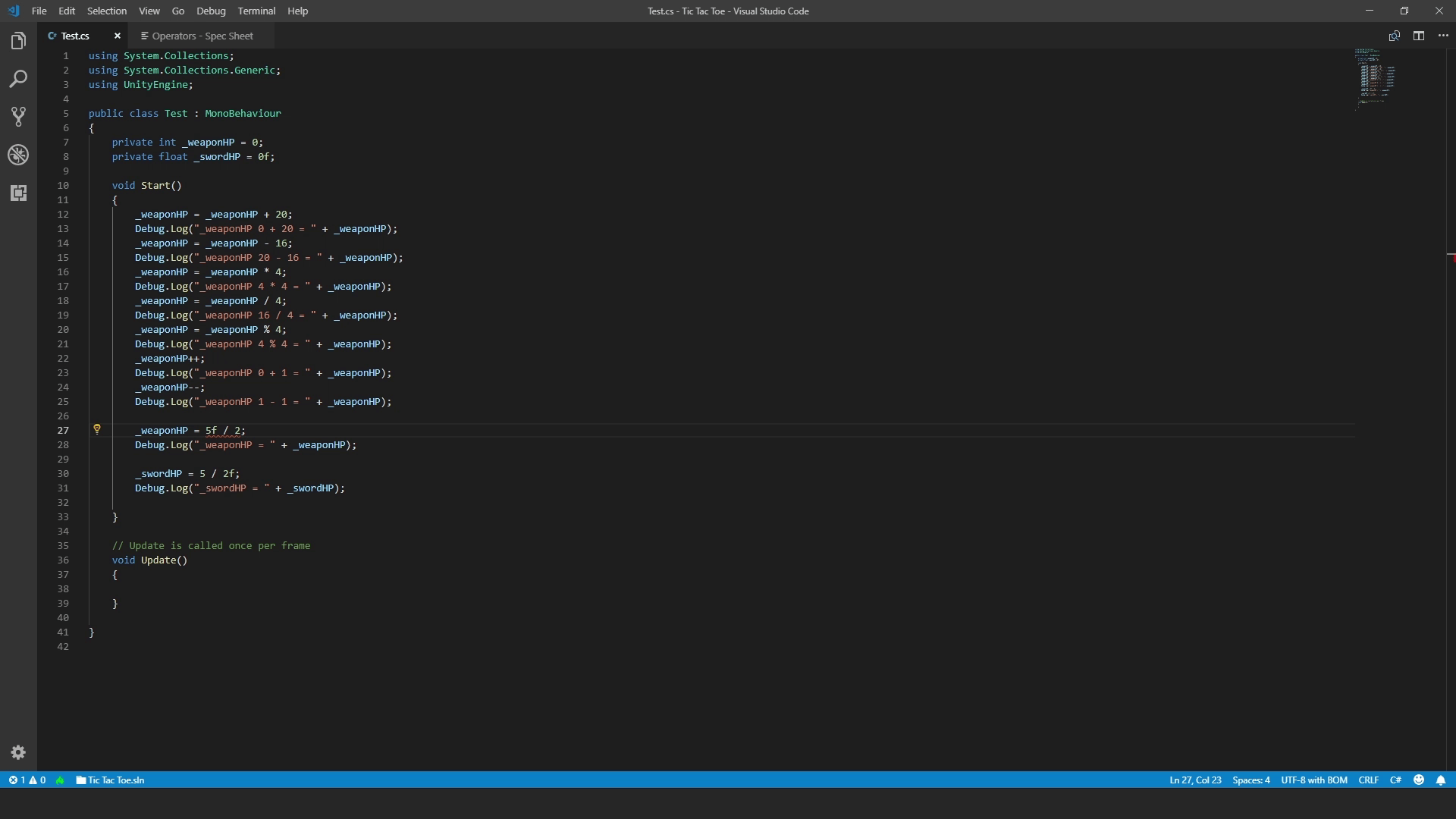
We got a red underline under one of them where we are calculating the value for _weaponHP.
While we don’t have this red underline where we are calculating the value for _swordHP.
Let’s hover over it and see what it says.
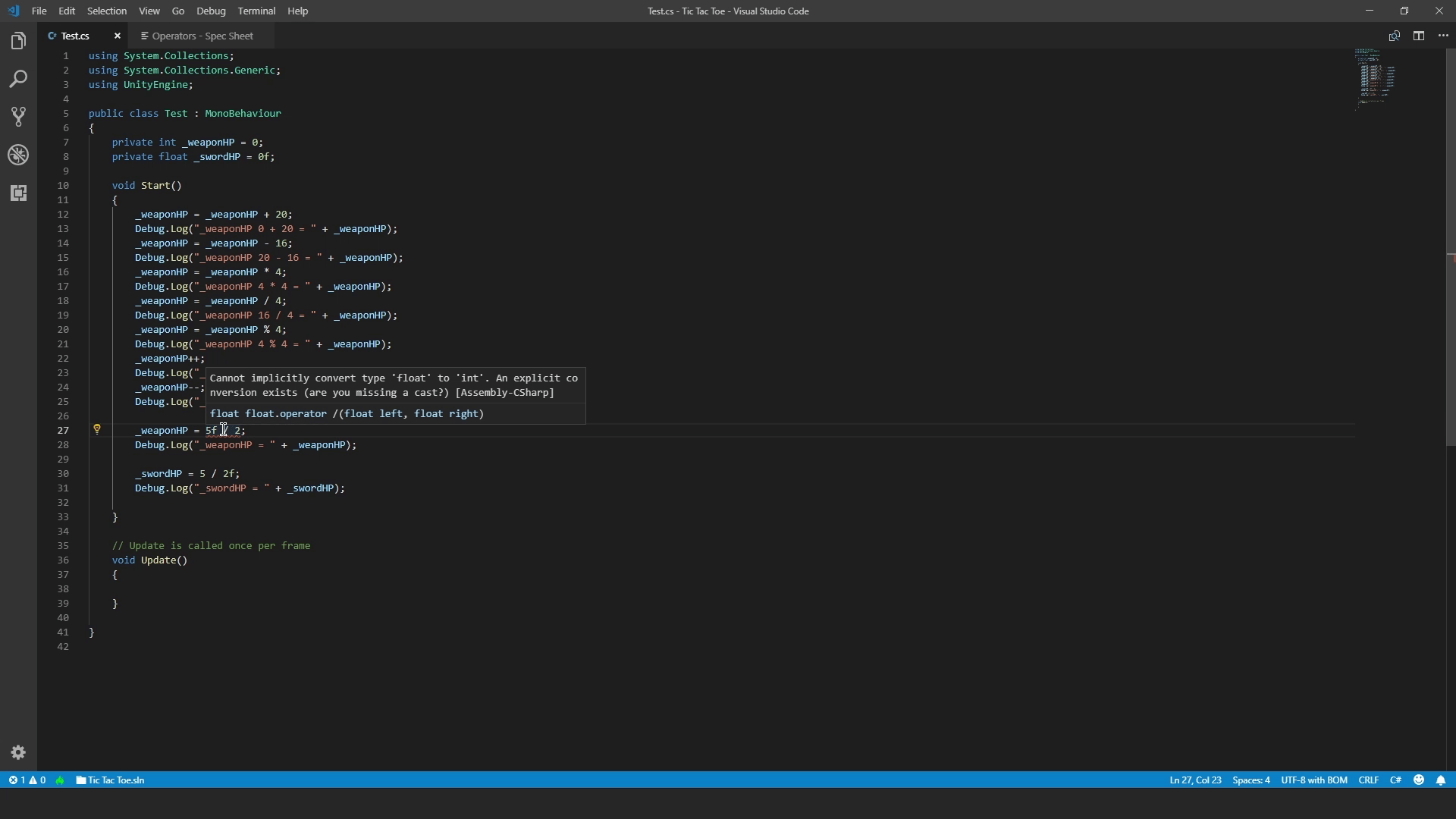
Cannot implicitly convert float to int. are you missing a cast?
This is because when you divide a float by an int, the resulting value will be a float value.
And as our variable _weaponHP is an int and not a float,
we can’t store the resulting value of float data type inside it, without converting the resulting value into an int value first.
The solution to this compile time error is to Typecast the resulting value to integer.
We can do this by surrounding the calculation with parenthesis and then typing (int) in front of it, like this.
// Everything Below is inside a Class.
private int _weaponHP = 0;
private float _swordHP = 0f;
void Start()
{
//...
//... Not Showing The Code Above
//...
_weaponHP = (int)(5f / 2);
Debug.Log("_weaponHP = " + _weaponHP);
_swordHP = 5 / 2f;
Debug.Log("_swordHP = " + _swordHP);
}
By doing so, we are explicitly telling the compiler to covert the resulting value which is float to int and will also remove the red underline that we are getting.
Now, let’s go back to unity and check the logs
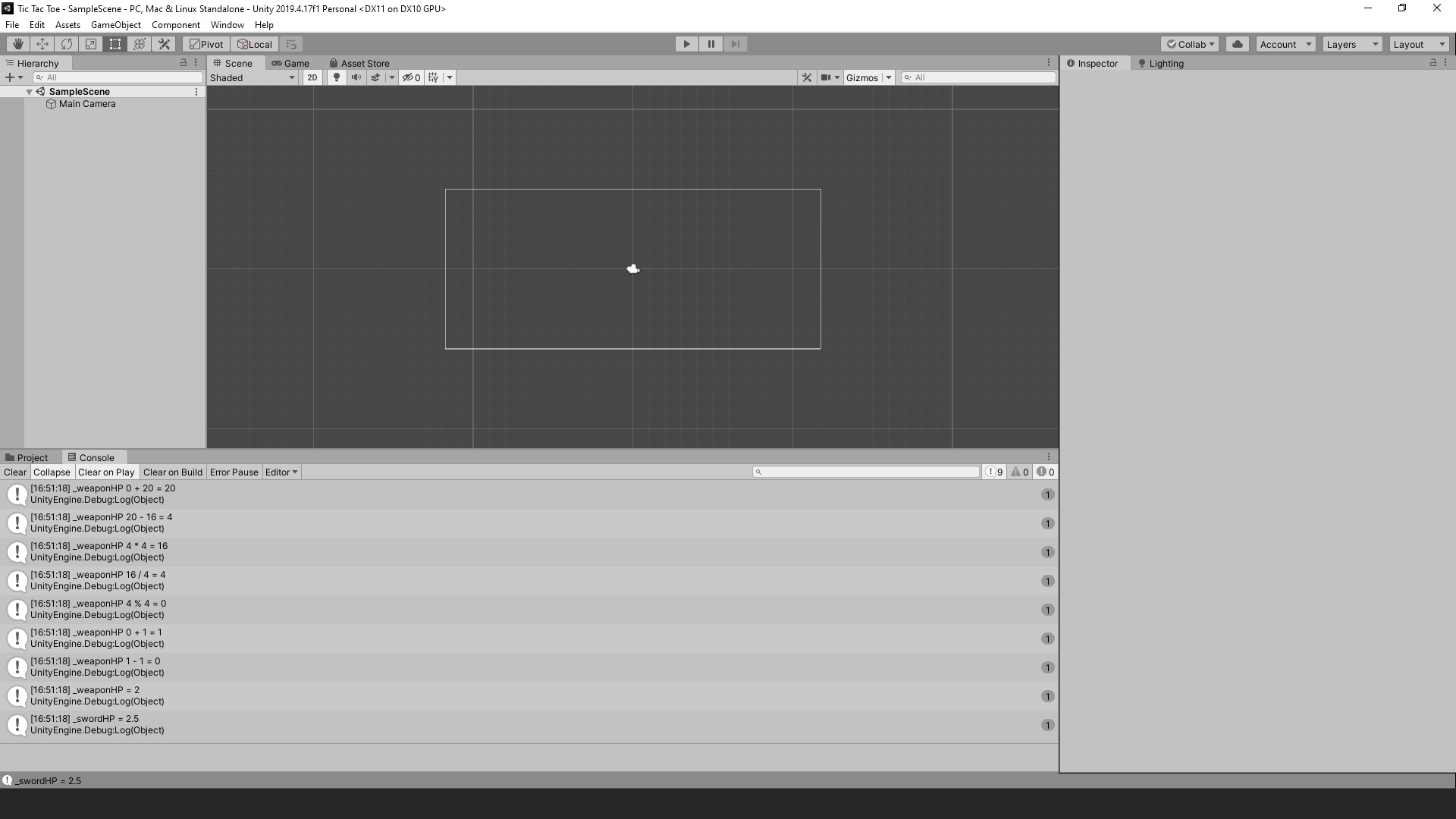
As you can see _weaponHP is 2 as we are typecasting the resulting value 2.5 to int
which is basically flooring 2.5 to 2 and then storing it in the _weaponHP variable.
while _swordHP is now 2.5 as we dividing a float value with an int value, which will make the resulting value a float value.
Another Use Of The Plus / Addition Arithmetic Operator
Man! that took a long time.
One last to cover is that the Plus / Addition Operator can also be used to join strings with other strings or to join strings with data inside variables.
As some of you might have noticed, when I’m logging things to the unity console,
Debug.Log("_weaponHP = " + _weaponHP);
I’m using the Plus / Addition Operator to join the string to the variable that I’m logging the value of.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords