Polymorphism is a Concept that comes into play when we start using Inheritance in our code
and using this concept correctly can give Game Developers the ability to alter Inherited Methods to better suite the Derived Class.
In this Article, we will take a close look at the Concept of Polymorphism to understand what exactly it is
and we will do it practically with an RPG Analogy about the Player having Multiple Types of Bows as his / her weapon.
So, without further ado, let’s get started!
This Post is Part of : How To Make A Game Series where I’ll be showing you how to become a game developer and will be going over the basics of Game Development, Step by Step, using the Unity Game Engine and C# Scripting Language to script the Game Logic.
If you are new to Game Development or Programming in general you should definitely go through this Unity Tutorials article series as it will set give you a kick-start towards becoming an exceptional Unity 2D or 3D Video Game Developer.
The Audience of your Game i.e. your Target Player Base is one of the most important factor when your are Designing and Developing a Game
Knowing who they are, their like, dislikes and their expectations from your Game & you as a Game Developer
can help you formulate a roadmap for you game and make important Game Design decisions.
So to help you in doing just that, I’ve written this article on Understanding The Target Player Base Of Your Game
that highlights some questions that you should ask yourself about your potential player base
so that you are focusing your time and efforts in the right places.
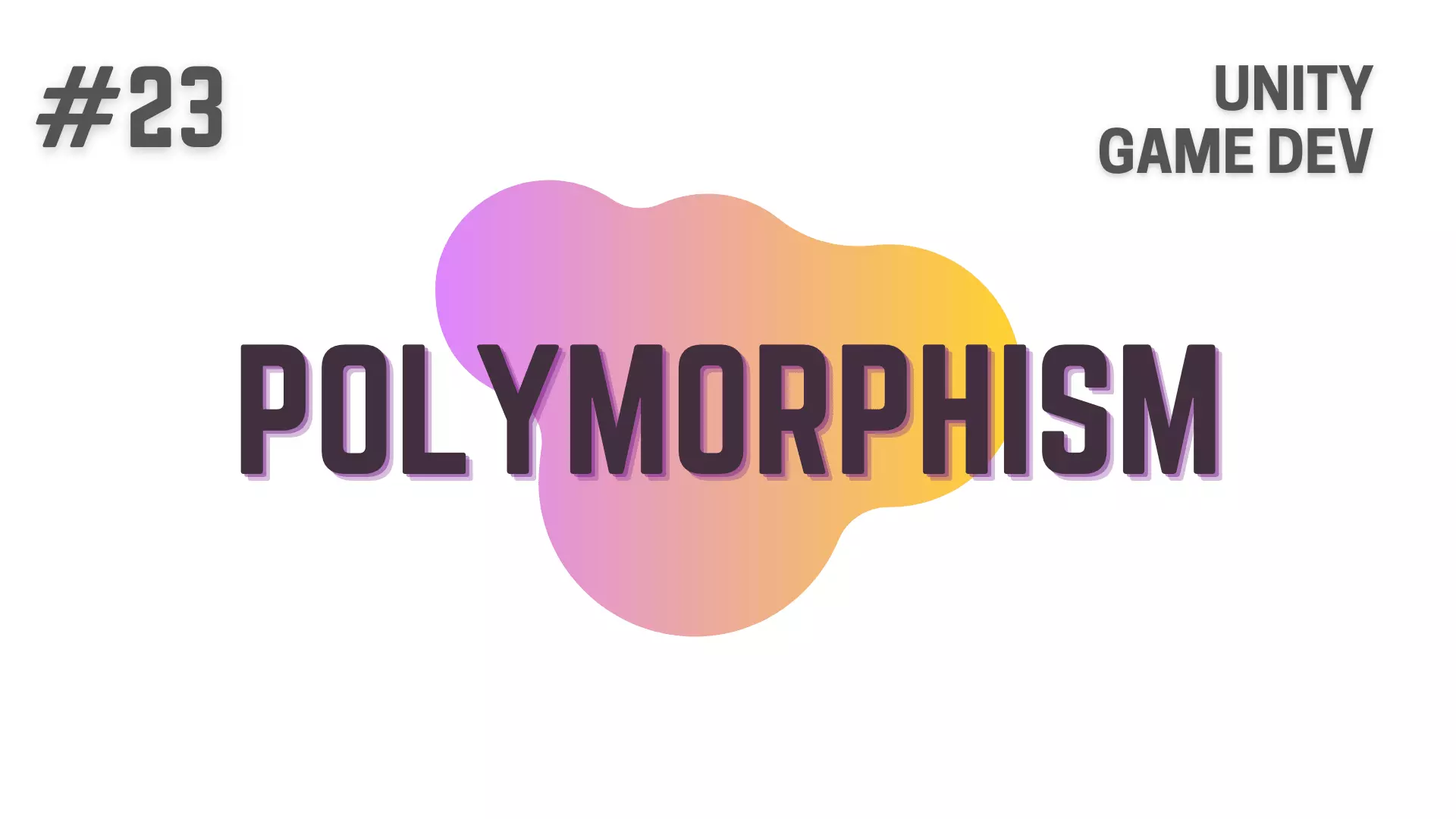
What is Polymorphism in C# | Unity Game Development Tutorial
When we use inheritance in our code, we inherit Variables & Methods from the Base Class into the Derived Class.
Now sometimes based on the problem that we are working on,
we would want the Inherited Methods to work a bit differently then it did in the Base Class.
To give more context, let’s go to the RPG analogy
and think about a game where the player wields various sort of Ranged Weapons like
- Crossbow
- Longbow
- and Ballista
All these three Ranged Weapons will be controlled by the player to attack his enemies
and will need to have different executions specific to each of these weapons
as they will have different Range, Fire Power, Damage, Animation, etc
that needs to be coded for each specific weapon.
Now all these 3 weapons are Types of Bows and will inherit from weapon_bow Class,
which will have the Shoot Method defined within it.
This Shoot Method through Inheritance will be derived in the specific scripts for each of the Bows i.e.
- bow_crossbow
- bow_longbow
- and bow_ballista
and as all the weapons will have different Range, Fire Power, Damage, Animation, etc
we will need to modify what the Shoot Method does for each of the specific Bows
by modifying the execution of the derived Shoot Method
and make it more specific to the weapon in question.
So All in All What is Polymorphism? | Unity Game Development Tutorial
All in all Polymorphism in the context of programming can be considered as a lingo for saying that
We will take the Methods defined within the Base Class
and then inside the Derived Classes of that Base Class
we will modify it’s execution i.e. it’s code block
to do different things based on the Derived Class in question.
What this allows us to do is perform a single action in multiple different ways.
In case of our RPG Analogy, we will define the Action named Shoot by defining a Method in the Base Class
and then Inherit it in all the Derived Classes and modify it’s execution in each of the Derived Class.
We will do this without changing the name of the method, as even though the execution is different for each Class
the action that we are performing is still just shooting an arrow.
So with that done, let’s dive directly into the code
Polymorphism in C# | Code Example | Unity Game Development Tutorial
First of all we will need to go to Unity and create the necessary C# Scripts i.e.
- Base class : weapon_bow
- Derived Classes : bow_crossbow, bow_longbow and bow_ballista
With that done, let’s open the weapon_bow C# Script in VS Code
Now to show Polymorphism, we will need to use the virtual and override modifiers / Keywords
with the Methods whose execution we want want to modify in our Base Class and the Derived Classes respectively.
So let’s go and define the Shoot Method
And as weapon_bow is our Base Class we will add the virtual modifier to the Shoot Method as shown below.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_bow : MonoBehaviour
{
public virtual void Shoot()
{
Debug.Log("weapon_bow shot an arrow!");
}
}
With that done, finally let’s call the Shoot Method in Start Method, so that the log inside will be printed in the Unity Console
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class weapon_bow : MonoBehaviour
{
void Start()
{
Shoot();
}
public virtual void Shoot()
{
Debug.Log("weapon_bow shot an arrow!");
}
}
Now let’s go to the bow_crossbow C# Script and modify it,
so that it will inherit from weapon_bow Class.
If you don’t know how Inheritance works in C# you can go through this article on Inheritance in which I’ve explained the concept in detail.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class bow_crossbow : weapon_bow
{
}
With Inheritance working correctly, we will define the Shoot Method in bow_crossbow Class again
but this time instead of the virtual keyword, we will use the override keyword with the Shoot Method.
and After that, let’s go to the Start Method and make a call to the Shoot Method
so that we can see the log in Unity Console.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class bow_crossbow : weapon_bow
{
void Start()
{
Shoot();
}
public override void Shoot()
{
Debug.Log("bow_crossbow shot an arrow!");
}
}
With that done, now let’s quickly do the same for the bow_longbow and bow_ballista C# Scripts.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class bow_longbow : weapon_bow
{
void Start()
{
Shoot();
}
public override void Shoot()
{
Debug.Log("bow_longbow shot an arrow!");
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class bow_ballista : weapon_bow
{
void Start()
{
Shoot();
}
public override void Shoot()
{
Debug.Log("bow_ballista shot an arrow!");
}
}
With that we have correctly implemented Polymorphism in all of our Scripts.
Now let’s go to Unity and check the logs.
Don’t forget to attach all the four C# Scripts to Game Objects in your Unity Scene!
Unity Log | Polymorphism In C# | Unity Game Development Tutorial
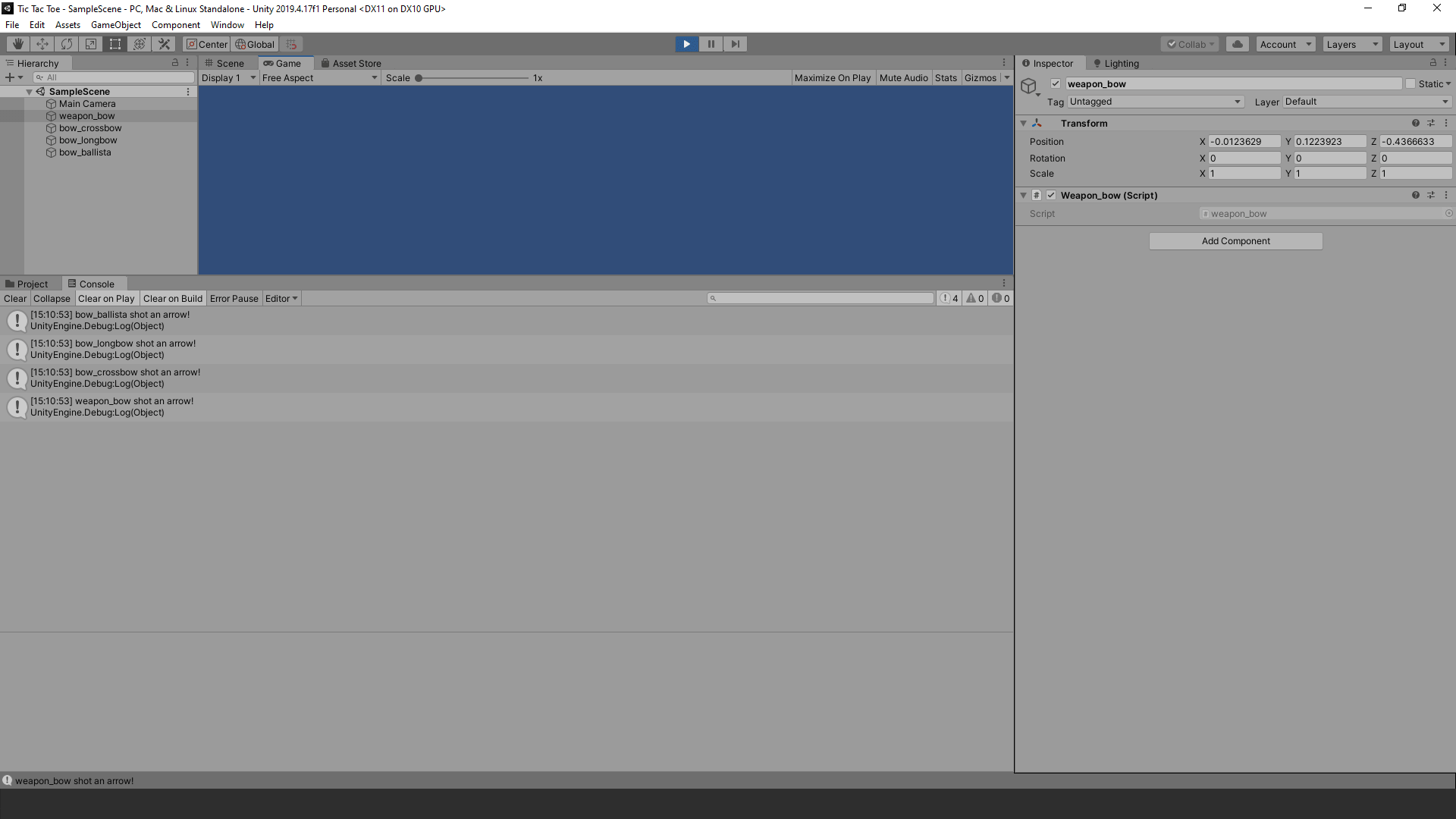
As you can see in the Unity Console,
Though we made a method call with the same method name i.e. Shoot, in all the 4 Classes
the logs are showing completely different output based on each specific bows.
Conclusion
Well folks, that does it for this article.
i hope that this information will be helpful to you.
Share this post and follow us on Social Media platforms if you think our content is great, so that you never lose the source of something you love.
If you like the content do go through the various articles in How To Make A Game Series that this post is a part of and also go through the other series we have on Bite Sized Tech.
Also we have a YouTube Channel : Bite Sized Tech where we upload Informational Videos and Tutorials like this regularly. So, if you are interested do subscribe and go through our Uploads and Playlists.
Follow Us On Social Media
Goodbye for now,
This is your host VP
Signing off.
Articles In How To Make A Game Series
Game Development Softwares (Game Engines, 2D Art, 3D Art, Code Editor, DAW)
Methods | Part 1 – Basics of Methods
Methods | Part 2 – Multiple, Required & Optional Parameters + Named Arguments
Methods | Part 3 – Overload Resolution
Methods | Part 4 – In, Ref and Out Keywords